project 2
.docx
keyboard_arrow_up
School
Central Piedmont Community College *
*We aren’t endorsed by this school
Course
121
Subject
Computer Science
Date
Dec 6, 2023
Type
docx
Pages
4
Uploaded by PresidentHackerMonkey2290
# 4/16/2023
# Project 2
# Write a program that will generate a bill for sending a telegram and
# give the user the opportunity to translate a message into Morse code.
# Customers are charged at a rate of $1.50 for blocks of 5 words and $0.50 for
single words.
# 1-Add an option to the menu to offer the user a choice of processing a data
file. Add another option to quit
# the program.
The menu should be the first thing displayed when your program
begins executing.
# The menu format should match the one in the Sample Output
# 2-Allow the user to continue making menu choices until they choose the
option to quit. Always display the menu
# before prompting the user for a menu option.
Properly handle an invalid
menu choice. The user should have an
# unlimited number of chances to enter a valid menu choice.
# 3-Validate the user’s input for number of words sent to not accept values
less than 1. Allow the user an
# unlimited number of chances to enter a valid number for words sent.
# 4-Modify the code you wrote in Project 2 to translate a single letter into a
Morse code. Your code
# should now be able to efficiently translate a complete message of any length
into Morse code.
# All white spaces in the message should be translated into 3 corresponding
white spaces in Morse code.
# 5-Add functionality to create and display telegram bills by reading input
from the TelegramData.txt text file.
# When the user selects the option to process a data file, telegram bills
should be created and displayed for every
# record in the file without the user needing to do anything else. See the
Sample Output for what the bill must contain.
# The TelegramData.txt file contains 5 records, but your program must be able
to process an identically formatted file
# with any number of records without counting the records.
# Function to translate a letter to Morse code
def
translate_to_morse
(
letter
):
morse_dict = {
'A'
:
'.-'
,
'B'
:
'-...'
,
'C'
:
'-.-.'
,
'D'
:
'-..'
,
'E'
:
'.'
,
'F'
:
'..-.'
,
'G'
:
'--.'
,
'H'
:
'
....
'
,
'I'
:
'..'
,
'J'
:
'.---'
,
'K'
:
'-.-'
,
'L'
:
'.-..'
,
'M'
:
'--'
,
'N'
:
'-.'
,
'O'
:
'---'
,
'P'
:
'.--.'
,
'Q'
:
'--.-'
,
'R'
:
'.-.'
,
'S'
:
'...'
,
'T'
:
'-'
,
'U'
:
'..-'
,
'V'
:
'...-'
,
'W'
:
'.--'
,
'X'
:
'-..-'
,
'Y'
:
'-.--'
,
'Z'
:
'--..'
}
return
morse_dict.
get
(
letter
.
upper
(),
''
)
# Function to input - getting data for client
def
process_telegram_bill
():
while True
:
customer_name =
input
(
"Enter customer's first and last name (or 'q' to
quit): "
)
# - Checking if input is q then exit the program
if
customer_name ==
'q'
:
break
street_address =
input
(
"Please enter your address: "
)
city =
input
(
"Please enter your city: "
)
city = city.
capitalize
()
state =
input
(
"Please enter your state (ex. NC for North Carolina): "
)
state = state.
upper
()
zip_code =
input
(
"Please enter your Zip code: "
)
num_words =
input
(
"Please enter how many words in your message to
send: "
)
# Validate number of words
while True
:
try
:
num_words =
int
(num_words)
if
num_words <
1
:
raise
ValueError
break
except
ValueError
:
num_words =
input
(
"Invalid input. Please enter the number of
words[at least 1 or greater]: "
)
# Count number of words and calculate cost
cost_block = num_words //
5
cost_single = num_words %
5
amount_billed = (cost_block *
1.5
) + (cost_single *
0.5
)
# Print the bill
print
()
print
(customer_name)
print
(street_address)
print
(
f'
{
city
}
,
{
state
} {
zip_code
}
'
)
print
(
f'Amount Owed: $
{
amount_billed
:
.2f
}
'
)
print
()
# Function - input - translating text to morse code
def
translate_message
():
message =
input
(
'Enter a message: '
)
# Translate each letter and print the result
result =
''
for
letter
in
message:
if
letter.
isalpha
():
result +=
translate_to_morse
(letter.
upper
())
elif
letter ==
''
:
result +=
''
else
:
result += letter +
''
# Output - printing out the morse code
print
(
f"Translation:
{
result
}\n
"
)
# Function - processing data from file MorseCodeDataFile.txt and outputting it
to print
def
process_data_file
():
print
(
'Processing Morse code data file...'
)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Add the ability to convert an entire message into Morse code, as well as the ability to handle a data file comprising numerous entries. Change your input validation code so that the user has an infinite number of opportunities to enter valid responses. Your program should run until the user selects the "quit" option from your menu.
Add a menu option to provide the user the option of processing a data file. Add another way to exit the program. When your software starts, the menu should be the first item that appears. The menu format should be the same as in the Sample Output.
Allow the user to make more menu selections until they select the option to exit. Before soliciting the user for a menu selection, always show the menu. Handle an incorrect menu option correctly. The user should have an infinite number of opportunities to enter a valid menu option.
Validate the user's input for the number of words sent so that numbers less than one are not accepted. Allow the user an unlimited…
arrow_forward
Python: I am trying to write a simple GUI based program with two input fields “Directory/filename” and “word” next to each other and one output text field below. Using recursion the program should go through all the files and folders searching for the entered word. If a match is found the path to the file should be printed in the text field + the content of line the word is found in . It should also print how many folders and files has been searched and how many times the word was found. Any ideas?
arrow_forward
Add comments for assignment p05Thanks! Run it with C, Linux
arrow_forward
Allow the user the choice of displaying either a random line or a random word from a file they specify. Your menu should allow them to choose these options either from the number of the option or from the capitalized letter. For example,
1) display random Word 2) display random Line 3) Quit
Should allow the user to select a random line display by either entering the number 2 or by entering a letter L (lower or upper case!).
arrow_forward
Python
The Springhill Amateur Golf Club has a tournament every weekend. The club president has asked you to write a Menu-Driven program which has the following functionality:
1. Allows for each player's name and golf score to be entered and saved in a text file named golf.txt.
2. Reads each player's name and golf score from the text file golf.txt and provides an average score.
3. The program will use one variable for the player's name and one variable for the score.
arrow_forward
Java
arrow_forward
OverviewYou should enter this task, ask the user for a word and then search for a file to see if that word is among the words in the file.
Task
Write a java programThis exercise comes with three text files named wordlist1.txt, wordlist2.txt and wordlist3.txt. These files contain a large amount of words in English. Each file contains more and more words. Each word in these files is on a separate line and the words contain only lowercase letters. Your task is to write a program that reads all these words from the file and checks if a keyword, which the user entered, is among the entered words. The program should also count the total number of words contained in the file used. The program should measure how long it takes to complete the search, ie how long it takes to read all the words and check if the keyword is among the words or not.
The program should start by asking the user for the word to be searched for. Then the words in the file should be counted and compared if the keyword…
arrow_forward
VB Small Project 18 – Numbers to a File
Write a Visual Basic program that asks the user for a series of numbers (at least five numbers). Write each number to a text file. Write each number on a separate line in the file.
arrow_forward
Computer Science
Using Java language
Write a procedure that generates a regular heightfield in data-files/model/heightfield.off with elevation given by the grayscale values of an image file. Use a file dialog to select the file, a number box to scale the xx and zz integer coordinates by, and a number box to set the scale that 1.0 corresponds to for height. Make a button labelled “Generate” that launches the generator. Display a message on screen while generating and then flush the G3D::ArticulatedModel cache, and reload the current scene on completion.
arrow_forward
Task – IV:
Write a program that makes a copy of the file. Your program will ask the user for the source file and
the new file names and then will read a line at a time from the input (source) file and prints it to the
output (new file) file with a line number.
You can create your own file "sample.txt" and add your favorite poem.
arrow_forward
Task: Loading data from files
This exercise will require you to load some information from files and use it in your program.
scene.txt contains a description of a series of shapes and colours to draw. You need to write code to read in the file data and draw the requested shapes in the correct colour. Each line in scene.txt will contain one of the following starting keywords followed by some data:
COLOUR followed by 3 values: R, G, B
CIRCLE followed by 3 values: X, Y, RADIUS
RECT Followed by 4 values: X, Y, W, H
LINE Followed by 4 values: X1, Y1, X2, Y2
CIRCLES are defined from the center. RECT's are defined from the top left. All values are space separated, and you can assume all input is correct (no errors). Please solve this program to draw the scene.
I can't upload the file scene.txt, so I decide to screenshot a file for you.
Subject: Java Programming
arrow_forward
Write a program that:
Opens a file named foofoo.txt
Write the phrase, Hi All!
Then writes the odd numbers from 1 – 1000
Then writes Goodbye
Closes correctly
Make sure that each write is on its own line
arrow_forward
1. Write a program that opens an output file with the filename my_name.txt, writes your name to the file, then closes the file.
2. Write a program that opens the my_name.txt file that was created by the program in problem 1, reads your name from the file, displays the name on the screen, then closes the file. Write code that does the following: opens an output file with the filename number_list.
arrow_forward
Design an application that can be used to read DVD titles from a text file. You do not have to write to the file, only write code to read the titles of DVDs from a text file called DVD.txt
arrow_forward
Answer fast else downvote
arrow_forward
Write the program FileComparison that compares two files. Two files have been provided for you, Quote.txt and Quote.docx, both containing movie quotes and are located in the /root/sandbox/ directory
Note: you will not be able to see the Quote.docx file.
Next write the file comparison application that displays the sizes of the two files as well as the ratio of their sizes to each other. To discover a file’s size, you can create a System.IO.FileInfo object using statements such as the following, where FILE_NAME is a string that contains the name of the file, and size has been declared as an integer:
FileInfo fileInfo = new FileInfo(FILE_NAME); size = fileInfo.Length;
Your program's output should look like the following:
The size of the Word file is FILE_SIZE and the size of the Notepad file is FILE_SIZE The Notepad file is x% of the size of the Word file
using System;
using static System.Console;
using System.IO;
class FileComparison
{
static void Main()
{
// Getting…
arrow_forward
Instructions
The history teacher at your school needs help in grading a True/False test. The students’ IDs and test answers are stored in a file. The first entry in the file contains answers to the test in the form:TFFTFFTTTTFFTFTFTFTTEvery other entry in the file is the student ID, followed by a blank, followed by the student’s responses. For example, the entry:ABC54301 TFTFTFTT TFTFTFFTTFTindicates that the student ID is ABC54301 and the answer to question 1 is True, the answer to question 2 is False, and so on. This student did not answer question 9. The exam has 20 questions, and the class has more than 150 students. Each correct answer is awarded two points, each wrong answer gets one point deducted, and no answer gets zero points.
Write a program in C++ that processes the test data. The output should be the student’s ID, followed by the answers, followed by the test score, followed by the test grade. Assume the following grade scale:90%–100%, A; 80%–89.99%, B; 70%–79.99%, C;…
arrow_forward
Instructions
The history teacher at your school needs help in grading a True/False test. The students’ IDs and test answers are stored in a file. The first entry in the file contains answers to the test in the form:TFFTFFTTTTFFTFTFTFTTEvery other entry in the file is the student ID, followed by a blank, followed by the student’s responses. For example, the entry:ABC54301 TFTFTFTT TFTFTFFTTFTindicates that the student ID is ABC54301 and the answer to question 1 is True, the answer to question 2 is False, and so on. This student did not answer question 9. The exam has 20 questions, and the class has more than 150 students. Each correct answer is awarded two points, each wrong answer gets one point deducted, and no answer gets zero points.
Write a program that processes the test data. The output should be the student’s ID, followed by the answers, followed by the test score, followed by the test grade. Assume the following grade scale:90%–100%, A; 80%–89.99%, B; 70%–79.99%, C; 60%–69.99%,…
arrow_forward
OWrite a program that reads data from a file containing integers that ends with -999.
Output the numbers that are divisible by 7
Output the numbers that are divisible by 11
Output the numbers that are not divisible by 7 or 11.
arrow_forward
Create a file in your project named employees.txt with the following data (you can simply create the file by hand rather than with Python code):
123 Bob Smith345 Anne Jones256 Carol Lee845 Steve Robert Anderson132 Jill Thompson
From your program's main function, give the user the following options: lookup a name based on ID number, lookup an ID number based on name, and quit the program.
OPTION 1: The user chooses to lookup a name based on ID number:
Use a try/except and ask the user to enter an integer.
If they don't enter an integer, print an error message.
If they do enter an integer, call a function named lookup_employee which takes the id as a parameter.
If an employee with the given id number is found, return the name.
Otherwise, return the string “Employee not found”
Back in main, print the return result.
OPTION 2: The user chooses to lookup an ID based on name:
Ask the user to enter the first and last name (don't ask for the middle name).
Call a function named…
arrow_forward
1. Random numbers
File: random_numbers.py
(Quick note: NEVER name your files the same as a Python module. If you call this file random.py it won't let you import the real random.)
(Another quick note: Don't let yourself get stuck on something for too long. If one part of one question starts taking you too long, just leave it, move on and come back to it later.)
Write a complete Python program that ask the user for quantity of random numbers and a maximum, then generates and store that many numbers between 0 and the maximum. When you've got this part working, add the following interesting additions:
minimum
maximum
random choice from existing list (Hint: if there are 7 numbers it will be a number at a random index 0-6)
the list reversed*
the list sorted*
Note*: Python's .reverse() and .sort() list methods do NOT return the reversed or sorted list... they modify the list in place.So, if you write something like:
print(numbers.sort())
You will not get what you expect. You'll sort the…
arrow_forward
Sample Output 2 Enter length of rectanglet: 2 Enter width of rectangle116 Enter length of rectangle: 2 Enter width of rectangle2:10 Area of rectangle is 12.00 Area of rectangle2 is 20.00 Area of rectangle could either be less than or equal to area of rectangle
arrow_forward
PYTHON!!!!!
A file concordance tracks the unique words in a file and their frequencies. Write a program that displays a concordance for a file. The program should output the unique words and their frequencies in alphabetical order. Variations are to track sequences of two words and their frequencies, or n words and their frequencies.
Below is an example file along with the program input and output:
example.txt
I AM SAM I AM SAM SAM I AM
Enter the input file name: example.txt
AM 3
I 3
SAM 3
The program should handle input files of varying length (lines).
Program produces correct output given input, test 1
Test Case:
Test program with example.txt
Input:
example.txt
Results:
AM 3
I 3
SAM 3
Program produces correct output given input, test 2
Test Case:
Test program with test file 2
Input:
test.txt
Results:
3/4 1
98 1
AND 2
GUARANTEED 1
INDEED 1
PERCENT 1
SUCCEED 1
WILL 2
YES 1
YOU 2
arrow_forward
python 3
arrow_forward
Create an inputfile named "inputFile.txt" (use "Note Pad") that contains the following data. Be sure that the last line of the file is empty (i.e., not even a space character). In other words, after entering the second line of data, press the <ENTER> key, save the file, then exit the editor.
5 10 6 923 7 13 40
Implement the following algorithm
Create & Open inputFileCreate & Open outputFileRead first line of data into variables a, b, c, dDOWHILE !EOF Create a unique formula that uses the variables a, b, c, & d. For example, value = (a*a) + b - c + d Output value into outputFile Read each line of data into variables a, b, c, dENDDOClose inputFileClose outputFile
Post the source code (embed the code in your response; DO NOT ATTACH THE C++ FILE).
arrow_forward
Python problem in jubyter
arrow_forward
Last lab, we modified the inventory program to write a file. This lab, we’ll modify it to read a file. First, take your program from the last lab. Add one more feature: at the end, just before you close the file, write the word “END” all by itself on a line. Run that program once to create a file of items with “END”. Now, copy that program to a new file cp lab7.cpp lab8.cpp so you can use it as a basis for this lab. Modify lab8 so instead of reading from cin and writing to a file, you read from your file and write to cout. The only tricky part is deciding when to stop reading. In lab7, you stopped reading when the user said they didn’t want to continue. For lab8, keep reading until you read “END” for the name of the produce. You can use “==” on strings, so if (product name == ”END”) will tell you that you can stop reading.
PHOTO OF WHAT IT SHOULD LOOK LIKE INCLUDED
My code for last lab:
#include <iostream>#include <string>#include <fstream>using namespace std;
int…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
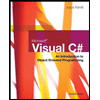
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
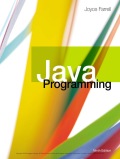
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
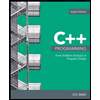
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Related Questions
- Add the ability to convert an entire message into Morse code, as well as the ability to handle a data file comprising numerous entries. Change your input validation code so that the user has an infinite number of opportunities to enter valid responses. Your program should run until the user selects the "quit" option from your menu. Add a menu option to provide the user the option of processing a data file. Add another way to exit the program. When your software starts, the menu should be the first item that appears. The menu format should be the same as in the Sample Output. Allow the user to make more menu selections until they select the option to exit. Before soliciting the user for a menu selection, always show the menu. Handle an incorrect menu option correctly. The user should have an infinite number of opportunities to enter a valid menu option. Validate the user's input for the number of words sent so that numbers less than one are not accepted. Allow the user an unlimited…arrow_forwardPython: I am trying to write a simple GUI based program with two input fields “Directory/filename” and “word” next to each other and one output text field below. Using recursion the program should go through all the files and folders searching for the entered word. If a match is found the path to the file should be printed in the text field + the content of line the word is found in . It should also print how many folders and files has been searched and how many times the word was found. Any ideas?arrow_forwardAdd comments for assignment p05Thanks! Run it with C, Linuxarrow_forward
- Allow the user the choice of displaying either a random line or a random word from a file they specify. Your menu should allow them to choose these options either from the number of the option or from the capitalized letter. For example, 1) display random Word 2) display random Line 3) Quit Should allow the user to select a random line display by either entering the number 2 or by entering a letter L (lower or upper case!).arrow_forwardPython The Springhill Amateur Golf Club has a tournament every weekend. The club president has asked you to write a Menu-Driven program which has the following functionality: 1. Allows for each player's name and golf score to be entered and saved in a text file named golf.txt. 2. Reads each player's name and golf score from the text file golf.txt and provides an average score. 3. The program will use one variable for the player's name and one variable for the score.arrow_forwardJavaarrow_forward
- OverviewYou should enter this task, ask the user for a word and then search for a file to see if that word is among the words in the file. Task Write a java programThis exercise comes with three text files named wordlist1.txt, wordlist2.txt and wordlist3.txt. These files contain a large amount of words in English. Each file contains more and more words. Each word in these files is on a separate line and the words contain only lowercase letters. Your task is to write a program that reads all these words from the file and checks if a keyword, which the user entered, is among the entered words. The program should also count the total number of words contained in the file used. The program should measure how long it takes to complete the search, ie how long it takes to read all the words and check if the keyword is among the words or not. The program should start by asking the user for the word to be searched for. Then the words in the file should be counted and compared if the keyword…arrow_forwardVB Small Project 18 – Numbers to a File Write a Visual Basic program that asks the user for a series of numbers (at least five numbers). Write each number to a text file. Write each number on a separate line in the file.arrow_forwardComputer Science Using Java language Write a procedure that generates a regular heightfield in data-files/model/heightfield.off with elevation given by the grayscale values of an image file. Use a file dialog to select the file, a number box to scale the xx and zz integer coordinates by, and a number box to set the scale that 1.0 corresponds to for height. Make a button labelled “Generate” that launches the generator. Display a message on screen while generating and then flush the G3D::ArticulatedModel cache, and reload the current scene on completion.arrow_forward
- Task – IV: Write a program that makes a copy of the file. Your program will ask the user for the source file and the new file names and then will read a line at a time from the input (source) file and prints it to the output (new file) file with a line number. You can create your own file "sample.txt" and add your favorite poem.arrow_forwardTask: Loading data from files This exercise will require you to load some information from files and use it in your program. scene.txt contains a description of a series of shapes and colours to draw. You need to write code to read in the file data and draw the requested shapes in the correct colour. Each line in scene.txt will contain one of the following starting keywords followed by some data: COLOUR followed by 3 values: R, G, B CIRCLE followed by 3 values: X, Y, RADIUS RECT Followed by 4 values: X, Y, W, H LINE Followed by 4 values: X1, Y1, X2, Y2 CIRCLES are defined from the center. RECT's are defined from the top left. All values are space separated, and you can assume all input is correct (no errors). Please solve this program to draw the scene. I can't upload the file scene.txt, so I decide to screenshot a file for you. Subject: Java Programmingarrow_forwardWrite a program that: Opens a file named foofoo.txt Write the phrase, Hi All! Then writes the odd numbers from 1 – 1000 Then writes Goodbye Closes correctly Make sure that each write is on its own linearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
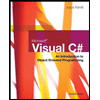
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
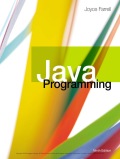
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
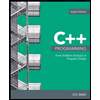
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning