day06notes
.py
keyboard_arrow_up
School
Georgia Institute Of Technology *
*We aren’t endorsed by this school
Course
1301
Subject
Computer Science
Date
Dec 6, 2023
Type
py
Pages
4
Uploaded by BrigadierBravery5404
"""
Day 06 - More conditionals
1. Recap
2. More Boolean Practice
a. Evaluating Boolean Expressions
b. Using Boolean Expressions in Conditionals
c. Returning a Boolean from a Function
d. Using Functions in Conditionals
3. Advanced Conditionals
a. Nested if statements
4. The "in" operator
a. Using "in" with strings
"""
# >>> Day 06 Miniquiz - Problem solving
# === Recap: Conditionals
# booleans
'''
val1 = 78
val2 = 89
comparison = val1 >= val2
print(comparison)
'''
# if/elif/else
'''
val4 = 100
val3 = 10
if val3 < 80:
print("first branch of conditional!")
elif val4 > 50:
print("second branch of conditional!")
else:
print("Nothing was satisfied!")
'''
# if/if vs if/elif
'''
val4=45
val3=100
if val4 > 20:
print("Hey!")
elif val3 > 90:
print("Hello!")
val4=45
val3=100
if val4 > 20:
print("Bye!")
if val3 > 90:
print("Buh-bye!")
'''
# === Conditionals in functions
# Example: Write a function that takes as input someone's
# grades on this course and returns their letter grade.
# HINT: You might need to go to the syllabus to find the
# weights for exams, homeworks, labs, participation and
# the final.
'''
def letterGrade(mid, hw, quiz, final, lab):
total = 0.4 * mid + 0.25 * hw + 0.05 * quiz + 0.2 * final + 0.1* lab
print(total)
if total >=90:
return "A"
elif total >=80:
return "B"
elif total >=70:
return "C"
elif total >=60:
return "D"
else:
return "F"
student1= letterGrade (90, 100, 100, 80, 100)
print(student1)
'''
# === Functions that return booleans
# Example: Write a function that checks whether a number is
# divisible by 4. The function should return the result
# as a bool.
'''
def isDivBy4(num):
remainder = num % 4
if remainder == 0:
return True
else:
return False
print(isDivBy4(16))
def oneLiner(num):
return num%4 == 0
'''
# Example: Write a function that checks whether a password
# is correct or not. The function should return the result
# as a bool.
'''
def isCorrectPwd(pwd):
databasePwd = "helloKitty500"
if pwd == databasePwd:
return True
else:
return False
'''
'''
print(isCorrectPwd("HelloKitty900"))
'''
# === Logical operators (not, and, or)
# not
'''
var23 = True
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Is the following syntax true for of conditional statements.
if condition
code
end
arrow_forward
Q6: Rewrite the following if statements using the conditional operator. (1 mark)
if(x == y)
z = x + y;
else
z = (x + y) / 2;
arrow_forward
Comments
Target Outcome: (CO2) Write algorithms to solve different programming problems using flowchart and pseudo-code.
No Comm
Problem:
The program will display the following when run:
1. Display Pass/Fail Data
2. Display Final Grade Classification
3. Display Mean and Median Score
Call the following function when the user selects from the menu:
1. Determine the number of students who passed (>-75%) and failed (<75%). Plot also the pass/fail data using a pie
chart.
2. Classify and plot (using bar graph) the final grades based on the following:
Expert (95%-100%)
ndar:
Compctent (85%-94%)
Novice (75%-84%)
Beginner (<75%)
3. Calculate the mean and median final gradc. Plot the mean and median score with the plot of all the nnal grades.
Create an algorithm for the programming problcm abovc using pscudo-code or flowchart.
29°C
earch
arrow_forward
Compare the use of if-else statements with the use of conditional operators.
arrow_forward
Can someone help me answer this in Coral programming language?
1) The year must be divisible by 4
2) If the year is a century year (1700, 1800, etc.), the year must be evenly divisible by 400
Some example leap years are 1600, 1712, and 2016.
Write a program that takes in a year and determines whether that year is a leap year.
Ex: If the input is:
1712
the output is:
1712 is a leap year.
Ex: If the input is:
1913
the output is:
1913 is not a leap year.
arrow_forward
Complete Answer
arrow_forward
..and .
.. . are the
conditional statements available in
VBA. *
O IF ... Then and Select Case
O For and While
O Do While and Do Until
O none of them
arrow_forward
Let s1 be " Welcome " and s2 be " welcome ". Write the code for the following statements:
(a) Check whether s1 is equal to s2 and assign the result to a Boolean variable isEqual.
arrow_forward
When are conditional statements appropriate to use? What are the advantages of using conditional statements? What are the key differences that distinguish each statement from the others?
arrow_forward
The condition of an IF statement evaluates to boolean only if the expression contains?
A. Logical operators
B. All of the choices given
C. Relational operators
D. Boolean operands
arrow_forward
In a conjunction of two statements,
even if one of the statements is false,
the whole statement is ....
..... .....
Select one:
O a. true or false
b. false
O c. None
O d. true
arrow_forward
With proper explanation else skip it
arrow_forward
Differentiate between break and continue statements with example.
arrow_forward
15.)
arrow_forward
Math Logic;
For each statement choose if it is simple or compound.
If compound, choose which type
*Conjunction
*Disjunction
*Conditional
*Bioconditional
a. Twilight is my favorite time to sit on the porch. (Simple or Compound)
b. I will get coffee if and only if I want to stay awake. (Simple or Compound)
c. Lemons are yellow and limes are green, (Simple or Compound)
arrow_forward
*2. Express the following Boolean expressions in simpler form; that is, use fewer operators. x is an in
(d) !(x <= y)
arrow_forward
Q: - by using if conditional statements, write a MATLAB code
to check the temperature as following:
high
normal
t> 40
20 < t<40
(low
t< 20
arrow_forward
3. The formula for determining compound interest is:
A represents the amount to earn; P is the principle that is initially invested; r is the interest rate earned; n is the number of times the interest is compounded; and t is the number of time periods for the investment. Write a program that prompts the user to enter P, r, n and t. The program should output the amount earned on the investment. 4. Look up the Pythagorean theorem if you are not already familiar with it. Use the following formula to solve for c in the formula: c = √a2 + b2. Use the proper functions from the cmath header file. Be sure to output the result.l
arrow_forward
(1) Prompt the user for a string that contains two strings separated by a comma. (1 pt)
Examples of strings that can be accepted:
Jill, Allen
Jill , Allen
Jill,Allen
Ex:
Enter input string:Jill, Allen
(2) Print an error message if the input string does not contain a comma. Continue to prompt until a valid string is entered. Note: If the input contains a comma, then assume that the input also contains two strings. (2 pts)Ex:
Enter input string:Jill AllenError: No comma in string.
Enter input string:Jill, Allen
(3) Extract the two words from the input string and remove any spaces. Store the strings in two separate variables and output the strings. (2 pts)Ex:
Enter input string:Jill, AllenFirst word: JillSecond word: Allen
(4) Using a loop, extend the program to handle multiple lines of input. Continue until the user enters q to quit. (2 pts)Ex:
Enter input string: Jill, Allen First word: Jill Second word: Allen Enter input string: Golden , Monkey First word: Golden Second word:…
arrow_forward
Select all the true statements for functions:
A. Capable of taking input.
B. Named group of statements.
C. Can return multiple values.
D. Can return only one value.
arrow_forward
1. The logical operator that both Boolean expressions have to be true for the overall Boolean expression to be true is
. (Use symbol only)
2. The logical operator that both Boolean expressions have to be false for the overall Boolean expression to be false is
. (Use symbol only)
arrow_forward
01/ Choose the correct answer.
1- The - -- is installed after the completion of the well and is used to control the flow of oil and
gas.
a BOP
b- conductor pipe
c- Christmas tree
d- casing pipe
2-The long and wide piece of casing which is the first string to be inserted in the hole is known as a
********
a drill collars
b- conductor pipe
e- drill pipe
d- casing pipe
3. ......... pipe transfer the spin and circulation between the pipe and the drill bit.
a- BHA
b- Drill
e- Heavy
d- Casing
4-
a- Drilling Mud
b- Cement
e- Chemical
d- none of above
5- During drilling process many faults might occur. If an engineer forgot an item in the wellbore, it
could be removed by a process called -
a-kicking
b-drilling
e-casing
d-fishing
6- The layer produced from the drilling mud on the sides of the borehole and forms during filtration is
slurry consists of cement slurry, water, and additives.
mulsion
b- filter cake
a-
e- cement slurry
d- formation fluid
7- When a phase is equally spread into another…
arrow_forward
Which of the following are true about looping statements?
(A It is a part of a computer program that makes code(s) repeat itself
(B It is also known as an iteration statement.
(c) An example of a looping statement is the if-else statement.
(D An example of a looping statement is the do-while statement.
(E) None of the other choices are correct.
arrow_forward
Can you solve this question
arrow_forward
1. Pick variables for the following English statements and then convert the statements to Boolean
statements.
a.
I'll will go to breakfast with Sam if George or Trevor comes, and I will not go if Bob
comes.
b. I'll go to the store if I need to buy: milk and eggs, or tp, or ice (as long as I already have
soda).
arrow_forward
Let p be “It is raining" and let q be "It is snowing". Translate each of the following logical expressions into English statements.
1. -p V ¬q
2. рла
3. тр — д
4. р 9
5. -p
arrow_forward
If the statements p and q are both true while statement r is false. which of the following has a false truth value?* a. If r then (p or q). b. Not (r and (not p or q)). c. (p or r) if and only if not q. d. If not (r and q) then p.
arrow_forward
4-
Write a Boolean expression for the following statement :
F is a "1" if A,B and c are all 1's or if only one of the variables is a
"0".
arrow_forward
7.
The rules of writing 'if-then-else', 'case-switch', 'while-until' and 'for' control flow statements are called _____ .
a.
Comments
b.
Functions
c.
Line length and wrapping
d.
Control Structure
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
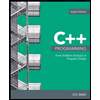
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Related Questions
- Is the following syntax true for of conditional statements. if condition code endarrow_forwardQ6: Rewrite the following if statements using the conditional operator. (1 mark) if(x == y) z = x + y; else z = (x + y) / 2;arrow_forwardComments Target Outcome: (CO2) Write algorithms to solve different programming problems using flowchart and pseudo-code. No Comm Problem: The program will display the following when run: 1. Display Pass/Fail Data 2. Display Final Grade Classification 3. Display Mean and Median Score Call the following function when the user selects from the menu: 1. Determine the number of students who passed (>-75%) and failed (<75%). Plot also the pass/fail data using a pie chart. 2. Classify and plot (using bar graph) the final grades based on the following: Expert (95%-100%) ndar: Compctent (85%-94%) Novice (75%-84%) Beginner (<75%) 3. Calculate the mean and median final gradc. Plot the mean and median score with the plot of all the nnal grades. Create an algorithm for the programming problcm abovc using pscudo-code or flowchart. 29°C earcharrow_forward
- Compare the use of if-else statements with the use of conditional operators.arrow_forwardCan someone help me answer this in Coral programming language? 1) The year must be divisible by 4 2) If the year is a century year (1700, 1800, etc.), the year must be evenly divisible by 400 Some example leap years are 1600, 1712, and 2016. Write a program that takes in a year and determines whether that year is a leap year. Ex: If the input is: 1712 the output is: 1712 is a leap year. Ex: If the input is: 1913 the output is: 1913 is not a leap year.arrow_forwardComplete Answerarrow_forward
- ..and . .. . are the conditional statements available in VBA. * O IF ... Then and Select Case O For and While O Do While and Do Until O none of themarrow_forwardLet s1 be " Welcome " and s2 be " welcome ". Write the code for the following statements: (a) Check whether s1 is equal to s2 and assign the result to a Boolean variable isEqual.arrow_forwardWhen are conditional statements appropriate to use? What are the advantages of using conditional statements? What are the key differences that distinguish each statement from the others?arrow_forward
- The condition of an IF statement evaluates to boolean only if the expression contains? A. Logical operators B. All of the choices given C. Relational operators D. Boolean operandsarrow_forwardIn a conjunction of two statements, even if one of the statements is false, the whole statement is .... ..... ..... Select one: O a. true or false b. false O c. None O d. truearrow_forwardWith proper explanation else skip itarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
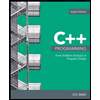
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning