MA544_PA1
.pdf
keyboard_arrow_up
School
Stevens Institute Of Technology *
*We aren’t endorsed by this school
Course
544
Subject
Computer Science
Date
Jan 9, 2024
Type
Pages
3
Uploaded by eliza1998
Programming Assignment - 1
Programming Assignment - 1
Question 1
Question 1
Write a function trace1D() that finds the trace of a matrix given by a one dimensional NumPy array. You can not
use the built-in function np.trace() or any other builtin function in any way.
Display the output of the follwing print(“Trace of the suggested matrix is: {}”.format(trace1D(np.arange(0,100,1))))
In [1]:
# import statements
import
import
numpy
numpy
as
as
np
np
import
import
math
math
import
import
sys
sys
# Write your function here
def
def
trace1D
(mat1D):
array_len
=
mat1D
.
shape[
0
]
print
(
"You have provided an array of length "
,array_len)
n
=
math
.
ceil (math
.
sqrt(array_len))
# Check if you have sufficient number of elements to form a square matrix
if
if
n
>
math
.
sqrt(array_len):
# sys.exit(message) raises an exception too
print
(
"Please provide sufficient number of elements for a square matrix. System ex
it."
)
sys
.
exit()
# Sum of the elements that will appear in the diagonal to give the trace
sum
= 0.0
for
for
k
in
in
range
(n):
sum
+=
#Your code here
return
return sum
In [5]:
# Show the output of the following
print
(
"Trace of the suggested matrix is:
{}
{}
"
.
format(trace1D(np
.
arange(
0
,
100
,
1
))))
Question 2
Question 2
Read an appropriate RGB-image of your choice in a 3-D Tensor named myRGB.
Perform the following operations on this tensor:
(A) Pad the image by 50 pixels on all sides. This operation is akin to putting a dark frame around the image.
Display this RGB image.
In [ ]:
# Follow the example from class. Modify it to the case of color images.
(B) Use a sliding window of a 3x3 matrix, K (referred to as a kernel), to perform an operation called convolution on
the original image. Display some of these images after convolution.
Note that you only need to use appropriate slicing of the source image, element wise product, and np.sum in
loops to perform this.
You have provided an array of length 100
Trace of the suggested matrix is: 495.0
Your Observations?
loops to perform this.
Display the images after convolutions using K=[1 0 -1;0 0 0;-1 0 1] and K = [0 -1 0; -1 4 -1; 0 -1 0]. Describe what
these convolutions have achieved.
To know more about convolution and how to achieve it, read Section 9.2 in
https://www.deeplearningbook.org/contents/convnets.html
.
Here is another resource on convolution:
https://developer.apple.com/library/archive/documentation/Performance/Conceptual/vImage/ConvolutionOperatio
In [ ]:
# Work with a B&W image.
myGray
=
# convert myRGB to monochromic
# Create a padded image from myGray by padding one pixel on all sides. Why?
myGray_padded
=
# Do it on myGray a B&W image
# If the convoluted image is called myGray_conv
myGray_conv
=
np
.
zeros_like(myGray)
# Python code for convolution that needs modification by you.
m,n
=
# Find dimension of the image
K
=
# Take appropriate Kernel
for
for
i
in
in
range
(m):
for
for
j
in
in
range
(n):
# Take appropriate slice of myGray_padded to get a (3x3) window on the image
myGray_window
=
# Your code here
# CODE for finding the i,j-th pixel on the convoluted image
myGray_conv[i,j]
=
# Your code here
# Display the convoluted image
Question 3
Question 3
IRIS flower dataset is one of the widely used resources. Load the IRIS data by using the code below. Normalize
this data by using z-scoring (z = (x - x_mean)/std). Don't use loops. You can use np.mean(), np.std() and basic
matrix operation with broadcasting. Visualize the distribution of this data using Matplotlib or other packages.
In [ ]:
# Python code
from
from
sklearn
sklearn
import
import
datasets
iris
=
datasets
.
load_iris()
.
data
In [ ]:
# Your code here
Question 4
Question 4
Modify the textual data example from NB1 to convert the document-term matrix into TF-IDF (term-frequency
inverse document frequency) matrix by using basic NumPy operations.
Term-frequency (TF)
Term-frequency (TF) (of a word in a document) is frequency of the word in a document divided by
total number of words in the document.
Inverse document frequency (IDF)
Inverse document frequency (IDF) of a word (all documents under consideration) is the natural log of
(total number of documents/number of documents having the given word).
See the discussion for an example
tf-idf(
t
,
d
) = tf(
t
,
d
) ⋅ idf(
t
)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
NumPy includes a submodule numpy.linalg where many functions are defined to allow
the user to do various things related to linear algebra. If A is a square matrix
represented as an object of type ndarray , the function eigvals () will return the
eigenvalues of A as an array. Which of the following code snippets would correctly
import the module and call this function with the matrix (A as input?
Note: There may be more than one correct answer! Select all that will work.
arrow_forward
CODE MUST BE IN OCAML LANGUAGE!
1. Create a type slidingTile, consisting of a char matrix and two integers x and y. The integers x and y are the coordinates of an empty tile.
2. Create a function val slide : slidingTile -> int -> int -> slidingTile = that given a slidingTile and two integers, that represent a location in the matrix, it slides the tile from the specified location to the empty tile, then returns the altered slidingTile. If provided location is not adjacent to the empty tile, or out of bounds, then return the slidingTile unaltered.
3. Create a function val print_tile : slidingTile -> unit = that prints a slidingTile on screen with the corresponding characters from the matrix. However, print an empty space where the empty tile is instead
# let a=...# print_tile a;;12345678# slide a 2 2;;# print_tile a;;12345678# slide a 3 2;;# print_tile a;;1234567 8# slide a 4 2;;# print_tile a;;1234567 8# slide a 2 2;;# print_tile a;;1234 6758
arrow_forward
Complete the function that adds 3 matrices together. The
matrices will be numpy type and of the same dimension of
3 x 3. There are several ways to do this, any correct
answer will be accepted.
[ ] # add the matrices a,b,c together, these will have
#been passed to the function
# as standard numpy matrices. Rememeber if you
#are unsure, just print the
# elements to see how to access the matrix values.
# there are several ways to do this, anyway
#you do this is fine
import numpy as np
def add_matrices (a,b,c):
# initiate the matrix based on the assumption
#that the matrix is 3x3.
matrix = np.zeros([3,3])
# YOUR CODE HERE
return matrix
## Check your code below using print command
## Random matrices are created here for checking
##your function
a = np.array([[3,2,1],[1,1,1],[1,1,1]])
b =
C =
np.array([[3,2,1], [2,2,2], [2,2,2]])
np.array([[3,3,3], [3,3,3], [3,3,3]])
arrow_forward
Write the function that returns the addresses of
the largest and smallest numbers in a matrix 20
that it takes as a parameter, the function
prototype is as follows, the return type from the
function is left blank, you define it appropriately
(navigate the matrix indices with pointer
addressing).
Function prototype:
„FindMinMaxAddress(int M, int col, int row)
arrow_forward
Create a program for Matrix with the following requirements:
1. Allow the user to select what operation to perform like: finding eigenvalues and eigenvectors, sketching the Gershgorin circles, and diagonalization.
2. Allow the user to input any size of the matrices and their elements.
3. Display the matrix input and the result of the operation.
arrow_forward
Write a function that returns the sum of all the elements in a specified column in a matrix using the following header:def sumColumn(m, columnIndex):
Write a test program that reads a 3x4 matrix and displays the sum of each column.
arrow_forward
Write a function that takes a matrix of order 3 and prints the transpose of it. The transpose should be stored in a different matrix.Test the function in main().For example:Matrix =1 2 34 5 67 8 9Transpose =1 4 72 5 83 6 9
write code for it in c++
arrow_forward
C++ code not Java
arrow_forward
Python problem
Problem StatementDevelop a function that receives an array and returns a tuple with its dimensions.
EntryMultiple lines, each line contains a list of numbers separated by a space and this line corresponds to a row in the matrix. At the end of the array, the character "*".
DepartureThe dimensions of the matrix in a tuple, (m, n) where m are the rows and n the columns. If it is not a valid array, the word "Error" must be printed without quotes.
Examples of
Input example 1
8 4 3 2 6 9 7 14 4 1 2 5 6 1 31 1 2 2 3 4 5 60 3 4 6 0 2 4 8*Output example 1
(4, 8)
Input example 2
1 6 8 9 5 4 1 2 5 4 8 25 3 5 6 9 8 2 9 6 3 4 66 2 4 8 6 9 1 0 3 61 2 3 4 5 6 7 8 9 1 1 2*Output example 2
Error
arrow_forward
c++ programming language
arrow_forward
I am trying to write a rotate function in C language.
arrow_forward
C language homework question :
arrow_forward
Write a function median_filter(img, s) that takes as its argument a numpy img array representing an image, and returns a numpy array obtained by applying to img the median filter. Again, the second argument s is the size of squares used by the filter.
arrow_forward
Write a function named lowerLeftQuarter that takes one argument, a matrix. The function then returns the lower left quarter of the input matrix as a new matrix. For example, if the input is 1 2 3 4 5 6 7 8 0 1 2 3 4 5 6 7 Then the following matrix should be returned. 0 1 4 5 If the input is 1 2 3 5 7 9 0 5 Then the following matrix should be returned. 7 9
arrow_forward
Matrix Multiplication• Write a multiplication function that accepts two 2D numpy arrays,and returns the product of the two. This function should test that thearrays are compatible (recall that the number of columns in the firstmatrix must equal the number of rows in the second matrix). Thisfunction should test the array sizes before attempting themultiplication. If the arrays are not compatible, the function shouldreturn -1.
solve in python
arrow_forward
In C programming:
Write a function printAllCourses() which receives an array of course pointers and the array’s size, then prints all courses in the array by calling printCourseRow()
arrow_forward
PROGRAMMING LANGUAGE: C++
Please test the code first before uploading here in bartleby. The expected outcome for each of the test case is also given in the screenshot. Follow accordingly. Thanks
arrow_forward
C++ please and not java
arrow_forward
Create a function named "onlyOdd".The function should accept a parameter named "numberArray".Use the higher order function filter() to create a new array that only containsthe odd numbers. Return the new array.// Use this array to test your function:const testingArray = [1, 2, 4, 17, 19, 20, 21];
arrow_forward
In statistics, the mode of a set of values is the value that occurs most often or with the greatest frequency. Write a function that accepts as arguments the following: A) An array of integers B) An integer that indicates the number of elements in the array The function should determine the mode of the array. That is, it should determine which value in the array occurs most often. The mode is the value the function should return. If the array has no mode (none of the values occur more than once), the function should return −1. (Assume the array will always contain non negative values.) Demonstrate your pointer prowess by using pointer notation instead of array notation in this function.
arrow_forward
Use pointers to write a function that finds the larg- est element in an array of integers. Use {6, 7, 9, 10, 15, 3, 99, -21} to test the function.
arrow_forward
ز٢
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
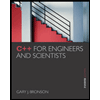
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Related Questions
- NumPy includes a submodule numpy.linalg where many functions are defined to allow the user to do various things related to linear algebra. If A is a square matrix represented as an object of type ndarray , the function eigvals () will return the eigenvalues of A as an array. Which of the following code snippets would correctly import the module and call this function with the matrix (A as input? Note: There may be more than one correct answer! Select all that will work.arrow_forwardCODE MUST BE IN OCAML LANGUAGE! 1. Create a type slidingTile, consisting of a char matrix and two integers x and y. The integers x and y are the coordinates of an empty tile. 2. Create a function val slide : slidingTile -> int -> int -> slidingTile = that given a slidingTile and two integers, that represent a location in the matrix, it slides the tile from the specified location to the empty tile, then returns the altered slidingTile. If provided location is not adjacent to the empty tile, or out of bounds, then return the slidingTile unaltered. 3. Create a function val print_tile : slidingTile -> unit = that prints a slidingTile on screen with the corresponding characters from the matrix. However, print an empty space where the empty tile is instead # let a=...# print_tile a;;12345678# slide a 2 2;;# print_tile a;;12345678# slide a 3 2;;# print_tile a;;1234567 8# slide a 4 2;;# print_tile a;;1234567 8# slide a 2 2;;# print_tile a;;1234 6758arrow_forwardComplete the function that adds 3 matrices together. The matrices will be numpy type and of the same dimension of 3 x 3. There are several ways to do this, any correct answer will be accepted. [ ] # add the matrices a,b,c together, these will have #been passed to the function # as standard numpy matrices. Rememeber if you #are unsure, just print the # elements to see how to access the matrix values. # there are several ways to do this, anyway #you do this is fine import numpy as np def add_matrices (a,b,c): # initiate the matrix based on the assumption #that the matrix is 3x3. matrix = np.zeros([3,3]) # YOUR CODE HERE return matrix ## Check your code below using print command ## Random matrices are created here for checking ##your function a = np.array([[3,2,1],[1,1,1],[1,1,1]]) b = C = np.array([[3,2,1], [2,2,2], [2,2,2]]) np.array([[3,3,3], [3,3,3], [3,3,3]])arrow_forward
- Write the function that returns the addresses of the largest and smallest numbers in a matrix 20 that it takes as a parameter, the function prototype is as follows, the return type from the function is left blank, you define it appropriately (navigate the matrix indices with pointer addressing). Function prototype: „FindMinMaxAddress(int M, int col, int row)arrow_forwardCreate a program for Matrix with the following requirements: 1. Allow the user to select what operation to perform like: finding eigenvalues and eigenvectors, sketching the Gershgorin circles, and diagonalization. 2. Allow the user to input any size of the matrices and their elements. 3. Display the matrix input and the result of the operation.arrow_forwardWrite a function that returns the sum of all the elements in a specified column in a matrix using the following header:def sumColumn(m, columnIndex): Write a test program that reads a 3x4 matrix and displays the sum of each column.arrow_forward
- Write a function that takes a matrix of order 3 and prints the transpose of it. The transpose should be stored in a different matrix.Test the function in main().For example:Matrix =1 2 34 5 67 8 9Transpose =1 4 72 5 83 6 9 write code for it in c++arrow_forwardC++ code not Javaarrow_forwardPython problem Problem StatementDevelop a function that receives an array and returns a tuple with its dimensions. EntryMultiple lines, each line contains a list of numbers separated by a space and this line corresponds to a row in the matrix. At the end of the array, the character "*". DepartureThe dimensions of the matrix in a tuple, (m, n) where m are the rows and n the columns. If it is not a valid array, the word "Error" must be printed without quotes. Examples of Input example 1 8 4 3 2 6 9 7 14 4 1 2 5 6 1 31 1 2 2 3 4 5 60 3 4 6 0 2 4 8*Output example 1 (4, 8) Input example 2 1 6 8 9 5 4 1 2 5 4 8 25 3 5 6 9 8 2 9 6 3 4 66 2 4 8 6 9 1 0 3 61 2 3 4 5 6 7 8 9 1 1 2*Output example 2 Errorarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
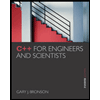
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr