3_ob85_meet
.docx
keyboard_arrow_up
School
Drexel University *
*We aren’t endorsed by this school
Course
540
Subject
Computer Science
Date
May 1, 2024
Type
docx
Pages
4
Uploaded by bbbtobi17
Tobi Bakare
04/19/2024
Week3Meet - 10 pts
Turn in on BBL as soon as complete, but before end of day Sunday following the lecture.
===============================
Reading a program
Read both programs. Be the computer and trace which line of code is executing in order. Keep track of what the current state of each variable is.
import java.util.Scanner;
/**********************************
* PuzzlePage.java
* Creates word puzzles for the Triangle
* @author Tammy Pirmann
* @version 20210407
*********************************/
public class PuzzlePage {
public static void main(String args[]){
Scanner keyboard = new Scanner(System.in);
WordScramble puzzle = new WordScramble();
System.out.print("Enter the solution word: ");
String solution = keyboard.nextLine();
System.out.printf("Your puzzle is: %s", puzzle.scramble(solution));
}
}
/*******************************
* WordScramble.java, Scrambles a given word
* @author Tammy Pirmann
* @version 20210407
*******************************/
public class WordScramble { private String solution;
//constructor
public WordScramble() {
solution = "NA";
}
//Setter
public void setSolution(String str){
solution = str.toUpperCase();
}
//Scrambles the solution String
public String scramble(String str){
setSolution(str);
String mix;
int a = solution.indexOf("A");
if (a >= 0) {
mix = solution.substring(a).concat(solution.substring(0,a));
}
int e = solution.indexOf("E");
if (e >= 0) {
mix = solution.substring(e).concat(solution.substring(0,e));
}
int i = solution.indexOf("I");
if (i >= 0) {
mix = solution.substring(i).concat(solution.substring(0,i));
}
int oh = solution.indexOf("O");
if (oh >= 0) {
mix = solution.substring(oh).concat(solution.substring(0,oh));
}
int u = solution.indexOf("U");
if (u >= 0) {
mix = solution.substring(u).concat(solution.substring(0,u));
}
//reverse it in case it still looks like the original word
return reverse(mix); }
//helper method to reverse the scrambled string
private String reverse(String str){
//base case
if (str.isEmpty()){
return str;
}
//Recursive call
return reverseString(str.substring(1)) + str.charAt(0);
}
}
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
X = 56
arrow_forward
Safari File Edit View History Bookmarks Window Help
= zyBooks
My library > CS 11: Introduction to Computer Science- C++ home >
1.19: LAB: Input and formatted output: Right-facing arrow
1.19 LAB: Input and formatted output: Right-facing arrow
Given two input integers for an arrow body and arrowhead (respectively), print a right-facing arrow.
Ex: If the input is:
01
the output is:
1
11
00000111
000001111
00000111
11
1
497630.3807866.qx3zqy7
LAB
ACTIVITY
learn.zybooks.com
1.19.1: LAB: Input and formatted output: Right-facing arrow
1 #include
2 using namespace std;
3
4 int main() {
5
6
7
8
9
10
11 }
int baseDigit;
int headDigit;
/* Type your code here. *,
return 0;
EzyBooks catalog
main.cpp
:i
0/10
((
Load default template...
Ơ
80
Fri Oct 13 3:27 AM
Help/FAQ kimberly sandoval
arrow_forward
read the question and answer it correctly
arrow_forward
Time during which a job is processed by the computer is
A.
Delay time
B.
Real time
C.
Execution time
D.
Down time
E.
None of the above
arrow_forward
C linux Program homework need help, dont give the wrong answer please, show the result and output plz.
also if you have definition that will help a lot
arrow_forward
a ng.cengage.com
Mind Tap Ce
1 Fall CS111.ONL02.Computer Science...
CENGAGE MINDTAP
egramming Exercise 1.6
Instructions
circle.py
1 radius
float (input('5:')
2 area 3.14 * (5 *5)
Write and test a program that
3
computes the area of a circle.
1. Request a number
representing a radius as
input from the user.
2. Use the formula 3.14 x
radius? to compute the
area.
3. Output this result with a
suitable label.
An example of the program
input and output is shown
below:
Enter the radius: 5
The area is 78.5 square u
nits.
The input value should not be
limited to whole numbers
SEP
14
arrow_forward
Use 8086 assembly language by using the EMU8086 - assembler and
microprocessor emulator solve the problems:
Task 03
Write a program that takes an input (not from user) to check whether a
year is leap year or not and print "Leap Day"
Task 04
Write a program that takes all 3 sides of a triangle and prints whether the
triangle is equilateral, isosceles or neither.
arrow_forward
San Pedro Belize Express has become the leading mode of transportation to Caye Caulker
and San Pedro and neighboring islands. The changes in travel restrictions and tracking due to
the Covid 19 has placed a demand on the company to keep better records of their
passengers.
The Company is wanting a secure ticketing system capable of keeping important and relevant
information from their customers
Create a python program that allows only authorized user to log into the system for ticketing
transactions and customer information queries
The program will accept names, phone number and SSID from User, Destination before
printing tickets granting them access to board the boat
The program should be able to print all customer and destination and price paid for
tickets(eacher customer records should be printed on different line in a list format/ table
format)
Destination,
San Pedro,
Name,
ID#
Fee
John Doe
2133
25
Billy Jean
Ricky Martin Caye Chapel 1322
Caye Caulker 3345
30
18.50
The program will…
arrow_forward
Language 8.
Error
arrow_forward
C Program Malloc
Write a program that swaps the values of X and Y with malloc.
Fill in missing parts in the provided code in the editor.
Output
Before swap X: 412
Before swap Y: 623
After swap X: 623
arrow_forward
Hint:
the first line correction: P1 EQU 90H
arrow_forward
486.
Mechanism that uses a special CPU scheduling algorithm along with multiprogramming is known as
a.
Time-sharing systems
b.
Multithread systems
c.
Scheduling systems
d.
Procedural systems
arrow_forward
SWE 431: Security
General Guidelines
A project group can consist of at most 5 individuals.
Option 1
Implement the RSA in your preferred programming language
For Java,the packages java.math.BigInteger and java.security.SecureRandom should be used. For other
languages, you are responsible to use similar packages.
Source code must be well documented (comments).
The prime numbers p and q should be at least 1024-bit.
The difference of two primes should be bigger than 2512 (for security).
A demonstration of encrypting a message (big mumber) and decrypting the ciphertext (big number)
should be given.
Your program should also include the encryption and decryption timing.
The public key component e must be set to be 65537.
Submit your program and a readme.txt (instructions on how to compile and run your program) to the
elearning dropbox.
arrow_forward
201.
Program execution services use to
a.
Control Program
b.
Delete Program
c.
Execute Program
d.
Update Programs
arrow_forward
Alert: Don't submit AI generated answer and give answer with proper explanation and step by step solution. (introduction,explanation,final answer).
arrow_forward
Don't reject if you did not have knowledge.
Assembly language.
On sim8085 simulator
arrow_forward
Here are some registers and storage locations during the run of a program.For this example, all numbers are hex.eax contains 300ebx contains 3000The doubleword at memory location 300 contains 500The doubleword at memory location 10 contains 70
What is in eax after the command addl $10, %eax executes?
a. 310
b. 510
c. 370
arrow_forward
i need the answer quickly
arrow_forward
The computer memory module speed is specified in catalogs or literature either by the speed in MHz (for example DDR4-xxxx) or in MB/s (for example PC4-xxxx) or both.
For example, if the memory speed is DDR4-4700, then the corresponding speed in MB/s is PC4-______
arrow_forward
Assembly language
The program will prompt the user for their name and then say hello to them using their name. For example, the interactive console of Repl.it might look like:
> bash main.shEnter your name: *Lauren Cabello*Hello,Lauren Cabello>
You will not have a library of routines to call upon for the printing and reading. You are manufacturing the complete setup and syscall.
After you complete the reading, remember that .bss is for your variables that will receive data and .data is for fixed information, like prompts.
main.asm
section .bss name: resb 32 ; 32 bytes for name
section .data ; YOUR PROMPTS AND LENGTHS HERE
section .textglobal _start
_start:
;; YOUR CODE HERE!;
exit:mov rax, 60 ; exitmov rdi, 0 ; r
arrow_forward
A program of 33 bytes is loaded into memory. The Data Segment is shown below:
.DATA
X DB 3Ah, 12, 10101101b
S DB 5 DUP(3Bh)
Y DW 33ABh
What is the offset address of Y?
0000
0009
0008
0007
arrow_forward
Write assembly language program.
arrow_forward
Determine the output of the following Pep/9 machine-language program when the input typed into the Pep/9 input window is 35
Remember: the left column only shows the memory address of the first byte on the line. Do not copy these memory addresses into the Pep/9 object code
window
0000 D1FC15
0003 F10013
0006 D1FC15
0009 F1FC16
000C D10013
000F F1FC16
0012 00
arrow_forward
Is there any benefit to busy waiting?
arrow_forward
What does it mean to "preemptive schedule"? Computer science
arrow_forward
write a programme in python to read the digits of a number recursively and find its summation
arrow_forward
Computer Science
A major appliance company has hired you to work on next-generation kitchen appliances. You have been assigned to write code for a new smart oven/range combo (that means both the burners on the top and the part where you stick the cookies to bake). Excluding the simple time-of-day clock, identify at least two places where you might use a timer in your code. Since this is a "smart" appliance, feel free to add meaningful features that could utilize the timer.
arrow_forward
define the error plss
arrow_forward
Computer Science: Which method of scheduling processes is shown to be the most efficient?
arrow_forward
ment basic file input/output methods
To verify, test, and debug any errors in the code
Module learning outcomes assessed by this coursework:
On successful completion of this assignment, students will be expected, at threshold level, to be able
LO # 5: Software development environments (editor, interpreter, debugger), and programmi
using Python programming language.
Task:
Students are required to
create
a Python program that performs customized Caesa
encryption/decryption, as follows:
A- The program should have a main menu, through which the user can choose whether he wants
to encrypt a text or decrypt it.
B- If the user chose to encrypt plaintext, he will be asked to enter his ID, which is also the name
of the input file (i.e. if student ID is 199999, then the input file name should be 199999.txt).
The user should not enter the file extension (i.e. .txt), instead, the program should append
the extension to the ID automatically.
C- The input file must contain the following information:…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
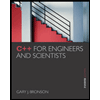
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
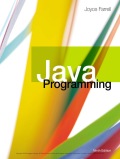
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Related Questions
- X = 56arrow_forwardSafari File Edit View History Bookmarks Window Help = zyBooks My library > CS 11: Introduction to Computer Science- C++ home > 1.19: LAB: Input and formatted output: Right-facing arrow 1.19 LAB: Input and formatted output: Right-facing arrow Given two input integers for an arrow body and arrowhead (respectively), print a right-facing arrow. Ex: If the input is: 01 the output is: 1 11 00000111 000001111 00000111 11 1 497630.3807866.qx3zqy7 LAB ACTIVITY learn.zybooks.com 1.19.1: LAB: Input and formatted output: Right-facing arrow 1 #include 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 } int baseDigit; int headDigit; /* Type your code here. *, return 0; EzyBooks catalog main.cpp :i 0/10 (( Load default template... Ơ 80 Fri Oct 13 3:27 AM Help/FAQ kimberly sandovalarrow_forwardread the question and answer it correctlyarrow_forward
- Time during which a job is processed by the computer is A. Delay time B. Real time C. Execution time D. Down time E. None of the abovearrow_forwardC linux Program homework need help, dont give the wrong answer please, show the result and output plz. also if you have definition that will help a lotarrow_forwarda ng.cengage.com Mind Tap Ce 1 Fall CS111.ONL02.Computer Science... CENGAGE MINDTAP egramming Exercise 1.6 Instructions circle.py 1 radius float (input('5:') 2 area 3.14 * (5 *5) Write and test a program that 3 computes the area of a circle. 1. Request a number representing a radius as input from the user. 2. Use the formula 3.14 x radius? to compute the area. 3. Output this result with a suitable label. An example of the program input and output is shown below: Enter the radius: 5 The area is 78.5 square u nits. The input value should not be limited to whole numbers SEP 14arrow_forward
- Use 8086 assembly language by using the EMU8086 - assembler and microprocessor emulator solve the problems: Task 03 Write a program that takes an input (not from user) to check whether a year is leap year or not and print "Leap Day" Task 04 Write a program that takes all 3 sides of a triangle and prints whether the triangle is equilateral, isosceles or neither.arrow_forwardSan Pedro Belize Express has become the leading mode of transportation to Caye Caulker and San Pedro and neighboring islands. The changes in travel restrictions and tracking due to the Covid 19 has placed a demand on the company to keep better records of their passengers. The Company is wanting a secure ticketing system capable of keeping important and relevant information from their customers Create a python program that allows only authorized user to log into the system for ticketing transactions and customer information queries The program will accept names, phone number and SSID from User, Destination before printing tickets granting them access to board the boat The program should be able to print all customer and destination and price paid for tickets(eacher customer records should be printed on different line in a list format/ table format) Destination, San Pedro, Name, ID# Fee John Doe 2133 25 Billy Jean Ricky Martin Caye Chapel 1322 Caye Caulker 3345 30 18.50 The program will…arrow_forwardLanguage 8. Errorarrow_forward
- C Program Malloc Write a program that swaps the values of X and Y with malloc. Fill in missing parts in the provided code in the editor. Output Before swap X: 412 Before swap Y: 623 After swap X: 623arrow_forwardHint: the first line correction: P1 EQU 90Harrow_forward486. Mechanism that uses a special CPU scheduling algorithm along with multiprogramming is known as a. Time-sharing systems b. Multithread systems c. Scheduling systems d. Procedural systemsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
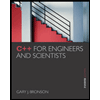
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
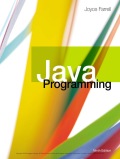
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT