HW09
.py
keyboard_arrow_up
School
Georgia Institute Of Technology *
*We aren’t endorsed by this school
Course
1301
Subject
Computer Science
Date
Dec 6, 2023
Type
py
Pages
2
Uploaded by PresidentMoon12744
"""
Georgia Institute of Technology - CS1301
Homework 9 - Recursion
"""
#########################################
"""
Function Name: numCoffees()
Parameters: num (int)
Returns: None (NoneType)
"""
def numCoffees(num):
if num ==0:
print("No more coffee!")
else:
print(f"Coffees left: {num}")
numCoffees(num-1)
#########################################
"""
Function Name: coffeeShopDescrambler()
Parameters: scrambledName (str)
Returns: shopName (str)
"""
def coffeeShopDescrambler(scrambledName):
if scrambledName == "":
return ""
else:
shopName=coffeeShopDescrambler(scrambledName[:-1])
if scrambledName[-1].isalpha() or scrambledName[-1]==" ":
shopName+=scrambledName[-1].lower()
return shopName
#########################################
"""
Function Name: atlCoffee()
Parameters: listOfshops (list)
Returns: shopsToVisit (dict)
"""
def atlCoffee(listOfshops):
if listOfshops == []:
return {}
else:
shopsToVisit=atlCoffee(listOfshops[1:])
if listOfshops[0][2]>5:
name=listOfshops[0][0]
area=listOfshops[0][1]
if area in shopsToVisit:
shopsToVisit[area].append(name)
else:
shopsToVisit[area]=[name]
shopsToVisit[area].sort()
return shopsToVisit
#########################################
"""
Function Name: balancedOrder()
Parameters: order (str)
Returns: isBalanced (bool)
"""
def balancedOrder(order):
if len(order)<=1:
return True
else:
if order[0].isupper() == order[-1].isupper():
return balancedOrder(order[1:-1])
else:
return False
#########################################
"""
Function Name: cafeJosh()
Parameters: menuItems (list)
Returns: finalItem (str)
"""
def cafeJosh(menuItems):
if menuItems==[]:
return ""
else:
finalItems= cafeJosh(menuItems[:-1])
if type(menuItems[-1]) is list:
selfstr=cafeJosh(menuItems[-1])
finalItems+=selfstr
else:
finalItems+=menuItems[-1]
return finalItems
#########################################
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Define Constant Arrays as Formal Parameters.
arrow_forward
Write in C Language
Description
Give you a matrix. Please output the elements in clockwise spiral order.
Input
First line is two integers m and n, shows number of rows and number of columns.
Followed m lines are content of the matrix.
Output
Elements of the matrix in clockwise spiral order.
Sample Input 1
3 3
1 2 3
4 5 6
7 8 9
Sample Output 1
1 2 3 6 9 8 7 4 5
arrow_forward
What are the advantages and disadvantages of using ordinal data types that are user-defined? What are the advantages and disadvantages of employing an associative array?
arrow_forward
in c++ using 2D Arrays
The following diagram represents an island with dry land (represented by “-“) surrounded by water ((represented by “#“).
##-##########
#-----------#
#-----------#
#------------ #
-----------#
#------X----#
#-----------#
#############
Two bridges lead off the island. A mouse (represented by “X”) is placed on the indicated square. Write a program to make the mouse take a walk across the island. The mouse is allowed to travel one square at a time, either horizontally or vertically. A random number from 1 to 4 should be used to decide which direction the mouse is to take; for the sake of uniformity assume that 1 = up, 2 = down, 3 = left, and 4 = right. Since the mouse is wearing cement mouse galoshes, the mouse drowns when he hits the water. He escapes when he steps on a bridge. You may generate a random number up to 100 times allowing the mouse to take 100 steps. If the mouse does not find a bridge by the 100th try, he will wither away and die of starvation.…
arrow_forward
Using pointers. C language. Can i have typewritten answer? Thank you.
arrow_forward
Give an example of use of Pointers in Java class.
arrow_forward
From a Programming language Haskell,
what could be a answer for these type declaration?
type1 :: a -> b -> c
type2 :: (a -> b -> c) -> (a,b) -> c
type3 :: (a -> b) -> (b -> c) -> a -> c
type4 :: (a -> b -> c) -> a -> (d -> b) -> d -> c
type5 :: (a -> b -> c) -> (d -> a) -> (e -> b) -> d -> e -> c
type6 :: (a -> a -> [a]) -> b -> (b -> [a] -> c) -> a -> c
type7 :: (a -> [b]) -> (b -> c) -> a -> c -> [c]
type8 :: ((a -> b) -> c -> d) -> b -> c -> d
type9 :: ((a,b) -> c) -> (c -> d) -> [(a,b)] -> [d]
arrow_forward
java uses fixed stack dynamic arrays for arrays of primitive types
True
False
arrow_forward
Write in C language
Description
Give you a matrix. Please output the elements in clockwise spiral order.
Input
First line is two integers m and n, shows number of rows and number of columns.
Followed m lines are content of the matrix.
Output
Elements of the matrix in clockwise spiral order.
Sample Input 1
3 3
1 2 3
4 5 6
7 8 9
Sample Output 1
1 2 3 6 9 8 7 4 5
arrow_forward
How can one simply copy a collection of shared pointers into another array using the C++ programming language? Create a list of potential answers to the problems you've been presented with in this scenario. Is it true that when shared pointers are copied, the objects they control are likewise copied along with them? Explain
arrow_forward
What are the advantages and disadvantages of adopting user-defined ordinal kinds when it comes to data types? An associative array has several advantages and disadvantages, which we shall cover in detail below.
arrow_forward
Explain Direct and Indirect Recursion.
arrow_forward
Pandas is a Python library used for working with data sets. It has functions for analyzing, cleaning, exploring, and manipulating data. The name "Pandas" has a reference to both "Panel Data", and "Python Data Analysis" and was created by Wes McKinney in 2008.
Everything in life has both advantages and disadvantages. I discuss 1 advantage and 1 disadvantage of using pandas for Python. Ensure that you attempt to explain your selections.
arrow_forward
What are the advantages and disadvantages of user-defined ordinal types as data types? What are the advantages and disadvantages of an associative array?
arrow_forward
Linked List Question
Which of the following statements are NOT correct about the ADTs?
ADTs are only supported in Java programming language.
All implementations of the same ADT will have the same time complexity for all the operations of the ADT.
ADTs allow programmers to focus on important aspects of data without worrying about implementation details.
arrow_forward
With an example discuss the operators used with pointers.
arrow_forward
Write answer in C++ Language. The Topic is Terrain Navigation. Concept of 2D Array will apply. Give source code and give also logic which is applying on this question. Hints are also given.
arrow_forward
Write the advantages and disadvantages of recursion.
arrow_forward
Programming language C++ is used.What are the benefits and drawbacks of using an array?
arrow_forward
Programming language C++ is used.
What are the benefits and drawbacks of using an array?
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
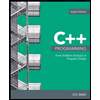
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Related Questions
- Define Constant Arrays as Formal Parameters.arrow_forwardWrite in C Language Description Give you a matrix. Please output the elements in clockwise spiral order. Input First line is two integers m and n, shows number of rows and number of columns. Followed m lines are content of the matrix. Output Elements of the matrix in clockwise spiral order. Sample Input 1 3 3 1 2 3 4 5 6 7 8 9 Sample Output 1 1 2 3 6 9 8 7 4 5arrow_forwardWhat are the advantages and disadvantages of using ordinal data types that are user-defined? What are the advantages and disadvantages of employing an associative array?arrow_forward
- in c++ using 2D Arrays The following diagram represents an island with dry land (represented by “-“) surrounded by water ((represented by “#“). ##-########## #-----------# #-----------# #------------ # -----------# #------X----# #-----------# ############# Two bridges lead off the island. A mouse (represented by “X”) is placed on the indicated square. Write a program to make the mouse take a walk across the island. The mouse is allowed to travel one square at a time, either horizontally or vertically. A random number from 1 to 4 should be used to decide which direction the mouse is to take; for the sake of uniformity assume that 1 = up, 2 = down, 3 = left, and 4 = right. Since the mouse is wearing cement mouse galoshes, the mouse drowns when he hits the water. He escapes when he steps on a bridge. You may generate a random number up to 100 times allowing the mouse to take 100 steps. If the mouse does not find a bridge by the 100th try, he will wither away and die of starvation.…arrow_forwardUsing pointers. C language. Can i have typewritten answer? Thank you.arrow_forwardGive an example of use of Pointers in Java class.arrow_forward
- From a Programming language Haskell, what could be a answer for these type declaration? type1 :: a -> b -> c type2 :: (a -> b -> c) -> (a,b) -> c type3 :: (a -> b) -> (b -> c) -> a -> c type4 :: (a -> b -> c) -> a -> (d -> b) -> d -> c type5 :: (a -> b -> c) -> (d -> a) -> (e -> b) -> d -> e -> c type6 :: (a -> a -> [a]) -> b -> (b -> [a] -> c) -> a -> c type7 :: (a -> [b]) -> (b -> c) -> a -> c -> [c] type8 :: ((a -> b) -> c -> d) -> b -> c -> d type9 :: ((a,b) -> c) -> (c -> d) -> [(a,b)] -> [d]arrow_forwardjava uses fixed stack dynamic arrays for arrays of primitive types True Falsearrow_forwardWrite in C language Description Give you a matrix. Please output the elements in clockwise spiral order. Input First line is two integers m and n, shows number of rows and number of columns. Followed m lines are content of the matrix. Output Elements of the matrix in clockwise spiral order. Sample Input 1 3 3 1 2 3 4 5 6 7 8 9 Sample Output 1 1 2 3 6 9 8 7 4 5arrow_forward
- How can one simply copy a collection of shared pointers into another array using the C++ programming language? Create a list of potential answers to the problems you've been presented with in this scenario. Is it true that when shared pointers are copied, the objects they control are likewise copied along with them? Explainarrow_forwardWhat are the advantages and disadvantages of adopting user-defined ordinal kinds when it comes to data types? An associative array has several advantages and disadvantages, which we shall cover in detail below.arrow_forwardExplain Direct and Indirect Recursion.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
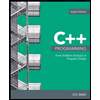
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning