CSC401_A2_v1
.pdf
keyboard_arrow_up
School
University of Toronto *
*We aren’t endorsed by this school
Course
401
Subject
Computer Science
Date
Feb 20, 2024
Type
Pages
9
Uploaded by HighnessTankLobster37
Computer Science 401
10 February 2024
St. George Campus
University of Toronto
Homework Assignment #2
Due: Friday, 8 March 2024 at 23h59m (11.59 PM),
Neural Machine Translation (MT) using Transformers
Email
:
csc401-2024-01-a2@cs.toronto.edu
. Please prefix your subject with
[CSC401
W24-A2]
.
All accessibility and extension requests should be directed to
csc401-2024-01@cs.toronto.edu
.
Lead TAs
: Julia Watson and Arvid Frydenlund.
Instructors
: Gerald Penn, Sean Robertson and Raeid Saqur.
Building the Transformer from Scratch
It is quite a cliche to say that the transformer architecture has revolutionized technology. It is the building
block that fuelled the recent headline innovations such as ChatGPT. Despite all those people who claimed
to work in a large language model, only a very selected and brilliant few understand and are familiar with
its internal workings. And you — who came from the prestigious and northern place called the University
of Toronto —
must
carry on the century-old tradition to be able to create a transformer-based language
model using only pen and paper.
Organization
You will build the transformer model from beginning to end, and train it to do some
basic but effective machine translation tasks using the Canadian Hansards data. In
§
1, we will guide you
through the process of implementing all the
building blocks
of a transformer model. In
§
2, you will use
the building blocks to put together the transformer
architecture
.
§
3 discusses the
greedy and beam
search
for decoded target sentence generation.
In
§
4, you’ll
train and evaluate
the model.
Finally,
in
§
5, you’ll use the trained mode to do some real machine translation and write-up your
analysis
report.
Goal
By the end of this assignment, you will acquire low-level understanding of the transformer archi-
tecture and implementation techniques of the entire data processing
→
training
→
evaluation pipeline of
a functioning AI application.
Starter Code
Unlike A1, the starter code of this assignment is distributed through MarkUs.
The
training data is located at
/u/cs401/A2/data/Hansard
on
teach.cs
.
We use Python version 3.10.13 on
teach.cs
.
That is, just run everything with the default
python3
command. You may need to add
srun
commands to request computational resources — please follow the
instructions in the following sections to proceed. You shouldn’t need to set up a new virtual environment
or install any packages on
teach.cs
.
You can work on the assignment on your local machine, but you
must make sure that your code works on
teach.cs
. Any test cases failed due to incompatibility issues will
not receive any partial marks.
Marking Scheme
Please see the
A2
rubric.pdf
file for detailed breakdown of the marking scheme.
Copyright
©
2024 University of Toronto. All rights reserved.
1
1
Transformer Building Blocks and Components [12 Marks]
Let’s start from the three types of building blocks of a transformer: the layer norm, multi-head attention
and the feed-forward modules (a.k.a. the MLP weights).
LayerNorm
The normalization layer computes the following.
Given an input representation
h
, the
normalization layer computes its mean
µ
and the standard deviation
σ
. Then, it outputs the normalized
features.
h
←
γ
(
h
−
µ
)
σ
+
ε
+
β
(1)
Using the instructions, please complete the
LayerNorm.forward
method.
FeedForwardLayer
The feed-forward layer is a two-layer fully connected feed-forward network.
As
shown in the following equation, the input representation
h
is fed through two layers of fully connected
layers. Dropout is applied after each layer, and ReLU is the activation function.
h
←
dropout(ReLU(
W
1
h
+
b
1
))
h
←
dropout(
W
2
h
+
b
2
)
(2)
Using the instructions, please complete the
FeedForwardLayer.forward
method.
MultiHeadAttention
Finally, you need to implement the most complicated but important component
of the transformer architecture: the multi-head attention module.
For the base case where there’s only
H
= 1 head, the attention score is calculated using the regular cross-attention algorithm:
dropout(softmax(
QK
⊤
√
d
k
))
V
(3)
Using the instructions, please complete the
MultiHeadAttention.attention
method.
Then, you need to implement the part where the query, key and value are split into
H
heads, and then
pass them through the regular cross-attention algorithm you have just implemented.
Next, you should
combine the results. Don’t forget to apply the linear combination and dropout when you output the final
attended representations.
Using the instructions, please complete the
MultiHeadAttention.forward
method.
2
Putting the Architecture (Enc-Dec) Together [20 Marks]
OK, now we have the building blocks, let’s put everything together and create the full transformer model.
We will start from a single transformer encoder layer and a single decoder layer.
Next, we build the
complete encoder and the complete decoder together by stacking the layers together. Finally, we connect
the encoder with the decoder and complete the final transformer encoder–decoder model.
TransformerEncoderLayer
You need to implement two types of encoder layers. Pre-layer normaliza-
tion (Figure 1a), as its name suggests, applies layer normalization before the representation is fed into the
next module. On the other hand, post-layer normalization (Figure 1b) applies layer normalization after
the representation is fed into the representation.
Using the instructions, please complete the
pre
layer
norm
forward
and the
post
layer
norm
forward
methods of the
TransformerEncoderLayer
class.
2
input:
h
Layer Norm
Multi-head Attention
Layer Norm
Feed-Forward
output:
h
+
+
(a) Pre-layer normalization for encoders.
input:
h
Multi-head Attention
Layer Norm
Feed-Forward
Layer Norm
output:
h
+
+
(b) Post-layer normalization for encoders.
Figure 1: Two types of
TransformerDecoderLayer
.
TransformerEncoder
You don’t need to implement the encoder class. The starter code contains the
implementation. Nonetheless, it will be helpful to read it, as it will a good reference for the following tasks.
TransformerDecoderLayer
Again, you need to implement both pre- and post-layer normalization.
Recall from lecture, there are two multi-head attention blocks for decoders. The first one is a self-attention
block and the second one is a cross-attention block.
Using the instructions, please complete the
pre
layer
norm
forward
and the
post
layer
norm
forward
methods of the
TransformerDecoderLayer
class.
input:
h
Layer Norm
Self Attention
Layer Norm
Cross Attention
Layer Norm
Feed-Forward
output:
h
+
+
+
(a) Pre-layer normalization for decoders.
input:
h
Self Attention
Layer Norm
Cross Attention
Layer Norm
Feed-Forward
Layer Norm
output:
h
+
+
+
(b) Post-layer normalization for decoders.
Figure 2: Two types of
TransformerEncoderLayer
.
TransformerDecoder
Similar to
TransformerEncoder
, you should pass the input through all the de-
coder layers. Make sure to add the LayerNorm module in the correct place depending if the model is pre-
or post-layer normalization. Finally, don’t forget to use the logit projection module on the final output.
3
Using the instructions, please complete the
TransformerDecoder.forward
method.
TransformerEncoderDecoder
After making sure that the encoder and the decoder are both built
properly, it’s time to put them together. You need to implement the following methods:
•
The method
create
pad
mask
is the helper function to pad a sequence to a specific length.
•
The method
create
causal
mask
is the helper function to create a causal (upper) triangular mask.
•
After finishing the two helper methods, you can implement the
forward
method that connect all the
dots. In particular, you first create all the appropriate masks for the inputs. Then, you feed them
through the encoder.
And, finally, you can get the final result by feeding everything through the
decoder.
3
MT with Transformers: Greedy and Beam-Search [20 Marks]
The
a2
transformer
model.py
file contains all the required functions to complete and detailed instructions
(with hints). Here we list the high-level methods/functions that you need to complete.
3.1
Greedy Decode
Let’s warm up by implementing the greedy algorithm. At each decoding step, compute the (log) proba-
bility over all the possible tokens. Then, choose the output with the highest probability and repeat the
process until all the sequences in the current mini-batch terminate.
Using the instructions, please complete the
TransformerEncoderDecoder.greedy
decode
method.
3.2
Beam Search
Now, it’s time for perhaps the hardest part of the assignment – beam search. But don’t worry, we have
broken everything down into smaller, and much simpler chunks. We will guide you step by step to complete
the entire algorithm.
Beam search is initiated by a call to the
TransformerEncoderDecoder.beam
search
decode
method call.
Recall from the lecture that its job is to generate
partial translations
(or,
hypotheses
) from the source tokens
during the decoding phase. So, the
beam
search
decode
method gets called whenever you are trying to gen-
erate decoded translations (e.g. from
TransformerRunner.[translate, compute
average
bleu
over
dataset]
etc.).
Complete the following functions in the
TransformerEncoderDecoder
class:
1.
initialize
beams
for
beam
search
: This function will initialize the beam search by taking the first
decoder step and using the top-k outputs to initialize the beams.
2.
expand
encoder
for
beam
search
: Beam search will process ‘batches‘ of size ‘batch
size * k‘ so we
need to expand the encoder outputs so that we can process the beams in parallel. (
Tip
: You should
call this from within the preceding function).
3.
repeat
and
reshape
for
beam
search
: It’s a relatively simple expand and reshaping function. See
how it’s called from the
.beam
search
decode
method and read the instructions in function com-
ments. (
Tip
: Review Torch.Tensor.expand).
4.
pad
and
score
sequence
for
beam
search
:
This function will pad the sequences with eos and
seq
log
probs with corresponding zeros.
It will then get the score of each sequence by summing
4
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Below are the files needed for the assignment. Thank you in advance. I've used my textbook, looked up guides, and reviewed my notes already.
FILE #1
<!DOCTYPE html>
<!--
Student Name: Jordan Collins
File Name: index.hmtl
Date: 10/13/2021
-->
<html lang="en">
<head>
<title>CH 4 Extend Your Knowledge</title>
<meta charset="utf-8">
<link rel=“stylesheet” href=“css/styles.css”>
</head>
<body>
<header>
<h1>CSS Colors</h1>
</header>
<main>
<div id="color">
<div id="orange">
<h2>Orange</h2>
<div class="box orange-hex">Hex Orange</div>
<div class="box orange-rgb">RGB Orange</div>
<div class="box orange-hsl">HSL Orange</div>
</div>
<div…
arrow_forward
Html code for sure in this design java
.
arrow_forward
Create a PHP page name it print.php
- This page should be reachable via the following
url: localhost/<std name>/<std id>/print
where std name has at least one char (no numbers) and std id has the format id-20xyz; xyz are three numbers
When a request send to print.php, then print student name and her/his id.
localhost/tara/id-20222/print
arrow_forward
JavaScript
arrow_forward
Estem.org/courses/64525/assignments/9460783?module_item_id=18078917
The following information can help you get started:
• Invitation Details: it boils down to when and where
o When: Time and date
• Where: Address
• Invitee List: Name and email
• Name: First Name, or First Name and Last Name
Email: Email address
. Other considerations:
After you complete your invitation, answer the following questions:
1. What type of data are time, date, and place? How are they different from the other data types on the
invite and guest list?
F4
A
Additional information worth including: dress code, directions, gifting, how to contact you.
. How will you know who is showing up? RSVP?
. Is there a theme to your invitation/design?
x
F5
%
F6
F7
DELL
F8
F9
ROMNA
F10
F11
PrtScr
arrow_forward
https://drive.google.com/file/d/1WvadErM-1ffp8gm2LcdqdMrtZ0gv3fJv/view?usp=sharing
here in that link there is my code can please add comments to my code and describe me what is happening
arrow_forward
JavaScript Dom Manipulation
Hi can anyone please help me fix my main.js file. We're not allowed to change anything in index.html. We have to make a button so that every time you click on it the count on the web page increases by one.
My code doesn't work and I don't know why. Every time I click the button, nothing happens. We just started learning about DOM and the only method we've learned so far is document.querySelector() Please help me understand dom events better. thank you so much!
var numTimesClicked = 0;
/* function handleClick(event) {
numTimesClicked++;
console.log('button clicked');
console.log('value of numTimesClicked:', numTimesClicked);
}
var $hot = document.querySelector('.hot-button');
var $click = document.querySelector('.click-count');
$click.addEventListener('click', handleClick); */
function handleClick(event) {
numTimesClicked++;
console.log('button clicked');
console.log('event:', event);
console.log('event.target', event.target);
}
var…
arrow_forward
JavaScript Dom Manipulation
Hi can anyone please help me fix my main.js file. We're not allowed to change anything in index.html. We have to make a button so that every time you click on it the count on the web page increases by one.
My code doesn't work and I don't know why. Every time I click the button, nothing happens. We just started learning about DOM and the only method we've learned so far is document.querySelector() Please help me understand dom events better. thank you so much!
main.js
var numTimesClicked = 0;
function handleClick(event) {
numTimesClicked++;
console.log('button clicked');
console.log('event:', event);
console.log('event.target', event.target);
}
var $click = document.querySelector('.click-count');
$click.addEventListener('click', handleClick);
function handleMouseover(event) {
console.log('button hovered');
console.log('event:', event);
console.log('event.target', event.target);
}
index.html
<!DOCTYPE html>
<html…
arrow_forward
Please write php and html code just
Thank you
arrow_forward
Setup
Branch name
assignment1
Directory
assignment1
Package name
assignment1
Instructions
Create a node server with the following requirements:
Use the dotenv package to manage your development environment variables.
PORT should be 3000
HOST should be localhost
Endpoints
/dotted
Only GET requests are allowed.
This endpoint will respond with the HTML content type.
This endpoint will take two required query parameters: word1 and word2.
The endpoint will take the two words and create a string that is the two words separated by enough “.” characters to make the length of the string 30.
For example if word1 is “turtle” and word2 is “153” the output should be: turtle.....................153
The response body should be the string wrapped in a <pre> tag.
/fizzBuzz
Only GET requests are allowed.
This endpoint will respond with the HTML content type.
This endpoint will take two required query parameters: start and end.
The endpoint will iterate from start to end and for each…
arrow_forward
A02-02
Create a webpage A2-Task2.html.
The webpage is displaying one image. Each time the user moves the mouse out of the image, the image is
changed to another one and one more 'sun' emoji is displayed under the image. When the number of 'suns'
reaches seven (7), all suns are deleted (reset) and start to be displayed again. For example, the 8th time user
moves the mouse out of the image, one 'sun' is shown, the 9th time - two 'suns', and so on.
Note:
- You should use two images. Each time the user moves the mouse out of the image, the image is changing. If
an image of "Winter" is displayed it will change to "Summer", and opposite, if an image of "Summer" is
displayed it will change to "Winter".
The special character code for the 'sun' is "☀".
-
Put the source code of your website A2-Task2.html here.
Make sure you also upload the A2-Task2.zip of all files below.
arrow_forward
HTML table created by PHP
CSS to set background color to "lightblue"
PHP: create strings representing numbers in various bases
Behavior: The page will look like this. The items are decimal and hexadecimal numbers from 1-20. The items are hexadecimal results of multiplication.
Sample output:
HW02 - Multiplication Table
Matrix
3
4
5
5 5 af
6
1234567 8 9 10 11 12 13 14 15 16 17 18 19 20
Multiply 123456789abcd ef 10 11 12 13 14
1 1 2 3 4 5 6 7 8 9 a b c d e f 10 11 12 13 14
22468 ac e 10 12 14 16 18 la lc le 20 22 24 26 28
3 3 6 9 cf 12 15 18 lb le 21 24 27 2a 2d 30 33|36|39|3c
4 4 8 C 10 14 18 lc 20 24 28 2c 30 34 ||38 ||3c||40||44||48||4c_||50
1419 le 23 28 2d 32 37 3c|41|46|4b|50|55|5a|5f|64
66 c 12 18 le 24 2a 30 36 3c 42 48|4e||54|5a 60 ||66 ||6c 72 78
7 7 e 15 lc 23 2a 31 38 3f 46 4d 54 5b ||62|69|70|77|7e||85 ||8c
8 8 10 18 20 28 30 38 40 48 50 58 60 68 70 78 80 88 90 98 a0
12 lb 24 2d 36 3f 48 51 5a 63 6c 75 7e 87 90 99 a2 ab b4
14 le 28 32 3c 46 50 5a 64 6e 78 82 8c 96 a0 laa b4…
arrow_forward
In python code, Using Index.html , use Regex to extract the text between the <li> html tags.
Index.html: Dwarf Planets
Pluto
Charon
Eris
Makemake
Ceres
Pluto (minor-planet designation: 134340 Pluto) is a dwarf planet in the Kuiper belt, a ring of bodies beyond the orbit of Neptune.Charon is almost half the size of Pluto. The little moon is so big that Pluto and Charon are sometimes referred to as a double dwarf planet system.Makemake is a member of a group of objects that orbit in a disc-like zone beyond the orbit of Neptune called the Kuiper Belt.Eris is the most massive and second-largest known dwarf lanet in the Solar System. It is a trans-Neptunian object in the scattered disk and has a high-eccentricity orbit.Ceres is a dwarf planet in the asteroid belt between the orbits of Mars and Jupiter.
arrow_forward
create this html page
image link
1
https://e.edim.co/205361572/aa3jBg6koe3i4Mf2.jpg?response-content-disposition=filename%3D%22iceland3.jpg%22%3B%20filename%2A%3DUTF-8%27%27iceland3.jpg&Expires=1641780938&Signature=TBGCpf9zKPkrR4zpG5dRqZlXJljdmhh2gjljcAGezSrwploAsEgZBFkGGmsMOLqZo7QUf1XB~zxmlMuj-AQkjBCn9cq3h~bzDB57MCEyBdCTxeJ6IGjBiKCICbXU-gsDdYNvKXNefF0LHv8tDqoxW0HoT73l5AjlamgFK17FHxodv~LvwINaFgDKtS80ZCZtKxBEHIecYqhQMI2Zs87oAlWgLCX8fYwZcqprxuWmgB-8Zi-lRtNS9PaPMQHiDkifq76xDzkn0vJcp1cUwG~WpIrKrQ8pUlPPs8atw93idqcg6C5K3bJbU0iF5ylQIiVs9MpNoATUikwJvd~JFrH89A__&Key-Pair-Id=APKAJMSU6JYPN6FG5PBQ
2…
arrow_forward
Write an HTML code that will display the following information (6 marks)
List of Students who were Admitted in 2023/2024Academic year
Student Number
Surname
Gender
Nationality
COURSE
BBIT-001-05
CHINEDU
M
NIGERIAN
BIT
BSCCS-001-06
ASHANTEE
F
GHANIAN
COMPUTER SCIENCE
BIT-001-07
POTTER
M
AMERICAN
BIT
BIS-001-08
JANICE
F
KENYAN
BIS
End of LISTING
arrow_forward
Due to Ramadan, your nearby community wants to launch an “Online Grocery Store” so that, people can purchase groceries from home and able to maintain social distance. This precaution will allow reducing the movement of people in Ramadan and minimize the spread of disease COVID-19. The XYZ store will offer various products. The user will be shown a list of products, which can be selected or deselected from the provided list. The user will need to get registered to buy Products. At the end of shopping, the customer can double-check all the Products added into his Cart and verify the total amount before payment. The Store Administrator will manage Products and assign orders to Delivery Boys.Draw an Object Model (Class Diagram) for the given scenario showing main objects, their attributes, functions and relationships.The tasks you have to do are:
Extract the main objects (entities) of above system.
Find the necessary attributes and functions that need to be associated with each object.…
arrow_forward
Create a PHP script to generate multiplication table. Use html tags. The user will enter the number of rows and columns in a text box. The user will then click the Generte button to generate a multiplication table. The user will then search for a value in a text box and highlight the value being searched.
arrow_forward
Please open this link and solve the
computer science assignment.
If there is any error in the link please tell
me in the comments.
https://drive.google.com/file/d/1RQ2OZk-
¿LSxpRyejKUMah1t2q15dbpVLCS/view?
usp=sharing
arrow_forward
Android Studio Question:
Please create the following design in xml.
Don't provide the java class i need the xml design with proper screenshot.
arrow_forward
php programming
Prepare such a B.php that works for any particular form method (works both with get and post method hint:request.)
arrow_forward
Golden Pulps: Devan Ryan manages the website Golden Pulps, where he shares tips on collecting and fun stories from the “golden age of comic books”—a period of time covering 1938 through the early 1950s. Devan wants to provide online versions of several classic comic books, which are now in the public domain.
He’s scanned the images from the golden age comic book, America’s Greatest Comics 001, published in March, 1941 by Fawcett Comics and featuring Captain Marvel. He’s written the code for the HTML file and wants you to help him develop a layout design that will be compatible with mobile and desktop devices.
Flex Layout Styles
Open the gp_layout.css file and go to the Flex Layout Styles section and insert a style rule to display the page body as a flexbox oriented as rows with wrapping.
As always, include the latest -webkit browser extension in all of your flex styles.
The page body content has two main elements. The section element with the ID #sheet contains the panels from the…
arrow_forward
DATA:
myBytes BYTE 10h,20h,30h,40hmyWords WORD 8Ah,3Bh,72h,44h,66hmyDoubles DWORD 1,2,3,4,5myPointer DWORD myDoubles
Questtion:
mov ax,[esi] ; AX = ?
arrow_forward
Use html to design
Exact same I need
arrow_forward
PS5: Webscraping
Suggested Solutions
Import BeautifulSoup, json, requesrts, and pandas.
In [ ]: from bs4 import BeautifulSoup
import pandas as pd
import requests
import re
import json
IMDB top 50 rated films.
The following URL, https://www.imdb.com/search/title/?groups=top_250&sort=user_rating, is a link to the top 50 rated films on IMDB. Create a pandas
DataFrame with three columns: Title, Year, and Rating, pulling the data from the webpage.
We can do this in steps. First, get the HTML code that generated the webpage.
In [ ]:
Using the "Inspect Element" tool in a browser, see that each film is displayed in a DIV with the class lister-item. Use BS to find all such elements
and store them in a list called films.
Then, create a list of the title of each film. Notice, by inspecting the HTML, that the title is contained inside of a tag (a link) that is itself inside of a
DIV with class lister-item-content . That is, for each film in the list films, find the div with the class…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
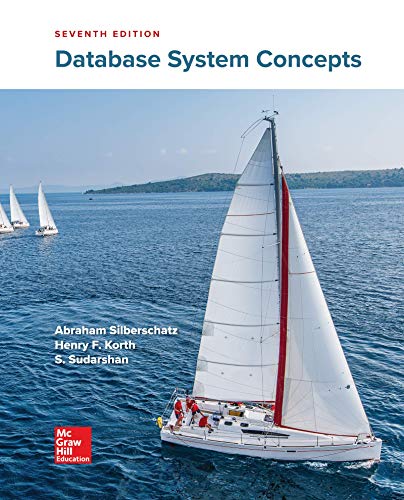
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
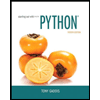
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
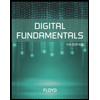
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
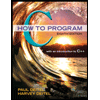
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
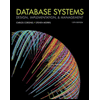
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
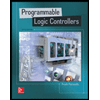
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Below are the files needed for the assignment. Thank you in advance. I've used my textbook, looked up guides, and reviewed my notes already. FILE #1 <!DOCTYPE html> <!-- Student Name: Jordan Collins File Name: index.hmtl Date: 10/13/2021 --> <html lang="en"> <head> <title>CH 4 Extend Your Knowledge</title> <meta charset="utf-8"> <link rel=“stylesheet” href=“css/styles.css”> </head> <body> <header> <h1>CSS Colors</h1> </header> <main> <div id="color"> <div id="orange"> <h2>Orange</h2> <div class="box orange-hex">Hex Orange</div> <div class="box orange-rgb">RGB Orange</div> <div class="box orange-hsl">HSL Orange</div> </div> <div…arrow_forwardHtml code for sure in this design java .arrow_forwardCreate a PHP page name it print.php - This page should be reachable via the following url: localhost/<std name>/<std id>/print where std name has at least one char (no numbers) and std id has the format id-20xyz; xyz are three numbers When a request send to print.php, then print student name and her/his id. localhost/tara/id-20222/printarrow_forward
- JavaScriptarrow_forwardEstem.org/courses/64525/assignments/9460783?module_item_id=18078917 The following information can help you get started: • Invitation Details: it boils down to when and where o When: Time and date • Where: Address • Invitee List: Name and email • Name: First Name, or First Name and Last Name Email: Email address . Other considerations: After you complete your invitation, answer the following questions: 1. What type of data are time, date, and place? How are they different from the other data types on the invite and guest list? F4 A Additional information worth including: dress code, directions, gifting, how to contact you. . How will you know who is showing up? RSVP? . Is there a theme to your invitation/design? x F5 % F6 F7 DELL F8 F9 ROMNA F10 F11 PrtScrarrow_forwardhttps://drive.google.com/file/d/1WvadErM-1ffp8gm2LcdqdMrtZ0gv3fJv/view?usp=sharing here in that link there is my code can please add comments to my code and describe me what is happeningarrow_forward
- JavaScript Dom Manipulation Hi can anyone please help me fix my main.js file. We're not allowed to change anything in index.html. We have to make a button so that every time you click on it the count on the web page increases by one. My code doesn't work and I don't know why. Every time I click the button, nothing happens. We just started learning about DOM and the only method we've learned so far is document.querySelector() Please help me understand dom events better. thank you so much! var numTimesClicked = 0; /* function handleClick(event) { numTimesClicked++; console.log('button clicked'); console.log('value of numTimesClicked:', numTimesClicked); } var $hot = document.querySelector('.hot-button'); var $click = document.querySelector('.click-count'); $click.addEventListener('click', handleClick); */ function handleClick(event) { numTimesClicked++; console.log('button clicked'); console.log('event:', event); console.log('event.target', event.target); } var…arrow_forwardJavaScript Dom Manipulation Hi can anyone please help me fix my main.js file. We're not allowed to change anything in index.html. We have to make a button so that every time you click on it the count on the web page increases by one. My code doesn't work and I don't know why. Every time I click the button, nothing happens. We just started learning about DOM and the only method we've learned so far is document.querySelector() Please help me understand dom events better. thank you so much! main.js var numTimesClicked = 0; function handleClick(event) { numTimesClicked++; console.log('button clicked'); console.log('event:', event); console.log('event.target', event.target); } var $click = document.querySelector('.click-count'); $click.addEventListener('click', handleClick); function handleMouseover(event) { console.log('button hovered'); console.log('event:', event); console.log('event.target', event.target); } index.html <!DOCTYPE html> <html…arrow_forwardPlease write php and html code just Thank youarrow_forward
- Setup Branch name assignment1 Directory assignment1 Package name assignment1 Instructions Create a node server with the following requirements: Use the dotenv package to manage your development environment variables. PORT should be 3000 HOST should be localhost Endpoints /dotted Only GET requests are allowed. This endpoint will respond with the HTML content type. This endpoint will take two required query parameters: word1 and word2. The endpoint will take the two words and create a string that is the two words separated by enough “.” characters to make the length of the string 30. For example if word1 is “turtle” and word2 is “153” the output should be: turtle.....................153 The response body should be the string wrapped in a <pre> tag. /fizzBuzz Only GET requests are allowed. This endpoint will respond with the HTML content type. This endpoint will take two required query parameters: start and end. The endpoint will iterate from start to end and for each…arrow_forwardA02-02 Create a webpage A2-Task2.html. The webpage is displaying one image. Each time the user moves the mouse out of the image, the image is changed to another one and one more 'sun' emoji is displayed under the image. When the number of 'suns' reaches seven (7), all suns are deleted (reset) and start to be displayed again. For example, the 8th time user moves the mouse out of the image, one 'sun' is shown, the 9th time - two 'suns', and so on. Note: - You should use two images. Each time the user moves the mouse out of the image, the image is changing. If an image of "Winter" is displayed it will change to "Summer", and opposite, if an image of "Summer" is displayed it will change to "Winter". The special character code for the 'sun' is "☀". - Put the source code of your website A2-Task2.html here. Make sure you also upload the A2-Task2.zip of all files below.arrow_forwardHTML table created by PHP CSS to set background color to "lightblue" PHP: create strings representing numbers in various bases Behavior: The page will look like this. The items are decimal and hexadecimal numbers from 1-20. The items are hexadecimal results of multiplication. Sample output: HW02 - Multiplication Table Matrix 3 4 5 5 5 af 6 1234567 8 9 10 11 12 13 14 15 16 17 18 19 20 Multiply 123456789abcd ef 10 11 12 13 14 1 1 2 3 4 5 6 7 8 9 a b c d e f 10 11 12 13 14 22468 ac e 10 12 14 16 18 la lc le 20 22 24 26 28 3 3 6 9 cf 12 15 18 lb le 21 24 27 2a 2d 30 33|36|39|3c 4 4 8 C 10 14 18 lc 20 24 28 2c 30 34 ||38 ||3c||40||44||48||4c_||50 1419 le 23 28 2d 32 37 3c|41|46|4b|50|55|5a|5f|64 66 c 12 18 le 24 2a 30 36 3c 42 48|4e||54|5a 60 ||66 ||6c 72 78 7 7 e 15 lc 23 2a 31 38 3f 46 4d 54 5b ||62|69|70|77|7e||85 ||8c 8 8 10 18 20 28 30 38 40 48 50 58 60 68 70 78 80 88 90 98 a0 12 lb 24 2d 36 3f 48 51 5a 63 6c 75 7e 87 90 99 a2 ab b4 14 le 28 32 3c 46 50 5a 64 6e 78 82 8c 96 a0 laa b4…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
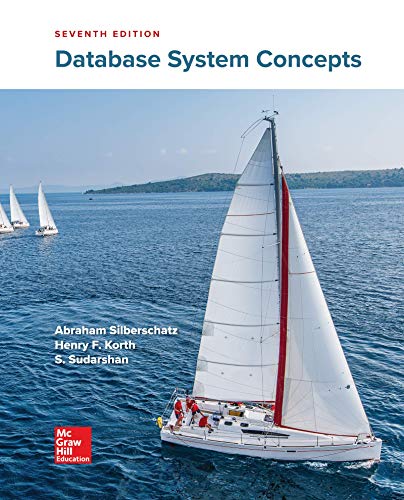
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
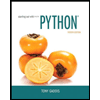
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
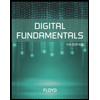
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
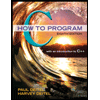
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
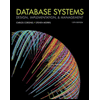
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
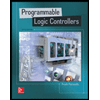
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education