LQR
.pdf
keyboard_arrow_up
School
University of Illinois, Urbana Champaign *
*We aren’t endorsed by this school
Course
353
Subject
Computer Science
Date
Apr 3, 2024
Type
Pages
7
Uploaded by elgelie7
LQR
March 1, 2024
1
LQR
[1]:
import
numpy
as
np
from
scipy
import
linalg
import
sympy
as
sym
This is the infinite-horizon
L
inear
Q
uadratic
R
egulator (LQR) optimal control problem:
minimize
u
[
t
0
,
∞
)
∫
∞
t
0
(
x
(
t
)
T
Qx
(
t
) +
u
(
t
)
T
Ru
(
t
)
)
dt
subject to
˙
x
(
t
) =
Ax
(
t
) +
Bu
(
t
)
,
x
(
t
0
) =
x
0
The integrand is
cost
. The integral is
total cost
. The square matrices
Q
and
R
are
weights
— you,
as a control designer, get to choose these weights.
The minimizer (i.e., the input that achieves
minimum total cost) is
u
(
t
) =
−
Kx
(
t
)
and the minimum (i.e., the minimum total cost) is
x
T
0
Px
0
where
P
and
K
can be found in python as follows:
P = linalg.solve_continuous_are(A, B, Q, R)
K = linalg.inv(R) @
B.T @ P
There are a number of reasons why LQR is a good choice for control design. At this point, we will
simply say that LQR makes it easy to get a working controller.
Why? Notice that, for this optimal control problem to make any sense, its minimum total cost —
i.e., the value of the integral for the “best” choice of
K
— has to be finite. Since the upper limit of
the integral is infinity, then — in order for the integral to be finite — the integrand has to converge
to zero (quickly).
The only way for it to do that is if the closed-loop system is asymptotically
stable. In other words,
the “best” choice of
K
has to produce a closed-loop system that
is asymptotically stable.
Put simply, the solution to the LQR problem, for any choice of weights, is a controller that produces
a stable closed-loop system — i.e., a “working controller.”
1
Let’s look at an example.
Suppose we want to design a controller by solving the LQR problem for the system described by
A
=
[
0
1
0
0
]
B
=
[
0
1
]
and for weights that are both identity matrices:
Q
=
I
2
×
2
=
[
1
0
0
1
]
R
=
I
1
×
1
=
[
1
]
First, we define the system:
[32]:
A
=
np
.
array([[
0.
,
1.
], [
0.
,
0.
]])
print
(
f'A =
{
A
.
tolist()
}
'
)
B
=
np
.
array([[
0.
], [
1.
]])
print
(
f'B =
{
B
.
tolist()
}
'
)
A = [[0.0, 1.0], [0.0, 0.0]]
B = [[0.0], [1.0]]
Second, we define the weights:
[33]:
Q
=
np
.
eye(
2
)
print
(
f'Q =
{
Q
.
tolist()
}
'
)
R
=
np
.
eye(
1
)
print
(
f'R =
{
R
.
tolist()
}
'
)
Q = [[1.0, 0.0], [0.0, 1.0]]
R = [[1.0]]
Third, we find the optimal cost matrix:
[34]:
P
=
linalg
.
solve_continuous_are(A, B, Q, R)
print
(
f'P =
{
P
.
tolist()
}
'
)
P = [[1.7320508075688763, 0.9999999999999993], [0.9999999999999993,
1.7320508075688772]]
Fourth, and finally, we find the optimal gain matrix:
[35]:
K
=
linalg
.
inv(R)
@
B
.
T
@
P
print
(
f'K =
{
K
.
tolist()
}
'
)
K = [[0.9999999999999993, 1.7320508075688772]]
As we claimed, this gain matrix makes the closed-loop system stable:
2
[36]:
linalg
.
eigvals(A
-
B
@
K)
[36]:
array([-0.8660254+0.5j, -0.8660254-0.5j])
Suppose we start with the following initial condition:
x
0
=
[
1
−
2
]
[37]:
x0
=
np
.
array([
1.
,
-2.
])
The input that we would apply at time
t
0
is, of course, the following:
u
(
t
0
) =
−
Kx
(
t
0
) =
−
Kx
0
Let’s compute it:
[38]:
u
= -
K
@
x0
print
(
f'u =
{
u
.
tolist()
}
'
)
u = [2.4641016151377553]
Let’s also compute the total cost
x
T
0
Px
0
that we would accumulate if we continued to apply this same controller
u
(
t
) =
−
Kx
(
t
)
for all time, starting from
x
(
t
0
) =
x
0
[39]:
total_cost
=
x0
.
T
@
P
@
x0
print
(
f'total_cost =
{
total_cost
}
'
)
total_cost = 4.660254037844388
Note that the total cost is a scalar quantity (just one real number, not a vector or matrix).
You try.
Consider this system:
A
=
[
1
3
−
2
2
]
B
=
[
−
1
1
]
3
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
proof, Lemma double_modus_ponens : forall P Q R S : Prop, (P->Q) -> (R->S) -> (P /\ R) -> (Q /\ S). in coq
arrow_forward
Runtime
HN3456 ∞ OHNM
2
3 Derive runtime as function of x.
4 Determine big-o upper bound.
5 Show work.
3.)
7 for (int i
8
9
10
11
12
13 }
1; i = 1; j--) {
for (int k = 1; k
=
for (int j
=
arrow_forward
Construct an NPDA that accepts L(G) for the following G:
arrow_forward
The branching part of the branch and bound algorithm that Solver uses to solve integer optimization models means that the algorithm
[a] creates subsets of solutions through which to search[b] searches through only a limited set of feasible integer solutions
[c] identifies an incumbent solution which is optimal
[d] uses a decision tree to find the optimal solution
2. The LP relaxation of an integer programming (IP) problem is typically easy to solve and provides a bound for the IP model.
[a] True [b] False
arrow_forward
Write the ô function of the following (1) dfa (8pe4 : Q×£ –→Q)and (2) nfa (
SNEA : Qx (EU{2})→ P(Q)) respectively, where E={a,b}.
DFA
а, b
(1)
b
a
Da,b
a,b
(2)
b
92
a
arrow_forward
a)
Why can Dijkstra's algorithm not work properly on graphs with negative
weighted edges? Explain with example.
Implement the greedy approach for coin change algorithm and show your step by
step approach to give change of 139 in coin change with {1, 3, 5, 25, 45, 60} unit
values.
c)
Write the procedure for calculating GCD and LCM of the following numbers.
Also write the answers at last.
2, 4, 5, 20
arrow_forward
Let P2(x) be the least squares interpolating polynomial for f(x) := sin(πx) on the interval [0,1] (with weight function w(x) = 1). Determine nodes (x0,x1,x2) for the second-order Lagrange interpolating polynomial Pˆ2(x) so that P2 = Pˆ2. You are welcome to proceed theoretically or numerically using Python.
arrow_forward
Procedure 1 (Local Search(y) with depth δ) t := 1.While t ≤ δ and ∃z : (H(z,y)=1 and f(z) > f(y)) do y := z. t := t + 1.If there is more than one Hamming neighbor with larger fitness, z may be chosen arbitrarily among them.Algorithm 1 ((1+1) Memetic Algorithm ((1+1) MA))
write correct algorithm otherwise you will get downvote.
arrow_forward
Procedure 1 (Local Search(y) with depth δ) t := 1.While t ≤ δ and ∃z : (H(z,y)=1 and f(z) > f(y)) do y := z. t := t + 1.If there is more than one Hamming neighbor with larger fitness, z may be chosen arbitrarily among them.Algorithm 1 ((1+1) Memetic Algorithm ((1+1) MA))
arrow_forward
(1) List the first four elements of L(G) in lexicographic order.
1,010, 111, 00100
O €, 1, 010, 111
O 01, 010, 111, 00100
10,010, 111, 00100
arrow_forward
Appendix A
10-Fold Cross Validation for Parameter Selection
Cross Validation is the standard method for evaluation in empirical machine learning. It can also be used
for parameter selection if we make sure to use the training set only.
To select parameter A of algorithm A(X) over an enumerated range d E [A1,..., A] using dataset D, we do
the following:
1. Split the data D into 10 disjoint folds.
2. For each value of A e (A1,..., Ar]:
(a) For i = 1 to 10
Train A(A) on all folds but ith fold
Test on ith fold and record the error on fold i
(b) Compute the average performance of A on the 10 folds.
3. Pick the value of A with the best average performance
Now, in the above, D only includes the training data and the parameter A is chosen without the knowledge
of the test data. We then re-train on the entire train set D using the chosen A and evaluate the result on
the test set.
arrow_forward
2. Given the following KB:
B, L → P
L>B
L
Apply inference by enumeration to show that the above KB could entail P
arrow_forward
Python
Big-O Coding/Solving
arrow_forward
Computer Science
Consider the d-Independent Set problem:
Input: an undirected graph G = (V,E) such that every vertex has degree less or equal than d.
Output: The largest Independent Set.
Describe a polynomial time algorithm Athat approximates the optimal solution by a factor α(d). Your must
write the explicit value of α, which may depend on d. Describe your algorithm in words (no pseudocode) and
prove the approximation ratio α you are obtaining. Briefly explain why your algorithm runs in polytime.
arrow_forward
4. Reducibility, NP-completeness
4a. Recall that linear ax + b = 0 is reducible to the quadratic ax²+bx+c=0. Show that the quadratic ax2+bx+c=0 is reducible to quartic ax*+bx+cx+dx+e=0 (Lec14.1). Is quartic reducible to quadratic?
4b. Consider the 4-way Partition Problem: Given a set S of positive integers, determine whether S can be partitioned into four subsets S1, S2, S3, and S4 such that the sum of the numbers in S, is equal to S2, S3, and S4. Demonstrate that the problem is NP-complete based on NP-completeness of the 2-way Partition Problem.
4c. Prove that P != NP.
arrow_forward
<s> I am Sam </s><s> Sam I am </s><s> I am Sam </s>
<s> I do not like green eggs and Sam </s>
If we use linear interpolation smoothing between a maximum-likelihood bigram model and a maximum-likelihood unigram model with lambda1 = 1⁄2 and lambda2=1⁄2, what is P(Sam|am)? Include <s> and </s> in your counts just like any other token.
arrow_forward
Let X = {l ∈ Z | l = 5a + 2 for some integer a}, Y = {m ∈ Z | m = 4b + 3 for some integer b}, and Z = {n ∈ Z | n = 4c − 1 for some integer c}.
Is X ⊆ Y ?
Is Y ⊆ Z?
arrow_forward
Question 8
Greedy best-fırst search is equivalent to A* search with all step costs set to 0.
O True
O False
Question 9
If you had implemented Uniform Cost Search (the graph search version) in
Programming Assignment 1, it would have found an optimal solution. (You may
assume that the path costs are kept with the nodes on the frontier and
explored lists and checked when comparing newly generated states to what
has been seen before.)
O True
O False
Question 10
A* search with an admissible heuristic always expands fewer nodes than
depth-first search.
O True
O False
arrow_forward
adgis s
o (6): = min { d(v) Ive V3 is the minimum
degree of G. 0612 Z9Nto
JJ
V PO
Go is
called K-connected if IGI>K and
GIX is connected for every set x CV
with 1X1
arrow_forward
Question 5:
Minimize the following boolean function K map:
F(A, B, C, D) = Em(0, 2, 8, 10, 14):
Σd(5 , 15 )
arrow_forward
2.
Let (Finite_DFA) = {w, Mx is a DFA and L(Mx) is finite}. Show that (Finite_DFA) is decidable.
arrow_forward
Let A = {x ∈ Z : x ≤ 3} and let B = {x ∈ Q : x2 = 9}. Is B ⊆ A? Give a brief reason for your answer.
arrow_forward
Minimize using K- map the logical
funclion F(x,Y,z)= SoP C1,3,5,4) ?
arrow_forward
Remove Null productions from the given CFG
S→bS | aA
A→ bA aF Λ
F→ bF | Λ
arrow_forward
Write Algorithm for Combining two mass functions.in: mapping m1 : ℘ ( ) \∅→ [0, 1] (the domain elements with non-zero range value is denoted by M1 ⊆ ℘ ( ) \ ∅); mapping m2 is defined similarly as m1out: combined mapping m3constant: set of hypothesis
arrow_forward
The following solution designed from a problem-solving strategy has been proposed for finding a minimum spanning tree (MST) in a connected weighted graph G:
Randomly divide the vertices in the graph into two subsets to form two connected weighted subgraphs with equal number of vertices or differing by at most Each subgraph contains all the edges whose vertices both belong to the subgraph’s vertex set.
Find a MST for each subgraph using Kruskal’s
Connect the two MSTs by choosing an edge with minimum wight amongst those edges connecting
Is the final minimum spanning tree found a MST for G? Justify your answer.
arrow_forward
Let NE NFA = {N| N is an nfa and L(N) = Ø}
1. Show the NENFA is in NP
2.Show that NENFA is in P
arrow_forward
Q1/ Simplify using K- map for the
following :
1- Y(A,B,C,D)= (6,14,4,12,9,11,1,3) 2-
Y(A,B,C,D)= E (5,7,13,15,2,10) 3-
Y(A,B,C,D)= E(1,3,5,7,15,13,9,11)
arrow_forward
Q3:
Solve this recurrence using domain transformation.
T(1) = 1
T(n) = T(n/2) + 6nlogn
arrow_forward
Write an approximate greedy algo for set cover problem and trace the algo to find min cost sub collection of 'S' that covers all the given elements
arrow_forward
L = {f in SAT | the number of satisfying assignments of f > 1/2 |f| }
Show that L is NP-complete
arrow_forward
2
Prove NP = PSPACE if TQBF ∈ NP . Prove P = PSPACE if TQBF ∈ P.
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
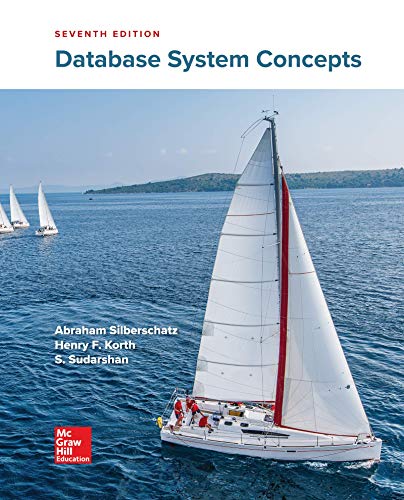
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
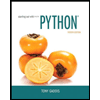
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
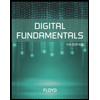
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
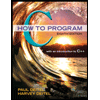
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
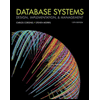
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
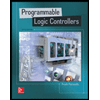
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- proof, Lemma double_modus_ponens : forall P Q R S : Prop, (P->Q) -> (R->S) -> (P /\ R) -> (Q /\ S). in coqarrow_forwardRuntime HN3456 ∞ OHNM 2 3 Derive runtime as function of x. 4 Determine big-o upper bound. 5 Show work. 3.) 7 for (int i 8 9 10 11 12 13 } 1; i = 1; j--) { for (int k = 1; k = for (int j =arrow_forwardConstruct an NPDA that accepts L(G) for the following G:arrow_forward
- The branching part of the branch and bound algorithm that Solver uses to solve integer optimization models means that the algorithm [a] creates subsets of solutions through which to search[b] searches through only a limited set of feasible integer solutions [c] identifies an incumbent solution which is optimal [d] uses a decision tree to find the optimal solution 2. The LP relaxation of an integer programming (IP) problem is typically easy to solve and provides a bound for the IP model. [a] True [b] Falsearrow_forwardWrite the ô function of the following (1) dfa (8pe4 : Q×£ –→Q)and (2) nfa ( SNEA : Qx (EU{2})→ P(Q)) respectively, where E={a,b}. DFA а, b (1) b a Da,b a,b (2) b 92 aarrow_forwarda) Why can Dijkstra's algorithm not work properly on graphs with negative weighted edges? Explain with example. Implement the greedy approach for coin change algorithm and show your step by step approach to give change of 139 in coin change with {1, 3, 5, 25, 45, 60} unit values. c) Write the procedure for calculating GCD and LCM of the following numbers. Also write the answers at last. 2, 4, 5, 20arrow_forward
- Let P2(x) be the least squares interpolating polynomial for f(x) := sin(πx) on the interval [0,1] (with weight function w(x) = 1). Determine nodes (x0,x1,x2) for the second-order Lagrange interpolating polynomial Pˆ2(x) so that P2 = Pˆ2. You are welcome to proceed theoretically or numerically using Python.arrow_forwardProcedure 1 (Local Search(y) with depth δ) t := 1.While t ≤ δ and ∃z : (H(z,y)=1 and f(z) > f(y)) do y := z. t := t + 1.If there is more than one Hamming neighbor with larger fitness, z may be chosen arbitrarily among them.Algorithm 1 ((1+1) Memetic Algorithm ((1+1) MA)) write correct algorithm otherwise you will get downvote.arrow_forwardProcedure 1 (Local Search(y) with depth δ) t := 1.While t ≤ δ and ∃z : (H(z,y)=1 and f(z) > f(y)) do y := z. t := t + 1.If there is more than one Hamming neighbor with larger fitness, z may be chosen arbitrarily among them.Algorithm 1 ((1+1) Memetic Algorithm ((1+1) MA))arrow_forward
- (1) List the first four elements of L(G) in lexicographic order. 1,010, 111, 00100 O €, 1, 010, 111 O 01, 010, 111, 00100 10,010, 111, 00100arrow_forwardAppendix A 10-Fold Cross Validation for Parameter Selection Cross Validation is the standard method for evaluation in empirical machine learning. It can also be used for parameter selection if we make sure to use the training set only. To select parameter A of algorithm A(X) over an enumerated range d E [A1,..., A] using dataset D, we do the following: 1. Split the data D into 10 disjoint folds. 2. For each value of A e (A1,..., Ar]: (a) For i = 1 to 10 Train A(A) on all folds but ith fold Test on ith fold and record the error on fold i (b) Compute the average performance of A on the 10 folds. 3. Pick the value of A with the best average performance Now, in the above, D only includes the training data and the parameter A is chosen without the knowledge of the test data. We then re-train on the entire train set D using the chosen A and evaluate the result on the test set.arrow_forward2. Given the following KB: B, L → P L>B L Apply inference by enumeration to show that the above KB could entail Parrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
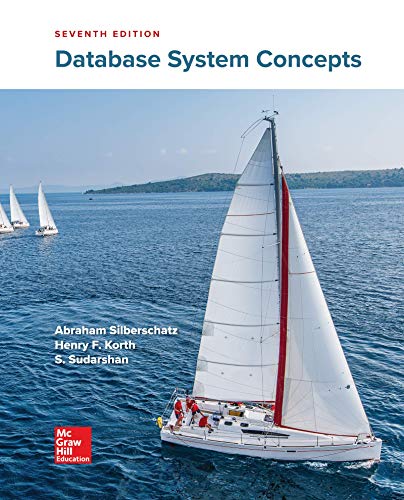
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
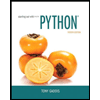
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
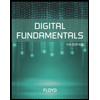
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
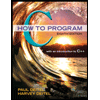
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
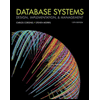
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
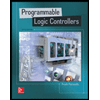
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education