You will write a program to process the lines in a text file using a linked list ADT and raw pointers. Node class You will create a class “Node” with the following private data attributes: line – line from a file (string) next - (pointer to a Node) Put your class definition in a header file and the implementation of the methods in a .cpp file. Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods: Accessors and mutators for each attribute Constructor that initializes the attributes to nulls (empty string and nullptr) LinkedList class You will create a class “LinkedList” with the following private data attributes: headPtr – raw pointer to the head of the list numItems – number of items in the list Put your class definition in a header file and the implementation of the methods in a .cpp file. Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods: Accessor to get the current size (numItems) Constructor that initializes numItems to zero and headPtr to nullptr Add a node – this method will take as input one string value. It will then create a node object and set the attribute. Then it will put it in the linked list at the correct position – ascending order – avoiding duplicates. You will do all of the work while building the linked list. toVector – returns vector with the contents of the list. You will use only the “push_back” method to get the strings into the vector. Client program Your program will ask the user for the file name, open the text file , read each line and invoke the addNode method. Make sure the file opened correctly before you start processing it. You do not know how many lines are in the file so your program should read until it gets to the end of the file. It will display the contents of the list (the lines that were read from the file in sorted order with no duplicates) using the LinkedList class method “toVector” that puts all of the lines into a vector and returns it to the program that will display it. Your client program will do the following: Read file name from user Display the number of lines that were read out of the file and were passed to the addNode method. Display the lines in your linked list using the class method toVector to get the lines. Display the number of lines in the vector. I have attached three files you can use to test your program. Blackboard handles files in a strange way sometimes. You may not be able to directly download the files, but you can open them, copy and paste the contents into a notepad file and save that file. You will write all of the code for processing the linked list - do not use any predefined objects in C++. You do not need to offer a user interface – simply display the number of lines read from the file, the list using the “toVector” method and the number of lines in the vector.
You will write a program to process the lines in a text file using a linked list ADT and raw pointers. Node class You will create a class “Node” with the following private data attributes: line – line from a file (string) next - (pointer to a Node) Put your class definition in a header file and the implementation of the methods in a .cpp file. Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods: Accessors and mutators for each attribute Constructor that initializes the attributes to nulls (empty string and nullptr) LinkedList class You will create a class “LinkedList” with the following private data attributes: headPtr – raw pointer to the head of the list numItems – number of items in the list Put your class definition in a header file and the implementation of the methods in a .cpp file. Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file. You will have the following public methods: Accessor to get the current size (numItems) Constructor that initializes numItems to zero and headPtr to nullptr Add a node – this method will take as input one string value. It will then create a node object and set the attribute. Then it will put it in the linked list at the correct position – ascending order – avoiding duplicates. You will do all of the work while building the linked list. toVector – returns vector with the contents of the list. You will use only the “push_back” method to get the strings into the vector. Client program Your program will ask the user for the file name, open the text file , read each line and invoke the addNode method. Make sure the file opened correctly before you start processing it. You do not know how many lines are in the file so your program should read until it gets to the end of the file. It will display the contents of the list (the lines that were read from the file in sorted order with no duplicates) using the LinkedList class method “toVector” that puts all of the lines into a vector and returns it to the program that will display it. Your client program will do the following: Read file name from user Display the number of lines that were read out of the file and were passed to the addNode method. Display the lines in your linked list using the class method toVector to get the lines. Display the number of lines in the vector. I have attached three files you can use to test your program. Blackboard handles files in a strange way sometimes. You may not be able to directly download the files, but you can open them, copy and paste the contents into a notepad file and save that file. You will write all of the code for processing the linked list - do not use any predefined objects in C++. You do not need to offer a user interface – simply display the number of lines read from the file, the list using the “toVector” method and the number of lines in the vector.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
You will write a program to process the lines in a text file using a linked list ADT and raw pointers.
Node class
You will create a class “Node” with the following private data attributes:
- line – line from a file (string)
- next - (pointer to a Node)
Put your class definition in a header file and the implementation of the methods in a .cpp file.
Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file.
You will have the following public methods:
- Accessors and mutators for each attribute
- Constructor that initializes the attributes to nulls (empty string and nullptr)
LinkedList class
You will create a class “LinkedList” with the following private data attributes:
- headPtr – raw pointer to the head of the list
- numItems – number of items in the list
Put your class definition in a header file and the implementation of the methods in a .cpp file.
Follow the style they use in the book of having a "#include" for the implementation file at the bottom of the header file.
You will have the following public methods:
- Accessor to get the current size (numItems)
- Constructor that initializes numItems to zero and headPtr to nullptr
- Add a node – this method will take as input one string value. It will then create a node object and set the attribute. Then it will put it in the linked list at the correct position – ascending order – avoiding duplicates. You will do all of the work while building the linked list.
- toVector – returns
vector with the contents of the list. You will use only the “push_back” method to get the strings into the vector.
Client program
Your program will ask the user for the file name, open the text file , read each line and invoke the addNode method. Make sure the file opened correctly before you start processing it. You do not know how many lines are in the file so your program should read until it gets to the end of the file.
It will display the contents of the list (the lines that were read from the file in sorted order with no duplicates) using the LinkedList class method “toVector” that puts all of the lines into a vector and returns it to the program that will display it.
Your client program will do the following:
- Read file name from user
- Display the number of lines that were read out of the file and were passed to the addNode method.
- Display the lines in your linked list using the class method toVector to get the lines.
Display the number of lines in the vector.
I have attached three files you can use to test your program. Blackboard handles files in a strange way sometimes. You may not be able to directly download the files, but you can open them, copy and paste the contents into a notepad file and save that file.
You will write all of the code for processing the linked list - do not use any predefined objects in C++. You do not need to offer a user interface – simply display the number of lines read from the file, the list using the “toVector” method and the number of lines in the vector.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
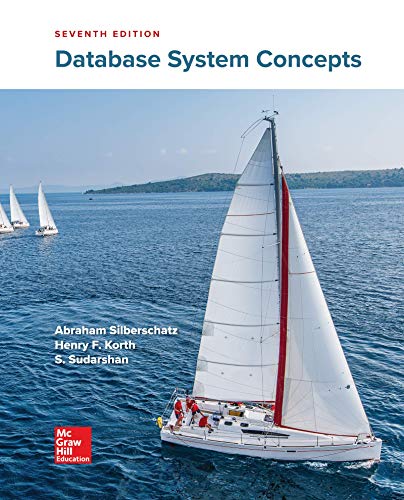
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
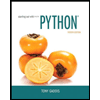
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
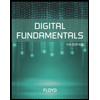
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
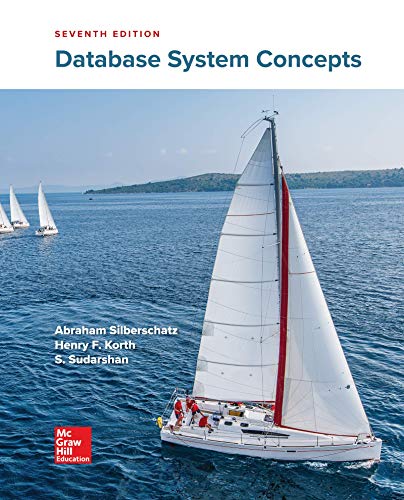
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
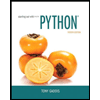
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
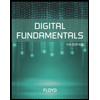
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
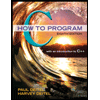
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
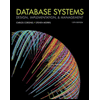
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
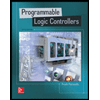
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education