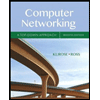
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Continuing from the example, modify the program so that it outputs the average grade in each course, and also shows which course has the highest average grade.
import java.util.Scanner;
public class Main
{
publicstaticvoid main(String[] args)
{
Scanner s = new Scanner(System.in);
int numCourses, numTests;
double[][] testScores;
System.out.print("How many courses are you taking? ");
numCourses = s.nextInt();
testScores = new double[numCourses][];
// allocate an array of references to 1-D arrays (rows)
for(int i = 0; i < numCourses; i++)
{
System.out.print("How many tests are in course number "
+ (i+1) + "? ");
numTests = s.nextInt();
// allocate this row: a 1-D array of doubles:
testScores[i] = new double[numTests];
}
for(int courseId=0; courseId < testScores.length; courseId++)
{
for(int testId=0; testId < testScores[courseId].length; testId++)
{
System.out.print("What was your grade on test "
+ (testId+1) + " for course "
+ (courseId+1) + "? ");
testScores[courseId][testId] = s.nextDouble();
}
System.out.println(); // blank line to separate courses
}
}
}
/* Sample Output:
How many courses are you taking? 5
How many tests are in course number 1? 4
How many tests are in course number 2? 2
How many tests are in course number 3? 1
How many tests are in course number 4? 3
How many tests are in course number 5? 2
What was your grade on test 1 for course 1? 80
What was your grade on test 2 for course 1? 88
What was your grade on test 3 for course 1? 92
What was your grade on test 4 for course 1? 79
What was your grade on test 1 for course 2? 78
What was your grade on test 2 for course 2? 77
What was your grade on test 1 for course 3? 81
What was your grade on test 1 for course 4? 69
What was your grade on test 2 for course 4? 75
What was your grade on test 3 for course 4? 79
What was your grade on test 1 for course 5? 90
What was your grade on test 2 for course 5? 95
*/
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Double any element's value that is less than controlValue. Ex: If controlValue = 10, then dataPoints = {2, 12, 9, 20} becomes {4, 12, 18, 20}. import java.util.Scanner; public class StudentScores { public static void main (String [] args) { Scanner scnr = new Scanner (System.in); final int NUM_POINTS = 4; int [] dataPoints new int [NUM_POINTS]; int controlValue; int i; controlValue = scnr. nextInt (); for (i = 0; i < dataPoints.length; ++i) { = scnr.nextInt (); dataPoints [i] } /* Your solution goes here * / for (i = 0; i < dataPoints.length; ++i) { System.out.print (dataPoints[i] + %3D " ") ; } System.out.println ();arrow_forwardimport java.util.Scanner; public class MealEstablishmentDirectory { public static void main (String[] args) { Scanner scnr = new Scanner(System.in); int newRating; String newState; MealEstablishment mealEstablishment1 = new MealEstablishment(); System.out.println("Default values: "); mealEstablishment1.print(); newRating = scnr.nextInt(); newState = scnr.next(); mealEstablishment1.setRating(newRating); mealEstablishment1.setState(newState); System.out.println("After mutator methods: "); mealEstablishment1.print(); } }arrow_forwardFind output. //filename: Test.java class Test{ int x = 10; public static void main(String[] args) { Test t = new Test(); System.out.println(t.x); } }arrow_forward
- using System; class main { publicstaticvoid Main(string[] args) { int number = 1; while (number <= 88) { int i; Random r = new Random(); int[] randNo=newint[3]; for(i=0;i<3;i++) { randNo[i]= r.Next(1,88); Console.WriteLine("Random Number between 1 and 88 is "+randNo[i]); } } } } This code is supposed to count from 1-88 and then select three random numbers from the list but instead it generates an infinite loop of random numers between 1-88. how can it be fixed?arrow_forwardFix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forwardCreate a Flowchart //Java Source Code :- import java.util.Scanner; public class bExpert { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.println("\nVALUES"); System.out.print("Value 1: "); int v1 = sc.nextInt(); System.out.print("Value 2: "); int v2 = sc.nextInt(); System.out.print("Value 3: "); int v3 = sc.nextInt(); System.out.println("\nPROCESS"); System.out.println("\n1. Summation"); System.out.println("2. Product"); System.out.println("3. Min / Max"); System.out.println("4. Odd / Even"); char st = 'y'; while(st != 'n'){ System.out.print("\nCHOICE: "); int ch = sc.nextInt(); switch(ch) { case 1: int sum = v1 + v2 + v3; System.out.println("\nSum = "+sum); break; case 2: int prod =…arrow_forward
- Insert XXX to output the student's ID. public class Student { private double myGPA; private int myID; public getIDC ) { return myID; } public static void main(String [] args) { Student s = new Student(); XXX } } System.out.println("Student ID: " + getID( )); System.out.printIn("Student ID: "); System.out.printIn("Student ID: " + s.getID( )); %3D System.out.printIn("Student ID: " + student.getID(0);arrow_forwardQuestion 2. package sortsearchassigncodeex1; import java.util.Scanner; import java.io.*; // // public class Sortsearchassigncodeex1 { // public static void fillArray(Scanner inputFile, int[] arrIn){ int indx = 0; //Complete code { arrIn[indx] = inputFile.nextInt(); indx++; } }arrow_forwardJAVA CODE: check outputarrow_forward
- import java.util.Scanner; public class TriangleArea { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Triangle triangle1 = new Triangle(); Triangle triangle2 = new Triangle(); // TODO: Read and set base and height for triangle1 (use setBase() and setHeight()) // TODO: Read and set base and height for triangle2 (use setBase() and setHeight()) System.out.println("Triangle with smaller area:"); // TODO: Determine smaller triangle (use getArea()) // and output smaller triangle's info (use printInfo()) }} public class Triangle { private double base; private double height; public void setBase(double userBase){ base = userBase; } public void setHeight(double userHeight) { height = userHeight; } public double getArea() { double area = 0.5 * base * height; return area; } public void printInfo() { System.out.printf("Base:…arrow_forwardimport java.util.Scanner; public class StateInfo { /* Your code goes here */ public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String stateCode; String stateName; stateCode = scnr.next(); stateName = scnr.next(); printStateInfo(stateCode, stateName); }}arrow_forwardimport java.util.Scanner; public class CharMatch { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); String userString; char charToFind; int strIndex; userString = scnr.nextLine(); charToFind = scnr.next().charAt(0); strIndex = scnr.nextInt(); /* Your code goes here */ }}arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
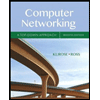
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
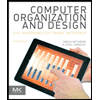
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
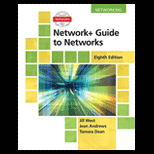
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
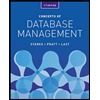
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
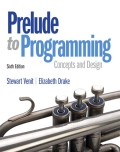
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
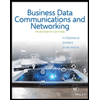
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY