CSCI 2600 Homework 2
.pdf
keyboard_arrow_up
School
Rensselaer Polytechnic Institute *
*We aren’t endorsed by this school
Course
2600
Subject
Computer Science
Date
Feb 20, 2024
Type
Pages
7
Uploaded by LieutenantGrasshopper913
CSCI 2600 — Principles of Software
Homework 2: Reasoning About Loops
Due: Tuesday, Feb. 13, 2024, 11:59:59 pm
Introduction
In this assignment you will prove correctness of loops using the techniques we discussed in class.
Submission Instructions
Follow the directions in the
version control handout
for cloning your hw02 git repo.The URI
that you will need to use to clone your personal repo for this homework would have the form of
https://submitty.cs.rpi.edu/git/s24/csci2600/hw02/RCSID
where
RCSID
is your RCS
ID. Submit your answers in a single .PDF file named
hw2_answers.pdf
in the
answers/
directory of your repository.
Submit your Dafny code as
problem1.dfy
,
problem2.dfy
,
problem3.dfy
, and
problem4.dfy
files in the
answers/
directory of your repository. Submit your answers in a single .PDF file
named
hw2_answers.pdf
in the answers/ directory of your repository.
You MUST type
up your answers. Handwritten solutions will not be accepted or graded, even if
they are scanned into a PDF file.
We recommend using
LaTeX
. If you have never used
LaTeX, take a look at this
tutorial
.
Be sure to commit and push the files to Submitty. Follow the directions in the
version control
handout
for adding and committing files.
Important: You must click the
Grade My Repository
button for you answers to
be graded. If you do not, they will not be graded and you will receive a zero for the
homework.
Problems
All answers should be placed in
answers/hw2
answers.pdf
except for Dafny files which should be
separate files with names as given in each problem description.
Problem 1 (8 pts.): Sum of natural numbers
Below we give, in Java syntax, the
sumn
method which computes the sum of the
n
natural numbers
from 1 to
n
. For example,
sumn
(0) == 0,
sumn
(1) == 1,
sumn
(2) == 3, and
sumn
(6) == 21.
//
Precondition :
n
>
= 0
int
sumn(
int
n)
{
int
i
= 0 ,
t =
0;
while
( i
<
n)
{
i
=
i
+
1;
t = t +
i ;
}
return
t ;
}
//
Postcondition :
t
= n
(n + 1)
/
2
a) Find a suitable loop invariant. (1 pt.)
b) Show that the invariant holds before the loop (base case). (1 pt.)
c) Show by induction that if the invariant holds after k-th iteration, and execution takes a k+1-st
iteration, the invariant still holds (inductive step). (2 pts.)
d) Show that the loop exit condition and the loop invariant imply the postcondition
t
=
n
∗
(
n
+1)
/
2.
(1 pt.)
e) Find a suitable decrementing function. Show that the function is non-negative before loop starts,
that it decreases at each iteration and that when it reaches 0 the loop is exited. (1 pts.)
f) Implement the sum of natural numbers in Dafny. (2 pts., autograded)
Comment out
method
Main
in your Dafny code before you submit your code on Submitty.
Method that implements the sum of natural numbers must be named
sumn
and have the
following header:
method sumn(n:
int) returns (t:
int)
Make sure to include the precondition and the postcondition, as well as your invariant and
the decrementing function.
Verify your code with Dafny before submitting.
Submit your Dafny code as a file named
problem1.dfy
in the
answers/
folder.
Problem 2 (15 pts.): Loopy square root
Below we give, in Dafny syntax, the square root method which should be computing the square
root of a number.
method loopysqrt(n:int) returns (root:int)
requires n >= 0
ensures root * root == n
{
root := 0;
var a := n;
while (a > 0)
//decreases //FILL IN DECREMENTING FUNCTION HERE
//invariant //FILL IN INVARIANT HERE
{
root := root + 1;
a := a - (2 * root - 1);
}
}
a) Test this code by creating the
Main()
method and calling
loopysqrt()
with arguments like
4
,
25
,
49
,
etc.
to convince yourself that this algorithm appears to be working correctly.
In
your answer, describe your tests and the corresponding output. (1 pt.)
b) Yet, the code given above fails to verify with Dafny.
One of the reasons for this is that it
actually does have a bug. More specifically, this code may produce the result which does not
comply with the specification. Write a test (or tests) that reveals the bug. In your answer,
describe your test(s), the corresponding outputs, and the bug that you found. Also, indicate
which part of the specification is violated. (1 pt.)
c) Now find and fix this bug.
Note that there might be several different ways of fixing the
bug. Use the method that you think would be the best. You are not allowed to change the
header of
loopysqrt()
or add, remove, or change any specifications or annotations. Do not
worry about Dafny verifying the code for now, just fix the bug and convince yourself that
loopysqrt()
is now correct. You are also required to keep the overall algorithm the same as
in the original version of the code. In your answer, describe how you fixed the bug and show
the output of the same tests you ran before after fixing the bug. (2 pts.)
d) Update the specification of
loopysqrt()
to match the way you fixed the bug. If you changed
the postcondition, make sure that it is the strongest possible postcondition. In your answer,
describe your changes and explain why they were necessary. (1 pt.)
e) Does your Dafny code verify now? Why or why not? If it doesn’t verify, does it mean that
your code still has bugs in it? (1 pt.)
f) If your Dafny code doesn’t verify, uncomment
invariant
and/or
decreases
annotations
and supply the actual invariant and/or decrementing function.
Make sure your code now
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Assignment3A:
Diagnosis Checker: For years, patients have used websites like WebMD to pre-diagnosis
themselves with a variety of illnesses before meeting with a doctor. As you might imagine,
medical professionals believe this can lead to incorrect treatment. To combat this, a local
hospital has asked you to develop a program that will help patients check if their symptoms
match the online diagnosis.
You will prompt the user to enter their illness. Based on this input, you will ask them if they are
experiencing certain symptoms. Depending on their answer, your program will indicate if the
Internet diagnosis is likely to be correct or not. The decision chart is shown below:
•
●
●
HGPS
0
Do they have brittle bones?
If no, it is likely not HGPS
■ If yes, it is possibly HGPS
Bokter Syndrome
O
Do they have a Vitamin C deficiency?
If no, it is likely not Bokter Syndrome
If yes, are they a sailor?
.
.
Lupus
.
If no, it is likely not Bokter Syndrome
If yes, it is possibly Bokter Syndrome
arrow_forward
At the end of this specific task, students should be able to:• Create and work with Loops• Handle and manipulate strings • (Learning Units 4 and 5).It is good practice to leave your main branch as your long live branch, this means that the code on this branch is always in perfect working order and tested. We make use of feature branches in order to ensure that any code we push to GitHub does not break our main branch. You can create a feature branch by running the following command.git checkout -b KhanbanTasks (you can use any branch name)** You are welcome to make use of GitHub desktop or your IDE to push code to GitHub if you are not comfortable with using the command line.You can now add the following functionality to your application:1. The users should only be able to add tasks to the application if they have logged in successfully.2. The applications must display the following welcome message: “Welcome to EasyKanban”.3. The user should then be able to choose one of the following…
arrow_forward
astfoodStats Assignment Description
For this assignment, name your R file fastfoodStats.R
For all questions you should load tidyverse, openintro, and lm.beta. You should not need to use any other libraries.
suppressPackageStartupMessages(library(tidyverse))
suppressPackageStartupMessages(library(openintro))
suppressPackageStartupMessages(library(lm.beta))
The actual data set is called fastfood.
Continue to use %>% for the pipe. CodeGrade does not support the new pipe.
Round all float/dbl values to two decimal places.
All statistics should be run with variables in the order I state
E.g., "Run a regression predicting mileage from mpg, make, and type" would be:
lm(mileage ~ mpg + make + type...)
To access the fastfood data, run the following:
fastfood <- openintro::fastfood
Create a correlation matrix for the relations between calories, total_fat, sugar, and calcium for all items at Sonic, Subway, and Taco Bell, omitting missing values with na.omit().
Assign the…
arrow_forward
java programming
arrow_forward
how to correct this exercise.
COP2002: Programming Logic & Design with PythonProject 4Project: Download and save the file named proj_4_to_fix_wishes.py. The program has five errors including a syntax error, runtime errors, and logic errors. Find and fix all the errors. You must also document the errors you found and how you fixed them. Since you can only upload a single file into Canvas, put the explanation of the errors at the top of the program, as comments. The output of your fixed program should look as shown:Sample Console Display:
Notes• You are not required to validate if the user enters a character or a string. The only validation is the one in the program: the user must enter values equal to or greater than 0.• You do not need to validate the user's response to the question, "Enter an item? y/n". If the user enters anything other than 'y' or 'n' the program can simply end.• Save the corrected file with the filename xxx_wishes_fixed.py (where xxx = your initials) or as…
arrow_forward
Step1-Study the scenario below and create a Java Program for Builders Warehouse, The program must consist of a class called Materials to handle the details of the materials.
Step 2-The program should read the materials details from the material.txt file then use a LinkedList to store the material details.
Step 3-Then finally the products stored in the LinkedList must be displayed like the sample code below:
Step 4-There must be clear code and a screenshot of the code running.
arrow_forward
Create a new C++ project in Visual Studio (or an IDE of your choice). Add the files listed below to your project:
BinaryTree.h Download BinaryTree.h
BinarySearchTree.h Download BinarySearchTree.h
main.cpp Download main.cpp
Build and run your project. The program should compile without any errors. Note that function main() creates a new BST of int and inserts the nodes 12, 38, 25, 5, 15, 8, 55 (in that order) and displays them using pre-order traversal. Verify that the insertions are done correctly. You may verify it manually or use the visualization tool at https://www.cs.usfca.edu/~galles/visualization/BST.html. (Links to an external site.)
Do the following:
1. add code to test the deleteNode and search member functions. Save screenshot of your test program run.
2. add code to implement the nodeCount member function of class BinaryTree. Test it and save screenshot of your test program run.
3. add code to implement the leafCount member function of class BinaryTree. Test it and…
arrow_forward
omplete this javascript file according to the individual instructionsgiven in the comments.
*** DO NOT CHANGE any of the code that you are not instructed to. */
////////////////////////// NOTE: Use the API endpoints available at// https://jsonplaceholder.typicode.com/// to get the data required in these exercises.// HINT: Read the documented Resources and Routes.// Also the guide:// https://jsonplaceholder.typicode.com/guide////////////////////////
Create a function named "getUser".// This function needs to accept a "userID"// parameter.// Use Fetch with Async/Await to request// the data for the requested user.// The function should return JSON data.
arrow_forward
create a script that will parse data from Rotten Tomatoes, a movie reviews website. The work you have to do is identical to what we covered in lectures 4 and 5, albeit for a different website. Please read and follow the instructions below very carefully.
Step 1
Your script should begin by defining two variables (after importing libraries, etc)
movie a string variable indicating the movie for which reviews will be parsed
pageNum the number of review pages to parse
For example, to parse the first 3 pages of the Gangs of New York reviews, set movie ‘’gangs_of_new_york” and pageNum = 3. Your code should go to the movie’s All Critics reviews page of rotten tomatoes, and parse the first three pages of reviews. Pagination on rotten tomatoes happens by clicking on the “Next” button.
Step 2
For each review contained in each of the pages you requested, parse the following information
The critic This should be 'NA' if the review doesn't have a critic’s name.
The rating. The rating…
arrow_forward
In c# program
arrow_forward
Write a program that creates a login name for a user, given the user's first name, last name, and a four-digit integer as input. Output the login
name, which is made up of the first five letters of the last name, followed by the first letter of the first name, and then the last two digits of
the number (use the % operator). If the last name has less than five letters, then use all letters of the last name.
Ex: If the input is:
Michael Jordan 1991
the output is:
Your login name: JordaM91
Ex: If the input is:
Kanye West 2024
the output is:
Your login name: WestK24
arrow_forward
Q4. The attached Java program (Recursion_Demo.java) (C# version is also
attached) demonstrates the concept of recursion. The program inputs the value
of n from the user and then the recursive RDemo method prints the following
sequence:
n (n-1) (n-2)... 1123... n
Execute the program and check it out for yourself. You will write a MIPS
program which MUST use a non-leaf procedure to implement this recursive
method. Your program will define a non-leaf procedure RDemo which will be
called recursively for n>=1. RDemo will return when n<1. Your program will
print the user prompt and take n as an input in the main program. The output
on the screen must be just similar to the Java program - there will be space
between the integers. Obviously, your MIPS program will not implement the
concept of class.
(See next page)
arrow_forward
WebConnect 3270
Sphinx of Hatsheps...
- Question Completion Status:
Based on this information, complete the code for numbered region 3.
QUESTION 10
("Enter a number:"))
Fill each of the numbered areas so that the code you create asks the user to enter a whole number using the keyboard and
store that number into variable x.
QUESTION 11
if(x<0 or x10):
print("Invalid number! ")
The piece of code above checks whether x is between 0 and 10, If not it prints a text.
Click
arrow_forward
please may you help me with this in java ide language
arrow_forward
JavaScript Loops
Create an HTML
document called
assignment2.html
that implements a webpage that
dynamically creates (using JavaScript code) a 15X15 HTML table and displays in each
cell the (row, column) information for that cell. Allow the user to select the number of
rows and columns to redraw a new table.
Follow these guidelines to implement your assignment:
1.
Create a JavaScript file called assignment2.js and write the code for a function
that draws the table.
2.
Invoke the function when the visitor clicks on the assignment2.html page.
3.
Load the assignment2.js file at the end of the body in assignment2.html.
4.
(
10% of the grade
) Accept user input for the number of rows and columns in the
table.
5.
(
10% of the grade
) Use good web design practices to enhance visually your
html page. Add a title, picture, colors, copyright line, etc.
arrow_forward
SE case study homework (PLAGIARISM WILL BE DETECTED !!!)
Please provide an upload link for the file
This case study is about a simplified Payphone coin system.1. The minimum price of a communication is 10 ryals.2. After the introduction of the currency, the user has 2 minutes to dial his number (this time iscounted by a time counter).3. The line can be free or busy.4. The Payphone consumes money as soon as the called person picks up and at each time unitgenerated by the counter.5. You can add pieces of money at any time.6. At the time of the hang-up, the balance of the currency introduced (the rest of the money) isreturned.
Required work:From this statement, you are asked to:1. Establish the use case diagram of this statement.2. Construct a sequence diagram that describes the nominal scenario of Payphoning use case inthis statement.
arrow_forward
nycflights13
For this assignment, you must name your R file nycflights13.R
*****use Rmarkdown file in Rstudios
For all questions you should load tidyverse and nycflights13. You should not need to use any other libraries.
If the tidyverse package is not installed, you’ll need to do a one-time installation from the Console Window in RStudio like this:install.packages("tidyverse")
If the nycflights13 package is not installed, you’ll need to do a one-time installation from the Console Window in RStudio like this:install.packages("nycflights13")
Load tidyverse with: suppressPackageStartupMessages(library(tidyverse))
Load nycflights13 with:suppressPackageStartupMessages(library(nycflights13))
The actual data set is called flights.See the nycflights13 package page and chapter 5 from the textbook for more info.
You can not attempt to install packages in CodeGrade.
Round all float/dbl values to two decimal places.
If your rounding does not work the way you expect, convert the tibble to a…
arrow_forward
nycflights13
For this assignment, you must name your R file nycflights13.R
*****use Rmarkdown file in Rstudios
For all questions you should load tidyverse and nycflights13. You should not need to use any other libraries.
If the tidyverse package is not installed, you’ll need to do a one-time installation from the Console Window in RStudio like this:install.packages("tidyverse")
If the nycflights13 package is not installed, you’ll need to do a one-time installation from the Console Window in RStudio like this:install.packages("nycflights13")
Load tidyverse with: suppressPackageStartupMessages(library(tidyverse))
Load nycflights13 with:suppressPackageStartupMessages(library(nycflights13))
The actual data set is called flights.See the nycflights13 package page and chapter 5 from the textbook for more info.
You can not attempt to install packages in CodeGrade.
Round all float/dbl values to two decimal places.
If your rounding does not work the way you expect, convert the tibble to a…
arrow_forward
Python program with unique solution not copied please.
arrow_forward
JavaScript
imageMosaic: compute image mosaic. You must implement the final version of the image mosaic algorithm we discussed in class (which includes the scaling of mosaic images and also randomization). Since the algorithm involves several nested loops and can take a while to run, this function will be implemented using a non-blocking loop so that the browser will not complain about its running speed. Details are in the comments embedded in the code.
function imageMosaic(input, output, mosaic_name) {
let width = input.width;
let height = input.height;
let mimages = mosaics[mosaic_name]; // mimages is an array of mosaic images
let w = mimages[0].width; // all mosaic images have the same size wxh
let h = mimages[0].height;
let num = mimages.length; // number of mosaic images
console.log('Computing Image Mosaic...');
let y = 0;
(function chunk() {
for(x = 0; x <= width-w; x += w) { // x loop
// ===YOUR CODE STARTS HERE===
/*
for(y=0; y<=input.height-h; y+=h) {
for (x=0;…
arrow_forward
JS events? Click code.
arrow_forward
ASSIGNMENTWrite a Java program that meets these requirements.• Create a NetBeans project named LastnameAssign1. Change Lastname to your last name. For example, my project would be named NicholsonAssign1.• In the Java file, print a welcome message that includes your full name.• The program should prompt for an XML filename to write too The filename entered must end with .xml and have at least one letter before the period. If it does not, print a warning and prompt again. Keep nagging the user until a proper filename is entered.• Prompt the user for 3 integers.o The three integers represent red, blue, and green channel values for a color.o Process the numbers according to the instructions belowo Once you have handled the 3 input values, prompt the user againo Keep prompting the user for 3 integers until the user enters the single word DONEo Reading input should not stop for any reason except for when the user enters DONE.o The user will not mix DONE and the numbers. The user will either…
arrow_forward
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
1. Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local drive.
2. Create a new project named LabExer6A. Set the project location to your own folder.
3. Create a simple guessing game (similar to Hangman or Hangaroo). In this game, the user guesses
a letter and then attempts to guess the word.
4. Create a Notepad file named words.txt which will store any number of words each written per line.
5. The Java program shall:
• randomly select a word from the list saved in words.txt;
• display a letter in which some of the letters are replaced by ?; for example, ED??A??ON
(for EDUCATION);
place the letter in the correct spot (or spots) in the word each time the user guesses a letter
correctly;
• inform the user if the guessed letter is not in the word; and
display a congratulatory message when the entire correct word has been deduced.
arrow_forward
Discord Bot Python question
I am just working on a project to create a discord bot and I was wondering how to change the prefix to @botname
Right now, the prefix code is as below:
bot = commands.Bot(command_prefix="!", intents=intents)
Let's say the bot name is BOT.
I want to make it so that if I go
@BOT command
it would give me the result.
arrow_forward
Complete this javascript file according to the individual instructions given in the comments. *** DO NOT CHANGE any of the code that you are not instructed to. */ //////////////////////// // NOTE: Use the API endpoints available at // https://jsonplaceholder.typicode.com/ // to get the data required in these exercises. // HINT: Read the documented Resources and Routes. // Also the guide: // https://jsonplaceholder.typicode.com/guide/ /////////////////////// // 1) Create a function named "getAllPosts". // Use Fetch with Async/Await to request // all the posts. The function should // return all the posts as JSON data. // 2) Create a function named "getAllUsers". // Use Fetch with Async/Await to request // all the posts. The function should // return all the posts as JSON data. // 3) Create a function named "getComments". // This function needs to accept a "postID" // parameter. // Use Fetch with Async/Await to request // all the comments for the postID that // is passed in as a…
arrow_forward
The file Names.txt is located in the folder input_files within your project. The content of that file is shown in the following figure
Java:
import java.io.FileInputStream;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Question2 {public static void main(String[] args) throws FileNotFoundException {/*** Part a* Finish creating an ArrayList called NameList that stores the names in the file Names.txt.*/ArrayList<String> NameList;/*** Part b* Replace null on the right-hand-side of the declaration of the FileInputStream object named inputStream* so that it is initialized correctly to the Names.txt file located in the folder specified in the question description*/FileInputStream inputStream = null;Scanner scnr = new Scanner(inputStream); //Do not modify this line of code/*** Part c* Using a loop and the Scanner object provided, read the names from Names.txt* and store them in NameList created in Part a.*//*** Part d* Reorder the…
arrow_forward
Given a list of employees, create a simple program that allows the user to insert, delete, display,
and search an employee. The program should input 10 full names of employees with employee
number as linked list. Also, a list of menus should be displayed.
These menus are Insert, Delete, Display and Search.
A. Insert - allows the user to insert one employee at a time with corresponding ID number
which serves as their link. All employees to be inserted must be inserted at the
end of the list.
B. Delete – allows the user to delete the employee from the list
C. Display – allows the user to display the list of employees
D. Search - allows the user to find a certain employee
These menus should be in loop once you test every operation based on the listed menu.
Use linked list as a data structure in generating a list of employees, and
Perform basic operations that can be applied in a linked list
arrow_forward
It should be designed as a Desktop Application (with GUI) developed with Java programming language.Note that you are not designing a Web Application or a Mobile Application.Create a project named “FavoriteBooks” which keeps a list of the books that a user has read and liked.This list is stored in a text document (.txt) file and for each book it keeps the following data separated by acomma (,):Book id, Title, Category, First Author Name and Surname, Award Winner, Rating, Year, Number of Pages, andShort Description. Book id: The data type of “Book id” is integer and will be incremented automatically by 1 whenever anew book is added to the list. Title: The data type of “Title” is String. Category: The data type of “Category” is String. It shows the category of a Book such as Arts, Biography,History, Science Fiction, Cookbook, Literature, etc.. First Author Name and Surname: The data type of “Author Name and Surname” is String. Award Winner: The data type of “Award” is String. If the…
arrow_forward
Q.Create an interesting educational information chat box that asks the user to give an input question and then answers the question given by user with a suitable answer. You can make use of list for different questions and answers.Save two to three answers for a single question and then for random selection of elements from those answers use random choice(list) method from random module that returns a random element. Also for a user question that doesn't matches with the questions present in the chat box it should display statement like "oops i can't answer that" "sorry i am not intelligent enough" "could u please ask something else" using random method so that every time one of these statements appear
**coding language python
***try using basic programming techniques in python without using arrays and pointers
* * * copy paste the program itself and also the screenshot of program and output
* * * use any type of data for questions such as name details of cities species etc depends on…
arrow_forward
The questions should be solved using Linux Bash Scripting. In the file there should be solution code and one/two screenshot for running of program. Student id and names should be written inside the file. The assignment should be sent as PDF. Upload Link: https://forms.office.com/r/JfpSa2dDi8 Question 1. Read "n" and generate following pattern according to givennas below. Ex: for given value forn:4\[ \begin{array}{l} 1 \\ 23 \\ 456 \\ 78910 \end{array} \]
arrow_forward
I need help with this one please, already tried but still getting a wrong answer
Scenario
You have been tasked with building a URL file validator for a web crawler. A web crawler is an application that fetches a web page, extracts the URLs present in that page, and then recursively fetches new pages using the extracted URLs. The end goal of a web crawler is to collect text data, images, or other resources present in order to validate resource URLs or hyperlinks on a page. URL validators can be useful to validate if the extracted URL is a valid resource to fetch. In this scenario, you will build a URL validator that checks for supported protocols and file types.
Task
Create two lists of strings – one list for Protocol called valid_protocols, and one list for storing File extension called valid_fileinfo. For this take the protocol list should be restricted to http, https and ftp. The file extension list should be .html, .csv and .docx.
Split an input named url, and then use the first…
arrow_forward
Dim x As Integer = 3
Private Sub Form1_Load(sender As System.Object, e As System. EventArgs) Handles MyBase.Load
x += 5
f1()
MsgBox (x)
End Sub
Sub f1()
X += 5
f2()
End Sub
Sub f2()
Dim x = 10
x += 5
End Sub
15
10
13
arrow_forward
.Write code for below problem. The input below is just an example and you should implement
independent from the input. Please paste the link to the answer shared using pastebin,
dotnetfiddle, jsfiddle, or any other similar web-site.
You will have an orthogonal triangle input from a file and you need to find the maximum sum of the numbers
according to given rules below;
1. You will start from the top and move downwards to an adjacent number as in below.
2. You are only allowed to walk downwards and diagonally.
3. You can only walk over NON PRIME NUMBERS.
4. You have to reach at the end of the pyramid as much as possible.
5. You have to treat your input as pyramid.
According to above rules the maximum sum of the numbers from top to bottom in below example is 24.
*1
*8 4
2 *69
85*9 3
As you can see this has several paths that fits the rule of NOT PRIME NUMBERS; 1>8>6>9, 1>4>6>9, 1>4>9>9
1 + 8 + 6 + 9 = 24. As you see 1, 8, 6, 9 are all NOT PRIME NUMBERS and walking over these yields the…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
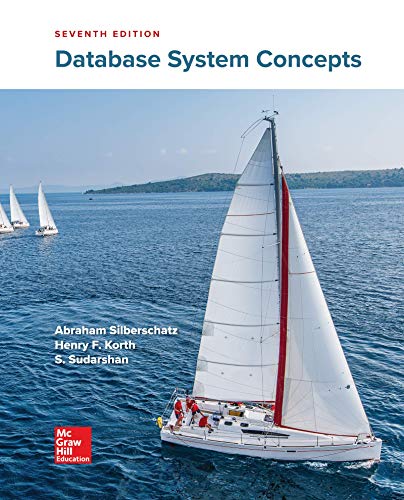
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
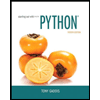
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
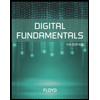
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
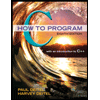
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
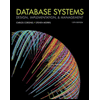
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
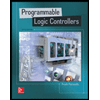
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Assignment3A: Diagnosis Checker: For years, patients have used websites like WebMD to pre-diagnosis themselves with a variety of illnesses before meeting with a doctor. As you might imagine, medical professionals believe this can lead to incorrect treatment. To combat this, a local hospital has asked you to develop a program that will help patients check if their symptoms match the online diagnosis. You will prompt the user to enter their illness. Based on this input, you will ask them if they are experiencing certain symptoms. Depending on their answer, your program will indicate if the Internet diagnosis is likely to be correct or not. The decision chart is shown below: • ● ● HGPS 0 Do they have brittle bones? If no, it is likely not HGPS ■ If yes, it is possibly HGPS Bokter Syndrome O Do they have a Vitamin C deficiency? If no, it is likely not Bokter Syndrome If yes, are they a sailor? . . Lupus . If no, it is likely not Bokter Syndrome If yes, it is possibly Bokter Syndromearrow_forwardAt the end of this specific task, students should be able to:• Create and work with Loops• Handle and manipulate strings • (Learning Units 4 and 5).It is good practice to leave your main branch as your long live branch, this means that the code on this branch is always in perfect working order and tested. We make use of feature branches in order to ensure that any code we push to GitHub does not break our main branch. You can create a feature branch by running the following command.git checkout -b KhanbanTasks (you can use any branch name)** You are welcome to make use of GitHub desktop or your IDE to push code to GitHub if you are not comfortable with using the command line.You can now add the following functionality to your application:1. The users should only be able to add tasks to the application if they have logged in successfully.2. The applications must display the following welcome message: “Welcome to EasyKanban”.3. The user should then be able to choose one of the following…arrow_forwardastfoodStats Assignment Description For this assignment, name your R file fastfoodStats.R For all questions you should load tidyverse, openintro, and lm.beta. You should not need to use any other libraries. suppressPackageStartupMessages(library(tidyverse)) suppressPackageStartupMessages(library(openintro)) suppressPackageStartupMessages(library(lm.beta)) The actual data set is called fastfood. Continue to use %>% for the pipe. CodeGrade does not support the new pipe. Round all float/dbl values to two decimal places. All statistics should be run with variables in the order I state E.g., "Run a regression predicting mileage from mpg, make, and type" would be: lm(mileage ~ mpg + make + type...) To access the fastfood data, run the following: fastfood <- openintro::fastfood Create a correlation matrix for the relations between calories, total_fat, sugar, and calcium for all items at Sonic, Subway, and Taco Bell, omitting missing values with na.omit(). Assign the…arrow_forward
- java programmingarrow_forwardhow to correct this exercise. COP2002: Programming Logic & Design with PythonProject 4Project: Download and save the file named proj_4_to_fix_wishes.py. The program has five errors including a syntax error, runtime errors, and logic errors. Find and fix all the errors. You must also document the errors you found and how you fixed them. Since you can only upload a single file into Canvas, put the explanation of the errors at the top of the program, as comments. The output of your fixed program should look as shown:Sample Console Display: Notes• You are not required to validate if the user enters a character or a string. The only validation is the one in the program: the user must enter values equal to or greater than 0.• You do not need to validate the user's response to the question, "Enter an item? y/n". If the user enters anything other than 'y' or 'n' the program can simply end.• Save the corrected file with the filename xxx_wishes_fixed.py (where xxx = your initials) or as…arrow_forwardStep1-Study the scenario below and create a Java Program for Builders Warehouse, The program must consist of a class called Materials to handle the details of the materials. Step 2-The program should read the materials details from the material.txt file then use a LinkedList to store the material details. Step 3-Then finally the products stored in the LinkedList must be displayed like the sample code below: Step 4-There must be clear code and a screenshot of the code running.arrow_forward
- Create a new C++ project in Visual Studio (or an IDE of your choice). Add the files listed below to your project: BinaryTree.h Download BinaryTree.h BinarySearchTree.h Download BinarySearchTree.h main.cpp Download main.cpp Build and run your project. The program should compile without any errors. Note that function main() creates a new BST of int and inserts the nodes 12, 38, 25, 5, 15, 8, 55 (in that order) and displays them using pre-order traversal. Verify that the insertions are done correctly. You may verify it manually or use the visualization tool at https://www.cs.usfca.edu/~galles/visualization/BST.html. (Links to an external site.) Do the following: 1. add code to test the deleteNode and search member functions. Save screenshot of your test program run. 2. add code to implement the nodeCount member function of class BinaryTree. Test it and save screenshot of your test program run. 3. add code to implement the leafCount member function of class BinaryTree. Test it and…arrow_forwardomplete this javascript file according to the individual instructionsgiven in the comments. *** DO NOT CHANGE any of the code that you are not instructed to. */ ////////////////////////// NOTE: Use the API endpoints available at// https://jsonplaceholder.typicode.com/// to get the data required in these exercises.// HINT: Read the documented Resources and Routes.// Also the guide:// https://jsonplaceholder.typicode.com/guide//////////////////////// Create a function named "getUser".// This function needs to accept a "userID"// parameter.// Use Fetch with Async/Await to request// the data for the requested user.// The function should return JSON data.arrow_forwardcreate a script that will parse data from Rotten Tomatoes, a movie reviews website. The work you have to do is identical to what we covered in lectures 4 and 5, albeit for a different website. Please read and follow the instructions below very carefully. Step 1 Your script should begin by defining two variables (after importing libraries, etc) movie a string variable indicating the movie for which reviews will be parsed pageNum the number of review pages to parse For example, to parse the first 3 pages of the Gangs of New York reviews, set movie ‘’gangs_of_new_york” and pageNum = 3. Your code should go to the movie’s All Critics reviews page of rotten tomatoes, and parse the first three pages of reviews. Pagination on rotten tomatoes happens by clicking on the “Next” button. Step 2 For each review contained in each of the pages you requested, parse the following information The critic This should be 'NA' if the review doesn't have a critic’s name. The rating. The rating…arrow_forward
- In c# programarrow_forwardWrite a program that creates a login name for a user, given the user's first name, last name, and a four-digit integer as input. Output the login name, which is made up of the first five letters of the last name, followed by the first letter of the first name, and then the last two digits of the number (use the % operator). If the last name has less than five letters, then use all letters of the last name. Ex: If the input is: Michael Jordan 1991 the output is: Your login name: JordaM91 Ex: If the input is: Kanye West 2024 the output is: Your login name: WestK24arrow_forwardQ4. The attached Java program (Recursion_Demo.java) (C# version is also attached) demonstrates the concept of recursion. The program inputs the value of n from the user and then the recursive RDemo method prints the following sequence: n (n-1) (n-2)... 1123... n Execute the program and check it out for yourself. You will write a MIPS program which MUST use a non-leaf procedure to implement this recursive method. Your program will define a non-leaf procedure RDemo which will be called recursively for n>=1. RDemo will return when n<1. Your program will print the user prompt and take n as an input in the main program. The output on the screen must be just similar to the Java program - there will be space between the integers. Obviously, your MIPS program will not implement the concept of class. (See next page)arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
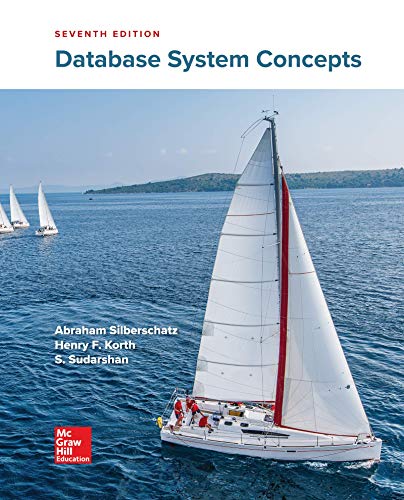
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
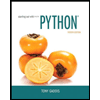
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
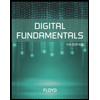
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
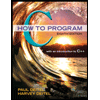
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
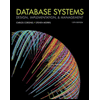
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
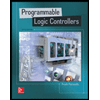
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education