_03 _ 2D Lists - Problem Set (1)
.pdf
keyboard_arrow_up
School
The University of Queensland *
*We aren’t endorsed by this school
Course
COMP4702
Subject
Computer Science
Date
Oct 30, 2023
Type
Pages
2
Uploaded by CountValor1816
2D Lists - Problem set
Complete the following programs. Comment your code as necessary. Any prep work for
the problems should be kept in your notebook. Be prepared to demo your solution
during an upcoming class. Your code will not only be evaluated as producing a correct
answer, it must also do so in an efficient, readable and well designed manner.
1. Create a function that accepts a 2D list tic tac toe board in the form:
game_board =
[ [ 0,1,2 ] ,
[ 0,1,2 ] ,
[ 0,0,0 ] ]
and returns who the winner is:
winner(game_board) # returns 1
Your Code:
2. Write a program to perform each task (in separate loops):
a.
Ask user to enter an integer
n
, then create a list of size
n
with all elements of the list set to 1
b.
Ask user to enter
n
integers (some positive and some negative), and place the
integers in the list starting with the first at the beginning of the list
c.
Switch the values at either end of the list
d.
Change any negative values to positive values (of the same magnitude)
e.
Set the variable sample_sum to the sum of the values of all the elements
f.
Print all the even numbers in the list
Your Code:
3. Write a function that accepts a list and returns a copy of the list in reverse order. Do
NOT
use the
name.reverse()
method.
Your Code:
4. Here is some data for a ICS2 class:
marks = [ [None, "Clyde", "Pinky", "Blinky", "Inky"],
["OOP", 45, 75, 90, 80],
["Sorts", 57, 87, 90, 88],
["Project", 70, 88, 94, 84]]
a) Write a function (called
function_a
) that finds a student by name and returns
the index of the column that they are in.
b) Write a function that takes a column ID and returns the name of the student.
c)
Write a function that takes the column ID of a student and calculates the average
for that student.
d) Write a function that returns the name of student with the highest average
g.
List the student(s) whose average is higher than the class average
Your Code:
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Exercise, maxCylinderVolume
F# system function such as min or methods in the list module such as List.map are not allowed
Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0.
Examples:
> maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304
arrow_forward
Question >
Not complete
Marked out of
1.50
Flag question
Previous page
Write a recursive function named get_palindromes (words) that takes a list of words as a parameter. The function should return a list of all the palindromes in the list. The function returns an
empty list if there are no palindromes in the list or if the list is empty. For example, if the list is ["racecar", "hello", "noon", "goodbye"], the function should return ["racecar", "noon"].
A palindrome is a word that is spelled the same forwards and backwards.
Note: The get_palindromes() function has to be recursive; you are not allowed to use loops to solve this problem.
For example:
Test
words = ["racecar", "hello", "noon", "goodbye", "test", 'aibohphobia'] ['racecar', 'noon', 'aibohphobia']
print (get_palindromes (words))
print (get_palindromes ([]))
print (get_palindromes (['this', 'is', 'test']))
Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %)
Result
Precheck Check
[]
[]
Next page
arrow_forward
Programming language is latest version of python
arrow_forward
Computer Science
Create a class that will store a list of names. Your class needs to include a function that will return
the name that appears first aphabetically, and another function that should return the name that
appears last alphabetically. You also need to include a function that will sort the list alphabetically. The class does not need to be case insensitive.
arrow_forward
Count dominators
def count_dominators(items):
An element of items is said to be a dominator if every element to its right (not just the one element that is immediately to its right) is strictly smaller than it. By this definition, the last item of the list is automatically a dominator. This function should count how many elements in items are dominators, and return that count. For example, dominators of [42, 7, 12, 9, 13, 5] would be the elements 42, 13 and 5.
Before starting to write code for this function, you should consult the parable of "Shlemiel the painter" and think how this seemingly silly tale from a simpler time relates to today's computational problems performed on lists, strings and other sequences. This problem will be the first of many that you will encounter during and after this course to illustrate the important principle of using only one loop to achieve in a tiny fraction of time the same end result that Shlemiel achieves with two nested loops. Your workload…
arrow_forward
PYTHON
Problem Statement
In Pascal's triangle, each number is the sum of the two numbers directly above it (see image). Complete a function called "pascal_next_row" which has one list parameter, previous_row. This function should use the function's input (the previous row of Pascal's triangle) to create a new list that is the next row in the triangle. The function should then return this new list (i.e., the next row of Pascal's triangle).
Below your function definition, ask the user for a non-negative integer, numrows, which represents the number of rows of Pascal's triangle to create/print. Then call your function multiple times to create a list of lists that represent Pascal's triangle with numrows rows. After your list of lists is created, print each row in the final list.
Sample Input 1
2
Sample Output 1
[1] [1, 1]
Sample Input 2
5
Sample Output 2
[1] [1, 1] [1, 2, 1] [1, 3, 3, 1] [1, 4, 6, 4, 1]
Starting Code
def pascal_next_row(previous_row):# this function…
arrow_forward
Yahtzee!
Yahtzee is a dice game that uses five die. There are multiple scoring abilities with the highest being a Yahtzee where all five die are the same. You will simulate rolling five die 777 times while looking for a yahtzee.
Program Specifications :
Create a list that holds the values of your five die.
Populate the list with five random numbers between 1 & 6, the values on a die.
Create a function to see if all five values in the list are the same and IF they are, print the phrase "You rolled ##### and its a Yahtzee!" (note: ##### will be replaced with the values in the list)
Create a loop that completes the process 777 times, simulating you rolling the 5 die 777 times, checking for Yahtzee, and printing the statement above when a Yahtzee is rolled.
When you complete the project please upload your .py file to the Project 2 folder by the due date.
arrow_forward
By python
arrow_forward
Exercise Objectives
Problem Description
Write a program that reads a string and mirrors it around the middle character.
Examples:
abcd becomes cdab.
abcde becomes deCab
AhmadAlami becomes AlamiAhmad
Page 1 of 2
Your program must:
• Implement function void reflect (char* str) which receives a string (array of characters) and
mirrors it. This function does not print anything.
•
Read from the user (in main()) a string and then print the string after calling function reflect().
•
Use pointers and pointer arithmetic only. The use of array notation and/or functions from the string.h
library is not allowed.
arrow_forward
17. The following function takes a parameter that is a list of integers; the list has at least one element. It is supposed to
find the integer that is the largest and find the position of that integer in the list. The function has two incorrect lines
of code that make the function compute the results incorrectly. Several lines are highlighted (bold, underlined) which
COULD be incorrect. In the following questions, choose the correct line of code that should be in the function.
1
def findlarge(intlist):
2
largest = 0
3
pos = 0
for i in range(1,len(intlist)):
if largest > intlist[i:
4
5
largest = intlist[i]
pos = i
return (largest, pos)
8
Select one of the following to correct a line of code in the function.
a. Line 2 should be: largest = -1
b. Line 2 should be: largest = intlist[0]
c. Line 4 should be: for i in range(0,len(intlist):
%3D
d. Line 4 should be: for i in range(1,len(intlist)-1):
e. Line 8 should be: return largest
18. Select one of the following to correct a line of code in…
arrow_forward
language = python
arrow_forward
C++ Programming Problem:
Newton is a brilliant mathematician and solving triangles problems fascinates him. Today he is given
a sequence of positive integers V1, V2, ., VN. You must choose three elements Vx, Vy, Vz (x, y, z is
vertices) such that the triangle formed have these properties: |XY|= Vz, |XZ|=Vy, |YZ|=Vx and the
angle |ZYXZ|= 0 satisfies cose2P/Q, where 0 should be maximum possible. Find any such triangle or
determine that it does not exist. Develop a C++ code which prints the value of x, y, z in a single line
and print "Invalid" if not possible.
Test Case:
412
9765
Result:
324
arrow_forward
OCaml Code: Please read the attached instructions carefully and show the correct code with the screenshot of the output. I really need help with this assignment.
arrow_forward
8-9. Messages: Make a list containing a series of short text messages. Pass the list to a function called show_messages(), which prints each text message.
8-10. Sending Messages: Start with a copy of your program from 8-9. Write a function called send_messages() that prints each text message and moves each message to a new list called sent_messages as it’s printed. After calling the function, print both of your lists to make sure the messages were moved correctly.
8-11. Archived Messages: Start with your work from 8-10. Call the function send_messages() with a copy of the list of messages. After calling the function, print both of your lists to show that the original list has retained its messages.
8-12. Sandwiches: Write a function that accepts several items a person wants on a sandwich. The function should have one parameter that collects as many items as the function call provides, and it should print a summary of the sandwich that’s being ordered. Call the function three times,…
arrow_forward
python program plz
arrow_forward
Create a soda beverage shopping cart program using C++ implementing the following:
Linked List - this will be used as the user's cart. This is where the items that the user will purchased are stored here before checking them out.
Array - use arrays to store user's stored products.
Pointers
Functions - Make sure that the rogram will have user-defined functions (e.g.: function for adding nodes, function for displaying the cart, etc.)
The program must have a menu where the user can select an action (e.g. Select product, View cart, Check out, etc.)
Soda options: Coca-Cola, Pepsi, Sprite, Royal, Mountain Dew
Tabular Format: In the table, you must include the code for each soda options, their price and quantity
The program must look like this:
Hi! Welcome to ABC GoDrink! Here are the drinks that we offer:
CODE
PRODUCT
SRP
a
Coca-Cola
24.99
b
Pepsi
22.99
c
Royal
24.99
d
Sprite
24.99
e
Mountain Dew
22.99
Menu
Select a product.
View My Cart and Proceed to Check-Out…
arrow_forward
Q1: Assignment Difficulty
Dan has a list of problems suitable for Assignment 4. The difficulties of these problems are stored in a list of integers . The problem’s difficulty is represented by (the higher the integer, the more difficult the problem).ai-tha[i]
Dan is too busy eating saltines to worry about Assignment 4 decisions, so he asks Michael the TA to select at least two problems from the list for the assignment. Since there are many possible subsets of the problems to consider and Michael has a life, he decides to consider only sublists (definition follows) of the list of problems.
To make grading the assignment easier, Michael wants to pick problems that don’t vary too much in difficulty. What is the smallest difference between the difficulties of the most difficult selected problem and the least difficult selected problem he can achieve by selecting a sublist of length at least 2 of the original list of problems?
Definition: A sublist of a list is any list you can obtain by…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
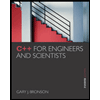
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Related Questions
- Exercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forwardQuestion > Not complete Marked out of 1.50 Flag question Previous page Write a recursive function named get_palindromes (words) that takes a list of words as a parameter. The function should return a list of all the palindromes in the list. The function returns an empty list if there are no palindromes in the list or if the list is empty. For example, if the list is ["racecar", "hello", "noon", "goodbye"], the function should return ["racecar", "noon"]. A palindrome is a word that is spelled the same forwards and backwards. Note: The get_palindromes() function has to be recursive; you are not allowed to use loops to solve this problem. For example: Test words = ["racecar", "hello", "noon", "goodbye", "test", 'aibohphobia'] ['racecar', 'noon', 'aibohphobia'] print (get_palindromes (words)) print (get_palindromes ([])) print (get_palindromes (['this', 'is', 'test'])) Answer: (penalty regime: 0, 0, 5, 10, 15, 20, 25, 30, 35, 40, 45, 50 %) Result Precheck Check [] [] Next pagearrow_forwardProgramming language is latest version of pythonarrow_forward
- Computer Science Create a class that will store a list of names. Your class needs to include a function that will return the name that appears first aphabetically, and another function that should return the name that appears last alphabetically. You also need to include a function that will sort the list alphabetically. The class does not need to be case insensitive.arrow_forwardCount dominators def count_dominators(items): An element of items is said to be a dominator if every element to its right (not just the one element that is immediately to its right) is strictly smaller than it. By this definition, the last item of the list is automatically a dominator. This function should count how many elements in items are dominators, and return that count. For example, dominators of [42, 7, 12, 9, 13, 5] would be the elements 42, 13 and 5. Before starting to write code for this function, you should consult the parable of "Shlemiel the painter" and think how this seemingly silly tale from a simpler time relates to today's computational problems performed on lists, strings and other sequences. This problem will be the first of many that you will encounter during and after this course to illustrate the important principle of using only one loop to achieve in a tiny fraction of time the same end result that Shlemiel achieves with two nested loops. Your workload…arrow_forwardPYTHON Problem Statement In Pascal's triangle, each number is the sum of the two numbers directly above it (see image). Complete a function called "pascal_next_row" which has one list parameter, previous_row. This function should use the function's input (the previous row of Pascal's triangle) to create a new list that is the next row in the triangle. The function should then return this new list (i.e., the next row of Pascal's triangle). Below your function definition, ask the user for a non-negative integer, numrows, which represents the number of rows of Pascal's triangle to create/print. Then call your function multiple times to create a list of lists that represent Pascal's triangle with numrows rows. After your list of lists is created, print each row in the final list. Sample Input 1 2 Sample Output 1 [1] [1, 1] Sample Input 2 5 Sample Output 2 [1] [1, 1] [1, 2, 1] [1, 3, 3, 1] [1, 4, 6, 4, 1] Starting Code def pascal_next_row(previous_row):# this function…arrow_forward
- Yahtzee! Yahtzee is a dice game that uses five die. There are multiple scoring abilities with the highest being a Yahtzee where all five die are the same. You will simulate rolling five die 777 times while looking for a yahtzee. Program Specifications : Create a list that holds the values of your five die. Populate the list with five random numbers between 1 & 6, the values on a die. Create a function to see if all five values in the list are the same and IF they are, print the phrase "You rolled ##### and its a Yahtzee!" (note: ##### will be replaced with the values in the list) Create a loop that completes the process 777 times, simulating you rolling the 5 die 777 times, checking for Yahtzee, and printing the statement above when a Yahtzee is rolled. When you complete the project please upload your .py file to the Project 2 folder by the due date.arrow_forwardBy pythonarrow_forwardExercise Objectives Problem Description Write a program that reads a string and mirrors it around the middle character. Examples: abcd becomes cdab. abcde becomes deCab AhmadAlami becomes AlamiAhmad Page 1 of 2 Your program must: • Implement function void reflect (char* str) which receives a string (array of characters) and mirrors it. This function does not print anything. • Read from the user (in main()) a string and then print the string after calling function reflect(). • Use pointers and pointer arithmetic only. The use of array notation and/or functions from the string.h library is not allowed.arrow_forward
- 17. The following function takes a parameter that is a list of integers; the list has at least one element. It is supposed to find the integer that is the largest and find the position of that integer in the list. The function has two incorrect lines of code that make the function compute the results incorrectly. Several lines are highlighted (bold, underlined) which COULD be incorrect. In the following questions, choose the correct line of code that should be in the function. 1 def findlarge(intlist): 2 largest = 0 3 pos = 0 for i in range(1,len(intlist)): if largest > intlist[i: 4 5 largest = intlist[i] pos = i return (largest, pos) 8 Select one of the following to correct a line of code in the function. a. Line 2 should be: largest = -1 b. Line 2 should be: largest = intlist[0] c. Line 4 should be: for i in range(0,len(intlist): %3D d. Line 4 should be: for i in range(1,len(intlist)-1): e. Line 8 should be: return largest 18. Select one of the following to correct a line of code in…arrow_forwardlanguage = pythonarrow_forwardC++ Programming Problem: Newton is a brilliant mathematician and solving triangles problems fascinates him. Today he is given a sequence of positive integers V1, V2, ., VN. You must choose three elements Vx, Vy, Vz (x, y, z is vertices) such that the triangle formed have these properties: |XY|= Vz, |XZ|=Vy, |YZ|=Vx and the angle |ZYXZ|= 0 satisfies cose2P/Q, where 0 should be maximum possible. Find any such triangle or determine that it does not exist. Develop a C++ code which prints the value of x, y, z in a single line and print "Invalid" if not possible. Test Case: 412 9765 Result: 324arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
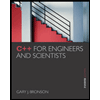
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Browse Popular Homework Q&A
Q: 3. Let X be a topological space and let f: X→ Sn a continuous map to the n-sphere.
Prove the…
Q: java method called switchThem that acc
Q: 24. A sinusoidal voltage is applied
to the resistive circuit in Figure
11-80. Determine the…
Q: 6.
HNO3/H2SO4
Nitration
Q: Problem 5.5: A kitchen oven has an interior volume V of 0.12 m³.
The air in the oven is preheated…
Q: Discuss the different regions of the brain and the behaviors/functions they may be responsible for.…
Q: Madison Company purchased a machine on February 1, 2018, for $200,000. On December 31,
2021, when…
Q: Find a topic relating to the Boundedness of Convergent Sequences for a final project
Q: A(-3,4)
-5
54 -3 -2 -1
a.) Domain:
b.) Range:
c.) f (-3) =
d.) f (0) =
e.) f (x) = 4 when x =
f.) f…
Q: Write a java program that allows a user to enter more than one number to be tested for a series of…
Q: Treating an immediate expense as a capital expenditure:
O overstates expenses and understates net…
Q: python help
Implement function four_letter() that takes as input a list of words (i.e., strings)…
Q: An electric turntable 0.790 m in diameter is rotating about a fixed axis with an
initial angular…
Q: 4. Show that Archimedean Principle is a consequence of the assertions that every
interval (a, b)…
Q: Write a program named Fibonacci that prints the Fibonacci sequence of numbers. The first two numbers…
Q: If prices are rising and a company is using LIFO, large purchases of inventory near the end of the…
Q: Electrolytes that release hydrogen ions in water are
Group of answer choices
A) bases.
B)…
Q: Overiew of IT Security Policy Framework
Q: Discuss the various biological steps that will take place in your body to extract the necessary…
Q: Using the provided budgeted information for production of 10,000 and 15,000 units,
prepare a…
Q: Acetaldehyde (CH3CHO) is an important chemical both industrially and biologically. For instance, it…
Q: For each of the structures listed below identify the class of lipids to which it belongs (fatty acid…
Q: What is the highest score?
Question 18 options:
9
96
156
9156
Q: n accounting for by-products, when the by-products are sold for more than the estimated sales value,…
Q: ure.
2 ΚΩ
4 ΚΩ
Μ
Use the differential equation approach to find io(t) for t> 0 in the network in
t =…