Module 2 code reflection
.docx
keyboard_arrow_up
School
Southern New Hampshire University *
*We aren’t endorsed by this school
Course
300
Subject
Computer Science
Date
Dec 6, 2023
Type
docx
Pages
2
Uploaded by CountIron10886
This program takes data from municipal government feed containing bids on properties
up for auction. This program takes and sorts this data in ascending order. 1 sorting method is
selection sort and the other is a quicksort. The selection sort took more than 10 seconds to
complete the sorting process and the quicksort only took a fraction of a second to sort the same
data. I didn’t experience much of a challenge with completing the missing code. I did, however,
have a lot of trouble with compiling the program. I used a few YouTube tutorials and advice from
Stack Overflow to correct the issue.
The program starts with creating the variables that will hold the bid data. The system then
prepares to collect the bid data and assign appropriate variables for each. The system then loads
the information from the CSV file.
The data is then split into high and low points, and each is sorted and swapped until the
full list is sorted.
This process is completed using quick sort.
The selection sort is then called and uses a 1 by 1 approach. As the system goes through
each element it is compared to the lowest stored element. If the item is smaller then it replaces
the element if not it moves on until the entire list is compared.
The display then prompts user to select one of the following options to view the
information processed in the code written above.
1.
Load bids
2.
Display all bids
3.
Selection Sort bids
4.
Quicksort bids
9.
Exit
Each selection except for “9” collects the data and time it takes to retrieve and sort the
data is calculated and displayed to the user.
The program ends with a “Good bye” message to the user.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Assignment 5A: Multiple Frequencies. In the last assignment, we calculated the frequency of
a coin flip. This required us to have two separate variables, which we used to record the number
of heads and tails. Now that we know about arrays, we can track the frequency of all numbers in
a randomly generated sequence.
For this program, you will ask the user to provide a range of values (from 1 to that number,
inclusive) and how long of a number sequence you want to generate using that number range.
You will then generate and save the sequence in an array. After that, you will count the number
of times each number occurs in the sequence, and print the frequency of each number.
Hints: You can use multiple arrays for this assignment. One array should hold the
number sequence, and another could keep track of the frequencies of each number.
Sample Output #1:
What's the highest number you want to generate?: 5
How Long of a number sequence do you want to generate?: 10
Okay, we'll generate 10…
arrow_forward
In this lab, we will practice the use of arrays.
We will write a program that follows the steps below:
Input 6 numbers from the user
user inputs numbers one at a time
Loop continuously until the exit condition below:
ask the user to search for a query number
if the query number is in the list: reports the first location of the query number in the list, change the number in that position to 0, show the updated list
if the query number is not in the list: exit the loop
You must write at least one new function:
findAndReplace(array1,...,query) – take in an array and query number as input (in addition to any other needed inputs); it will return the first location of the query number in the list (or -1 if it is not in the list) and will change the number at that location to 0.
You may receive the number inputs and print all outputs in int main if you wish. You may report location based on 0-indexing or 1-indexing (but you need 0-indexing to access the array elements!).
arrow_forward
c#
Write a program , that allows the user to enter an array of elements (integers) then sort these elements in ascending.
Hint:
first define the array, then loop to enter the elements of the array, and finally use the usual sorting code.
arrow_forward
Using c++
Create this program in this given instructions.
Using a random number generator, create a list of 500 integers. Perform a benchmark analysis using some of the sorting algorithms from this chapter. What is the difference in execution speed?
Implement the bubble sort using simultaneous assignment.
A bubble sort can be modified to “bubble” in both directions. The first pass moves “up” the list, and the second pass moves “down.” This alternating pattern continues until no more passes are necessary. Implement this variation and describe under what circumstances it might be appropriate.
arrow_forward
Write a program , that allows the user to enter an array of elements (integers) then sort these elements in ascending.
Hint:
first define the array, then loop to enter the elements of the array, and finally use the usual sorting code.
arrow_forward
True or False.
A simple variable, also called a scalar variable, is one that is unrelated to any other variable in the computer’s internal memory.
The bubble sort algorithm gets its name from the fact that as the larger values drop to the bottom of the array, the smaller values rise, like bubbles, to the top.
Programmers use arrays to temporarily store related data in the internal memory of the computer.
Different variables in the same array may have different data types.
The elements in an array can be used just like any other variables.
When an array is sorted in ascending order, the first element contains the largest value and the last element contains the smallest value.
When passing an array by reference in C++, you do not include the address-of operator before the formal parameter’s name in the function header.
You distinguish one variable in a one-dimensional array from another variable in the same array using a unique integer,…
arrow_forward
You are given two different methods for sorting the values in an array: Sort1 and Sort2. You have determined that Sort1 = O(nlog n) and Sort2 = O(n2).
You need to choose one of these two sorting methods to sort lists in a program you are writing, but you would like to choose the one which completes the fastest.
Given this information, is either of these two methods guaranteed to be complete faster than the other? If so, explain which one is faster and why. If not, explain why not.
arrow_forward
IN C PROGRAMMING LANGUAGE AND COMMENT EVERY LINE PLEASE SO I CAN UNDERSTAND EVERY STEP , The selection sort is one of several techniques for sorting an array. A selection sort compares every element of an array with all the other elements of the array and exchanges their values if they are out of order. After the first pass of a selection sort, the first array element is in the correct position; after the second pass the first two elements of the array are in the correct position, and so on. Thus, after each pass of a selection sort, the unsorted portion of the array contains one less element. Write and test a function that implements this sorting method.
arrow_forward
a C++ program that will load 100 integers into array n. Separate the even from the odd valued elements. The procedure should place the even values in ascending order starting from the first location of the original array and place the odd values in ascending order starting from the last position of the original array.
Attached photo is the supposed result of the program created.
arrow_forward
Problem 1 – Adding contactsIn this problem, you will create the skeleton for your entire assignment. In the next problems, you will add more functionality to your program.Our goal on this problem is to create a Rolodex to store names and e-mails (using 1D or 2D arrays, you can choose). The Rolodex can store up to 256 contacts. The Rolodex will store the contact's name as one String and the contact's e-mail as another String.Your application will work as follows:1. Upon starting, it will greet the user and prompt the user to select an option (an integer)2. There are three options in problem 1:1 – to add contactnote: If the Rolodex is full, i.e. there are 256 contacts stored, this option should print: "Roldex is Full" and ask the user to input a new option5 – to print all contacts0 – to exitIf the user inputs an incorrect value, the program should prompt for an option againIf the users select option 1:1. The application will then ask for the contact's name and e-mail (see the example for…
arrow_forward
Computer science
arrow_forward
Programming
arrow_forward
Officer Jenny is in charge of training new police recruits. She just gave her students a multipe-choice quiz.
She wants to produce a summary of student performance for all questions where fewer than 60% of the
recruits got the question right. Write a program to accomplish this task.
Using Numpy Arrays
Although in principle this question could be solved using lists only. our goal is to gain practice working
with arrays. Therefore, you must convert the quiz data to an array and perform all calculations using array
operations. Before you start anything, make sure you have imported the numpy module. This module is
NOT standard, so if you are working on your own machine, you may need to install it first.
Quiz Data
A starter file is provided for you that contains the quiz data as a list-of-lists. The data is organized such that
each sublist represents the quiz result for a single student. Each sublist is the same length and contains
multiple integers, indicating the student's score on each…
arrow_forward
Python use
arrow_forward
Please give me correct solution.
arrow_forward
Language is C++
Lab10B: Binary Bubbles. Binary search is a very fast searching algorithm, however it requires a set of numbers to be sorted first. For this lab, create an array full of 11 integers which the user will generate. Like in the previous lab, assume that the values will be between -100 and +100. Then, using the sorting algorithm called BubbleSort, put the array in the correct order (from lowest to highest number). After this, please printthe array to the screen. Finally, search the array for the target value using Binary Search.The BinarySearch code will implement the algorithm described in the lecture slides. During this, you should print out a few key values which help Binary Search function. For example, this algorithm focuses on a low, mid, and high which correspond to the indices in the array the algorithm is currently considering and searching. Printing these values during the search process will help with debugging and fixing any issues. • BubbleSort sorts the array…
arrow_forward
Alert dont submit AI generated answer.
arrow_forward
create an INDEXCEPTION value of the unique vowels and unique consonants that the name that was given to you can create. Use python
processStep 1: For every unique vowel of a name, you need to get the first index of that vowel from the original string.Step 2: For each vowel index you get from step 1, you will get the unique consonant from that position. if the index is out of scope of the consonant array, ignore that vowel index.Step 3: For each unique consonant that you get from step 2, you need to get the first index of that consonant from the original string.Step 4: Get the value of indexception by adding all index you get from step 3.outputTO BE DISPLAYED- 2 lists that consists of unique vowels and unique consonants- The vowel, the vowel index, the consonant, the consonant indexFINAL OUTPUT- The total indexception value of the name that was given to you.
arrow_forward
Let's add one last user-defined method to the program.
This method, called FindName, is to return an integer representing the index (position) in the array where the name is found or 1
otherwise.
The approach is to use a simple for loop to search the array and examine each location to see if the name stored at the location is what
you are searching for. If it is, return the array index. If you search the entire array and do not find the name, return a -1.
The method header will look like:
public static int FindName(string name, string[] arrItems, int num)
Add the following lines of code to Main after the call to Reverse Dump
string locateName "Sue";
int found =
if(found >= 0)
FindName(locateName, dataArray, n);
else
Console.WriteLine("{0} was found in the array", locateName);
Console.WriteLine("{0} does not exist in the array", locateName);
Provide the details (the code) of your FindName method.
Copy
Copy
arrow_forward
Write code that includes a Sub Procedure that includes an array initialized with integers, statements that print the total number of integers in the array, the sum of all integers in the array, and a For Each loop that prints the elements of the array. Include another For Each loop that add the elements of the array to a listbox.
arrow_forward
This is method is in the Java Arrays and uses the most efficient sort algorithm possible to sort an array. We should always use it in practice because it has been verified by years of use by Java programmer._______
arrow_forward
Imagine you want to use insertion sort for sorting a deck of cards, where the suits are ordered [Clubs, Spades, Diamonds, Hearts]. Thus, all the clubs will be ordered [Ace, 1, 2.., Queen, King], then all the Spades, etc. Create the pseudocode for this problem
arrow_forward
do both tasks
arrow_forward
This program is written in pseudocode."Design a program that generates a 7-ddigit lottery number. The program should have an Integer array with 7 elements. Write a loop that steps through the array, randomly generating a number in the range of 0 through 9 for each element. Then write another loop that displays the contents of the array."Just like my previous question, I'm not sure how to combine arrays with loops, in addition to the random function.
arrow_forward
Multi Dimensional Arrays in C Program
Ask the user for the number of rows and columns of a two-dimensional array and then its integer elements.
Then, ask the user for another integer value which represents a boogeyman. A boogeyman is a value that we intend to find from a given array.
Find and output the coordinates of the boogeyman by this format:
"BOOGEYMAN LOCATED AT ROW {row_number}, COLUMN {column_number}!"
Input
1. One line containing an integer for the number of rows
2. One line containing an integer for the number of columns
3. Multiple lines containing at least one integer each line for the elements of the array
Sample
1 4 2 3
9 1 2 3
4 2 1 1
9 2 8 3
4. One line containing an integer for the boogeyman's value
Output
Print the row of the position first and then the column of the position. Note that the row and the column is 0-based (i.e. it starts at 0 and not at 1).
It is guaranteed that the boogeyman exists and only once in the 2D array.
Enter# of rows: 4
Enter…
arrow_forward
Assignment
A scientist has developed a mathematical model for a physical process, and he wants to check how
good is model is. To evaluate the correctness of his model, he wants to test the results of his model
under certain parameters and compare them with experimental results.
Write a program that first reads the number of tests (testCount) as an int followed by the results of
each test according to the model as a double array (testModel) and finally the results of each test
according to experiments as a double array (testExperiment). Then, the program should calculate
the error of the model by evaluating the average of the absolute values (i.e., mutlak değer) of the
differences between the model result and experimental result (see formula below) using a function.
ItestModel,- textExperiment,
testCount
NOTE: Individual absolute value of the difference between the model value and the experiment value
calculations MUST be done in the function. Every other functionality MUST be done in…
arrow_forward
Assignment
A scientist has developed a mathematical model for a physical process, and he wants to check how good is model is. To evaluate the correctness of
his model, he wants to test the results of his model under certain parameters and compare them with experimental results.
Write a program that first reads the number of tests (testCount) as an int followed by the results of each test according to the model as a double
array (testModel) and finally the results of each test according to experiments as a double array (testExperiment). Then, the program should
calculate the error of the model by evaluating the average of the ratio of absolute values (i.e., mutlak değer) of the differences between the model
result and experimental result to the model result (see formula below) using a function.
testCount testModel, - textExperiment,
testModel,
Error=
Input
testCount
NOTE: Individual ratio of absolute value of the difference between the model value and the experiment value to the model…
arrow_forward
Write a loop snippet (just a piece of code, not the whole program) that will sum every third element in a 1D array. The size of the array is held in the variable MAX. You will start with the first element in the array. The name of the array is testarray.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
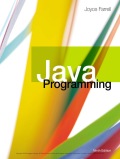
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Related Questions
- Assignment 5A: Multiple Frequencies. In the last assignment, we calculated the frequency of a coin flip. This required us to have two separate variables, which we used to record the number of heads and tails. Now that we know about arrays, we can track the frequency of all numbers in a randomly generated sequence. For this program, you will ask the user to provide a range of values (from 1 to that number, inclusive) and how long of a number sequence you want to generate using that number range. You will then generate and save the sequence in an array. After that, you will count the number of times each number occurs in the sequence, and print the frequency of each number. Hints: You can use multiple arrays for this assignment. One array should hold the number sequence, and another could keep track of the frequencies of each number. Sample Output #1: What's the highest number you want to generate?: 5 How Long of a number sequence do you want to generate?: 10 Okay, we'll generate 10…arrow_forwardIn this lab, we will practice the use of arrays. We will write a program that follows the steps below: Input 6 numbers from the user user inputs numbers one at a time Loop continuously until the exit condition below: ask the user to search for a query number if the query number is in the list: reports the first location of the query number in the list, change the number in that position to 0, show the updated list if the query number is not in the list: exit the loop You must write at least one new function: findAndReplace(array1,...,query) – take in an array and query number as input (in addition to any other needed inputs); it will return the first location of the query number in the list (or -1 if it is not in the list) and will change the number at that location to 0. You may receive the number inputs and print all outputs in int main if you wish. You may report location based on 0-indexing or 1-indexing (but you need 0-indexing to access the array elements!).arrow_forwardc# Write a program , that allows the user to enter an array of elements (integers) then sort these elements in ascending. Hint: first define the array, then loop to enter the elements of the array, and finally use the usual sorting code.arrow_forward
- Using c++ Create this program in this given instructions. Using a random number generator, create a list of 500 integers. Perform a benchmark analysis using some of the sorting algorithms from this chapter. What is the difference in execution speed? Implement the bubble sort using simultaneous assignment. A bubble sort can be modified to “bubble” in both directions. The first pass moves “up” the list, and the second pass moves “down.” This alternating pattern continues until no more passes are necessary. Implement this variation and describe under what circumstances it might be appropriate.arrow_forwardWrite a program , that allows the user to enter an array of elements (integers) then sort these elements in ascending. Hint: first define the array, then loop to enter the elements of the array, and finally use the usual sorting code.arrow_forwardTrue or False. A simple variable, also called a scalar variable, is one that is unrelated to any other variable in the computer’s internal memory. The bubble sort algorithm gets its name from the fact that as the larger values drop to the bottom of the array, the smaller values rise, like bubbles, to the top. Programmers use arrays to temporarily store related data in the internal memory of the computer. Different variables in the same array may have different data types. The elements in an array can be used just like any other variables. When an array is sorted in ascending order, the first element contains the largest value and the last element contains the smallest value. When passing an array by reference in C++, you do not include the address-of operator before the formal parameter’s name in the function header. You distinguish one variable in a one-dimensional array from another variable in the same array using a unique integer,…arrow_forward
- You are given two different methods for sorting the values in an array: Sort1 and Sort2. You have determined that Sort1 = O(nlog n) and Sort2 = O(n2). You need to choose one of these two sorting methods to sort lists in a program you are writing, but you would like to choose the one which completes the fastest. Given this information, is either of these two methods guaranteed to be complete faster than the other? If so, explain which one is faster and why. If not, explain why not.arrow_forwardIN C PROGRAMMING LANGUAGE AND COMMENT EVERY LINE PLEASE SO I CAN UNDERSTAND EVERY STEP , The selection sort is one of several techniques for sorting an array. A selection sort compares every element of an array with all the other elements of the array and exchanges their values if they are out of order. After the first pass of a selection sort, the first array element is in the correct position; after the second pass the first two elements of the array are in the correct position, and so on. Thus, after each pass of a selection sort, the unsorted portion of the array contains one less element. Write and test a function that implements this sorting method.arrow_forwarda C++ program that will load 100 integers into array n. Separate the even from the odd valued elements. The procedure should place the even values in ascending order starting from the first location of the original array and place the odd values in ascending order starting from the last position of the original array. Attached photo is the supposed result of the program created.arrow_forward
- Problem 1 – Adding contactsIn this problem, you will create the skeleton for your entire assignment. In the next problems, you will add more functionality to your program.Our goal on this problem is to create a Rolodex to store names and e-mails (using 1D or 2D arrays, you can choose). The Rolodex can store up to 256 contacts. The Rolodex will store the contact's name as one String and the contact's e-mail as another String.Your application will work as follows:1. Upon starting, it will greet the user and prompt the user to select an option (an integer)2. There are three options in problem 1:1 – to add contactnote: If the Rolodex is full, i.e. there are 256 contacts stored, this option should print: "Roldex is Full" and ask the user to input a new option5 – to print all contacts0 – to exitIf the user inputs an incorrect value, the program should prompt for an option againIf the users select option 1:1. The application will then ask for the contact's name and e-mail (see the example for…arrow_forwardComputer sciencearrow_forwardProgrammingarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
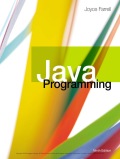
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT