cse160_practice7
.py
keyboard_arrow_up
School
University of Washington *
*We aren’t endorsed by this school
Course
160
Subject
Computer Science
Date
May 26, 2024
Type
py
Pages
2
Uploaded by GrandFlag10766
# Name: Sara Duan
# CSE 160
# Spring 2024
# Checkin 7
# Problem 1
def first_character(input_list):
'''
Write a one-line list comprehension that returns a list containing
the first letter of each string in the input list.
Returns an empty list if the input list has no elements
Arguments:
input_list: a list of strings. You may assume all strings
are at least one character long
Returns: a list of strings
'''
return [s[0] for s in input_list]
assert first_character([]) == []
assert first_character(['a']) == ['a']
assert first_character(['cse160']) == ['c']
assert first_character(['one', 'two']) == ['o', 't']
assert first_character(['one', 'two', 'three', 'four', 'five']) == \
['o', 't', 't', 'f', 'f']
assert first_character(['1']) == ['1']
assert first_character(['one', 'one', 'one']) == ['o', 'o', 'o']
assert first_character(['fourty-two', 'life', 'universe', 'everything']) == \
['f', 'l', 'u', 'e']
# Problem 2
def larger_elements(input_list, input_num):
'''
Write a one line list comprehension that returns a list
containing all the elements of a given list that
are larger than a given number.
Reutrn an empty set if input_list is empty
Arguments:
input_list: a list of integers
input_num: an integer
Returns: A list containing all the elements of the input_list higher
than input_num
'''
return [x for x in input_list if x > input_num]
assert larger_elements([], 5) == []
assert larger_elements([5], 5) == []
assert larger_elements([6], 5) == [6]
assert larger_elements([4], 5) == []
assert larger_elements([1, 2, 3], 5) == []
assert larger_elements([5, 6, 7, 8], 5) == [6, 7, 8]
assert larger_elements([-1, 5, 134, -15, 2], 100) == [134]
assert larger_elements([-1, 64, 3, -20, 2], -5) == [-1, 64, 3, 2]
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
List
Write a complete Java program in the that will require the user to input and store 10 double data type values in a list. The program should output the following information:
lowest value
the highest value
the sum (also called total) of all the input values
the average value.
Note: the input data maybe positive or negative.
arrow_forward
Im Python please
arrow_forward
paython programing question
Write a program that creates a list of N elements (Hint: use append() function), swaps the first and the last element and prints the list on the screen. N should be a dynamic number entered by the user.
Sample output:
How many numbers would you like to enter?: 5
Enter a number: 34
Enter a number: 67
Enter a number: 23
Enter a number: 90
Enter a number: 12
The list is: [12, 67,23, 90, 34]
arrow_forward
python program plz
arrow_forward
python code easy way pls thank you
arrow_forward
Python Programming
Write a program that asks the user to enter a series of ten numbers. The program is to store the numbers in a list:
Write a function that accepts the created list as an argument and displays the following:
The lowest number in the list
The highest number in the list
The total of the values in the list
The average of the numbers in the list
Ask the user to enter a number, this number will be used in the following step.
Write a function that accepts the created list and the number as arguments. The function is to display all the numbers in the list that are greater than then number.
arrow_forward
11
Language Python
arrow_forward
HAPTER 5: Lists and Dictiona
st membership > 51621 O
WorkArea
Instructions
eadline: 03/
11:59pm EDT
Given that k contains an integer and that play_list has been defined to be a list,
write a expression that evaluates to True if the value assigned to k is an element of
play_list.
Additional Notes: play_list and k should not be modified
arrow_forward
Ex1: a) Write a function for the user to enter a positive integer n with a value between 10 and 1000. If entered correctly, the function generates a list containing n random values that do not overlap between 1 and 5000 and the function returns the newly created list. Conversely, if entered incorrectly, the program will ask to re-enter until it is correct. Cases where n is considered incorrect:(i)- If the user enters a non-integer data type (like string, float, bool, ...)The program will display the error message 'Must enter a positive integer. Requires re-entry'.(ii)- If the input value is of the correct integer data type but the value is not in the range10 to 1000, the program gives the error 'Only values between 10 and 1000. Re-enter required'.Installation requirements: must use the try ... except statement to handle possible exceptionsin the program.
b) Write a function that lists the amicable numbers in the list just created in a)
(If there are no amicable numbers in the list,…
arrow_forward
In Python please
arrow_forward
OCaml Code: Please read the attached instructions carefully and show the correct code with the screenshot of the output. I really need help with this assignment.
arrow_forward
Return Growing NumList
This function will be given a single number, it should return a list of strings of numbers. Each string in the list
will only contain a single number repeated an arbitrary amount of times. The number each string will contain
will be equal to the current string's index+1. The number in the string should be repeated the same number of
times as the string's index+1. Each number in the string should be separated by a space. This list should stop
when its size equals the max number specified.
Signature:
public static ArrayList returnGrowingNumList(int max)
Example:
INPUT: 3
OUTPUT: [1, 22, 333]
INPUT: 6
OUTPUT: [1, 22, 333, 444 4, 55555, 666666]
arrow_forward
In Python please
arrow_forward
QUESTION 4
in phython language
Write a program that creates a list of N elements (Hint: use append() function), swaps the first and the last element and prints the list on the screen. N should be a dynamic number entered by the user.
Sample output:
How many numbers would you like to enter?: 5
Enter a number: 34
Enter a number: 67
Enter a number: 23
Enter a number: 90
Enter a number: 12
The list is: [12, 67,23, 90, 34]
arrow_forward
FUNCTION ONE: MAINO
In this lab we will create three functions. The first will be called
main. Main will simply be the first function executed and will
call the other two funetions. It will take in no arguments and
will only create an empty list and call the other two functions.
FUNCTION TWO: GETDATAO
Getdata() will take in a list and then prompt the user to enter a
song as a string in the format of song-band. You may assume
the user will correctly enter this data. These songs will be
appended to our list. The user can continue entering songs until
they enter "exit".
FUNCTION THREE: PLAYRANDOMO
PlayRandom() will take in a list. It will then generate a random
number and use it as index to select a random song to print
"Now Playing: RandomSongHere".
SAMPLE OUTPUT
Enter a song title and band (song-band) or exit to quit: I Found-
Amber Run
Enter a song title and band (song-band) or exit to quit: Bark At
The Moon-Ozzy Osbourne
Enter a song title and band (song-band) or exit to quit: Real…
arrow_forward
This.
arrow_forward
Assume my_list is a list of integer values. Write a list comprehension statement in Python that makes a duplicate of my_list named copy_list.
arrow_forward
++
arrow_forward
Write a function that accepts a list as an argument and calculates the sum of each element of the list.
arrow_forward
Contact list: Binary Search
A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name.
Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1).
int FindContact(ContactInfo contacts[], int size, string contactName)
Ex: If the input is:
3 Frank 867-5309 Joe 123-5432 Linda…
arrow_forward
1- Programming Problem Part 1
Write a function that will have a list as an input, the task of the function is to check if all the elements in the list are unique,( i.e. no repetition of any value has occurred in the list), then the function returns true otherwise it returns false. Your program should include a main method that call the method to test it.
(If you would use built-in functions in Python, use lists’ or dictionaries’ methods as we studied their time efficiency in class)
arrow_forward
/// in python ///
def get_words_last_1():"""The function should take a list of wordsas an input parameter and return a new list of stringswith the last character removed. (Use string slicing)If the word is empty or has only 1 character, then skip it.""" pass
arrow_forward
PYTHON CODE - WRITE PYTHON FUNCTION
arrow_forward
OCaml Code: Attached are the instructions. Make sure to read the instructions carefully and write your own test cases to make sure the code works. Make sure to include a screenshot of the correct code with the test cases that is used along with the output of the code being passed.
arrow_forward
in PYTHON
Write a function that accepts a list as an argument and calculates the sum of each elementof the list.
arrow_forward
Algorithm Efficiency
1- Programming Problem Part 1
Write a function that will have a list as an input, the task of the function is to check if all the elements in the list are unique,( i.e. no repetition of any value has occurred in the list), then the function returns true otherwise it returns false. Your program should include a main method that call the method to test it.
(If you would use built-in functions in Python, use lists’ or dictionaries’ methods as we studied their time efficiency in class)
2- Algorithm AnalysisFor the function you implemented in part 1, please calculate T(n), which represents the running time of your algorithm in terms of n. Where n is the length of the list. Then find the order of magnitude of your algorithm (Big O).
3- Algorithms Comparison
Please find another algorithm that solves part 1, write the code, calculate T(n) and find Big O. Then compare the efficiency with the algorithm from part1 to determine the more efficient one.
arrow_forward
Write a function that accepts a list as an argument (assume the list contains integers) and returns the total of the values in the list.
arrow_forward
Q3-Range Function: Using the range() function Write a Python a program that generates and prints the following lists for positive integer N.
1. The list DN of integers in the range [0, N-1]
2. The list EN of even integers in the range [0, N-1]
3. The list AN of even integers in the range [-N, N]
N = 20
ON List (range (N))
EN=
AN
print('DN', DN)
print('EN=, EN)
print("AN=¹, AN)
Solve ALL three parts please
arrow_forward
Rewrite the following function using a while loop:
def sum_of_list(lst):
"""Docstring: rewrite the sum of all values in a list
Parameters: lst: a list of numbers
Return: a number
"""
sum = 0
for item in lst:
sum *= item
return sum
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
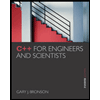
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Related Questions
- List Write a complete Java program in the that will require the user to input and store 10 double data type values in a list. The program should output the following information: lowest value the highest value the sum (also called total) of all the input values the average value. Note: the input data maybe positive or negative.arrow_forwardIm Python pleasearrow_forwardpaython programing question Write a program that creates a list of N elements (Hint: use append() function), swaps the first and the last element and prints the list on the screen. N should be a dynamic number entered by the user. Sample output: How many numbers would you like to enter?: 5 Enter a number: 34 Enter a number: 67 Enter a number: 23 Enter a number: 90 Enter a number: 12 The list is: [12, 67,23, 90, 34]arrow_forward
- python program plzarrow_forwardpython code easy way pls thank youarrow_forwardPython Programming Write a program that asks the user to enter a series of ten numbers. The program is to store the numbers in a list: Write a function that accepts the created list as an argument and displays the following: The lowest number in the list The highest number in the list The total of the values in the list The average of the numbers in the list Ask the user to enter a number, this number will be used in the following step. Write a function that accepts the created list and the number as arguments. The function is to display all the numbers in the list that are greater than then number.arrow_forward
- 11 Language Pythonarrow_forwardHAPTER 5: Lists and Dictiona st membership > 51621 O WorkArea Instructions eadline: 03/ 11:59pm EDT Given that k contains an integer and that play_list has been defined to be a list, write a expression that evaluates to True if the value assigned to k is an element of play_list. Additional Notes: play_list and k should not be modifiedarrow_forwardEx1: a) Write a function for the user to enter a positive integer n with a value between 10 and 1000. If entered correctly, the function generates a list containing n random values that do not overlap between 1 and 5000 and the function returns the newly created list. Conversely, if entered incorrectly, the program will ask to re-enter until it is correct. Cases where n is considered incorrect:(i)- If the user enters a non-integer data type (like string, float, bool, ...)The program will display the error message 'Must enter a positive integer. Requires re-entry'.(ii)- If the input value is of the correct integer data type but the value is not in the range10 to 1000, the program gives the error 'Only values between 10 and 1000. Re-enter required'.Installation requirements: must use the try ... except statement to handle possible exceptionsin the program. b) Write a function that lists the amicable numbers in the list just created in a) (If there are no amicable numbers in the list,…arrow_forward
- In Python pleasearrow_forwardOCaml Code: Please read the attached instructions carefully and show the correct code with the screenshot of the output. I really need help with this assignment.arrow_forwardReturn Growing NumList This function will be given a single number, it should return a list of strings of numbers. Each string in the list will only contain a single number repeated an arbitrary amount of times. The number each string will contain will be equal to the current string's index+1. The number in the string should be repeated the same number of times as the string's index+1. Each number in the string should be separated by a space. This list should stop when its size equals the max number specified. Signature: public static ArrayList returnGrowingNumList(int max) Example: INPUT: 3 OUTPUT: [1, 22, 333] INPUT: 6 OUTPUT: [1, 22, 333, 444 4, 55555, 666666]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
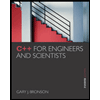
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr