Chapter 3 Assignments
.doc
keyboard_arrow_up
School
Houston Community College *
*We aren’t endorsed by this school
Course
6182-58114
Subject
Computer Science
Date
Feb 20, 2024
Type
doc
Pages
4
Uploaded by CorporalBookPheasant38
Chapter 3 Assignment
Debugging Exercises. 1. Try to debug each program without using Java.
2. Then, (a) copy each program to TextPad; (b) save each program as a Java file (in the “Save As” window, change the “Save as type” on the 3
rd
line from the bottom of the window from “All Files” to “Java”, using the dropdown arrow; (c) change the “File name” to the name of the program; the name of the first program is “DebugThree1”; (d) fix and run the program.
First Debug Program: (Be sure that your output is complete and makes sense)
// This class calculates a waitperson's tip as 15% of the bill
public class DebugThree1
{
public static void main(String args[])
{
double myCheck = 50.00;
double yourCheck = 19.95;
System.out.println("Tips are");
calcTip(mycheck);
calctip(yourCheck);
}
public static int calcTip(int bill)
//int
double
{
final double RATE = 0.15;
tip = bill + RATE;
System.out.println("The tip should be at least " + tip);
}
}
Second Debug Program:
// This application displays some math facts
public class DebugThree2
{
public static void main(String args[])
{
int a = 2, b = 5, c = 10;
add(a, b);
add(b, c);
subtract(c, a); }
public static void add(
int a, int b
)
{
System.out.println("The sum of " + a +
and + b + is + a + b);
}
public void subtract(
int a, int b
)
{
System.out.println("The difference between " +
1
a + and + b + is + a - b);
}
}
Third Debug Program:
// This program calculates tutition bills as credits times rate per credit hour
public class DebugThree3
{
public static void main(String args[])
{
int myCredits = 13
;
int yourCredits = 17
;
double rate = 75.84
;
System.out.println("My tuition:");
tuitionBill(myCredits, rate);
System.out.println("Your tuition:");
tuitionBill(myCredits, rate);
}
public static void tuitionBill(
int myCredits, float rate)
{
System.out.println("Total due " + (rate*credits));
}
}
Case 1:
1. Do the following:
a.
Create a class named Eggs. Its main() method has one integer variable named numberOfEggs. Prompt the user to enter the number of eggs and assign the value to numberOfEggs. (Remember that you will need to convert the String the user enters to an int before assigning it to numberOfEggs.) Save the application as Eggs.java.
b.
Create a method to which you pass numberOfEggs and which will then calculate and display the number of dozens. Name the method appropriately. In this method, calculate the number of eggs in dozens; for example, 50 eggs is 4 full dozen (with 2 eggs remaining). Display "The number of dozens is XX", where XX is the actual number of FULL
dozens of eggs.
c.
In your main method, call the method that you just created, and pass it numberOfEggs. d.
puExecute your application twice. The first time, enter 13 at the prompt. The second time, enter 44 at the prompt. 2
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Task: Loading data from files
This exercise will require you to load some information from files and use it in your program.
scene.txt contains a description of a series of shapes and colours to draw. You need to write code to read in the file data and draw the requested shapes in the correct colour. Each line in scene.txt will contain one of the following starting keywords followed by some data:
COLOUR followed by 3 values: R, G, B
CIRCLE followed by 3 values: X, Y, RADIUS
RECT Followed by 4 values: X, Y, W, H
LINE Followed by 4 values: X1, Y1, X2, Y2
CIRCLES are defined from the center. RECT's are defined from the top left. All values are space separated, and you can assume all input is correct (no errors). Please solve this program to draw the scene.
I can't upload the file scene.txt, so I decide to screenshot a file for you.
Subject: Java Programming
arrow_forward
Java
arrow_forward
Complete the following incomplete program (begin by copy-pasting that into a new program file). The completed program will provide a user interface to draw as many circles as the user wants only by clicking in the window. After getting the number of circles (n) to draw as input from the user through the provided interface, the program should iterate n times. In each iteration it should wait for the user to click twice and draw a circle with center at the point where the user clicked first and with radius calculated as its distance from the second point where the user clicked. It should then fill the circle with a random color.
Hints:
Distance between two points with coordinates x1 & y1 and x2 & y2 and can be calculated as sqrt((x1-x2)2 + (y1-y2)2 ).
To generate a random color, use randint(0,255) from the random libray to generate a random number between 0 and 255 (both inclusive). Use it three times to get the r, g and b values to generate a random color
from graphics import…
arrow_forward
1) Write a Java program to read a CSV (Comma separated values) text file. The text file will contain comma separated values in each line. There will be multiple lines with each line containing the same number of values. Ask the user to enter the path for the CSV file for reading the file.
After reading the data from the file output the data to the console (one line at a time) with the data in each line in properly aligned columns. Then write the data to a serialized file called "credit.ser" to the local project folder .
2) In the second program, read the serialized file and output the data in columns to the console (one line at a time) with the data in each line in properly aligned columns.
arrow_forward
Create a text file of names, (3 names per line, 5 lines in total) followed by an age for each name. Go through the file and sum the ages. Create an output file with each persons name followed by age and then a line at the end of the output file that says “sum of all ages is: x”. Simple Java programming. Should include things like bufferedReader, fileReader, printWriter, fileWriter, file close, try-with-resources, Scanner, catch/try, etc. Include Java file and txt file. Thank you.
arrow_forward
Q3: Write a C# program that reads two files (One.txt, Two.txt), where the first file contains
the names of teachers and the other contains their addresses. Then, put the information (name
and addresses) of the teachers to "Three.txt" file in the following form
">:>".
arrow_forward
Write a program that writes 100 integers created randomly into a file. Integers are separatedby a space in the file. Read the data back from the file and display the sorted data. Yourprogram should prompt the user to enter a file- name. If the file already exists, do not overrideit.
arrow_forward
1. Write a program that opens an output file with the filename my_name.txt, writes your name to the file, then closes the file.
2. Write a program that opens the my_name.txt file that was created by the program in problem 1, reads your name from the file, displays the name on the screen, then closes the file. Write code that does the following: opens an output file with the filename number_list.
arrow_forward
Can you help me write the following C++ program:
Add a search command to the file viewer created. Thiscommand asks the user for a string and finds the first line that containsthat string. The search starts at the first line that is currently displayedand stops at the end of the file. If the string is found, then the file willbe scrolled down so that when it is redisplayed, the line that contains thestring is the first line at the top of the window.If the string is not found, the program prints the following error messageat the top of the screen:ERROR: string X was not foundwhere X is replaced by the string entered by the user. In that case, theprogram redisplays the lines that the user was viewing before the searchcommand was executed.Modify the program as little as possible. Make sure you assign new responsibilities to the most appropriate components. Use the C++ string operation find.
The file viewer code is given below:
// FileViewer.cpp
#include "FileViewer.h"
using namespace…
arrow_forward
3. Write a program that asks the user for the name of a file. The program should display the contents of the file with each line preceded with a line number followed by a colon. The line numbering should start at 1. Use the numbers.txt file to test your program.
(Hint: Open the file and read every string stored in it. Use a variable to keep a count of the number of lines that are read from the file.)
Comment your source code and describe your code to someone who may be viewing it for the first time.
PLACE SCREEN SHOTS OF THE PYTHON CODE AND ALL I/O BELOW.
Please use Python Programming
arrow_forward
Computer Science
Using Java language
Write a procedure that generates a regular heightfield in data-files/model/heightfield.off with elevation given by the grayscale values of an image file. Use a file dialog to select the file, a number box to scale the xx and zz integer coordinates by, and a number box to set the scale that 1.0 corresponds to for height. Make a button labelled “Generate” that launches the generator. Display a message on screen while generating and then flush the G3D::ArticulatedModel cache, and reload the current scene on completion.
arrow_forward
Java Program /The Output attached by image
OverviewYou should enter this task, ask the user for a word and then search for a file to see if that word is among the words in the file.
Task
This exercise comes with three text files named wordlist1.txt, wordlist2.txt and wordlist3.txt. These files contain a large amount of words in English. Each file contains more and more words. Each word in these files is on a separate line and the words contain only lowercase letters. Your task is to write a program that reads all these words from the file and checks if a keyword, which the user entered, is among the entered words. The program should also count the total number of words contained in the file used. The program should measure how long it takes to complete the search, ie how long it takes to read all the words and check if the keyword is among the words or not.
The program should start by asking the user for the word to be searched for. Then the words in the file should be counted and…
arrow_forward
Java Program /The Output attached by image
OverviewYou should enter this task, ask the user for a word and then search for a file to see if that word is among the words in the file.
Task
This exercise comes with three text files named wordlist1.txt, wordlist2.txt and wordlist3.txt. These files contain a large amount of words in English. Each file contains more and more words. Each word in these files is on a separate line and the words contain only lowercase letters. Your task is to write a program that reads all these words from the file and checks if a keyword, which the user entered, is among the entered words. The program should also count the total number of words contained in the file used. The program should measure how long it takes to complete the search, ie how long it takes to read all the words and check if the keyword is among the words or not.
The program should start by asking the user for the word to be searched for. Then the words in the file should be counted and…
arrow_forward
Java Program /The Output attached by image
OverviewYou should enter this task, ask the user for a word and then search for a file to see if that word is among the words in the file.
Task
This exercise comes with three text files named wordlist1.txt, wordlist2.txt and wordlist3.txt. These files contain a large amount of words in English. Each file contains more and more words. Each word in these files is on a separate line and the words contain only lowercase letters. Your task is to write a program that reads all these words from the file and checks if a keyword, which the user entered, is among the entered words. The program should also count the total number of words contained in the file used. The program should measure how long it takes to complete the search, ie how long it takes to read all the words and check if the keyword is among the words or not.
The program should start by asking the user for the word to be searched for. Then the words in the file should be counted and…
arrow_forward
A Comma Separated Value (CSV) text file called movies.txt is located in the directory of your Eclipse Project.( If your Eclipse Project name is Assignment2, the text file is located under Assignment2, NOT src folder)
It contains data for one movie on each line.Each Movie contains the following data items:Title(String), Year(int-4 digits), Runtime(double).
Write Java program(s) to do the following:1) Read the movies.txt Text file and write the data as a Serialized file called movies.ser to the local directory(same as movies.txt).2) Read the movies.ser serialized file and output the data on to the Console in the format shown below.
The spacing for the output is: Title(20), Year (8) and Runtime (10.2)Title and Year are left-justified, Runtime is right-justified.(You can use your own text file to test your program)
Sample output:
Title Year Runtime aaaaaaaaaaa aaaa aa.aa...... ...... ...........…
arrow_forward
9.Code please.
In this exercise you will create a program that will be used in a digital library to format and sort journal entries based on the authors last name. Each entry has room to store only the last name of the author. Begin by removing the first name "Isaac" from the string variable journal_entry_1 by using the string function erase. Do not forget to also remove the whitespace so that the string variable journal_entry_1 will then contain the string "Newton" with no whitespaces. The journal entries should be sorted alphabetically based on the authors last name. For example, the last name "Brown" should come before the last name "Davis" Create an if statement so that if the last name contained within journal_entry_2 is alphabetically less than the last name contained within journal_entry_1, then the string values are swapped using the string function swap. You may use either of the comparison operators < > in the if statement but remember that following ASCII, "A" is…
arrow_forward
AaBbCcDd AaBbCc
I U abe x,
I Normal
TNo Spac
Font
Paragraph
... 2
3
5 6
Write a program that asks the user for the names of two files. The
first file should be opened for reading and the second file should
be opened for writing. the program should read the contents of
the first file, change all characters to lowercase, and store the
results in the second file. The second file will be a copy of the first
file, except that all the characters will be lowercase. Use Notepad
or another text editor to create a simple file that can be used to
test the program
Required Submission:
1. Java Program
2. Text file created using Notepad
3. Exception Handling (Especially when reading File not found,
End of the file, etc)
2. Sample Output (taken using Screenshot) on a Word document
arrow_forward
Java language
arrow_forward
Task 3: Summary
We would like to be able to generate a summary for each logfile to give us a rough idea of its contents.
Instructions
Create a file called Summary.java.
As with Task 1, open the file for reading in a try-catch block.
Read through the entire file, counting how many of each message/event type occurs.
Print out a summary with the count of each event/message type.
As a sanity check: the total number of message/event type counts should equal 1000.
Hint: a useful method could be String.split.
Sample Input/Output
Example 1:
Summary -------------------- Notifications: 322 Warnings: 326 Errors: 352
Cannot find logfile! Exiting...
arrow_forward
In Java, the File class is used to represent the data in a directory entry. Write a program that uses the File class to print all the attributes of a file.
arrow_forward
C++ Question:
See Attached picture. Follow all instructions. Please create the code yourself. Thanks!!!
arrow_forward
How do I do this? JAVA. Comment out each step please!!!
arrow_forward
task 18 in java
please include comments on the code so i can understand it better.
arrow_forward
Given a text file containing the availability of food items, write a program that reads the information from the text file and outputs the available food items. The program should first read the name of the text file from the user. The program then should read the text file, line by line. If a food is available, the program should output the available food item in the following format: name (category) -- description
Assume the text file contains the category, name, description, and availability of at least one food item, separated by a tab character ('\t').
Hints: Use the find() function to find the index of a tab character in each row of the text file. Use the substr() function to extract the text separated by the tab characters.
Ex: If the input of the program is:
food.txt
and the contents of food.txt are:
Sandwiches Ham sandwich Classic ham sandwich Available Sandwiches Chicken salad sandwich Chicken salad sandwich Not available Sandwiches Cheeseburger Classic cheeseburger Not…
arrow_forward
Python - Next Birthdate
In this task, we will write a program that reads birthdate data from a given CSV file, and given the current date, determines which person's birthday will be celebrated next.
Create a function with the following signature:
nextBirthdate(filename, date)
filename: parameter, which represents the CSV filename.
date: parameter, which represents the current date.
The function should open the CSV file, read the birthdate data, and determine which person's birthday will be celebrated next, given the current date. The name of the person should be returned. In other words, given a date, find the person whose birthday is next.
Sample Run
birthdates.csv
Draven Brock, 01/21/1952
Easton Mclean, 09/02/1954
Destiny Pacheco, 10/10/1958
Ariella Wood, 12/20/1961
Keely Sanders, 08/03/1985
Bryan Sloan,04/06/1986
Shannon Brewer, 05/11/1986
Julianne Farrell,01/29/2000
Makhi Weeks, 03/20/2000
Lucian Fields, 08/02/2018
Function Call
nextBirthdate("birthdates.csv", "01/01/2022")
Output…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
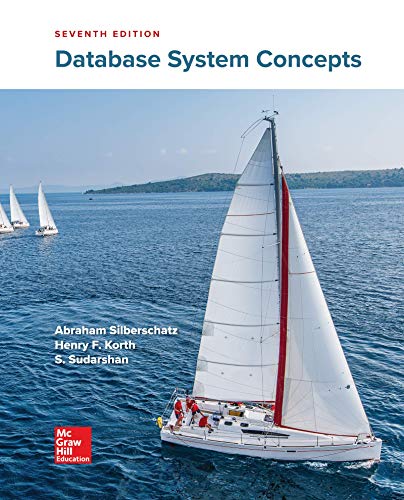
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
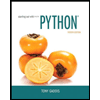
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
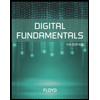
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
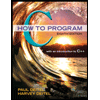
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
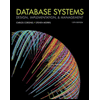
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
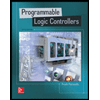
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Task: Loading data from files This exercise will require you to load some information from files and use it in your program. scene.txt contains a description of a series of shapes and colours to draw. You need to write code to read in the file data and draw the requested shapes in the correct colour. Each line in scene.txt will contain one of the following starting keywords followed by some data: COLOUR followed by 3 values: R, G, B CIRCLE followed by 3 values: X, Y, RADIUS RECT Followed by 4 values: X, Y, W, H LINE Followed by 4 values: X1, Y1, X2, Y2 CIRCLES are defined from the center. RECT's are defined from the top left. All values are space separated, and you can assume all input is correct (no errors). Please solve this program to draw the scene. I can't upload the file scene.txt, so I decide to screenshot a file for you. Subject: Java Programmingarrow_forwardJavaarrow_forwardComplete the following incomplete program (begin by copy-pasting that into a new program file). The completed program will provide a user interface to draw as many circles as the user wants only by clicking in the window. After getting the number of circles (n) to draw as input from the user through the provided interface, the program should iterate n times. In each iteration it should wait for the user to click twice and draw a circle with center at the point where the user clicked first and with radius calculated as its distance from the second point where the user clicked. It should then fill the circle with a random color. Hints: Distance between two points with coordinates x1 & y1 and x2 & y2 and can be calculated as sqrt((x1-x2)2 + (y1-y2)2 ). To generate a random color, use randint(0,255) from the random libray to generate a random number between 0 and 255 (both inclusive). Use it three times to get the r, g and b values to generate a random color from graphics import…arrow_forward
- 1) Write a Java program to read a CSV (Comma separated values) text file. The text file will contain comma separated values in each line. There will be multiple lines with each line containing the same number of values. Ask the user to enter the path for the CSV file for reading the file. After reading the data from the file output the data to the console (one line at a time) with the data in each line in properly aligned columns. Then write the data to a serialized file called "credit.ser" to the local project folder . 2) In the second program, read the serialized file and output the data in columns to the console (one line at a time) with the data in each line in properly aligned columns.arrow_forwardCreate a text file of names, (3 names per line, 5 lines in total) followed by an age for each name. Go through the file and sum the ages. Create an output file with each persons name followed by age and then a line at the end of the output file that says “sum of all ages is: x”. Simple Java programming. Should include things like bufferedReader, fileReader, printWriter, fileWriter, file close, try-with-resources, Scanner, catch/try, etc. Include Java file and txt file. Thank you.arrow_forwardQ3: Write a C# program that reads two files (One.txt, Two.txt), where the first file contains the names of teachers and the other contains their addresses. Then, put the information (name and addresses) of the teachers to "Three.txt" file in the following form ">:>".arrow_forward
- Write a program that writes 100 integers created randomly into a file. Integers are separatedby a space in the file. Read the data back from the file and display the sorted data. Yourprogram should prompt the user to enter a file- name. If the file already exists, do not overrideit.arrow_forward1. Write a program that opens an output file with the filename my_name.txt, writes your name to the file, then closes the file. 2. Write a program that opens the my_name.txt file that was created by the program in problem 1, reads your name from the file, displays the name on the screen, then closes the file. Write code that does the following: opens an output file with the filename number_list.arrow_forwardCan you help me write the following C++ program: Add a search command to the file viewer created. Thiscommand asks the user for a string and finds the first line that containsthat string. The search starts at the first line that is currently displayedand stops at the end of the file. If the string is found, then the file willbe scrolled down so that when it is redisplayed, the line that contains thestring is the first line at the top of the window.If the string is not found, the program prints the following error messageat the top of the screen:ERROR: string X was not foundwhere X is replaced by the string entered by the user. In that case, theprogram redisplays the lines that the user was viewing before the searchcommand was executed.Modify the program as little as possible. Make sure you assign new responsibilities to the most appropriate components. Use the C++ string operation find. The file viewer code is given below: // FileViewer.cpp #include "FileViewer.h" using namespace…arrow_forward
- 3. Write a program that asks the user for the name of a file. The program should display the contents of the file with each line preceded with a line number followed by a colon. The line numbering should start at 1. Use the numbers.txt file to test your program. (Hint: Open the file and read every string stored in it. Use a variable to keep a count of the number of lines that are read from the file.) Comment your source code and describe your code to someone who may be viewing it for the first time. PLACE SCREEN SHOTS OF THE PYTHON CODE AND ALL I/O BELOW. Please use Python Programmingarrow_forwardComputer Science Using Java language Write a procedure that generates a regular heightfield in data-files/model/heightfield.off with elevation given by the grayscale values of an image file. Use a file dialog to select the file, a number box to scale the xx and zz integer coordinates by, and a number box to set the scale that 1.0 corresponds to for height. Make a button labelled “Generate” that launches the generator. Display a message on screen while generating and then flush the G3D::ArticulatedModel cache, and reload the current scene on completion.arrow_forwardJava Program /The Output attached by image OverviewYou should enter this task, ask the user for a word and then search for a file to see if that word is among the words in the file. Task This exercise comes with three text files named wordlist1.txt, wordlist2.txt and wordlist3.txt. These files contain a large amount of words in English. Each file contains more and more words. Each word in these files is on a separate line and the words contain only lowercase letters. Your task is to write a program that reads all these words from the file and checks if a keyword, which the user entered, is among the entered words. The program should also count the total number of words contained in the file used. The program should measure how long it takes to complete the search, ie how long it takes to read all the words and check if the keyword is among the words or not. The program should start by asking the user for the word to be searched for. Then the words in the file should be counted and…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
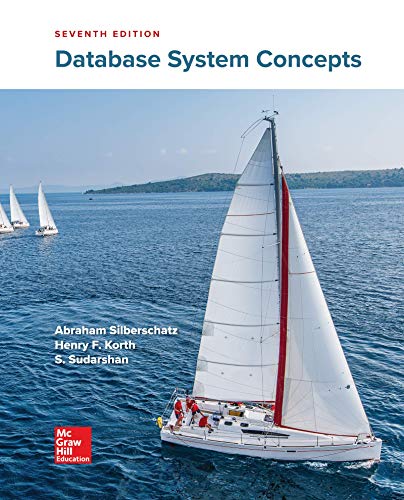
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
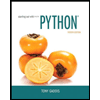
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
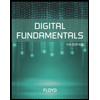
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
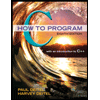
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
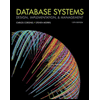
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
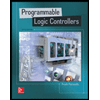
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education