Assignment1 (1)
.pdf
keyboard_arrow_up
School
Toronto Metropolitan University *
*We aren’t endorsed by this school
Course
123
Subject
Computer Science
Date
Dec 6, 2023
Type
Pages
1
Uploaded by ramabarham18
CIND 123 - Data Analytics: Basic Methods - Assignment 1 (10%)
2023-10-17
Question 1 (32 points)
Q1a (8 points)
Create and print a vector x with all integers from 15 to 100 and a vector y containing multiples of 5 in the same range. Hint: use seq()function. Calculate the difference in lengths of the vectors x and y. Hint: use length()
insert your answer here:
# START OF ANSWER FOR Q1a
#Create and print a vector x with all integers from 4 to 115
x <-c(4:115)
print(x)
##
[1]
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
##
[19]
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
##
[37]
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
##
[55]
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
##
[73]
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
##
[91]
94
95
96
97
98
99 100 101 102 103 104 105 106 107 108 109 110 111
## [109] 112 113 114 115
#finding length of x
Lx<-length(x)
#Creating a vector y containing multiples of 4 in the same range as x
y <- seq(4, 115, by = 4)
print(y)
##
[1]
4
8
12
16
20
24
28
32
36
40
44
48
52
56
60
64
68
72
76
## [20]
80
84
88
92
96 100 104 108 112
#finding length of y
Ly<-length(y)
#Calculating the difference in lengths between vectors x and y
print(Lx-Ly)
## [1] 84
# END OF ANSWER FOR Q1a
Q1b (8 points)
Create a new vector, y_square, with the square of elements at indices 1, 3, 7, 12, 17, 20, 22, and 24 from the variable y. Hint: Use indexing rather than a for loop. Calculate the mean and median of the FIRST five values from
y_square.
Insert your answer here.
# START OF ANSWER FOR Q1b
#Create a new vector,y_square, with the square of elements at indices 1, 3, 7, 12, 17, 20, 22, and 24 from the variable
#y. Hint: Use indexing rather than a for loop. Calculate the mean and median of the FIRST five values from y_square.
#INSERT ANSWER HERE
#creating variable y with values of 1, 3, 7, 12, 17, 20, 22, 24
y<-c(1, 3, 7, 12, 17, 20, 22, 24)
#squaring the values from y under new variable y_square
y_square<-(y^2)
#Calculating the mean and median of the FIRST five values from y_square.
mean_first_five<-(mean(y_square[1:5]))
median_first_five<-(median(y_square[1:5]))
# printing the mean
print(mean_first_five)
## [1] 98.4
#printing the median
print(median_first_five)
## [1] 49
# END OF ANSWER FOR Q1b
Q1c (8 points)
For a given factor variable of factorVar <- factor(c(1, 6, 5.4, 3.2)), would it be correct to use the following commands to convert factor to number?
as.numeric(factorVar)
If not, explain your answer and provide the correct one.
Insert your answer here.
# START OF ANSWER FOR Q1c
# It is incorrect to only use the command "as.numeric(factorVar)" to convert the factor to number.
# In order to correctly convert a factor to a number, you need to either: 1) use as.charactor() to convert the values in the factorVar, then use as.numeric() to convert the values to numbers;
# or 2) use level() to extract the level labels, then use as.numeric() to convert the labels to numbers
# I will be using method 1 to provide the correct solution:
factorVar <- factor(c(1, 6, 5.4, 3.2))
#Using as.charactor() to convert the values in the factorVar
characterVar <- as.character(factorVar)
#Using as.numeric() to convert the values to numbers;
numericVar <- as.numeric(characterVar)
# Testing if the factor is cateogrized as a numeric class
is.numeric(numericVar)
## [1] TRUE
# END OF ANSWER FOR Q1c
Q1d (8 points)
A comma-separated values file dataset.csv consists of missing values represented by Not A Number (null) and question mark (?). How can you read this type of files in R? NOTE: Please make sure you have saved the dataset.csv file
at your current working directory.
Insert your answer here.
# START OF ANSWER FOR Q1d
# Set the file path
file_path <- "dataset.csv"
# Read the CSV file, specifying the missing values
read.csv(file_path, na.strings = c("null", "?"))
##
X1
X2
X3
X4
X5
X6
X7
X8
X9 X10
## 1
11
12
13
14
15
16
17
18
19
20
## 2
21
22
23
24
25
26
27
28
29
30
## 3
31
32
33
34
35
36
37
38
39
40
## 4
41
42
43
44
45
NA
47
48
49
50
## 5
51
52
53
NA
55
56
57
NA
59
60
## 6
61
62
63
64
65
66
67
68
69
70
## 7
71
72
NA
74
75
76
77
78
79
80
## 8
81
82
83
84
85
86
87
88
89
NA
## 9
91
92
93
94
95
96
97
98
99 100
## 10
NA 102 103 104 105 106 107 108 109 110
## 11 111 112 113 114 115 116 117 118 119 120
## 12 121 122 123 124 125 126 127 128 129 130
## 13 131 132 133 134 135 136 137 138 139
NA
## 14 141 142 143 144 145 146 147 148 149 150
## 15 151 152 153 154 155 156 157 158 159 160
## 16 161 162 163 164
NA 166 167 168 169 170
# END OF ANSWER FOR Q1d
Question 2 (32 points)
Q2a (8 points)
Compute:
sigma ((-1)^x / (x!)^2) with x ranging from 5 to 20
Hint: Use factorial(n) to compute
Insert your answer here.
# Initialize the sum variable
sum_value <- 0
# Iterate from 5 to 20
for (n in 5:20) {
sum_value <- sum_value + ((-1)^n / (factorial(n)^2))
}
# Compute the final result
print(sum_value)
## [1] -6.755419e-05
# END OF ANSWER FOR Q2a
Q2b (8 points)
Compute:
pi-product ((4 * n) + (1/2^n)) with n ranging from 1 to 5
NOTE: The pi-product symbol represents multiplication.
Insert your answer here.
# START OF ANSWER FOR Q2b
# Initialize the product variable
product_value <- 1
# Calculate the product
for (n in 1:5) {
product_value <- product_value * (4 * n + 1/(2^n))
}
# Calculate the final result
print(product_value)
## [1] 144833.6
# END OF ANSWER FOR Q2b
Q2c (8 points)
Describe what the following R command does: c(0:5)[NA]
Insert your answer here.
# START OF ANSWER FOR Q2c
# The R command c(0:5)[NA] creates a numeric vector containing the numbers 0 through 5.
# The c() function is what creates the vector and (0:5) describes the range from 0 to 5.
vector <- c(0:5)
# The [NA] is an index which stands for (Not Available]. This index extracts elements from the vector.
Output <- c(0:5)[NA]
# However, since the index is NA, the command will generate a vector of NA values with the same length as the original vector, where each element is NA:
# Example: Output - [1] NA NA NA NA NA NA
print(Output)
## [1] NA NA NA NA NA NA
#In summary, the command creates a vector of NA values with the same length as the numbers 0 through 5.
# END OF ANSWER FOR Q2c
Q2d (8 points)
Describe the purpose of is.vector(), is.character(), is.numeric(), and is.na() functions? Please use x <- c(“a”, “b”, NA, 2) to explain your description.
Insert your answer here.
# START OF ANSWER FOR Q2d
# The purpose of these functions is to determine the data type of the object by determining if it's TRUE or FALSE.
# I will be using the value x <- c("a", "b", NA, 2) to explain my description.
x <- c("a", "b", NA, 2)
# The purpose of the is.vector() function checks whether an object is a vector or not.
is_vector_output <- is.vector(x)
print(is_vector_output)
# Output: TRUE since x is a vector.
## [1] TRUE
# The purpose of the is.character() function checks whether an object is a character or not.
is_character_output <- is.character(x)
print(is_character_output) # Output: TRUE for the first two elements ("a" and "b") and FALSE for NA and 2.
## [1] TRUE
# The purpose of the is.numeric() function checks whether an object is a numeric type (i.e., numbers).
is_numeric_output <- is.numeric(x)
print(is_numeric_output)
# In this case, the output is: FALSE (a) FALSE (b) FALSE (NA) TRUE (2)
## [1] FALSE
# The purpose of the is.na() function checks whether elements of an object are missing (NA).
is_na_output <- is.na(x)
print(is_na_output)
# Output: FALSE (a) FALSE (a) TRUE (NA) FALSE (2)
## [1] FALSE FALSE
TRUE FALSE
# END OF ANSWER FOR Q2d
Question 3 (36 points)
The airquality dataset contains daily air quality measurements in New York from May to September 1973. The variables include Ozone level, Solar radiation, wind speed, temperature in Fahrenheit, month, and day. Please see the
detailed description using help(“airquality”).
Install the airquality data set on your computer using the command install.packages(“datasets”). Then load the datasets package into your session.
library(datasets)
Question 3a (4 points)
Display the first 10 rows of the airquality data set.
Insert your answer here.
library(datasets)
#Using the head() function to display the first 10 rows present in the input data frame.
head(airquality, n = 10)
##
Ozone Solar.R Wind Temp Month Day
## 1
41
190
7.4
67
5
1
## 2
36
118
8.0
72
5
2
## 3
12
149 12.6
74
5
3
## 4
18
313 11.5
62
5
4
## 5
NA
NA 14.3
56
5
5
## 6
28
NA 14.9
66
5
6
## 7
23
299
8.6
65
5
7
## 8
19
99 13.8
59
5
8
## 9
8
19 20.1
61
5
9
## 10
NA
194
8.6
69
5
10
# END OF ANSWER FOR Q3a
Question 3b (8 points)
Compute the average of the first four variables (Ozone, Solar.R, Wind and Temp) for the fifth month using the sapply() function. Hint: You might need to consider removing the NA values; otherwise, the average will not be
computed.
Insert your answer here.
# START OF ANSWER FOR Q3b
library(datasets)
# Define a function to calculate the mean excluding NA values
omitna_mean <- function(value) {
mean(value, na.rm = TRUE)
}
# Use sapply to apply the function to each column
mean_values <- sapply(airquality[1:5, ], omitna_mean)[0:4]
# Print the mean values
print(mean_values)
##
Ozone Solar.R
Wind
Temp
##
26.75
192.50
10.76
66.20
# END OF ANSWER FOR Q3b
Question 3c (8 points)
Construct a boxplot for the all Wind and Temp variables, then display the values of all the outliers which lie beyond the whiskers.
Insert your answer here.
# START OF ANSWER FOR Q3c
library(datasets)
# Airquality$Wind represents the Wind variable, and airquality$Temp represents the Temperature variable.These are the variables/columns that will be used for the boxplot.
# The names refer to first boxplot, labeled "Wind", and the second,
labeled "Temp".
boxplot(airquality$Wind, airquality$Temp, names = c("Wind", "Temp"), outline = TRUE)
# outline is equal to TRUE to display the outliers.
outliers <- boxplot.stats(airquality$Wind)$out
# Printing outliers which lie beyond the whiskers.
outliers <- c(outliers, boxplot.stats(airquality$Temp)$out)
print(outliers)
## [1] 20.1 18.4 20.7
# END OF ANSWER FOR Q3c
Question 3d (8 points)
Compute the upper quartile of the Wind variable with two different methods. HINT: Only show the upper quartile using indexing. For the type of quartile, please see
https://www.rdocumentation.org/packages/stats/versions/3.6.2/topics/quantile
.
Insert your answer here.
# START OF ANSWER FOR Q3d
# Method 1: Using quantile() function
#calculating the upper quartile (third quartile) probs set to 75% and type set to 7
quartile_method1 <- quantile(airquality$Wind, probs = 0.75, type = 7)
print(quartile_method1)
##
75%
## 11.5
# Method 2: Using indexing
#Sorting the Wind variable from the 'airquality' dataset.
wind_sorted <- sort(airquality$Wind)
# Calculating number of elements in the sorted list and assigning it to variable 'x'.
x <- length(wind_sorted)
# Calculating the upper quartile by taking the value at the position corresponding to 75% of the data when sorted.
quartile_method2 <- wind_sorted[(x * 0.75)]
print(quartile_method2)
## [1] 11.5
# END OF ANSWER FOR Q3d
Question 3e (8 points)
Construct a pie chart to describe the number of entries by Month. HINT: use the table() function to count and tabulate the number of entries within a Month.
Insert your answer here.
# START OF ANSWER FOR Q3e
library(datasets)
# Counting the number of entries by Month.
count <- table(airquality$Month)
# Create a pie chart
pie(count, labels = names(count), main = "Number of Entries by Month")
# END OF ANSWER FOR Q3e
Discover more documents: Sign up today!
Unlock a world of knowledge! Explore tailored content for a richer learning experience. Here's what you'll get:
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Q3: a- Write a program to pass a two dimensional square matrix as a parameter to a function; this
function is used to convert the elements of the first row of the matrix to the average elements of the
second and fourth rows; finally display the matrix after change.
arrow_forward
Q2:
Write a MATLAB code to create a
matrix having 20 rows and 30
columns then calculate both S1
and S2, where S1 is the sum of the
elements having values equal or
less than the mean value of the
matrix and S2 is the sum of the
matrix elements with values
greater than the mean value of
the matrix.
Your answer
arrow_forward
Q5) Run the code below to check on the model behavior for different polynomials (2,3, 5,10,20). Comment on the generated figure.
In [26]: # Hyperparam initialization
eta = 0.25
epochs = 500000 # This will take a while but you can set it to 10000.
Poly_degree_values = [2, 3, 5, 10, 20]
# Initializing plot
plt.title( "Regression Lines for Different polynomials")
plt.scatter(data.GranulesDiameter, data.Beachslope, label='Traning Data')
# iterating over different alpha values (This is going to take a while)
for i in Poly_degree_values:
polynomial x = GeneratePolynomialFeatures (X, i)
thetaInit - np.zeros( (polynomial_x.shape[1],1))
theta, losses = gradientDescent (polynomial_x, Y, thetaInit, eta, epochs)
poly = PolynomialFeatures (i)
plot SimpleNonlinearRegression line (theta, X, poly)
plt.legend(Poly_degree_values)
plt.show()
Regression Lines for Different polynomials
2
25
10
20
20
15
10
0.2
0.3
0.4
0.5
0.6
0.7
0.8
In [271: # Write your response here
arrow_forward
Directions: Read each item carefully. Create command/codes based on the information requested on each item. (R programming)
Create a list that contains a numeric vector from (1 to 30, 34, 45, 47, and 50 to 70), a character vector that repeat the elements A, B, and C 30 times, and a 2 x 3 matrix that contains a number from 101 to 106 filled by rows.
arrow_forward
Complete the following Code
arrow_forward
Horizontal sequence :VIRL
Vertical sequence:MKF
Scoring rules: g/o = -3, g/e = -1, match or mismatch - from PAM250 substitution matrix below.
NW algorithm.
1. Complete the scoring matrix.
Scoring matrix with PAM250 scores:
V
I
R
L
M
K
F
2. Set up, initialize and complete the NW matrix.
3. Retrace, align and score alignment(s).
Use the arrows and circles for the matrix and path(s).
V
I
R
L
M
K
F
Align and score all optimal alignments here.
PLZ the arrows and circles for the matrix and path(s) AND SHOW ALL possible Alignment
arrow_forward
Horizontal sequence :VIRL
Vertical sequence:MKF
Scoring rules: g/o = -3, g/e = -1, match or mismatch - from PAM250 substitution matrix below.
NW algorithm.
1. Complete the scoring matrix.
Scoring matrix with PAM250 scores:
V
I
R
L
M
K
F
2. Set up, initialize and complete the NW matrix.
3. Retrace, align and score alignment(s).
Use the arrows and circles for the matrix and path(s).
V
I
R
L
M
K
F
Align and score all optimal alignments here.
arrow_forward
Horizontal sequence :RIVL
Vertical sequence:FMK
Scoring rules: g/o = -3, g/e = -1, match or mismatch - from PAM250 substitution matrix below.
SW algorithm.
1. Complete the scoring matrix.
Scoring matrix with PAM250 scores:
R
I
V
L
F
M
K
2. Set up, initialize and complete the SW matrix.
3. Retrace, align and score alignment(s).
Use the arrows and circles for the matrix and path(s).
R
I
V
L
F
M
K
Align and score all optimal alignments here.
PLZ the arrows and circles for the matrix and path(s) AND SHOW ALL possible Alignment
arrow_forward
Q2 :
1. Write two ways to display the following matrix
(
3
4
5
6
A =
13
14
15
16
17
2.
()
4
3
Let A =
4
9
a) using the columns operator (:), creat a column vector that contains all the columns of A.
b) using the columns operator (:), creat a column vector that contains all the Rows of A.
c) using the columns operator (:),creat a Row vector that contains the 1st row and 3rd columns of A.
d) using the columns operator (:), creat a column vector that contains all the columns of A.
e) using the columns operator (:), creat a column vector that contains all the columns of A.
arrow_forward
Write a PHP code to:
Create the following two dimensional array $students:
ID
Name
Score
100
Ali
76
200
Khaleed
33
300
Fatema
89
400
Sumaya
45
Write the function nbFail($list) that takes a multidimensional array $list as parameter and returns the number of failed students (student fails if the score is less than 50).
Write the required PHP code to display the array $students in an HTML table (using foreach statement) and the number of failed students and passed students (use the function defined in question b).
arrow_forward
Vector Creation (creating equally spaced vectors)
Write a script with commands to create the following vectors and assign to the indicated variable names.
■ Create an evenly-spaced row vector A with elements starting at 0 and ending at 50 with increments of 0.5.
▪ Create a row vector B with 80 evenly-spaced elements starting at 0 and ending at л/2.
Your code should not include the following MATLAB functions or keywords: for, while.
Hint: one line of your solution should start with
A =
and the other line of your solution should start with
B =
arrow_forward
Function Name: odd_even_diagParameters: a 2D list (list of lists)Returns: list of lists
Description: Given a 2-dimensional matrix (list of lists) with any size (n*n), modify it according to the following rules:
Find the sum of the main diagonal.
If the sum is an odd number, change all the values of the given matrix (except the main diagonal) to 0.
If the sum is an even number, change all the values of the given matrix (except the main diagonal) to 1.
Return the resulting matrix.
Example 1:If argument is:
[[1, 2], [4, 3]]
odd_even_diag should return:
[[1, 1], [1, 3]]
because the sum 1 + 3 is even.
Example 2:If argument is:
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
odd_even_diag should return:
[[1, 0, 0], [0, 5, 0], [0, 0, 9]]
because the sum 1 + 5 + 9 is odd.
arrow_forward
2x3 matrix.tlab.
arrow_forward
Please true or false
arrow_forward
Q1: write a program to read the elements of a two dimensional square matrix which consists
of 4 rows and 4 columns then convert it to the following matrix.
133 3
513 3
5513
55 5 1
arrow_forward
c) .Use loops to create a matrix in which the value of each element is two times its row
number minus three times its column number. For example, the value of element (2,5)
2*2-3*5=-11
AAT --
arrow_forward
Lab 1 - 2D Lists
For this lab, you will work with two-dimensional lists in Python. Do the following:
1. [4 points] Write a function that returns the sum of all the elements in a specified column in a matrix using the following header:
def sumColumn(matrix, columnindex)
2. [2 points] Write a function display() that displays the elements in a matrix row by row, where the values in each row are displayed on a separate line. Use a single
space to separate different values. Sample output from this function when it is called on a 3 X4 matrix is as follows:
2.5 3.0 4.0 1.5
1.5 4.0 2.0 7.5
3.5 1.0 1.0 2.5
3. [4 points] Given this partial code
def getMatrix(numRows, numColumns):
"returns a matrix with the specified number of rows and columns"
m = [] # the 2D list (i.e., matrix), initially empty
for row in range(numRows):
matrix_dimensions_string = str(numRows) + "-by-" + str(numColumns)
line = input("Enter a "+ matrix_dimensions_string + " matrix row for row "+ str(row) + ": ")
return m
write a…
arrow_forward
get_column_choice(): this function will allow us to ask the players which pocket they want to grab stones from. Since we know which row they are grabbing from (since it is not allowed to grab stones from your opponent’s side), we only need to get the column they want. As previously stated, we are using letters to represent the columns to make it easier on the player. This function accepts the current player (should be a 0 or 1), the board, and a list of letters (the same as the list you passed to the print_game_board function) and it will return the index of the column being chosen.
The function should ask the player to enter a column letter, then it should check that the conditions listed below hold. If they don’t meet these conditions, it should ask again until it gets a valid letter, otherwise it should return the corresponding column index for that letter.
Letter entered must be on the game board. It can be lower case or upper case. (Hint: this doesn’t need to be hard coded,…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
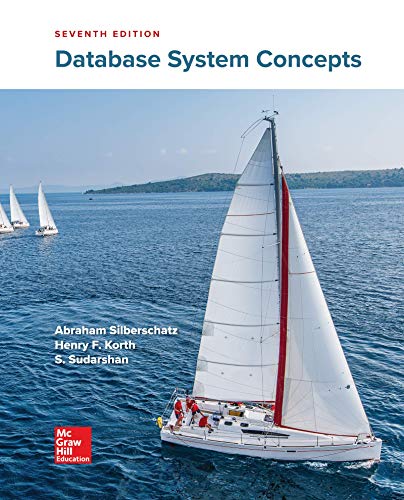
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
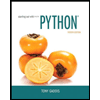
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
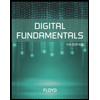
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
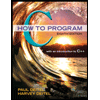
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
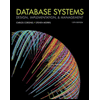
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
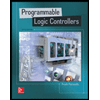
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Q3: a- Write a program to pass a two dimensional square matrix as a parameter to a function; this function is used to convert the elements of the first row of the matrix to the average elements of the second and fourth rows; finally display the matrix after change.arrow_forwardQ2: Write a MATLAB code to create a matrix having 20 rows and 30 columns then calculate both S1 and S2, where S1 is the sum of the elements having values equal or less than the mean value of the matrix and S2 is the sum of the matrix elements with values greater than the mean value of the matrix. Your answerarrow_forwardQ5) Run the code below to check on the model behavior for different polynomials (2,3, 5,10,20). Comment on the generated figure. In [26]: # Hyperparam initialization eta = 0.25 epochs = 500000 # This will take a while but you can set it to 10000. Poly_degree_values = [2, 3, 5, 10, 20] # Initializing plot plt.title( "Regression Lines for Different polynomials") plt.scatter(data.GranulesDiameter, data.Beachslope, label='Traning Data') # iterating over different alpha values (This is going to take a while) for i in Poly_degree_values: polynomial x = GeneratePolynomialFeatures (X, i) thetaInit - np.zeros( (polynomial_x.shape[1],1)) theta, losses = gradientDescent (polynomial_x, Y, thetaInit, eta, epochs) poly = PolynomialFeatures (i) plot SimpleNonlinearRegression line (theta, X, poly) plt.legend(Poly_degree_values) plt.show() Regression Lines for Different polynomials 2 25 10 20 20 15 10 0.2 0.3 0.4 0.5 0.6 0.7 0.8 In [271: # Write your response herearrow_forward
- Directions: Read each item carefully. Create command/codes based on the information requested on each item. (R programming) Create a list that contains a numeric vector from (1 to 30, 34, 45, 47, and 50 to 70), a character vector that repeat the elements A, B, and C 30 times, and a 2 x 3 matrix that contains a number from 101 to 106 filled by rows.arrow_forwardComplete the following Codearrow_forwardHorizontal sequence :VIRL Vertical sequence:MKF Scoring rules: g/o = -3, g/e = -1, match or mismatch - from PAM250 substitution matrix below. NW algorithm. 1. Complete the scoring matrix. Scoring matrix with PAM250 scores: V I R L M K F 2. Set up, initialize and complete the NW matrix. 3. Retrace, align and score alignment(s). Use the arrows and circles for the matrix and path(s). V I R L M K F Align and score all optimal alignments here. PLZ the arrows and circles for the matrix and path(s) AND SHOW ALL possible Alignmentarrow_forward
- Horizontal sequence :VIRL Vertical sequence:MKF Scoring rules: g/o = -3, g/e = -1, match or mismatch - from PAM250 substitution matrix below. NW algorithm. 1. Complete the scoring matrix. Scoring matrix with PAM250 scores: V I R L M K F 2. Set up, initialize and complete the NW matrix. 3. Retrace, align and score alignment(s). Use the arrows and circles for the matrix and path(s). V I R L M K F Align and score all optimal alignments here.arrow_forwardHorizontal sequence :RIVL Vertical sequence:FMK Scoring rules: g/o = -3, g/e = -1, match or mismatch - from PAM250 substitution matrix below. SW algorithm. 1. Complete the scoring matrix. Scoring matrix with PAM250 scores: R I V L F M K 2. Set up, initialize and complete the SW matrix. 3. Retrace, align and score alignment(s). Use the arrows and circles for the matrix and path(s). R I V L F M K Align and score all optimal alignments here. PLZ the arrows and circles for the matrix and path(s) AND SHOW ALL possible Alignmentarrow_forwardQ2 : 1. Write two ways to display the following matrix ( 3 4 5 6 A = 13 14 15 16 17 2. () 4 3 Let A = 4 9 a) using the columns operator (:), creat a column vector that contains all the columns of A. b) using the columns operator (:), creat a column vector that contains all the Rows of A. c) using the columns operator (:),creat a Row vector that contains the 1st row and 3rd columns of A. d) using the columns operator (:), creat a column vector that contains all the columns of A. e) using the columns operator (:), creat a column vector that contains all the columns of A.arrow_forward
- Write a PHP code to: Create the following two dimensional array $students: ID Name Score 100 Ali 76 200 Khaleed 33 300 Fatema 89 400 Sumaya 45 Write the function nbFail($list) that takes a multidimensional array $list as parameter and returns the number of failed students (student fails if the score is less than 50). Write the required PHP code to display the array $students in an HTML table (using foreach statement) and the number of failed students and passed students (use the function defined in question b).arrow_forwardVector Creation (creating equally spaced vectors) Write a script with commands to create the following vectors and assign to the indicated variable names. ■ Create an evenly-spaced row vector A with elements starting at 0 and ending at 50 with increments of 0.5. ▪ Create a row vector B with 80 evenly-spaced elements starting at 0 and ending at л/2. Your code should not include the following MATLAB functions or keywords: for, while. Hint: one line of your solution should start with A = and the other line of your solution should start with B =arrow_forwardFunction Name: odd_even_diagParameters: a 2D list (list of lists)Returns: list of lists Description: Given a 2-dimensional matrix (list of lists) with any size (n*n), modify it according to the following rules: Find the sum of the main diagonal. If the sum is an odd number, change all the values of the given matrix (except the main diagonal) to 0. If the sum is an even number, change all the values of the given matrix (except the main diagonal) to 1. Return the resulting matrix. Example 1:If argument is: [[1, 2], [4, 3]] odd_even_diag should return: [[1, 1], [1, 3]] because the sum 1 + 3 is even. Example 2:If argument is: [[1, 2, 3], [4, 5, 6], [7, 8, 9]] odd_even_diag should return: [[1, 0, 0], [0, 5, 0], [0, 0, 9]] because the sum 1 + 5 + 9 is odd.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
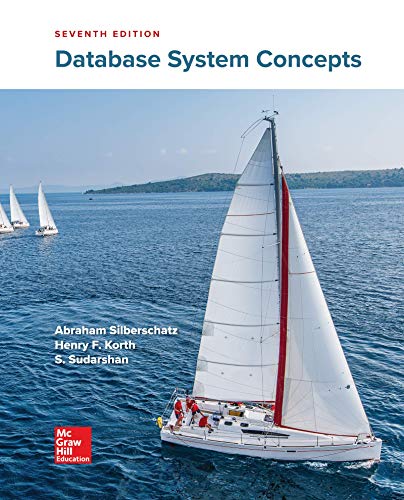
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
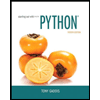
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
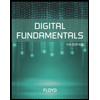
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
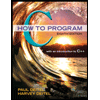
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
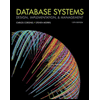
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
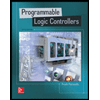
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education