COMSW4701_HW3
.pdf
keyboard_arrow_up
School
Columbia University *
*We aren’t endorsed by this school
Course
4701
Subject
Computer Science
Date
Dec 6, 2023
Type
Pages
5
Uploaded by SuperGoldfish3864
COMS W4701: Artificial Intelligence, Spring 2023
Homework 3
Instructions:
Compile all solutions, plots, and analysis for this assignment in a single PDF file
(typed, or handwritten
legibly
if absolutely necessary). Problems 2 and 3 will ask you to use and
minimally modify provided scripts; you will not be turning in your code for these problems. For
problem 4, implement your solutions directly in the provided Python file(s) and turn these in along
with the PDf file to Gradescope.
Please be mindful of the deadline, as late submissions are not
accepted, as well as our course policies on academic honesty.
Problem 1: Twenty-One (16 points)
Consider a one-player version of the game twenty-one as a Markov decision process. The objective
is to draw cards one at a time from an infinite deck of playing cards and acquire a card sum as
large as possible without going over 21.
For now we will have ten integer states in
{
12
, ...,
21
}
representing the card sum (sums smaller than 12 are trivially played). At each turn we can take
one of two actions from state
s
.
Stopping
yields a reward equal to
s
and immediately ends the
game.
Hitting
yields zero reward, and we will either transition to a state
s
′
with probability
1
13
where
s < s
′
≤
21, or immediately end the game (“bust”) with probability
s
−
8
13
.
1. Write down the Bellman optimality equations for the value
V
(
s
) of each state. While you can
do so by writing 10 separate equations, each equation form is similar enough that you can
write one compact expression for
V
(
s
) that applies to all of them (use summation notation).
2. We perform value iteration starting with
V
0
(
s
) = 0 for all states. What are the values
V
1
(
s
)
in the next iteration? Have we found the optimal values
V
∗
?
3. Now consider taking a policy iteration approach. Suppose that we have an initial policy
π
1
,
which is to
hit
in every state. What are the associated values
V
π
1
(
s
)? You should be able to
reason this out without having to solve a linear system or write an iterative program.
4. Perform the next step of policy iteration to find
π
2
. Have we found the optimal policy
π
∗
?
Problem 2: Twenty-One Redux (18 points)
We will now modify the twenty-one MDP to incorporate a special rule regarding the ace card. If a
player’s hand contains an ace, it can add either 1 or 11 to the card sum, provided that the total sum
does not exceed 21. If the latter case is possible, we say that the hand contains a
usable ace
. The
number of states is now doubled to twenty, and each can be represented by a tuple
s
= (
sum, ace
),
where
sum
is the card sum as before and
ace
can be either
True
or
False
.
Suppose we take an action from state
s
= (
sum, ace
). As before,
stopping
returns reward equal to
sum
and immediately ends the game. The possible transitions for
hitting
are as follows, all with
zero reward:
1
•
Transition to state
s
′
= (
sum
′
, ace
), where
sum < sum
′
≤
21, with probability
1
13
.
•
If
ace
is
True
, transition to state
s
′
= (
sum
′
, False
), where 12
≤
sum
′
< sum
, with proba-
bility
1
13
, or state
s
′
= (
sum, False
) with probability
4
13
.
•
Else if
ace
is
False
, immediately end the game with probability
sum
−
8
13
.
As an example, let
s
= (16
, True
).
Our hand has a usable ace (ace value is 11) and card sum
16.
We then choose to
hit
.
We may draw a value between 1 and 5, which brings us to states
(17
, True
)
, ...,
(21
, True
) with probability
1
13
each. We may draw a value between 6 and 9, which
brings the card sum over 21, so we “remove” usability of the ace and decrease the excessive card
sum by 10. This brings us to states (12
, False
)
, ...,
(15
, False
) with probability
1
13
each. Finally,
we may draw a value of 10, which brings our state to (16
, False
) with probability
4
13
.
The provided
twentyone.ipynb
script initializes an array storing the 20 state values. The first 10
are the
ace
=
False
states, and the last 10 are the
ace
=
True
states.
Given a discount factor
gamma
, the
value
iteration()
method can be used to find the optimal values for this problem.
The code outside the main loop assigns the transition probabilities for
hitting
in a 20
×
20 array,
allowing us to compute the Bellman update using a simple matrix-vector product.
1. Run three instances of value iteration with the following values of discount factor:
γ
= 1,
γ
= 0
.
9, and
γ
= 0
.
5. For each instance, use
matplotlib
to produce a separate scatter plot
with the x-axis ranging from 12 to 21. Use two different markers to plot the values of states
with and without a usable ace, and show a legend as well. Copy these plots to your PDF.
2. What are the optimal values and actions of the states with no usable ace? Explain why they
either differ or remain the same (depending on your observations) from those of the previous
problem when aces could only have a value of 1.
3. For
γ
= 1, what are the optimal actions in each state with a usable ace?
Explain the
differences in strategy compared to the corresponding states without a usable ace.
4. Explain how and why the optimal strategies in usable ace states change as we decrease
γ
.
Problem 3: Bernoulli Bandits (18 points)
You will be simulating a
K
-armed
Bernoulli
bandit in
bandits.ipynb
; each arm gives either a
reward of 1 with a set probability
p
or 0 with probability 1
−
p
.
means
is a list of the
p
probabilities
for each arm.
strat
and
param
describe the strategy used to play this bandit (
ε
-greedy or UCB)
along with the parameter value (
ε
or
α
), and the bandit is simulated
M
times for
T
timesteps each.
The returned quantities are the average regret and average frequency that the best (highest mean)
arm is chosen at each timestep.
execute()
simulates the bandit given the same arguments above, except that
params
is expected
to be a list, so that we can compare the simulation results for different values of
ε
or
α
.
The
returned values from
bernoulli
bandit()
are plotted vs time on separate plots (note that regret
is plotted cumulatively). The x-axis is logarithmic, so that the trends are better visualized over
time.
Thus, logarithmic growth will appear to be linear on these plots, while linear growth will
appear to be exponential.
2
For each part of this problem, you will only need to set the arguments as instructed and then call
execute()
. You will then copy these plots into your submission and provide analysis. Make sure
to have a good understanding for what each plot is showing you before responding to the questions.
1. Let’s first try an “easier” problem: a 2-armed bandit with means 0
.
3 and 0
.
7, simulated for
T
= 10000 timesteps and averaged over
M
= 100 simulations. Use
ε
-greedy with
ε
values
0
.
1
,
0
.
2
,
0
.
3, and then use UCB with
α
values 0
.
1
,
0
.
2
,
0
.
3 (you can ignore any divide by zero
warnings). Show the two sets of plots and briefly answer the following questions.
•
For
ε
-greedy, which parameter value does better on the two metrics over the long term?
Why might your answer change if we look at a shorter time period (e.g.,
t <
100)?
•
How does the value of
α
affect whether UCB does better or worse than
ε
-greedy? How
does
α
affect the order of growth of regret?
2. Now let’s consider a “harder” 2-armed bandit with means 0
.
5 and 0
.
55. Perform the same
simulations as in the previous part with all other values unchanged.
Show the two sets of
plots and briefly answer the following questions.
•
Compare
ε
-greedy’s performance on this problem vs the previous one on the two metrics.
For this problem, do we need greater or fewer (or about the same) number of trials to
maximize the frequency of playing the best arm?
•
Compare the performance of
ε
-greedy vs UCB on this problem on the two metrics.
Which generally seems to be less sensitive to varying parameter values and why?
3. For the last experiment, simulate a 4-armed bandit with means 0
.
2
,
0
.
4
,
0
.
6
,
0
.
8, keeping all
other values the same.
Do so using both
ε
-greedy and UCB, and show both sets of plots.
Briefly answer the following questions.
•
Among the three bandit problems, explain why
ε
-greedy performs the worst on this one
in terms of regret, particularly for larger values of
ε
.
•
How does UCB compare to
ε
-greedy here when
α
is properly chosen (i.e., look at the
best performance of UCB)? Explain any differences that you observe.
Problem 4: Frozen Lake (48 points)
Frozen Lake is a classical test example for reinforcement learning algorithms included in the Gym
API. It is a grid world problem in which most states (“Frozen”) yield zero reward, the “Goal” is
a terminal state that yields
+1
reward, and all other terminal states (“Holes”) yield zero reward.
The agent may move in one of the four cardinal directions from any Frozen state, but transitions
may be stochastic and unknown by the agent, so it will employ reinforcement learning.
First set up the poetry virtual environment as in past homeworks. It is essential that you do so
to avoid importing and setting up the Gym libraries yourself. You will be implementing certain
functions in the
QLearningAgent
class in
agent.py
. To run your code and simulate the environ-
ment, you can modify parameters in and run
main.py
. The agent will then train for 10000 episodes
and evaluate its learned policy on 100 episodes. If the
render
parameter is set to
True
, a small
graphic will pop up showing the agent’s trajectory during each evaluation episode.
In addition,
the evaluation success rate, learned state values (remember that
V
(
s
) = max
a
Q
(
s, a
)), true state
values (computed using value iteration), and value error norm will be printed on the console.
3
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Please use python and python file i/o to solve the problem. Create an input file input4_1.txt as shown below, solve the problem and print the output to output4_1.txt file as shown in the question.
arrow_forward
Please use python and python file i/o to solve the problem. Create an input file input4_1.txt as shown below, solve the problem and print the output to output4_1.txt file as shown in the question.
arrow_forward
just do the first step. and the program should be in python and includ as many different functions as possible .
arrow_forward
By use python language
arrow_forward
Note: Please do this in Python 3
The format of the data file is in CSV (excel).
arrow_forward
The example of the two CSV files is attached below. One of the file is the crime database and the other one is the suspect database.
Specification
Your task is to write a python program that will take three CSV file names on the command line. The first CSV file contains STR counts for DNAs found in a list of crime scenes; the second CSV file contains a list of suspect's names and their DNA sequences; the third CSV file name is the output file where you write a CSV file that maps each suspect's name to the list of Crimes that have DNAs matching the suspect.
Your program will take three file names on the command line:
The first command line argument is a file name for a crime database file in csv file format. The header row would look like this:CrimeID,STR1,STR2,STR3,...Where each STRi is a short DNA sequence that is composed of DNA bases A/C/G/T. And each row will comprise of a CrimeID of the form CIDXXXXX, an integer count for each of the STRs.
The second command line argument is a…
arrow_forward
Code should be in Python.
Given a text file containing the availability of food items, write a program that reads the information from the text file and outputs the available food items. The program first reads the name of the text file from the user. The program then reads the text file, stores the information into four separate lists, and outputs the available food items in the following format: name (category) -- description
Assume the text file contains the category, name, description, and availability of at least one food item, separated by a tab character ('\t').
(Examples in image)
arrow_forward
Java only
Design, implement and test a Java class that processes a series of triangles. For this assignment, triangle data will be read from an input file and the program’s output will be written to another file. The output file will also include a summary of the data processed. You must use at least one dialog box in this program.
The data for each triangle will be on a separate line of the input file, with the input data echoed and the results referred to by the line number (see example).
On initialization, the program will prompt the user for both an input and an output file. If a non-existent input file is specified, the appropriate exception must be handled, resulting in an error message. For the exception case, re-prompt the user for the correct input file. Once the I/O file is specified, the program will read in and process all the entries in the file. The output of the program will be written to the specified output file and echoed to the console.
The program will…
arrow_forward
Use Python 3 for this question.
Do the sentiment analysis based on a text file which contains numerous tweets in its raw text format. All tweets collected in this file contains a key word specified by the instructor. The key word being used is “Trump”.
You can use the raw tweets file provided by the following link: tinyurl.com/46ntt3a5. You will need to convert this to a .txt file for this assignment.
Your raw tweets file size at least needs to be 350K.
After you have the raw tweets file ready, you need to perform the following tasks in a Jupyter notebook file. First you need to clean up the tweet file content to the best you can. For each word that is not a “stop word” (and, the, a, is, as, …), assign a value +1 for positive sentiment or a value -1 for negative sentiment. A list of “positive” words will be provided to you. You can easily find a list of “stop words”, and a list of “negative” words online. For the words that is not in positive/negative/stop words, count as ‘others’.…
arrow_forward
Using Pandas library in python - Calculate student grades project
Pandas is a data analysis library built in Python. Pandas can be used in a Python script, a Jupyter Notebook, or even as part of a web application. In this pandas project, you’re going to create a Python script that loads grade data of 5 to 10 students (a .csv file) and calculates their letter grades in the course. The CSV file contains 5 column students' names, score in-class participation (5% of final grade), score in assignments (20% of final grade), score in discussions (20% of final grade), score in the mid term (20% of final grade), score in final (25% of final grade). Create the .csv file as part of the project submission
Program Output
This will happen when the program runs
Enter the CSV file
Student 1 named Brian Tomas has a letter grade of B+
Student 2 named Tom Blank has a letter grade of C
Student 3 named Margo True has a letter grade of A
Student 4 named David Atkin has a letter grade of B+
Student 5 named…
arrow_forward
Must show it in Python:
Please show step by step with comments.
Please show it in simplest form.
Input and Output must match with the Question
Please go through the Question very carefully.
arrow_forward
Instructions:
I need Python help.
The problem I am trying to figure out so that when we work a similar problem in class I can do my assignment. Tonights assignment is a participation thing, Friday I need to do a similar assignment in class for a grade.
Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements:
Each row contains the title, rating, and all showtimes of a unique movie.
A space is placed before and after each vertical separator ('|') in each row.
Column 1 displays the movie titles and is left justified with a minimum of 44 characters.
If the movie title has more than 44…
arrow_forward
Python - Next Birthdate
In this task, we will write a program that reads birthdate data from a given CSV file, and given the current date, determines which person's birthday will be celebrated next.
Create a function with the following signature:
nextBirthdate(filename, date)
filename: parameter, which represents the CSV filename.
date: parameter, which represents the current date.
The function should open the CSV file, read the birthdate data, and determine which person's birthday will be celebrated next, given the current date. The name of the person should be returned. In other words, given a date, find the person whose birthday is next.
Sample Run
birthdates.csv
Draven Brock, 01/21/1952
Easton Mclean, 09/02/1954
Destiny Pacheco, 10/10/1958
Ariella Wood, 12/20/1961
Keely Sanders, 08/03/1985
Bryan Sloan,04/06/1986
Shannon Brewer, 05/11/1986
Julianne Farrell,01/29/2000
Makhi Weeks, 03/20/2000
Lucian Fields, 08/02/2018
Function Call
nextBirthdate("birthdates.csv", "01/01/2022")
Output…
arrow_forward
Please use python and python file i/o to solve the problem. Create an input file input5_1.txt as shown below, solve the problem and print the output to output5_1.txt file as shown in the question.
arrow_forward
Design a python program with comments
arrow_forward
I know you are using Al tools to generate answer but I don't want these answers. If I see Al
or plagiarism in my response I'll definitely reduce rating and will report for sure. So be
careful. I'll take serious action.
Scenario involving a list of books stored in a file named "Books.txt." Create a Python program
that performs the following tasks:
Output all books in your list.
Output books published between the years 2015 and 2020.
Calculate the average rating of all books.
Create a histogram of the number of books in each genre. Each element in the histogram
should represent the count of books in a specific genre.
Take all the books in "Books.txt" and store them in a Python set.
Output all unique authors of the books.
Output the total number of entries in your set.
Books.txt:
"The Silent Observer," 2018, Mystery, Emily Williams
"Beyond the Horizon," 2016, Fantasy, David Thompson
"Code Breakers," 2019, Thriller, Sarah Miller
"Infinite Realms," 2017, Sci-Fi, Mark Anderson
"Whispers of…
arrow_forward
Create a program in C language for reading and writing student records. Submit all necessary source codes and files to run this
program (it is recommended to compress all files and submit a single file).
First create text file "student_records.txt". The file should look like:
Name
Surname
Quiz 1
Quiz 2
Quiz 3
Ada
Lovelace
93
88
97
Babbage
Turing
Нopper
Charles
26
80
70
Alan
74
85
92
Grace
99
87
100
a. Read the file contents and load them into an array of Record structs.
b. The record struct has the member variables:
o char array name
o char array surname
o int quiz_1
o int quiz_2
o int quiz_3
c. Write a function that calculates a students quiz average. The function takes a Record struct and returns a double.
d. Create two new records for students inside your main program.
e. Create a new text file with the new records to a a file called "student_records_new.txt"
arrow_forward
Code this in C.
Project name : SurveyFilenames: survey.h, survey.c, main.c
Survey Data AnalysisComputers are commonly used to compile and analyze the results of surveys andopinion polls. Each response is a number from 1 to 9. The program computes for the mean,median and mode of the values. Start with a minimum of 10 responses. If there would be moreresponses, increase the size.Mean – arithmetic averageMedian – middle valueMode – value that occurs most frequentlyThe following are the declarations that will be used by the program:int SIZE 10typedef int* Statistician;void add(Statistician answer, int *count, int *SIZE, int item);- Doubles the size of answer when it is full- Data should be sorted after every insertion.float mean(Statistician answer, int count);float median(Statistician answer, int count);int max(Statistician answer, int count);int min(Statistician answer, int count);int range(Statistician answer, int count);void mode(int freq[], int *freqsize, Statistician answer, int…
arrow_forward
In this and the following two exercises (8.10 and 8.11), you will carry out some simple processing on data in a file. Each line of the file has two integer values separated by a comma; there are no spaces. The first value is the weight of an individual (in pounds) and the second value is the height of the individual (in inches). The aim of the exercise is to construct a program that will compute the average weight and height.
The name of the file is "data.txt". The data file looks as follows:
arrow_forward
Python Problem Description:
Kevin is a freelance video producer who makes TV commercials for local businesses. When he makes a commercial, he usually films several short videos. Later, he puts these short videos together to make the final commercial. He has asked you to write the following two programs.
A program that allows him to enter the running time (in seconds) of each short video in a project. The running times are saved to a file.
A successful test run for this program would be as shown below:
A program that reads the contents of the file, displays the running times, and then displays the total running time of all the segments. A successful test run for this program would be as shown below:
arrow_forward
Use loop to solve this python program and use Error handling:IOError (File not found)
arrow_forward
Program in Python: You are responsible for computing the votes of the election. We have 5 candidates: Abel, Barbara, Carl, Denise and Eugenie. Make a program / script that reads from a file the total votes that each of them had in that order (first line from Abel, second line from Barbara, third line from Carl, fourth line from Denise and fifth line from Eugenie). (voters only have the option to vote for one of the 5, with no blank or null votes).
arrow_forward
Assistance is needed for Python please
The objective is to create a code in Python that can extract the columns highlighted in blue (Column T and AB3) and output them to a .txt file. The code should be able to generate the text file with the columns that are highlighted in blue and store them on a separate folder. Below shows how the Test.xlsm file looks like as well as how the text file should look like when the code extracts and outputs it.
Google drive to access Test.xlsm file: https://drive.google.com/drive/folders/16utzb5_h7yMCN8_13E_JqasfcpykZOYr?usp=sharing
What my code outputs is shown in the picture (O1.png)
What I would like for my code to output is the columns next to each other (Right Example.png)
The current code is able to output Columns T and AB3 but I am not able to output them next to each other as shown above. We would also like for the code to be capable of storing the text file to a separate folder. Below is the code I have been working on.
#package to read xlsm…
arrow_forward
Please use python and python file i/o to solve the problem. Create an input file input3_1.txt as shown below, solve the problem and print the output to output3_1.txt file as shown in the question.
Input:
The first line contains two integers N and M (1
arrow_forward
I need Python help. The problem I am trying to figure out so that when we work a similar problem in class I can do my assignment. Tonights assignment is a participation thing, Friday I need to do a similar assignment in class for a grade.
Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements:
Each row contains the title, rating, and all showtimes of a unique movie.
A space is placed before and after each vertical separator ('|') in each row.
Column 1 displays the movie titles and is left justified with a minimum of 44 characters.
If the movie title has more than 44 characters, output the…
arrow_forward
Complete the following incomplete program (begin by copy-pasting that into a new program file). The completed program will provide a user interface to draw as many circles as the user wants only by clicking in the window. After getting the number of circles (n) to draw as input from the user through the provided interface, the program should iterate n times. In each iteration it should wait for the user to click twice and draw a circle with center at the point where the user clicked first and with radius calculated as its distance from the second point where the user clicked. It should then fill the circle with a random color.
Hints:
Distance between two points with coordinates x1 & y1 and x2 & y2 and can be calculated as sqrt((x1-x2)2 + (y1-y2)2 ).
To generate a random color, use randint(0,255) from the random libray to generate a random number between 0 and 255 (both inclusive). Use it three times to get the r, g and b values to generate a random color
from graphics import…
arrow_forward
Draws their own one storey house (american kitchen+bathroom) with an area of 50 to 100 square meters by constructing the structural beams and columns in sap2000. In the SketchUp program, the walls and some necessary staffs in the kitchen, and bathroom are drawn. At the end, a BIM model will be created by combining the tekla trimble program and the files drawn in these two different programs
****(please write all step, how did you do on the sap 2000,sketchup and combined on the tekla trimble)****
arrow_forward
Task 2
Write a python program to find the longest word in a file. Then print the word.
Input: [in a file]
I love Python Programming
It is very easy to understand the code
It can code very hard problems easily in python code
Python programming is the best programming language
Output:
Programming
Hint(1):
You can use split function to separate the words into a list.
Hint(2):
Use function readlines()
arrow_forward
The instruction is in the attached photo. Use python
file(input.txt)
LadnoonefeelsreadyNoonefeelshedeservesitAndyouknowwhyBecausenoonedoesItisgracepureandsimpleWeareinherentlyunworthysimplybecausewearehumanandallhumanbeingsayeandelvesanddwarvesandalltheotherracesareflawedButtheLightlovesusanywayItlovesusforwhatwesometimescanrisetoinraremomentsItlovesusforwhatwecandotohelpothersAnditlovesusbecausewecanhelpitshareitsmessagebystrivingdailytobeworthyeventhoughweunderstandthatwecannotevertrulybecomesoSostandtheretodayasIdidfeelingthatyoucannotpossiblydeserveitoreverbeworthyandknowthatyouareinthesameplaceeverysinglepaladinhaseverstood
arrow_forward
A company stores payment information for their employee working on a project and paid on a
project basis, in a text file whose name is entered through keyboard (see a sample input file
below). The first line of the file indicates the number of employees and each subsequent line
corresponds to information related to an employce payment (employee name, number of hours
worked and his/her hourly wage). Write a python program that reads such a file and displays
the employees' names, their hours worked, and their salaries. Finally, it displays the total
Your
program should consider the following erroncous cases:
рaуmеnt.
• The file does not open/exist
The format of the data in the file is incorrect (e.g. hours = "1-" or salary = "unkown")
in which case a RuntimeError exception should be raised and the processing should
continue
Enter input filename: employees.txt
Employee
Hours
Salary
Badria Al-Harthi: 11
Youcef Al-Busaidi: 19 720
Salim Al-Hajri:
Majid Al-Sinani:
Amer Al-Shidani: 10 unkown.…
arrow_forward
Use C language only.
Create a program that will ask for
Enter number of students: (for this example 2)
Enter student 1's name:
Enter student 1's age:
Enter student 2's name: E
nter student 2's age:
At the end, the program shall display two class lists: one with arranged name (ascending order) and the other one arranged according to their age (ascending order).
The two lists should contain the complete details of the students as follows: Sorted by name:
1. name, age
2. name, age
Sorted by age:
1. name, age
2. name, age
If the number of students is less than one, the program will display "no students to display."
The letter case (uppercase or lowercase) shall have no bearing in the arrangement of names. Please use the "fgets()" in getting the name.
arrow_forward
Answer in Python please
arrow_forward
PYTHON PROGRAMMING
Files are here: http://www.cse.msu.edu/~cse231/Online/Projects/Project05/
This exercise is about data manipulation.
In this project, we will process agricultural data, namely the adoption of different Genetically Modified (GM) crops in the U.S. The data was collected over the years 2000-2016.
In this project, we are interested in how the adoption of different GM food and non-food crops has been proceeding in different states. We are going to determine the minimum and maximum adoption by state and the years when the minimum and maximum occurred.
Assignment Specifications:
The data files The data files that you use are yearly state-wise percentage plantings for each type of crop:
• alltablesGEcrops.csv: the actual data from the USDA.
• data1.csv: data modified to test this project.
• data2.csv: data modified to test this project, but you do not get to see it
Input: This is real data so it is messy and you have to deal with that. Here are the constraints.…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
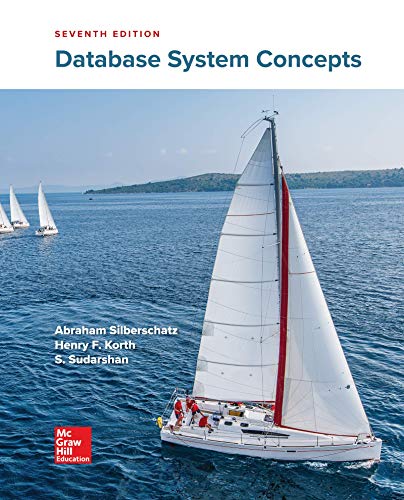
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
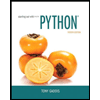
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
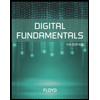
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
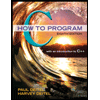
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
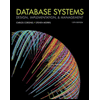
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
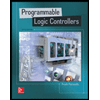
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Please use python and python file i/o to solve the problem. Create an input file input4_1.txt as shown below, solve the problem and print the output to output4_1.txt file as shown in the question.arrow_forwardPlease use python and python file i/o to solve the problem. Create an input file input4_1.txt as shown below, solve the problem and print the output to output4_1.txt file as shown in the question.arrow_forwardjust do the first step. and the program should be in python and includ as many different functions as possible .arrow_forward
- By use python languagearrow_forwardNote: Please do this in Python 3 The format of the data file is in CSV (excel).arrow_forwardThe example of the two CSV files is attached below. One of the file is the crime database and the other one is the suspect database. Specification Your task is to write a python program that will take three CSV file names on the command line. The first CSV file contains STR counts for DNAs found in a list of crime scenes; the second CSV file contains a list of suspect's names and their DNA sequences; the third CSV file name is the output file where you write a CSV file that maps each suspect's name to the list of Crimes that have DNAs matching the suspect. Your program will take three file names on the command line: The first command line argument is a file name for a crime database file in csv file format. The header row would look like this:CrimeID,STR1,STR2,STR3,...Where each STRi is a short DNA sequence that is composed of DNA bases A/C/G/T. And each row will comprise of a CrimeID of the form CIDXXXXX, an integer count for each of the STRs. The second command line argument is a…arrow_forward
- Code should be in Python. Given a text file containing the availability of food items, write a program that reads the information from the text file and outputs the available food items. The program first reads the name of the text file from the user. The program then reads the text file, stores the information into four separate lists, and outputs the available food items in the following format: name (category) -- description Assume the text file contains the category, name, description, and availability of at least one food item, separated by a tab character ('\t'). (Examples in image)arrow_forwardJava only Design, implement and test a Java class that processes a series of triangles. For this assignment, triangle data will be read from an input file and the program’s output will be written to another file. The output file will also include a summary of the data processed. You must use at least one dialog box in this program. The data for each triangle will be on a separate line of the input file, with the input data echoed and the results referred to by the line number (see example). On initialization, the program will prompt the user for both an input and an output file. If a non-existent input file is specified, the appropriate exception must be handled, resulting in an error message. For the exception case, re-prompt the user for the correct input file. Once the I/O file is specified, the program will read in and process all the entries in the file. The output of the program will be written to the specified output file and echoed to the console. The program will…arrow_forwardUse Python 3 for this question. Do the sentiment analysis based on a text file which contains numerous tweets in its raw text format. All tweets collected in this file contains a key word specified by the instructor. The key word being used is “Trump”. You can use the raw tweets file provided by the following link: tinyurl.com/46ntt3a5. You will need to convert this to a .txt file for this assignment. Your raw tweets file size at least needs to be 350K. After you have the raw tweets file ready, you need to perform the following tasks in a Jupyter notebook file. First you need to clean up the tweet file content to the best you can. For each word that is not a “stop word” (and, the, a, is, as, …), assign a value +1 for positive sentiment or a value -1 for negative sentiment. A list of “positive” words will be provided to you. You can easily find a list of “stop words”, and a list of “negative” words online. For the words that is not in positive/negative/stop words, count as ‘others’.…arrow_forward
- Using Pandas library in python - Calculate student grades project Pandas is a data analysis library built in Python. Pandas can be used in a Python script, a Jupyter Notebook, or even as part of a web application. In this pandas project, you’re going to create a Python script that loads grade data of 5 to 10 students (a .csv file) and calculates their letter grades in the course. The CSV file contains 5 column students' names, score in-class participation (5% of final grade), score in assignments (20% of final grade), score in discussions (20% of final grade), score in the mid term (20% of final grade), score in final (25% of final grade). Create the .csv file as part of the project submission Program Output This will happen when the program runs Enter the CSV file Student 1 named Brian Tomas has a letter grade of B+ Student 2 named Tom Blank has a letter grade of C Student 3 named Margo True has a letter grade of A Student 4 named David Atkin has a letter grade of B+ Student 5 named…arrow_forwardMust show it in Python: Please show step by step with comments. Please show it in simplest form. Input and Output must match with the Question Please go through the Question very carefully.arrow_forwardInstructions: I need Python help. The problem I am trying to figure out so that when we work a similar problem in class I can do my assignment. Tonights assignment is a participation thing, Friday I need to do a similar assignment in class for a grade. Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
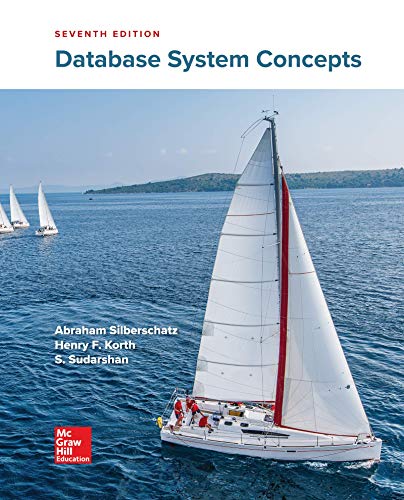
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
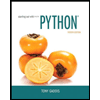
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
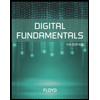
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
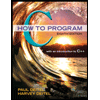
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
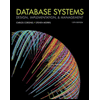
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
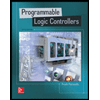
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education