Worksheet - Python Basics (1)
.docx
keyboard_arrow_up
School
George Washington University *
*We aren’t endorsed by this school
Course
3119
Subject
Computer Science
Date
Apr 3, 2024
Type
docx
Pages
2
Uploaded by BrigadierTroutPerson755
Worksheet – Python Basics
1 - Name 4 built-in data types: ____________ ____________ ____________ ____________
2 – In each example, we create a variable x. What is the data type of our variable x? x = 3.9
x = “abc123”
x = “ ”
x = ‘a’
x = False
x = 100
x = -0.5
x = -37
x = ‘123’
x = ’True’
3 - Name 4 built-in functions:
4 – True or False: Python is an interpreted language. 5 – True or False: Python is case-sensitive. 6 – True or False: Variable names can have spaces. 7 – True or False: For variable names, lower case is frequently used. 8 – True or False: For variable names, underscores can be used. 9 –The proper style for variable names in Python is:
a) camel case, as in acctName b ) snake case, as in acct_name
10 – True or False: This is a proper variable name: value1
11 – True or False: This is a proper variable name: 1value
12 – True or False: Data types must be stated when declaring variables. 13 – True or False: We can use single quotes or double quotes for strings. 14– True or False: It is possible to change the data type of a variable.
15 - What are the two reserve word values for the bool data type? _________ _________
16 - What data type must we always pass to the input() function? ________________________
17 - What data type does the input() function always return? ___________________________
18- Given the following code, list the following:
z = float(input(‘Enter your gpa: ’))
variable(s): ______________ literal(s): ___________________________________________
function(s) called: _____________________________________________________
data types used: ______________________________________________
19 - Given the following code, list the following:
x = 5; y = 6.6
print(x, y); z = input(‘Enter a num: ’)
variable(s): _____________________________________________________
literal(s): _____________________________________________________
function(s) called: _________________________________________________
argument(s): _____________________________________________________
data types used: ______________________________________________
20 – All code below works, but there are style rules not followed. Rewrite the statements:
acctNumber = 1234
acct_number=1234
print(‘Joe’,’Smith’)
stuName=input( ‘Enter your name: ‘ )
x=2
print(‘x is’,x)
21 – Describe the steps (in order) that are performed for this line of code:
z = float(input(‘Enter your gpa: ’))
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
JAVA LANGUAGE
Body Mass Index Version 2.0
by CodeChum Admin
Recall that according to the National Heart, Lung, and Blood Institute of the National Institutes of Health, body mass index (BMI) is a measure of body fat based on height and weight that applies to adult men and women. It is used to monitor one's health by determining whether one is underweight, overweight, has normal weight or is obese. It is computed based as follows (when using standard weight in pounds and height in inches):
BMI = 703 x (weight/(height2))
Furthermore, people with BMI scores that are less than 18.5 are said to be underweight. Those with scores between 18.5–24.9 (inclusive) are of normal weight. Those with scores between 25–29.9 (inclusive) are said to be overweight. And those with scores 30 or higher are obese.
Input
The input is composed of two positive integers representing the weight in pounds and the height in inches.
140·65
Output
A single line containing two values: the computed…
arrow_forward
Q5 PYTHON MULTIPLE CHOICE
Code Example 4-1
def get_username(first, last):
s = first + "." + last
return s.lower()
def main():
first_name = input("Enter your first name: ")
last_name = input("Enter your last name: ")
username = get_username(first_name, last_name)
print("Your username is: " + username)
main()
A. Refer to Code Example 4-1: What is the scope of the variable named s ?
a.
global
b.
local
c.
global in main() but local in get_username()
d.
local in main() but global in get_username()
arrow_forward
Upper, Dis-upper
Code in C language
arrow_forward
Assignment Name
JavaScript Concepts III
Assignment Filename
lastname-circleArea.html (replace lastname with your last name).
area.js
Assignment Description
This assignment demonstrates the following concepts: JavaScript Functions
Assignment Instructions
Write a JavaScript program that calculates the area of a circle:
Write a function that calculates the area of a circle given the radius.
Formula: Math.PI * (radius * radius)
Your program code should contain appropriately named variables.
Use documentation to identify various components of your program.
Call the function once and pass a radius value of 10.75 to it.
Use the starter files and complete the logic to satisfy the instructions.
To access the files, download and save the circleArea.zip folder attached to this assignment.
Then unzip the compressed folder to reveal the included files: circleArea.html and area.js. Be sure that the two files are in the same folder. Then write your code in the area.js…
arrow_forward
python
arrow_forward
Python Returning function
function name: is_prime
parameters: a number n
returns: True if n is prime
behavior: Check the values in the range [2, n) and return False if n is divible by any of them.
function name: bound_0_to_100
parameters:a number grade
returns: the grade with a minimum of 0 and a maximum of 100
behavior: In addition to exceeding 100, sometimes a grade will be so spectacularly bad that it falls below 0. In that case we want to normalize it to 0 so all grades fall into the range [0, 100]. ex: bound_0_to_100(75) returns 75, bound_0_to_100(103) returns 100, and bound_0_to_100(-5) returns 0
function name: bigger
parameters: two values a and b
returns: whichever of the two is greatest
behavior: ex: bigger(3, 9) returns 9
arrow_forward
java language coding please
Object Relationship and File IO
Write a program to perform statistical analysis of scores for a class of students. The class may have up to 40 students.There are five quizzes during the term. Each student is identified by a four-digit student ID number.
The program is to print the student scores and calculate and print the statistics for each quiz. The output is in the same order as the input; no sorting is needed. The input is to be read from a text file. The output from the program should be similar to the following:
Here is some sample data (not to be used) for calculations:
Stud Q1 Q2 Q3 Q4 Q5
1234 78 83 87 91 86
2134 67 77 84 82 79
1852 77 89 93 87 71
High Score 78 89 93 91 86
Low Score 67 77 84 82 71
Average 73.4 83.0 88.2 86.6 78.6
The program should print the lowest and highest scores for each quiz.
Plan of Attack
Learning Objectives
You will apply the following topics in this assignment:
File Input operations.
Working and…
arrow_forward
python regular expressions
arrow_forward
python regular expressions
arrow_forward
python multiple choice
Code Example 4-1
def get_username(first, last):
s = first + "." + last
return s.lower()
def main():
first_name = input("Enter your first name: ")
last_name = input("Enter your last name: ")
username = get_username(first_name, last_name)
print("Your username is: " + username)
main()
5. Refer to Code Example 4-1: What is the scope of the variable named s ?a. globalb. localc. global in main() but local in get_username()d. local in main() but global in get_username()
arrow_forward
Java Prog
arrow_forward
Allowed language: C language The output should be the same with example and please code correctly. Pls do not copy from other questions
arrow_forward
Java - Data Visualization (this is not graded)
arrow_forward
C++ LANGUAGE
Create a menu system program using Function:
Menu System
1 – converting ft to inch
2 – Area of a circle
3 – Exit
C++ LANGUAGE
Discussion Array
Store the 12 numbers in an arrayNum by using input. Then output the 12 numbers on array.
Example:
4 – 6 – 7 – 8 – 9 – 5 – 3 - 1- 22 – 2 – 33 - 8
upload your screenshot of the codes and output.
arrow_forward
CodeWorkout
Gym
Course
Q Search
kola shreya@ columbusstate.edu
Search exercises...
X274: Recursion Programming Exercise: Cannonballs
X274: Recursion Programming Exercise:
Cannonballs
Spherical objects, such as cannonballs, can be stacked to form a pyramid with one cannonball at the top,
sitting on top of a square composed of four cannonballs, sitting on top of a square composed of nine.
cannonballs, and so forth.
Given the following recursive function signature, write a recursive function that takes as its argument the
height of a pyramid of cannonballs and returns the number of cannonballs it contains.
Examples:
cannonball(2) -> 5
Your Answwer:
1 public int cannonball(int height) {
3.
4}
Check my answer!
Reset
Next exercise
Feedback
arrow_forward
C# language
arrow_forward
COSC 1336 – Programming Fundamentals IProgram 7 – Repetition Structures and Files
Python ProgrammingThe trustees of a small college are considering voting a pay raise for their faculty members. They want to grant a 7 percent raise for those earning more than $50,000.00, a 4 percent raise for those earning more than $60,000.00 and 5.5 percent raise for all others. However before doing so, they want to know how much this will cost. Write a program that will print the pay raise for each faculty member, the total amount of the raises, and the average of the raises. Also, print the total faculty payroll before and after the raise. Use the end of file as a sentinel value. The input data is available in program_7.pngDo NOT use any logical operator in the program. Run your program with the input file, program7.txt
Important Notes:
Follow the requirements.
Needs percentage applied and Raise amount of each salary displayed.
Total amount of Salary and Total of Raised salary should be displayed…
arrow_forward
COSC 1336 – Programming Fundamentals IProgram 7 – Repetition Structures and Files
Python ProgrammingThe trustees of a small college are considering voting a pay raise for their faculty members. They want to grant a 7 percent raise for those earning more than $50,000.00, a 4 percent raise for those earning more than $60,000.00 and 5.5 percent raise for all others. However before doing so, they want to know how much this will cost. Write a program that will print the pay raise for each faculty member, the total amount of the raises, and the average of the raises. Also, print the total faculty payroll before and after the raise. Use the end of file as a sentinel value. The input data is available in program_7.pngDo NOT use any logical operator in the program. Run your program with the input file, program7.txt
Use sep='' , ',.2f' where needed.
arrow_forward
C++A new video store in your neighborhood is about to open. However, it does not have a program to keep track of its videos and customers. The store managers want someone to write a program for their system so that the video store can operate.
The program will require you to design 2 ADTs as described below:
[1] VIDEO ADT
Data
Operations
Video_ID (preferably int, auto-generated)
Movie Title
Genre
Production
Number of Copies
Movie Image Filename
[1] Insert a new video
[2] Rent a video; that is, check out a video
[3] Return a video, or check in, a video
[4] Show the details of a particular video
[5] Display all videos in the store
[6] Check whether a particular video is in the store
[2] CUSTOMER PARENT ADT
Data
Operations
Customer_ID (preferably int, auto-generated)
Name
Address
[1] Add Customer
[2] Show the customer details
[3] Print list of all customers
[3] CUSTOMER-RENT CHILD ADT
Customer_ID (
Video_ID (of all rented videos of a…
arrow_forward
个人空间
Python Programming
作业作答
->
https://mooc1.chaoxing.com/mooc2/work/dowork?courseld=222701483&classld=50725394&cpi=102339231&workld=19472480&answerld...
Score
GPA
e.g
Exercise 4.3
= 100
5.0
99: GPA is4.9
290
24.0
92: GPA is4.2
91: GPA is4.1
Write codes to transform a
90: GPA is4.0
given score to GPA following the
89: GPA is3.9
rules shown in the right table.
280
2 3.0
82: GPA is3.2
81: GPA is3.1
Submit the screenshot of your
80: GPA is3.0
test results and check if it's what
79: GPA is2.9
you expected.
2 70
22.0
72: GPA is2.2
71: GPA is2.1
For example,
70: GPA is2.0
69: GPA is1.9
if score=76, then GPA=2.6
if score=88, then GPA=3.8
2 60
21.0
62: GPA is1.2
61: GPA is1.1
if score=32, then GPA=0
60: GPA is1.0
O A 59: GFA 0
44: GPA is 0
< 60
arrow_forward
C++ programming
Chapter(s) Covered:
Chapter 1-8
Concepts tested by the program:
Working with one dimensional parallel arrays
Use of functions
Use of loops and conditional statements
Project Description
The Lo Shu Magic Square is a grid with 3 rows and 3 columnsshown below.
The Lo Shu Magic Square has the following properties:
The grid contains the numbers 1 – 9 exactly. Each number 1 – 9must not be used more than once. So, if you were to add up thenumbers used,
The sum of each row, each column and each diagonal all add upto the same number,
Write a program that simulates a magic square using 3 onedimensional parallel arrays of integer type.
Each one the arrays corresponds to a row of the magicsquare.
The program asks the user to enter the values of the magicsquare row by row and informs the user if the grid is a magicsquare or not.
See the sample outputs for more clarification.
Project Specifications
Input for this project:
Values of the grid (row by row)
Output for this…
arrow_forward
Must be in C#
Answer properly do attach output screenshot else downvote
arrow_forward
python
The intent of this program is to manage a set of contacts. Each contact will have data associated with it:
Id – number/integer
First Name – string
Last Name – string
Age – number/integer
Phone Number – string
Email – string
Anything else you’d like to add to make yours unique (can result in extra credit)
Gender – character or string (m/f/o)
Twitter ID, Facebook Id, etc
You must allow the customer to do the following actions on the contact list:
List all contacts
Add contact
Delete contact
Edit contact
Exit program
You should leverage a database (PostgreSQL) to save everything to the DB and read from it.
You should use classes for this assignment. This means you should have two classes:
Contact – all the attributes/properties described above with appropriate constructor. Methods:
Add (constructor - __init__(p_id, p_fname, p_lname, p_age, p_phone, p_email, p_gender)
Edit
Contact List (contact_list) – built on Python list (or creating one within the constructor), you…
arrow_forward
Python Returning Funtion
function name: bound_0_to_100
parameters:a number grade
returns: the grade with a minimum of 0 and a maximum of 100
behavior: In addition to exceeding 100, sometimes a grade will be so spectacularly bad that it falls below 0. In that case we want to normalize it to 0 so all grades fall into the range [0, 100]. ex: bound_0_to_100(75) returns 75, bound_0_to_100(103) returns 100, and bound_0_to_100(-5) returns 0
arrow_forward
Programming language Visual Basic
arrow_forward
PYTHON PROGRAMMING
Chess Moves Mapper using Shortest path algorithm
As the title indicates, you will need to determine the number of moves it will take for the different chess pieces from their current position to any other tile in the board. While a standard chess board will have an 8 x 8 grid chess board, your code should be able to handle boards of different dimensions.
For this program the board will be blank. The only piece will be the one inputted.
Input Format
s1, s2, x1, y1, piece
All inputs end with a '\n'
s1, s2 - horizontal size and vertical size of the board respectively.
x1, y1 - starting position of the piece. Refer to Figure 3. for coordinate convention
piece - name of the piece. Use the following names. [pawn, rook, knight, bishop, queen, king]
Given the initial starting position of a given piece, you will be asked to determine the minimum number of moves needed to reach any position in the tile. A sample setup is shown below as well as a visualization of the…
arrow_forward
Top-Scorer
Code in C language
arrow_forward
PYTHON HOMEWORK QUESTION
def area(side1, side2): return side1 * side2
s1 = 12s2 = 6
Select all statements that correctly call the area function.
A. answer = area(s1,s2)B. print(f'The area is {area(s1,s2)}')C. area(s1,s2)D. result = area(side1,side2)
arrow_forward
CodeWorkout
Gym
Course
Search exercises...
Q Search
kola shreya@columbus
X275: Recursion Programming Exercise: Check Palindrome
X275: Recursion Programming Exercise: Check
Palindrome
Write a recursive function named checkPalindrome that takes a string as input, and returns true if the string is a
palindrome and false if it is not a palindrome. A string is a palindrome if it reads the same forwards or backwards.
Recall that str.charAt(a) will return the character at position a in str. str.substring(a) will return the substring of
str from position a to the end of str,while str.substring(a, b) will return the substring of str starting at position
a and continuing to (but not including) the character at position b.
Examples:
checkPalindrome ("madam") -> true
Your Answer:
1 public boolean checkPalindrome (String s) {
4
CodeWorkout © Virginia Tech
About
License
Privacy
Contact
arrow_forward
Programming Language: PHP
arrow_forward
python regular expressions
arrow_forward
Python
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
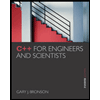
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Related Questions
- JAVA LANGUAGE Body Mass Index Version 2.0 by CodeChum Admin Recall that according to the National Heart, Lung, and Blood Institute of the National Institutes of Health, body mass index (BMI) is a measure of body fat based on height and weight that applies to adult men and women. It is used to monitor one's health by determining whether one is underweight, overweight, has normal weight or is obese. It is computed based as follows (when using standard weight in pounds and height in inches): BMI = 703 x (weight/(height2)) Furthermore, people with BMI scores that are less than 18.5 are said to be underweight. Those with scores between 18.5–24.9 (inclusive) are of normal weight. Those with scores between 25–29.9 (inclusive) are said to be overweight. And those with scores 30 or higher are obese. Input The input is composed of two positive integers representing the weight in pounds and the height in inches. 140·65 Output A single line containing two values: the computed…arrow_forwardQ5 PYTHON MULTIPLE CHOICE Code Example 4-1 def get_username(first, last): s = first + "." + last return s.lower() def main(): first_name = input("Enter your first name: ") last_name = input("Enter your last name: ") username = get_username(first_name, last_name) print("Your username is: " + username) main() A. Refer to Code Example 4-1: What is the scope of the variable named s ? a. global b. local c. global in main() but local in get_username() d. local in main() but global in get_username()arrow_forwardUpper, Dis-upper Code in C languagearrow_forward
- Assignment Name JavaScript Concepts III Assignment Filename lastname-circleArea.html (replace lastname with your last name). area.js Assignment Description This assignment demonstrates the following concepts: JavaScript Functions Assignment Instructions Write a JavaScript program that calculates the area of a circle: Write a function that calculates the area of a circle given the radius. Formula: Math.PI * (radius * radius) Your program code should contain appropriately named variables. Use documentation to identify various components of your program. Call the function once and pass a radius value of 10.75 to it. Use the starter files and complete the logic to satisfy the instructions. To access the files, download and save the circleArea.zip folder attached to this assignment. Then unzip the compressed folder to reveal the included files: circleArea.html and area.js. Be sure that the two files are in the same folder. Then write your code in the area.js…arrow_forwardpythonarrow_forwardPython Returning function function name: is_prime parameters: a number n returns: True if n is prime behavior: Check the values in the range [2, n) and return False if n is divible by any of them. function name: bound_0_to_100 parameters:a number grade returns: the grade with a minimum of 0 and a maximum of 100 behavior: In addition to exceeding 100, sometimes a grade will be so spectacularly bad that it falls below 0. In that case we want to normalize it to 0 so all grades fall into the range [0, 100]. ex: bound_0_to_100(75) returns 75, bound_0_to_100(103) returns 100, and bound_0_to_100(-5) returns 0 function name: bigger parameters: two values a and b returns: whichever of the two is greatest behavior: ex: bigger(3, 9) returns 9arrow_forward
- java language coding please Object Relationship and File IO Write a program to perform statistical analysis of scores for a class of students. The class may have up to 40 students.There are five quizzes during the term. Each student is identified by a four-digit student ID number. The program is to print the student scores and calculate and print the statistics for each quiz. The output is in the same order as the input; no sorting is needed. The input is to be read from a text file. The output from the program should be similar to the following: Here is some sample data (not to be used) for calculations: Stud Q1 Q2 Q3 Q4 Q5 1234 78 83 87 91 86 2134 67 77 84 82 79 1852 77 89 93 87 71 High Score 78 89 93 91 86 Low Score 67 77 84 82 71 Average 73.4 83.0 88.2 86.6 78.6 The program should print the lowest and highest scores for each quiz. Plan of Attack Learning Objectives You will apply the following topics in this assignment: File Input operations. Working and…arrow_forwardpython regular expressionsarrow_forwardpython regular expressionsarrow_forward
- python multiple choice Code Example 4-1 def get_username(first, last): s = first + "." + last return s.lower() def main(): first_name = input("Enter your first name: ") last_name = input("Enter your last name: ") username = get_username(first_name, last_name) print("Your username is: " + username) main() 5. Refer to Code Example 4-1: What is the scope of the variable named s ?a. globalb. localc. global in main() but local in get_username()d. local in main() but global in get_username()arrow_forwardJava Progarrow_forwardAllowed language: C language The output should be the same with example and please code correctly. Pls do not copy from other questionsarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
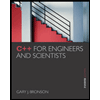
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr