You will write a program that will read data from a file that contains countries that export oil and the percentage of the world's oil supply exported by those countries. The data will be stored in two separate one- dimensional arrays and used to display the country the exports the smallest percentage of the world's oil supply. Instructions: • Add the import java.util.*; and import java.io.*; statements before the class and create a main program inside the class. • In the main program, do the following: o Create a Scanner object that will be associated with a file named oil.txt. Each line of this file contains the name of a country and the percentage of the world's oil supply exported by the country. o Define a String array with 15 elements that will contain the names of the countries. o Define a double array with 15 elements that will contain the percentages of the world's oil supply exported by each country. o Call a void method named readData that has three parameters: a Scanner, a String array, and a double array. o Call a method named findSmallest that returns a String and has three parameters: a String array, a double array, and an integer for the length of the arrays. • The readData method should use a while loop to store the name of each country in the String array and store the percentage for each country in the double array. • The findSmallest method should use a loop of any type to find the smallest percentage in the double array and return a string containing both the country with the smallest percentage and the percentage for that country. Here is a sample output: Venezuela exports the smallest percentage of oil at 2.31%.
You will write a program that will read data from a file that contains countries that export oil and the percentage of the world's oil supply exported by those countries. The data will be stored in two separate one- dimensional arrays and used to display the country the exports the smallest percentage of the world's oil supply. Instructions: • Add the import java.util.*; and import java.io.*; statements before the class and create a main program inside the class. • In the main program, do the following: o Create a Scanner object that will be associated with a file named oil.txt. Each line of this file contains the name of a country and the percentage of the world's oil supply exported by the country. o Define a String array with 15 elements that will contain the names of the countries. o Define a double array with 15 elements that will contain the percentages of the world's oil supply exported by each country. o Call a void method named readData that has three parameters: a Scanner, a String array, and a double array. o Call a method named findSmallest that returns a String and has three parameters: a String array, a double array, and an integer for the length of the arrays. • The readData method should use a while loop to store the name of each country in the String array and store the percentage for each country in the double array. • The findSmallest method should use a loop of any type to find the smallest percentage in the double array and return a string containing both the country with the smallest percentage and the percentage for that country. Here is a sample output: Venezuela exports the smallest percentage of oil at 2.31%.
Chapter16: Graphics
Section: Chapter Questions
Problem 1DE
Related questions
Question
100%
Need help with my intro to java assignment, would appreiciate it if the code is in simply terms. Thanks in advance, the oil txt is below.

Transcribed Image Text:You will write a program that will read data from a file that contains
countries that export oil and the percentage of the world's oil supply
exported by those countries. The data will be stored in two separate one-
dimensional arrays and used to display the country the exports the smallest
percentage of the world's oil supply.
Instructions:
• Add the import java.util.*; and import java.io.*; statements before
the class and create a main program inside the class.
• In the main program, do the following:
o Create a Scanner object that will be associated with a file
named oil.txt. Each line of this file contains the name of a
country and the percentage of the world's oil supply exported
by the country.
o Define a String array with 15 elements that will contain the
names of the countries.
o Define a double array with 15 elements that will contain the
percentages of the world's oil supply exported by each country.
o Call a void method named readData that has three parameters:
a Scanner, a String array, and a double array.
o Call a method named findSmallest that returns a String and
has three parameters: a String array, a double array, and an
integer for the length of the arrays.
• The readData method should use a while loop to store the name of
each country in the String array and store the percentage for each
country in the double array.
• The findSmallest method should use a loop of any type to find the
smallest percentage in the double array and return a string containing
both the country with the smallest percentage and the percentage for
that country. Here is a sample output:
Venezuela exports the smallest percentage of oil at 2.31%.

Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
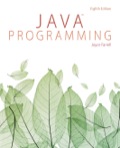
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
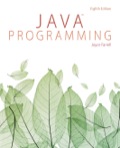
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage