Heffron_Joseph_Lab_05
.docx
keyboard_arrow_up
School
University of Cincinnati, Main Campus *
*We aren’t endorsed by this school
Course
1090C
Subject
Computer Science
Date
Feb 20, 2024
Type
docx
Pages
9
Uploaded by AgentJackal749
IT 1090C Computer Programming I IT 6090C Java Programming Prof. Tom Wulf
Lab 05 Conditionals 20 pts (3 gr or extra credit pts)
Learning Goals:
Conditional Structures in Java if, if else, cascaded if, nested if, switch
Input with Scanner
Testing. Make sure your programs run by testing them. Correct them if they do not.
This is an individual lab. Complete each of the programs here. (I may do the first with you in class.) Note that these are programs that we previously did as pseudo code. Copy or re-write the pseudo code as single line // java comments as an outline for your program. Create a separate IntelliJ java project for each program using the names I specified.
Don’t forget to code the pseudo code for your program first within the java main() section as single line comments // comment….. Then code the java statements for the pseudo code. In each
task, use a test suite that covers each of the logical paths the program can take. e.g. (for the first program) user enters an item less than $100, or user enters one > $100 Your screen shots should support that you did this testing! (Provide shots for each case, for each task.)
We will use scanner for input. Since we cant loop yet, we can only halt the program under control if the user makes an error. Later, we can loop back and repeat until they get it right.
If your instructor directed you to use GitHub create a single repo for the lab. All University Students should be using GitHub.
Mini lecture:
We use the Scanner class to get input from the user through the console. We have to import the Scanner class and create an instance of Scanner using the default input stream System.in.
import java.util.Scanner;
// imports go at the top of the file BEFORE the class!
Scanner in = new Scanner(System.in);
// it is very common to name the Scanner “in” or console
// Scanner has a variety of methods that read data of a specific Java type: int val = in.
nextInt()
; // reads an int value throws an error if the input cannot be an int
Copyright © 2019-2020, University of Cincinnati, Ohio. All rights reserved.
double doubleVal = in.
nextDouble()
; // reads a double value error if the input isn’t a double
String line = in.
nextLine
(); // reads an entire line to a \n newline character as a String
String word = in.
next
(); // reads part of the line up to a delimiter (space) or the \n …
The methods that return numeric types can throw an error if the input cannot be parsed into the correct type. The following methods allow you to safely determine if you can successfully read the numeric value:
in.
hasNextInt();
// returns true if nextInt will succeed
in.
hasNextDouble();
// returns true if nextDouble will succeed Since anything the user types can be read in as a legal java String, the next() and nextLine() methods cannot generate an error. We will need to use the java if and if .. else structures for this. So let’s take a look at them now.
if (CONDITION)
{
Code statements in this block execute if the CONDITION is true
}
// this is the end of the block like the endIf in our pseudo code
And here is the if..else:
if (CONDITION)
{
Code statements in this block execute if the CONDITION is true
}
else
{
Code statements in this block execute if the CONDITION is false
} // this is the end of the block like the endIf in our pseudo code
C
ascaded if:
if(CONDITION)
{
}
else if (CONDITON)
{
}
// more else if (CONDITON) blocks go here
Copyright © 2019-2020, University of Cincinnati, Ohio. All rights reserved.
else // no condition a default when all the other if tests fail
{
}
Here is an example code fragment that shows how to safely input a number:
import java.util.Scanner; // before the class at top of the file
// this code is in main:
Scanner in = new Scanner(System.in); // create a Scanner in to read from the console
double wage = 0;
System.out.print(“Enter your hourly wage: “);
if(in.hasNextDouble())
{
// OK safe to read in a double
wage = in.nextDouble(); in.nextLine(); // clear the buffer
System.out.println(“\nYou said your wage was: “ + wage);
}
In this example (above) we leave wage equal to 0 if the user enters something other than a valid amount. (i.e. double)
Here is a better approach with if else:
import java.util.Scanner; // before the class at top of the file
// this code in main:
Scanner in = new Scanner(System.in);
double wage = 0;
String trash = “”; // use for bad input which will read as a String
System.out.print(“Enter your hourly wage: “);
if(in.hasNextDouble())
{
// OK safe to read in a double
wage = in.nextDouble(); Copyright © 2019-2020, University of Cincinnati, Ohio. All rights reserved.
in.nextLine(); // clears the newline from the buffer
}
else
{ // Not a double can’t use nextDouble() read as String with nextLine() instead
trash = in.nextLine(); // Ok have to read the input as a String
System.out.println(“\nYou said your wage was: “ + trash);
System.out.println(“Run the program again and enter a valid amount!”);
}
As you test each program, you have to run it multiple times. Instead of screen shots, just copy the output window from IntelliJ into this doc for EACH test run. There will be several
for each program. Please make sure that your output is readable, that’s what we use to grade your work.
Task 1 (5 pts): Project name: ShipCostCalculator
[Create a new Java file with this name and include a main method OR rename the existing main.java. Add your new file to the GitHub repo and commit it.]
An application program where the user enters the price of an item and the program computes shipping costs. If the item price is $100 or more, then shipping is free otherwise it is 2% of the price. The program should output the shipping cost and the total price.
Test runs: (insert the output widow copies here for the test runs)
- valid input less than 100
- valid input greater than 100
Be sure to add the file to the repo and commit and push it.
Copyright © 2019-2020, University of Cincinnati, Ohio. All rights reserved.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
PROBLEM STATEMENT:
An anagram is a word that has been rearranged from another word, check tosee if the second word is a rearrangement of the first word.
public class AnagramComputation{public static boolean solution(String word1, String word2){// ↓↓↓↓ your code goes here ↓↓↓↓return true;}}
Can you help me with this java question the language is java please use the code I gave
arrow_forward
Please answer 6 and 7 as they are associated altogether.
6. The FixProgram.java below has some errors.a. Fix the errors so that the program successfully compiles and runs.
class FixProgram{ public static void main(String[] args) { System.out.println('Hello World!') }}
7. Change the SampleProgram.java program below.a. So that it displays Programming is fun! Instead of Hello World!
class SampleProgram{ public static void main(String[] args) { System.out.println("Hello World!"); }}
arrow_forward
Explain More on Expressions in while Statements.
arrow_forward
Complete the following sentences.
MATLAB includes a special debugging tool called a
debugger, which is embedded into the Edit/Debug Window. This tool allows you to walk through the execution of your program one statement
at a time, and to examine the values of any variables at each step along the way.
arrow_forward
White-box testing is defined as. Is it required to do white-box tests?
arrow_forward
very fast
arrow_forward
What advantages do assert statements have?
arrow_forward
"Cohesion" and "Coupling" are used interchangeably in the field of computer programming.
arrow_forward
Don't reject the question.
Provide answer step by step
arrow_forward
You are tasked to researched about the conditional operator in java. You are to give the meaning, syntax and a sample program. You are also to tasked to compare it with if and switch in terms of functionality and use.
arrow_forward
The design pattern Singleton is mainly used to avoid the new
operator in Java.
True
O False
arrow_forward
*** Complete in Java Program**
Use Gambler's Ruin Example
Gambler's ruin. Suppose a gambler makes a series of fair $1 bets, starting with $50, and continue to play until she either goes broke or has $250. What are the chances that she will go home with $250, and how many bets might she expect to make before winning or losing? Gambler.java is a simulation that can help answer these questions. It takes three command-line arguments, the initial stake ($50), the goal amount ($250), and the number of times we want to simulate the game.
Implement and modify Gambler's ruin simulation from the textbook or textbook website. Modify, to show the results from several simulations not only one, in a formatted table. Compute the average over all simulations.
arrow_forward
please code in java and dont use advanced coding.. keep it basic
arrow_forward
True or False:
(a) In a debugger, we can choose whether to step into, step over, or step out of a particular method call.
(b) Debuggers, tests, and println() statements are all legitimate ways to help us find errors in our code.
arrow_forward
None
arrow_forward
Small Program assignment based on basic java features (Class, Object, Constructor, Method, Output, If-else, Loop, Array Etc)
Note: Only use those above mention .
arrow_forward
Please show an example of how or how to solve coding the problem from Starting out with Java from control structures through data structures 4th edition: chapter 20 programming challenge 4
arrow_forward
Write short note on:
i. Name Mangling
ii.Conversion Constructor
iii. Explict Consructor
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
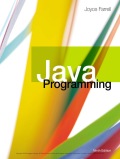
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Related Questions
- PROBLEM STATEMENT: An anagram is a word that has been rearranged from another word, check tosee if the second word is a rearrangement of the first word. public class AnagramComputation{public static boolean solution(String word1, String word2){// ↓↓↓↓ your code goes here ↓↓↓↓return true;}} Can you help me with this java question the language is java please use the code I gavearrow_forwardPlease answer 6 and 7 as they are associated altogether. 6. The FixProgram.java below has some errors.a. Fix the errors so that the program successfully compiles and runs. class FixProgram{ public static void main(String[] args) { System.out.println('Hello World!') }} 7. Change the SampleProgram.java program below.a. So that it displays Programming is fun! Instead of Hello World! class SampleProgram{ public static void main(String[] args) { System.out.println("Hello World!"); }}arrow_forwardExplain More on Expressions in while Statements.arrow_forward
- Complete the following sentences. MATLAB includes a special debugging tool called a debugger, which is embedded into the Edit/Debug Window. This tool allows you to walk through the execution of your program one statement at a time, and to examine the values of any variables at each step along the way.arrow_forwardWhite-box testing is defined as. Is it required to do white-box tests?arrow_forwardvery fastarrow_forward
- You are tasked to researched about the conditional operator in java. You are to give the meaning, syntax and a sample program. You are also to tasked to compare it with if and switch in terms of functionality and use.arrow_forwardThe design pattern Singleton is mainly used to avoid the new operator in Java. True O Falsearrow_forward*** Complete in Java Program** Use Gambler's Ruin Example Gambler's ruin. Suppose a gambler makes a series of fair $1 bets, starting with $50, and continue to play until she either goes broke or has $250. What are the chances that she will go home with $250, and how many bets might she expect to make before winning or losing? Gambler.java is a simulation that can help answer these questions. It takes three command-line arguments, the initial stake ($50), the goal amount ($250), and the number of times we want to simulate the game. Implement and modify Gambler's ruin simulation from the textbook or textbook website. Modify, to show the results from several simulations not only one, in a formatted table. Compute the average over all simulations.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
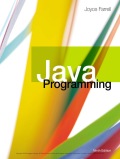
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT