hw04
.py
keyboard_arrow_up
School
University of California, Berkeley *
*We aren’t endorsed by this school
Course
61A
Subject
Computer Science
Date
Apr 3, 2024
Type
py
Pages
4
Uploaded by rayleehe
HW_SOURCE_FILE = 'hw04.py'
###############
# Questions #
###############
def intersection(st, ave):
"""Represent an intersection using the Cantor pairing function."""
return (st+ave)*(st+ave+1)//2 + ave
def street(inter):
return w(inter) - avenue(inter)
def avenue(inter):
return inter - (w(inter) ** 2 + w(inter)) // 2
w = lambda z: int(((8*z+1)**0.5-1)/2)
def taxicab(a, b):
"""Return the taxicab distance between two intersections.
>>> times_square = intersection(46, 7)
>>> ess_a_bagel = intersection(51, 3)
>>> taxicab(times_square, ess_a_bagel)
9
>>> taxicab(ess_a_bagel, times_square)
9
"""
return (abs(street(a)-street(b))) + (abs(avenue(a)-avenue(b)))
def squares(s):
"""Returns a new list containing square roots of the elements of the
original list that are perfect squares.
>>> seq = [8, 49, 8, 9, 2, 1, 100, 102]
>>> squares(seq)
[7, 3, 1, 10]
>>> seq = [500, 30]
>>> squares(seq)
[]
"""
mylist = []
for x in s:
root = (x ** (1/2))
if x % root == 0:
mylist.append(round(root))
return mylist
def g(n):
"""Return the value of G(n), computed recursively.
>>> g(1)
1
>>> g(2)
2
>>> g(3)
3
>>> g(4)
10
>>> g(5)
22
>>> from construct_check import check
>>> check(HW_SOURCE_FILE, 'g', ['While', 'For'])
True
"""
sum = 0
if n == 0:
return 0
elif n <= 3:
return n
else:
sum += g(n - 1) + 2 * g(n - 2) + 3 * g(n - 3)
return sum
def g_iter(n):
"""Return the value of G(n), computed iteratively.
>>> g_iter(1)
1
>>> g_iter(2)
2
>>> g_iter(3)
3
>>> g_iter(4)
10
>>> g_iter(5)
22
>>> from construct_check import check
>>> check(HW_SOURCE_FILE, 'g_iter', ['Recursion'])
True
"""
sum = 0
i, x, y, z = 4, 3, 2, 1
if n <= 3:
return n
else:
while i <= n:
sum = x + 2 * y + 3 * z
x, y, z = sum, x, y
i += 1
return sum
def count_change(amount):
"""Return the number of ways to make change for amount.
>>> count_change(7)
6
>>> count_change(10)
14
>>> count_change(20)
60
>>> count_change(100)
9828
>>> from construct_check import check
>>> check(HW_SOURCE_FILE, 'count_change', ['While', 'For'])
True
"""
m = 2 ** amount
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
#Data is given in the order: Serial Number | Item Name | Quantity | Priceprice_list = [["01","Keyboard",4,200.0],["02","Mouse",4,150.0],["03","Printer",2,300.0]]
#Write Code to increase the price of all the items by 50 taka
#Code1
#Write code to add a new item to the list using user input
#Code2
print()#Print the list using nested loops
#Code3
#Sample output given below:
Enter Serial: 05Enter Item Name: MonitorEnter Quantity: 200Enter Price: 50
01Keyboard4250.0-----------------02Mouse4200.0-----------------03Printer2350.0-----------------05Monitor20050-----------------
arrow_forward
Plse use c# programming language
arrow_forward
Looking for this in Python, please.
Shopping list assignment (Parallel Array)
Create three global variables with the proper data types:
sub_totaltotal_pricesales_tax
Sales tax is 9.5%
Seven items minimum
Create a shopping list (List, Tuple or Dictionary) of your choice (Items in list)
Create a price list (Price for each item in shopping list)
Create and print your parallel array (match each item to its price)
Print the list with its index numbers
Select one or more items from the shopping list using the index number
Print your selected items with price
Print sub total (base price of item(s) before tax)
Print sales tax amount (before adding it into total price)
Calculate and print total price with tax included. (this is what you would pay walking out the door)
Round to two decimal points
arrow_forward
The above screen is used to browse the products table it uses the ProductDAO.java file, it calls the getProductByCriteria() function which should accept a parameter, this parameter should be the criteria that would be built from the two combo boxes and the text box, and queries the products table.The getProductByCriteria() function should return a list of Product objects that matches the criteria specified.
Modify the function so that it accepts a string paramater consisting of the fields from the combo boxes and a price number from the text box separated by commas and use this string to modify the query in the function to return the desired result set.
public List<Product> getProductByCriteria() //MAKE MODIFICATIONS TO THIS FUNCTION SO IT CAN TAKE PARAMETER(S)
{
if (openConnection())
{
try{
List<Product> items = new ArrayList <> ();
Product temp = null;
String…
arrow_forward
Dec2Hex function :
def decimal_to_hex(number): hex_map = {0: '0', 1: '1', 2: '2', 3: '3', 4: '4', 5: '5', 6: '6', 7: '7', 8: '8', 9: '9', 10: 'A', 11: 'B', 12: 'C', 13: 'D', 14: 'E', 15: 'F'} hex_digits = [] while number > 0: hex_digits.append(hex_map[number % 16]) number //= 16 # Reverse the order of the hex digits and join them to form the final hex string hex_string = ''.join(reversed(hex_digits)) return f'0x{hex_string}' if hex_string else '0x0'
Perform a Profile of your Dec2Hex function.
Write a function that takes a timedate object as a parameter andcalculates the number of years from NOW to the time in the timedateobject.
Add unit-testing code to your Dec2Hex exercise, and then perform aUnit test of the updated Dec2Hex code.
arrow_forward
Use Python Programming - restaurant reservation program. The application offers different reservation rate for adults and children. (see sample below)
RESTAURANT RESERVATION SYSTEMSystem Menua. View all Reservationsb. Make Reservationc. Delete Reservationd. Generate Reporte. Exit
2. When the VIEW RESERVATIONS is clicked, this will display all the reservations made:
# Date Time Name Adults Children 1 Nov 10, 2020 10:00 am John Doe 1 1 2 Nov 25, 202011:00 am Michelle Franks 2 1 3 Dec 10, 2020 9:00 am Ella Flich 1 1 4 Dec 21, 2020 12:00 pmDylan Cloze 2 1
3. If the user selects MAKE RESERVATION, the user needs to input the following:
a. Name (String)b. Date (String)c. Time (String)d. No of Adults (Integer)e. No of Children (Integer)
Note: Adult is 500 per head and Kids is 300 per head reservation, save the data in a text file.
4. If DELETE RESERVATION is selected, the user needs to input the reservation number to remove the existing reservation.
5. If the GENERATE REPORT is clicked,…
arrow_forward
Use Python Programming - restaurant reservation program. The application offers different reservation rate for adults and children. (see sample below)
RESTAURANT RESERVATION SYSTEMSystem Menua. View all Reservationsb. Make Reservationc. Delete Reservationd. Generate Reporte. Exit
2. When the VIEW RESERVATIONS is clicked, this will display all the reservations made:
# Date Time Name Adults Children 1 Nov 10, 2020 10:00 am John Doe 1 1 2 Nov 25, 202011:00 am Michelle Franks 2 1 3 Dec 10, 2020 9:00 am Ella Flich 1 1 4 Dec 21, 2020 12:00 pmDylan Cloze 2 1
3. If the user selects MAKE RESERVATION, the user needs to input the following:
a. Name (String)b. Date (String)c. Time (String)d. No of Adults (Integer)e. No of Children (Integer)
Note: Adult is 500 per head and Kids is 300 per head reservation, save the data in a text file.
4. If DELETE RESERVATION is selected, the user needs to input the reservation number to remove the existing reservation.
5. If the GENERATE REPORT is clicked,…
arrow_forward
The parameter represents converted station data for a single station.
is_app_only
The function should return True if and only if the given station requires the use of an app
because it does NOT have a kiosk. Recall that a station requires the use of an app if and only
("Station") -> bool
if the string that the constant NO_KIOSK refers to is part of its station name.
arrow_forward
switch_player(): we will allow the players to choose their own names, but for our purposes, it is easier to think of them as number (0 and 1, for indexing purposes). We will display their names to them on the screen, but in the background, we will keep track of them based on their number. This function should take 1 argument, an integer (either 0 or 1) representing the player and should return the opposite integer (1 if 0 is entered and 0 if 1 is entered). This is a simple function, but it will make our code cleaner later. Use your constants!
Using Thonny
arrow_forward
PYTHON CODING
This function takes a list of points and then returns a new list of points, which starts with the first point that was given in the list, and then followed by points closest to the start point.
Here is the function that needs to be created:
def solution_path(points) : # insert code here return path
Please use any of the following function to develop the solution_path function
Distance function - calculates the distance between two points
def distance(p1, p2) : distance = sqrt (((p1 [0] - p2 [0]) **2) + ((p1 [1] - p2 [1]) **2)) return (distance)
Find_closest function - calculates the closest point to the starting point
def find_closest(start_point, remaining_points): closest_distance = 99999 for coordinate in remaining_points: dist = distance(start_point, coordinate) if(dist < closest_distance): closest_distance = dist closest_point = coordinate return closest_point
Path_distance function -…
arrow_forward
See attached images.
Please help - C++
arrow_forward
In cell C18 type a VLOOKUP function to find the corresponding letter grade (from column D) for the name in A18. The table array parameter is the same as in B18. Type FALSE for the range lookup parameter. Copy the formula in C18 to C19:C22Notice this formula works correctly for all cells. Range lookup of FALSE means do an exact match on the lookup value whether or not the table array is sorted by its first column.
In cell E11, type an IF function that compares the score in B11 with the minimum score to pass in A5. If the comparison value is true, display Pass. Otherwise, display Fail. Copy the formula in E11 to E12:E15. Did you use appropriate absolute and relative references so the formula copied properly?
In cell F11, type an IF function that compares the grade in D11 with the letter F (type F). If these two are not equal, display Pass. Otherwise, display Fail. Copy the formula in F11 to F12:F15
arrow_forward
Part B - reading CSV files
You will need a Python repl to solve part B.
Define a Python function named cheapest_rent_per_state that has one parameter. The parameter is a string representing the name of a CSV file. The CSV file will be portion of a dataset published by the US
government showing the median (middle) rent in every county in the US. Each row in the CSV file has the same format
Area Name, Efficiency, 1-Bedroom, 2-Bedroom, 3-Bedroom, 4-Bedroom, State Code
The data in the Area Name and State Code columns are text. The data in all of the other columns are decimal numbers.
Your function will need to use the accumulator pattern to return a dictionary. The keys of that dictionary will be the state codes read in from the file (state codes are at index 6). For each key in the dictionary, it's
value should be the smallest median rent for an efficiency in that state (median rents for an efficiency are at index 1).
Important Hints:
* You will really, really want to use the built-in csv…
arrow_forward
Write a PHP code to:
Create the following two dimensional array $students:
ID
Name
Score
100
Ali
76
200
Khaleed
33
300
Fatema
89
400
Sumaya
45
Write the function nbFail($list) that takes a multidimensional array $list as parameter and returns the number of failed students (student fails if the score is less than 50).
Write the required PHP code to display the array $students in an HTML table (using foreach statement) and the number of failed students and passed students (use the function defined in question b).
arrow_forward
get_column_choice(): this function will allow us to ask the players which pocket they want to grab stones from. Since we know which row they are grabbing from (since it is not allowed to grab stones from your opponent’s side), we only need to get the column they want. As previously stated, we are using letters to represent the columns to make it easier on the player. This function accepts the current player (should be a 0 or 1), the board, and a list of letters (the same as the list you passed to the print_game_board function) and it will return the index of the column being chosen.
The function should ask the player to enter a column letter, then it should check that the conditions listed below hold. If they don’t meet these conditions, it should ask again until it gets a valid letter, otherwise it should return the corresponding column index for that letter.
Letter entered must be on the game board. It can be lower case or upper case. (Hint: this doesn’t need to be hard coded,…
arrow_forward
55 BMW 228i 2008 122510 7800 65
Honda Accord 2011 93200 9850 45
Toyota Camry 2010 85300 9500 25
Honda Civic 2010 86400 9100 15
Mazda Zoom 3 2013 72450 8950 35
Mazda Cx7 2009 102200 7300 5
Toyota Corolla 2013 68900 10100 75
Ford Mustang 2008 112500 13200 95
Mercedes Benz C250 2012 65300 17300
arrow_forward
Computer Science
csv file "/dsa/data/all_datasets/texas.csv"
Task 6:
Write a function "county_locator" that allows a user to enter in a name of a spatial data frame and a county name and have a map generated that shows the location of that county with respect to other counties (by using different colors and/or symbols).
Hint: function(); $county ==; plot(); add=TRUE.
arrow_forward
CCDConnect < C X
Dashboard - CCD X
M5 Assignment 1 x ▸ How to install Or X
https://mycourses.cccs.edu/d21/le/content/83491/viewContent/6197961/View
Requirements for Your M5 Assignment 1 Pseudocode for Program Integration
For the first module, write the pseudocode to process these tasks:
(Note: lines beginning with # are comments with tips for you)
2. Define a class called Dice
PDF Oracle 12c SQL (
1. From the random module import randint to roll each die randomly
a. # in pseudocode, import a random function
b. # the name is helpful for the next M5-2 assignment
a. In Python, the syntax has a colon after it: class Dice():
b. In pseudocode, you can specify it generally or be specific
3. Under the class declaration, list the attributes. Here are some tips:
a. # attributes are what we know about a single die (dice is plural)
b. # self is the first attribute in Python and must always appear first
c. # add a num_sides attribute and to set it to 6 for the 6 sides on the dice
4. Define a method…
arrow_forward
In Python,
grades_dict = {'Wally': [87,96,70], 'Eva': [100,87,90], 'Sam': [94,77,90], 'Katie': [100,81,82],
'Bob': [83, 65, 85]}
Ask user to enter the names of the rows, i.e., indices.
You can use: len(grades) to get the number of rows of the DataFrame. In addition, using the
sort_index() method, ask user whether they wish to sort by rows or by columns and whether to
sort in ascending or descending order (do not use any if-else statements)
arrow_forward
The following numbers are inserted into an empty LLRBT in the given order: 11, 2, 14, 25, 15, 13, 16. Please draw the resulting LLRBT.
arrow_forward
Python Code:
Use def functions.
Please upload the code. Thank you.
Stop when and only when the user has exactly five courses.
Whenever the user must add courses, accept a comma-separated list of courses, and add each of them.
Before adding the course, remove any whitespace around its name, and correct its capitalization.
Whenever the user must drop courses, accept a comma-separated list of courses, and delete each of them.
Treat the names the same way and the comparisons will be easy.
You need to check for, and ignore, them trying to drop courses they aren't taking!
arrow_forward
Create a simple program for a quiz
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
Create a folder named LastName_FirstName (ex. Dahan yoon) in your local
Create a new project named LabExer5B. Set the project location to your own
The program shall:
contain an array of 10 multiple choice questions with three (3) choices each and
require the user to choose among A, B, or C;
Note: Cases are ignored. Lowercase letters are acceptable (a, b, c).
Create a try-catch structure to handle three (3) exceptions. These are when the user inputs the following:
an invalid letter (not A, B, or C)
a number or any special character
blank (no answer)
Prompt the user that he can answer again if any of the three (3) exceptions is
Display the score.
arrow_forward
C++
Code with comments and output screenshot is must for an Upvote. Thank you
arrow_forward
Python Programming Assignment: Create a dictionary that takes a basketball team's information (that includes team's name and points) as parameter, creates a teams_dict and returns it. Also, create a parameter for write_file parameter that opens current results text file in write mode and writes the dictionary information to file as per format and closes the file.
arrow_forward
python function!: a function called popular_color with one parameter, of type dict[str, str] of names and favorite colors. The return type is a str and it is the most commonly occuring color. If two colors are tied for popularity, the first color shown will be picked.
arrow_forward
In Java Language Write Code below Image
arrow_forward
def sw_vehicle_search(cargo_capacity: int, max_speed: int, cost: int) -> list:
This function will use the https://swapi.dev/ API to collect its information. This function is meant to be used to search for vehicles in Star Wars that meet the specified criteria. The function should return a list of names of the vehicles that have cargo capacities and a max speed greater than or equal to the value specified but also cost less than or equal to the cost given. Vehicles with "unknown" or "none" for any of the mentioned categories should not be regarded.
Keep in mind that not all of the vehicles in star wars are returned in one request to https://swapi.dev/api/vehicles/. The JSON object returned by this request has a next attribute that represents the next page of information. You must make multiple requests for each page to collect all vehicle information.
You might find it helpful to use the following API handle(s):
https://swapi.dev/api/vehicles/ - to retrieve all vehicles in Star Wars…
arrow_forward
def sw_vehicle_search(cargo_capacity: int, max_speed: int, cost: int) -> list:
This function will use the https://swapi.dev/ API to collect its information. This function is meant to be used to search for vehicles in Star Wars that meet the specified criteria. The function should return a list of names of the vehicles that have cargo capacities and a max speed greater than or equal to the value specified but also cost less than or equal to the cost given. Vehicles with "unknown" or "none" for any of the mentioned categories should not be regarded.
Keep in mind that not all of the vehicles in star wars are returned in one request to https://swapi.dev/api/vehicles/. The JSON object returned by this request has a next attribute that represents the next page of information. You must make multiple requests for each page to collect all vehicle information.
You might find it helpful to use the following API handle(s):
https://swapi.dev/api/vehicles/ - to retrieve all vehicles in Star Wars…
arrow_forward
Create a Code Blocks project named LinkedLists. This program will use a set of data structures into which the user will enter
contact information.
Declare a Contactinfo struct pointer. It should have felds for contact information and also contain a next feld.
Create a getContactlnfo function that asks for contact information and places the information into a struct.
Call getContactinfo ten times from a for loop, and each time add the new data structure to the end of the list (use a function
named addContactinfoToList( struct Contactlnfo "info, struct Contactinfo 'newlnfo) to ad the new data structure to the list).
Write a function that displays all of the data in the list.
arrow_forward
without using files
develop a library management system application. Morespecifically, your application should use an array of structuresto keep the details of the booksand the user should be able to add a new book, search for a book and find available books toborrow. The book details entered by the user should be stored in a structure using thefollowing details:• Book ISBN• Book title• Author full name• Publication Year• Availability for loan [yes/no]
The user of this application should have the following options in the form of a nice presentedmenu.Add a book: The user should add all the details of each book by answering a set of questions(book isbn? Book title? author? year?). The availability for loan is randomly generated by theprogram (y: yes, n:no). Assume that the maximum number of books that the user can giveyou is 50.Search for a book: The user should be given three options here.▪ Search by the title: The user will input a keyword and the program willsearch for all the titles…
arrow_forward
Python code
arrow_forward
Attached File: a2provided:
grades = [[71, 14, 41, 87, 49, 93],[66, 73, 54, 55, 94, 79],[20, 51, 77, 20, 86, 15],[79, 63, 72, 57, 63, 73],[30, 66, 78, 65, 41, 45],[11, 83, 51, 47, 68, 63],[99, 43, 85, 86, 24, 75],[67, 102, 11, 84, 64, 109],[16, 24, 101, 78, 55, 89],[100, 26, 37, 95, 106, 100],[55, 77, 30, 34, 28, 15],[87, 67, 13, 71, 67, 83],[95, 65, 94, 56, 15, 92],[102, 23, 36, 39, 60, 39],[90, 59, 96, 105, 83, 16],[101, 40, 17, 12, 44, 36],[39, 63, 96, 12, 65, 82],[95, 20, 105, 34, 69, 95],[95, 93, 75, 18, 105, 102],[35, 15, 82, 106, 73, 30],[80, 52, 67, 49, 11, 88],[86, 17, 44, 75, 78, 49],[22, 60, 74, 110, 92, 37],[13, 14, 15, 82, 75, 57],[71, 106, 15, 77, 30, 98],[43, 80, 76, 85, 102, 53],[26, 98, 60, 80, 104, 79],[12, 28, 40, 106, 88, 84],[82, 75, 101, 29, 51, 75],[20, 31, 84, 35, 19, 85],[84, 63, 56, 101, 83, 102],[25, 106, 36, 24, 61, 80],[86, 75, 89, 47, 28, 27],[38, 48, 22, 72, 53, 83],[70, 34, 46, 86, 32, 58],[83, 59, 38, 16, 94, 104],[62, 110, 13, 12, 13, 83],[90, 59, 55,…
arrow_forward
Twitter Data:
twitter_url = 'https://raw.githubusercontent.com/Explore-AI/Public-Data/master/Data/twitter_nov_2019.csv'twitter_df = pd.read_csv(twitter_url)twitter_df.head()
Question: Write the Number of Tweets per Day
Write a function which calculates the number of tweets that were posted per day.
Function Specifications:
It should take a pandas dataframe as input.
It should return a new dataframe, grouped by day, with the number of tweets for that day.
The index of the new dataframe should be named Date, and the column of the new dataframe should be 'Tweets', corresponding to the date and number of tweets, respectively.
The date should be formated as yyyy-mm-dd, and should be a datetime object. Hint: look up pd.to_datetime to see how to do this.
arrow_forward
Tasks
2
Task 1: Write a function called calculate_statistics that accepts a list of numbers and returns three values:
.
The sum of all numbers.
.
The average of the numbers.
.
The maximum number.
•
Task 2: Write a program that contains a global list of students. Create a function add_student that takes a name
as an argument and appends it to the global student's list. The function should also print the updated list of
students
• Task 3:Write a function build_profile that accepts a person's first and last name and an arbitrary number of
keyword arguments (**kwargs). The function should return a dictionary representing the person's profile,
including any additional information passed via the keyword arguments.
•
Task 4: Write a function make_multiplier that accepts a number n and returns another function that multiplies its
argument by n. Use the returned function to create multipliers for 2and 3
• Task 5: Write a recursive function named sum_of_digits that takes a non-negative integer…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
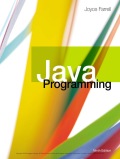
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Related Questions
- #Data is given in the order: Serial Number | Item Name | Quantity | Priceprice_list = [["01","Keyboard",4,200.0],["02","Mouse",4,150.0],["03","Printer",2,300.0]] #Write Code to increase the price of all the items by 50 taka #Code1 #Write code to add a new item to the list using user input #Code2 print()#Print the list using nested loops #Code3 #Sample output given below: Enter Serial: 05Enter Item Name: MonitorEnter Quantity: 200Enter Price: 50 01Keyboard4250.0-----------------02Mouse4200.0-----------------03Printer2350.0-----------------05Monitor20050-----------------arrow_forwardPlse use c# programming languagearrow_forwardLooking for this in Python, please. Shopping list assignment (Parallel Array) Create three global variables with the proper data types: sub_totaltotal_pricesales_tax Sales tax is 9.5% Seven items minimum Create a shopping list (List, Tuple or Dictionary) of your choice (Items in list) Create a price list (Price for each item in shopping list) Create and print your parallel array (match each item to its price) Print the list with its index numbers Select one or more items from the shopping list using the index number Print your selected items with price Print sub total (base price of item(s) before tax) Print sales tax amount (before adding it into total price) Calculate and print total price with tax included. (this is what you would pay walking out the door) Round to two decimal pointsarrow_forward
- The above screen is used to browse the products table it uses the ProductDAO.java file, it calls the getProductByCriteria() function which should accept a parameter, this parameter should be the criteria that would be built from the two combo boxes and the text box, and queries the products table.The getProductByCriteria() function should return a list of Product objects that matches the criteria specified. Modify the function so that it accepts a string paramater consisting of the fields from the combo boxes and a price number from the text box separated by commas and use this string to modify the query in the function to return the desired result set. public List<Product> getProductByCriteria() //MAKE MODIFICATIONS TO THIS FUNCTION SO IT CAN TAKE PARAMETER(S) { if (openConnection()) { try{ List<Product> items = new ArrayList <> (); Product temp = null; String…arrow_forwardDec2Hex function : def decimal_to_hex(number): hex_map = {0: '0', 1: '1', 2: '2', 3: '3', 4: '4', 5: '5', 6: '6', 7: '7', 8: '8', 9: '9', 10: 'A', 11: 'B', 12: 'C', 13: 'D', 14: 'E', 15: 'F'} hex_digits = [] while number > 0: hex_digits.append(hex_map[number % 16]) number //= 16 # Reverse the order of the hex digits and join them to form the final hex string hex_string = ''.join(reversed(hex_digits)) return f'0x{hex_string}' if hex_string else '0x0' Perform a Profile of your Dec2Hex function. Write a function that takes a timedate object as a parameter andcalculates the number of years from NOW to the time in the timedateobject. Add unit-testing code to your Dec2Hex exercise, and then perform aUnit test of the updated Dec2Hex code.arrow_forwardUse Python Programming - restaurant reservation program. The application offers different reservation rate for adults and children. (see sample below) RESTAURANT RESERVATION SYSTEMSystem Menua. View all Reservationsb. Make Reservationc. Delete Reservationd. Generate Reporte. Exit 2. When the VIEW RESERVATIONS is clicked, this will display all the reservations made: # Date Time Name Adults Children 1 Nov 10, 2020 10:00 am John Doe 1 1 2 Nov 25, 202011:00 am Michelle Franks 2 1 3 Dec 10, 2020 9:00 am Ella Flich 1 1 4 Dec 21, 2020 12:00 pmDylan Cloze 2 1 3. If the user selects MAKE RESERVATION, the user needs to input the following: a. Name (String)b. Date (String)c. Time (String)d. No of Adults (Integer)e. No of Children (Integer) Note: Adult is 500 per head and Kids is 300 per head reservation, save the data in a text file. 4. If DELETE RESERVATION is selected, the user needs to input the reservation number to remove the existing reservation. 5. If the GENERATE REPORT is clicked,…arrow_forward
- Use Python Programming - restaurant reservation program. The application offers different reservation rate for adults and children. (see sample below) RESTAURANT RESERVATION SYSTEMSystem Menua. View all Reservationsb. Make Reservationc. Delete Reservationd. Generate Reporte. Exit 2. When the VIEW RESERVATIONS is clicked, this will display all the reservations made: # Date Time Name Adults Children 1 Nov 10, 2020 10:00 am John Doe 1 1 2 Nov 25, 202011:00 am Michelle Franks 2 1 3 Dec 10, 2020 9:00 am Ella Flich 1 1 4 Dec 21, 2020 12:00 pmDylan Cloze 2 1 3. If the user selects MAKE RESERVATION, the user needs to input the following: a. Name (String)b. Date (String)c. Time (String)d. No of Adults (Integer)e. No of Children (Integer) Note: Adult is 500 per head and Kids is 300 per head reservation, save the data in a text file. 4. If DELETE RESERVATION is selected, the user needs to input the reservation number to remove the existing reservation. 5. If the GENERATE REPORT is clicked,…arrow_forwardThe parameter represents converted station data for a single station. is_app_only The function should return True if and only if the given station requires the use of an app because it does NOT have a kiosk. Recall that a station requires the use of an app if and only ("Station") -> bool if the string that the constant NO_KIOSK refers to is part of its station name.arrow_forwardswitch_player(): we will allow the players to choose their own names, but for our purposes, it is easier to think of them as number (0 and 1, for indexing purposes). We will display their names to them on the screen, but in the background, we will keep track of them based on their number. This function should take 1 argument, an integer (either 0 or 1) representing the player and should return the opposite integer (1 if 0 is entered and 0 if 1 is entered). This is a simple function, but it will make our code cleaner later. Use your constants! Using Thonnyarrow_forward
- PYTHON CODING This function takes a list of points and then returns a new list of points, which starts with the first point that was given in the list, and then followed by points closest to the start point. Here is the function that needs to be created: def solution_path(points) : # insert code here return path Please use any of the following function to develop the solution_path function Distance function - calculates the distance between two points def distance(p1, p2) : distance = sqrt (((p1 [0] - p2 [0]) **2) + ((p1 [1] - p2 [1]) **2)) return (distance) Find_closest function - calculates the closest point to the starting point def find_closest(start_point, remaining_points): closest_distance = 99999 for coordinate in remaining_points: dist = distance(start_point, coordinate) if(dist < closest_distance): closest_distance = dist closest_point = coordinate return closest_point Path_distance function -…arrow_forwardSee attached images. Please help - C++arrow_forwardIn cell C18 type a VLOOKUP function to find the corresponding letter grade (from column D) for the name in A18. The table array parameter is the same as in B18. Type FALSE for the range lookup parameter. Copy the formula in C18 to C19:C22Notice this formula works correctly for all cells. Range lookup of FALSE means do an exact match on the lookup value whether or not the table array is sorted by its first column. In cell E11, type an IF function that compares the score in B11 with the minimum score to pass in A5. If the comparison value is true, display Pass. Otherwise, display Fail. Copy the formula in E11 to E12:E15. Did you use appropriate absolute and relative references so the formula copied properly? In cell F11, type an IF function that compares the grade in D11 with the letter F (type F). If these two are not equal, display Pass. Otherwise, display Fail. Copy the formula in F11 to F12:F15arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
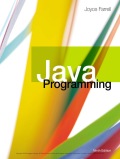
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT