hw2-s24
.pdf
keyboard_arrow_up
School
University of California, Riverside *
*We aren’t endorsed by this school
Course
153
Subject
Computer Science
Date
May 24, 2024
Type
Pages
6
Uploaded by MagistrateNarwhal4033
Homework 2 for CS153 (Spring 2024) Due: 5/6/2024
Instructions: Problem 1: (3 points) Write code, including clear synchronization logic, to simulate a set of dominos (number is N) that have to fall in order. Each domino is a thread that has a local variable i that indicates its number.
Problem 2 (6 points) A local laundromat with 3 washing machines is implementing
synchronization between customers using
semaphores. Each customer first allocates a machine (the machine number is
returned by allocate(), and when they are done returns the machine using release.
Available is an array keeping track of whether each of the 3 machines is available
(which is represented as 1; 0 is unavailable).
1. int allocate()
/* Finds and allocates a free washing machine. */
2. {
3. int i; //i is not shared
4. nfree.wait();
5. for (i=0; i < 3; i++)
6.
if (available [i] != 0 {
7.
available[i] = 0;
8.
return i;
9.
}
10.
}
11.
12. release(int machine) /* Release machine */
13.
{
14.
available[machine] = 1;
15.
nfree.signal();
16.
}
(a) (2 points) What is the purpose of nfree? What should it be intialized to?
(b) (4 points) Unfortunately, sometimes two customers were getting assigned the
same machine, and a fight followed. Explain by tracking two threads representing
the customers that fought, exactly how they got assigned the same machine.
Problem 3 (8 points). You are writing code for the voting machines for an upcoming election. You use shared counters, one for each candidate to keep track of the votes as they come from the different voting machines. You can think of each machine as a thread: every time it receives a vote, it increments a counter for that candidate. (a)
(2 point) Explain what could go wrong with this implementation if we do not use synchronization (b)
(2 points) Use Semaphores to solve this problem without changing the logic of the code other than adding the semaphore operations. You can show pseudo code or describe you changes clearly. Are there different alternatives as to where to place the semaphores?
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Fill in the blanks:5. The ( 6 ) is used to implement mutual exclusion where it can be decremented by aprocess and incremented by another, but the value must either be 0 or 1.6. If deadlock prevention approach is used to deal with deadlocks in a system, the ( 7 )condition can be prevented using the direct method.7. Two threads may share the memory space, but they cannot share the same ( 8 )8. Consider round-robin (RR) scheduling algorithm is implemented with 2 seconds timeslice and it is now selecting a new process; if we have 3 blocked processes (A, B, and C),and A has been waiting the longest, then A would need to wait a period of ( 9 ) secondsto be selected.9. In real-time systems, if a task appears at random times, then it is considered ( 10 ).
arrow_forward
Explain the terms "thread safety" and "race condition" in the context of multithreading.
arrow_forward
CONCURRENT AND PARALLEL PROGRAMMING
Implement a server class that control access to server using semaphores and listen to port 5555, also the server uses thread pools to manage client request, select a thread count that should optimal on thread count.
arrow_forward
Is it possible, in your opinion, for a single threaded process to get deadlocked without affecting any other threads? If you could elaborate on your answer in the following sentence, that would be great.
arrow_forward
doable without textbook
arrow_forward
In what situations might thread preemption be problematic?
arrow_forward
The following question is related to Threading
Task-1:
Write a c program that creates 5 threads and prints which thread is running and after the thread is closed, a new thread starts its execution. Each thread should run sequentially one by one.
OUTPUT:
thread-1 running
thread-1 closed
thread-2 running
thread-2 closed
arrow_forward
Part 3: Interrupt handling (30%)In Java multithreading environment, one thread can send an interrupt to another by calling theinterrupt() method on the Thread object for the target thread (i.e., the thread to beinterrupted). To handle interrupts in a target thread, Java allows two approaches. One is performedby writing an exception handler for InterruptedException (only applicable if the targetthread is invoking methods which throw that exception such as sleep). The other approach isperformed by periodically checking the interrupt status flag Thread.interrupted andperforming the handling routine when that flag is set to true.Write a Java program that illustrates the use of the two approaches described above. Your programshould start by creating two threads, each thread should use different interrupt handling approach.Then, the program needs to send interrupts to each one of the created threads such that a threadneeds to return (i.e., stop execution) after receiving an interrupt from…
arrow_forward
In this particular situation, a solution that just makes use of a single
thread is preferable than one that makes use of several threads.
arrow_forward
Here, a solution that uses a single thread is preferable than one that uses several threads.
arrow_forward
In this particular situation, a solution with a single thread is preferable than a solution with several threads.
arrow_forward
Explain the concept of thread pool in the context of dynamic multithreading.
arrow_forward
Lab 4 Directions
Write a C program called threadcircuit to run on ocelot which will provide a multithreaded solution to the circuit-satisfiability problem which will compute for what combinations of input values will the circuit output the value 1. This is the sequential solution, which is also attached. You should create 6 threads and divide the 65,536 test cases among them. For example, if p=6, each thread would be responsible for roughly 65,536/6 number of iterations (if it's not divisible, some threads can end up with one more iteration than the others). The test cases must be allocated in a cyclic fashion one by one.
#include <stdio.h>#include <sys/time.h>/* Return 1 if 'i'th bit of 'n' is 1; 0 otherwise */#define EXTRACT_BIT(n,i) ((n&(1<<i))?1:0)int check_circuit (int z) { int v[16]; /* Each element is a bit of z */ int i; for (i = 0; i < 16; i++) v[i] = EXTRACT_BIT(z,i); if ((v[0] || v[1]) && (!v[1] || !v[3]) && (v[2] || v[3])…
arrow_forward
Explain the concept of thread safety and race conditions. Provide a detailed example of a multithreaded program that demonstrates a race condition and propose a solution to eliminate it.
arrow_forward
Answer the given question with a proper explanation and step-by-step solution.
show work
arrow_forward
A deadlock condition can occur in concurrent code if two or more threads each hold a resource and are waiting for another thread to release a resource they need to proceed.
Question 10 options:
True
False
arrow_forward
Explain the terms "thread safety" and "race condition" in the context of multithreaded programming. Provide an example of a situation where race conditions can occur and how to prevent them.
arrow_forward
Please write your answer clearly .
arrow_forward
Describe the difference between cooperative (or non-preemptive) multitasking in
thread execution and non-cooperative (or preemptive) multitasking.
arrow_forward
In the four diagrams illustrated below, show which of them result in deadlock? For those situations that will result in deadlock, provide the cycle of resources and threads. For those situations which are not in deadlock, show by illustration the order in which the threads may complete their executions
arrow_forward
Describe the terms "thread synchronization" and "thread safety" in the context of multithreading.
arrow_forward
Suppose I have two threads that perform a few operations, a mutex mx and a condition variable cond,
as follows:
Thread 1:{
q();
lock(mx);
t();
wait(cond);
unlock(mx);
n();
}
Thread 2:{
lock(mx);
i();
signal(cond);
1();
unlock(mx);
}
Which of the following orderings are possible? Select ALL correct answers (The notation "a→b"
means that the call to a() "happens before" the call to b().)
O one of the threads could block forever, while the other thread finishes
O q>i>l>t→n x
O i>q>l>t→n x
O i>q>t→n→I
O q>i>t→I→n
q→t→i>l»n[
O both threads could block forever
Select all possible options that apply. e
X 0%
arrow_forward
Write a java program to create a Thread using Runnable Interface
arrow_forward
Write java program for the following
Create a child thread class for calculating base b to the power p
Create a single object of each thread in the main thread, and stop the execution of main thread until the termination of all child threads using join method
arrow_forward
One way to avoid deadlock is to schedule threads carefully. Assume the following characteristics of threads T1, T2, T3 and T4:
• T1 (at some point) acquires and releases locks L1, L2 and L5
• T2 (at some point) acquires and releases locks L2, L3 and L4
• T3 (at some point) acquires and releases locks L1, L2 and L4
• T4 (at some point) acquires and releases locks L3, L4 and L5
For which schedules below is deadlock possible?
(a) T4, T2, T3, and T1 run concurrently.
(b) T1 and T2 run concurrently to completion, then T3 runs to completion, then T4 runs.
(c) T1 and T3 run concurrently to completion, then T2 and T4 run concurrently to completion.
O
(d) T2 and T4 run concurrently to completion, then T1 runs to completion.
(e) T1 and T4 run concurrently to completion, T1 finishes first after which T4 continuous to run to completion with T2.
O
arrow_forward
Use Ue CUR
Question 10
Consider the following code:
#pragma omp parallel for
for(int i= 1:1<= 12; ++)
a) = i
Rewrite the code so the values of i assigned to each thread are the following:
P1: 1, 2, 7, 8
P2: 3, 4, 9, 10
P3: 5, 6, 11, 12
Ise the editor to format vour answer
arrow_forward
List the 4 conditions that can cause a deadlock for concurrent processes P1 and P2.
(short answer is ok)
arrow_forward
Investigate how race conditions can be avoided by using a mutex and locks, as well as other thread management techniques in C++.
arrow_forward
In the four diagrams illustrated below, show which of them result in deadlock? For those which are not in deadlock, show by illustration the order in which the threads may complete their executions situations that will result in deadlock, provide the cycle of resources and threads. For those situations
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
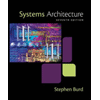
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Related Questions
- Fill in the blanks:5. The ( 6 ) is used to implement mutual exclusion where it can be decremented by aprocess and incremented by another, but the value must either be 0 or 1.6. If deadlock prevention approach is used to deal with deadlocks in a system, the ( 7 )condition can be prevented using the direct method.7. Two threads may share the memory space, but they cannot share the same ( 8 )8. Consider round-robin (RR) scheduling algorithm is implemented with 2 seconds timeslice and it is now selecting a new process; if we have 3 blocked processes (A, B, and C),and A has been waiting the longest, then A would need to wait a period of ( 9 ) secondsto be selected.9. In real-time systems, if a task appears at random times, then it is considered ( 10 ).arrow_forwardExplain the terms "thread safety" and "race condition" in the context of multithreading.arrow_forwardCONCURRENT AND PARALLEL PROGRAMMING Implement a server class that control access to server using semaphores and listen to port 5555, also the server uses thread pools to manage client request, select a thread count that should optimal on thread count.arrow_forward
- Is it possible, in your opinion, for a single threaded process to get deadlocked without affecting any other threads? If you could elaborate on your answer in the following sentence, that would be great.arrow_forwarddoable without textbookarrow_forwardIn what situations might thread preemption be problematic?arrow_forward
- The following question is related to Threading Task-1: Write a c program that creates 5 threads and prints which thread is running and after the thread is closed, a new thread starts its execution. Each thread should run sequentially one by one. OUTPUT: thread-1 running thread-1 closed thread-2 running thread-2 closedarrow_forwardPart 3: Interrupt handling (30%)In Java multithreading environment, one thread can send an interrupt to another by calling theinterrupt() method on the Thread object for the target thread (i.e., the thread to beinterrupted). To handle interrupts in a target thread, Java allows two approaches. One is performedby writing an exception handler for InterruptedException (only applicable if the targetthread is invoking methods which throw that exception such as sleep). The other approach isperformed by periodically checking the interrupt status flag Thread.interrupted andperforming the handling routine when that flag is set to true.Write a Java program that illustrates the use of the two approaches described above. Your programshould start by creating two threads, each thread should use different interrupt handling approach.Then, the program needs to send interrupts to each one of the created threads such that a threadneeds to return (i.e., stop execution) after receiving an interrupt from…arrow_forwardIn this particular situation, a solution that just makes use of a single thread is preferable than one that makes use of several threads.arrow_forward
- Here, a solution that uses a single thread is preferable than one that uses several threads.arrow_forwardIn this particular situation, a solution with a single thread is preferable than a solution with several threads.arrow_forwardExplain the concept of thread pool in the context of dynamic multithreading.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
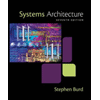
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning