prep5
.py
keyboard_arrow_up
School
University of Toronto *
*We aren’t endorsed by this school
Course
148
Subject
Computer Science
Date
Jan 9, 2024
Type
py
Pages
4
Uploaded by EarlPheasant3869
"""CSC148 Prep 5: Linked Lists
=== CSC148 Fall 2023 ===
Department of Computer Science,
University of Toronto
This code is provided solely for the personal and private use of
students taking the CSC148 course at the University of Toronto.
Copying for purposes other than this use is expressly prohibited.
All forms of distribution of this code, whether as given or with
any changes, are expressly prohibited.
Authors: David Liu and Diane Horton
All of the files in this directory and all subdirectories are:
Copyright (c) 2020 David Liu and Diane Horton
=== Module Description ===
This module contains the code for a linked list implementation with two classes,
LinkedList and _Node.
NOTE: There is an additional task in the prep5_starter_tests.py file.
"""
from __future__ import annotations
from typing import Any
from python_ta.contracts import check_contracts
@check_contracts
class _Node:
"""A node in a linked list.
Note that this is considered a "private class", one which is only meant
to be used in this module by the LinkedList class, but not by client code.
Attributes:
- item:
The data stored in this node.
- next:
The next node in the list, or None if there are no more nodes.
"""
item: Any
next: _Node | None
def __init__(self, item: Any) -> None:
"""Initialize a new node storing <item>, with no next node.
"""
self.item = item
self.next = None
# Initially pointing to nothing
@check_contracts
class LinkedList:
"""A linked list implementation of the List ADT.
Private Attributes:
- _first:
The first node in the linked list, or None if the list is empty.
"""
_first: _Node | None
def __init__(self) -> None:
"""Initialize an empty linked list.
"""
self._first = None
def print_items(self) -> None:
"""Print out each item in this linked list."""
curr = self._first
while curr is not None:
print(curr.item)
curr = curr.next
##########################################################################
# Part 1
#
# For each of the following linked list methods, read its docstring
# and then complete its implementation.
# You should use the provided *linked list traversal* code template
# as your starting point, but of course you should modify it as necessary!
#
# NOTE: the first two methods are new special methods (you can tell by the
# double underscores), and enable some special Python behaviour that we've
# illustrated in the doctests.
#
# At the bottom of this file, we've included some helpers
# to create some basic linked lists for our doctests.
##########################################################################
def __len__(self) -> int:
"""Return the number of elements in this list.
>>> lst = LinkedList()
>>> len(lst)
# Equivalent to lst.__len__()
0
>>> lst = three_items(1, 2, 3)
>>> len(lst)
3
"""
i = 0
curr = self._first
while curr is not None:
i += 1
curr = curr.next
return i
def __contains__(self, item: Any) -> bool:
"""Return whether <item> is in this list.
Use == to compare items.
>>> lst = three_items(1, 2, 3)
>>> 2 in lst
# Equivalent to lst.__contains__(2)
True
>>> 4 in lst
False
"""
curr = self._first
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
In the linked list below, find the value of "TEMP->Next->Data".
list1
a.
David
b.
Leah
c.
Robert
d.
Miriam
arrow_forward
2. Each student at Middlesex County College takes a different number of courses, so the
registrar has decided to use linear linked lists to store each student's class schedule
and an array to represent the entire student body.
A portion of this data structure is shown below:
link
Sec cr
CSC16213
→HISHO 24
$||1||
1/1234
2/2 357
CSC236/4
37
These data show that the first student (ID: 1111) is taking section 1 of CSC162 for 3
credits and section 2 of HIS101 for 4 credits; the second student is not enrolled; the
third student is enrolled in CSC236 section 4 for 3 credits.
s
Write a class for this data structure. Provide methods for creating the original array,
inserting a student's initial class schedule, adding a course, and dropping a course. Include
a menu-driven program that uses the class.
arrow_forward
Its a java data structures class. Lab directions is also given on second page thank you
arrow_forward
Project 3: Doubly-Linked List
The purpose of this assignment is to assess your ability to:
▪Implement sequential search algorithms for linked list structures.
▪Implement sequential abstract data types using linked data
▪Analyze and compare algorithms for efficiency using Big-O notation
Use the DLinkedList provided in class. You must not change any of the existing code in this file! You will add your code to this file.
Add the following methods:
A remove() method that removes a single node containing the value passed in as a parameter from the list. Method signature is provided and may not be changed.
An insertOrder() method that inserts a value into the list in such a way that the list remains sorted in ascending order. Method signature is provided and may not be changed.
An insertOrderUnique() method that does the same as insertOrder() with the additional requirement that the value is only inserted in the list if it is unique. Method signature is provided and may not be changed.
A…
arrow_forward
question 2:
import requests
from bs4 import BeautifulSoup
import pandas as pd
url = "https://en.wikipedia.org/wiki/Greenhouse_gas"
res = requests.get(url)
soup = BeautifulSoup(res.content, "html.parser")
# Find the table of interest
table = soup.find("table", {"class": "wikitable sortable"})
# Extract the header and data rows
header = [th.text.strip() for th in table.find("tr").find_all("th")]
data = [[td.text.strip() for td in tr.find_all("td")] for tr in table.find_all("tr")[1:]]
# Create the DataFrame
df = pd.DataFrame(data, columns=header)
import requestsfrom bs4 import BeautifulSoupimport pandas as pd
url = "https://en.wikipedia.org/wiki/Greenhouse_gas"res = requests.get(url)soup = BeautifulSoup(res.content, "html.parser")
# Find the table of interesttable = soup.find("table", {"class": "wikitable sortable"})
# Extract the header and data rowsheader = [th.text.strip() for th in table.find("tr").find_all("th")]data = [[td.text.strip() for td in tr.find_all("td")] for tr in…
arrow_forward
question 2:
import requests
from bs4 import BeautifulSoup
import pandas as pd
url = "https://en.wikipedia.org/wiki/Greenhouse_gas"
res = requests.get(url)
soup = BeautifulSoup(res.content, "html.parser")
# Find the table of interest
table = soup.find("table", {"class": "wikitable sortable"})
# Extract the header and data rows
header = [th.text.strip() for th in table.find("tr").find_all("th")]
data = [[td.text.strip() for td in tr.find_all("td")] for tr in table.find_all("tr")[1:]]
# Create the DataFrame
df = pd.DataFrame(data, columns=header)
import requestsfrom bs4 import BeautifulSoupimport pandas as pd
url = "https://en.wikipedia.org/wiki/Greenhouse_gas"res = requests.get(url)soup = BeautifulSoup(res.content, "html.parser")
# Find the table of interesttable = soup.find("table", {"class": "wikitable sortable"})
# Extract the header and data rowsheader = [th.text.strip() for th in table.find("tr").find_all("th")]data = [[td.text.strip() for td in tr.find_all("td")] for tr in…
arrow_forward
in c++
Create a single linked list that contains the data (age) of your friends. Perform basic operations including insertion, deletion, searching and display. The insertion operation should only allow a friend’s data to be inserted in sorted order only.
arrow_forward
There are some differences between the linked list and the array but the main difference between the list and the array is
a.
List is a dynamic data structure while an array is also dynamic data
b.
Array is a dynamic data structure while a List is a static data because it has a fixed size
c.
List is a static data structure while an array is also a static data because it has a variable size
d.
List is a dynamic data structure while an array is a static data because it has a fixed size
arrow_forward
PARTS file with Part# as the hash key (K) includes records with the following Part# values:2360, 3760, 5232, 4692, 4871, 2659, 1821, 3074, 7115, 1620, 2428, 1943,
4750, 2975, 4981, 1111, 3123, 3211, 5654, and 2208. The hash file has 30 cells , numbered 0 to 29. Eachcell holds one record.
Calculate the average search length for searching all entries.
arrow_forward
The stack that based on linked list has
a.
Two data attributes
b.
One data attribute
c.
No data attributes
d.
Three data attributes
arrow_forward
Attach File
Browse My Computer
QUESTION 2
- use Eclipse to create a project.
create a new class containing a main function.
- Create a function describing the bubble sort to sort a LinkedList of Integers in the decreasing order (from the largest number to the smallest number). The
function should accept a LinkedList and returm a LinkedLlist. Add also statements to trace the progress of bubble sort to display the LinkedList contents after each
exchange.
The main function should contain at least two test examples of the bubble sort function that you have create on various ordered and random LinkedList of
Integers.
NB: Send only the Java file containing your work.
If the program dose not compile, marks will be reduced.
if the program dose not properly as described above, marks will be reduced.
Attach File
Browse My Computer
Click Save and Submit to save and submit. Click Save All Answers to save all answers.
XI 門
arrow_forward
return_dict() that takes the name of a CSV file as the input parameter and returns a dictionary, where each key is the Reporting_PHU_ID and the value is a list containing the following data.
name of CSV file is "data23.csv"
arrow_forward
Lect 1A: linked-list
HW 1 link list : Create Visual C++ project and.
Due Tomorrow, 4:00 AM
Posted 3:19 PM
Project name should be 'HW1+student name for example 'HW1 Ahmed Ali Ahmed'
18
-Compress the project file as zip file and upload it here.
-Screenshots for the entire codes and classes
Turned in
Assigned
-Screenshot for the result.
View assignment
تسجبل محاطرة يوم 5/5/2021
Posted 3:14 PM
...
arrow_forward
3. By python Create a list dynamically in Python. It should have data items of all datatypes. The size should be 10.
arrow_forward
The stack that based on linked list has Select one: a. No data attributes b. Two data attributes c. One data attribute d. Three data attributes
arrow_forward
Inserting Nodes into the List:
In the following code (The Code is written By C) : there are 3 functions :
display, insert_begin, and delete.
The Request is : Modify the code and add two functions with comments :
First Function (insert_last) : to insert values from last.
Second Function (insert_mid): to insert values from Middle.
Please Run the code before send it... thanks...
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
The code:
#include<stdio.h>#include<string.h>#include<stdlib.h>#include<math.h>
struct node{int info;struct node* next;};
struct node* start;
void display();void insert_begin();
void delete();
int main(){
int choice;start = NULL;
while (1){printf("\n\t\t MENU \t\t\n");printf("\n1.Display\n");printf("\n2.Insert at the…
arrow_forward
get_total_cases() takes the a 2D-list (similar to database) and an integer x from this set {0, 1, 2} as input parameters. Here, 0 represents Case_Reported_Date, 1 represents Age_Group and 2 represents Client_Gender (these are the fields on the header row, the integer value represents the index of each of these fields on that row). This function computes the total number of reported cases for each instance of x in the text file, and it stores this information in a dictionary in this form {an_instance_of_x : total_case}. Finally, it returns the dictionary and the total number of all reported cases saved in this dictionary.
arrow_forward
In this project, you will implement a single linked list structure that represents a list of
Tawjihi records and do different operations on the records within that linked list. The inputs
for the project will be the two Tawjihi files (One file for West Bank and the other for Gaza
records). Each line in those files contains the Tawjihi record of a student (seat number,
average, and branch). You may use the code you wrote/implemented during the lectrure
to create and store Tawjihi record data into your single Linked List(s). You are free to use
as many linked lists as needed.
YOU MAY NOT USE ARRAYS IN THIS PROJECT.
For good user experience, you will need to implement a user interface.
In the user interface there should be a way to select between WestBank or Gaza as well as an
option to select between branchs "Literary or Science". According to the previouse selction the
following functions will operate in the specfic selected data above:
1. An option to insert new Tawjihi record into the…
arrow_forward
When a new node is inserted into an array based list at an index, which of the following is FALSE?
Select one:
A.All elements from the index to the end of the list will be shifted to one higher value
B.The size of the array will be increased to accommodate the new element
C.The new element will be placed in the array at the next position after the specified index
D.The element at the index position will be replaced with the newly inserted element
arrow_forward
https://panasonickitchen.com/robots.txt
arrow_forward
Define the function number_in_trophic_class(tli3_values, classification) which takes a list of trophic level index values and a trophic classification, returning the number of lakes in that trophic class. For this version of the question you MUST use a list comprehension. You cannot use a for loop or while loop in your answer.
Notes:
You must include, and use/call your trophic_class function in your submission.
Your tropic_class function must work in the same way it did for Question 2.
You can assume that classification will always be valid, i.e. it will always be one of the states in the following list:
['Hypertrophic', 'Supertrophic', 'Eutrophic', 'Mesotrophic', 'Oligotrophic', 'Microtrophic', 'Ultra-microtrophic']
There are several ways to proceed, but you will probably find either the len function or the count method of the list class useful in conjunction with your list comprehension.
For example:
Test
Result
tli3_list_1 = [4.1061, 2.54561, 4.16276, 2.33801,…
arrow_forward
Artificial intelligence subject:
Question 3: Create a dataframe having students list as indexes while marks in form of columns.
Add a column having total marks of students
students = ['std1','std2','std3'], phy = [43,51,72], maths = [33,56,73], bio = [54,51,42]
arrow_forward
import json, datetime, csvfrom pprint import pprint
Question 3 - Using the cleaned list of lists from question 1, create a dictionary of dictionaries where each key in the outer dictionary is an author whose last name starts with the letter 'S'. - Each inner dictionary should contain the number of books that author has written as 'Number of Books' - It should contain the earliest book title for that author based on year with the key 'First Book' - Finally it should contain the latest book title for that author based on year with the key 'Last Book' - If an author has only one book put the same title in both places - Return the dictionary of dictionaries
def author_history(cleaned_list):
Args: cleaned_list (list) Returns: dictionary of dictionaries
Output: {'SafranFoer,Jonathan': {'First Book': 'ExtremelyLoudandIncrediblyClose', 'Last Book': 'ExtremelyLoudandIncrediblyClose',…
arrow_forward
Programming
arrow_forward
Computer Science
Build up a web application for product catalogue for a book, Write a PHP program to create a hyper linked list of programming languages covered in that book. By clicking the hyperlink send the user to titles of the text books related to the selected programming languages
php code.
arrow_forward
From the following code that collects the data entered by the user of the animals according to their type of food. Pastebin: https://pastebin.com/VuBfRp0s
I have an error when deleting animals. It asks me for the code and when I enter it, it says that it deletes the animal. However, it does not disappear from the list and the same animal continues to be printed. What I can do?
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
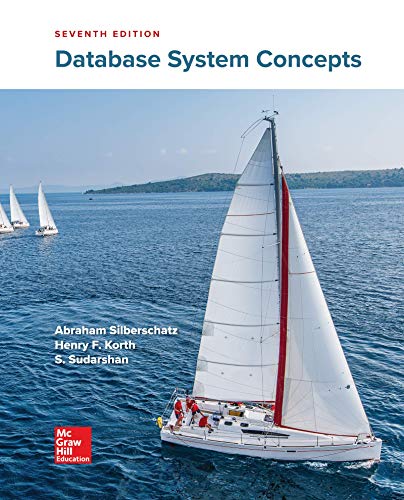
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
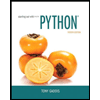
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
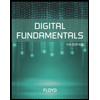
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
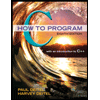
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
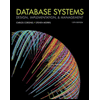
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
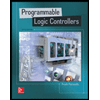
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- In the linked list below, find the value of "TEMP->Next->Data". list1 a. David b. Leah c. Robert d. Miriamarrow_forward2. Each student at Middlesex County College takes a different number of courses, so the registrar has decided to use linear linked lists to store each student's class schedule and an array to represent the entire student body. A portion of this data structure is shown below: link Sec cr CSC16213 →HISHO 24 $||1|| 1/1234 2/2 357 CSC236/4 37 These data show that the first student (ID: 1111) is taking section 1 of CSC162 for 3 credits and section 2 of HIS101 for 4 credits; the second student is not enrolled; the third student is enrolled in CSC236 section 4 for 3 credits. s Write a class for this data structure. Provide methods for creating the original array, inserting a student's initial class schedule, adding a course, and dropping a course. Include a menu-driven program that uses the class.arrow_forwardIts a java data structures class. Lab directions is also given on second page thank youarrow_forward
- Project 3: Doubly-Linked List The purpose of this assignment is to assess your ability to: ▪Implement sequential search algorithms for linked list structures. ▪Implement sequential abstract data types using linked data ▪Analyze and compare algorithms for efficiency using Big-O notation Use the DLinkedList provided in class. You must not change any of the existing code in this file! You will add your code to this file. Add the following methods: A remove() method that removes a single node containing the value passed in as a parameter from the list. Method signature is provided and may not be changed. An insertOrder() method that inserts a value into the list in such a way that the list remains sorted in ascending order. Method signature is provided and may not be changed. An insertOrderUnique() method that does the same as insertOrder() with the additional requirement that the value is only inserted in the list if it is unique. Method signature is provided and may not be changed. A…arrow_forwardquestion 2: import requests from bs4 import BeautifulSoup import pandas as pd url = "https://en.wikipedia.org/wiki/Greenhouse_gas" res = requests.get(url) soup = BeautifulSoup(res.content, "html.parser") # Find the table of interest table = soup.find("table", {"class": "wikitable sortable"}) # Extract the header and data rows header = [th.text.strip() for th in table.find("tr").find_all("th")] data = [[td.text.strip() for td in tr.find_all("td")] for tr in table.find_all("tr")[1:]] # Create the DataFrame df = pd.DataFrame(data, columns=header) import requestsfrom bs4 import BeautifulSoupimport pandas as pd url = "https://en.wikipedia.org/wiki/Greenhouse_gas"res = requests.get(url)soup = BeautifulSoup(res.content, "html.parser") # Find the table of interesttable = soup.find("table", {"class": "wikitable sortable"}) # Extract the header and data rowsheader = [th.text.strip() for th in table.find("tr").find_all("th")]data = [[td.text.strip() for td in tr.find_all("td")] for tr in…arrow_forwardquestion 2: import requests from bs4 import BeautifulSoup import pandas as pd url = "https://en.wikipedia.org/wiki/Greenhouse_gas" res = requests.get(url) soup = BeautifulSoup(res.content, "html.parser") # Find the table of interest table = soup.find("table", {"class": "wikitable sortable"}) # Extract the header and data rows header = [th.text.strip() for th in table.find("tr").find_all("th")] data = [[td.text.strip() for td in tr.find_all("td")] for tr in table.find_all("tr")[1:]] # Create the DataFrame df = pd.DataFrame(data, columns=header) import requestsfrom bs4 import BeautifulSoupimport pandas as pd url = "https://en.wikipedia.org/wiki/Greenhouse_gas"res = requests.get(url)soup = BeautifulSoup(res.content, "html.parser") # Find the table of interesttable = soup.find("table", {"class": "wikitable sortable"}) # Extract the header and data rowsheader = [th.text.strip() for th in table.find("tr").find_all("th")]data = [[td.text.strip() for td in tr.find_all("td")] for tr in…arrow_forward
- in c++ Create a single linked list that contains the data (age) of your friends. Perform basic operations including insertion, deletion, searching and display. The insertion operation should only allow a friend’s data to be inserted in sorted order only.arrow_forwardThere are some differences between the linked list and the array but the main difference between the list and the array is a. List is a dynamic data structure while an array is also dynamic data b. Array is a dynamic data structure while a List is a static data because it has a fixed size c. List is a static data structure while an array is also a static data because it has a variable size d. List is a dynamic data structure while an array is a static data because it has a fixed sizearrow_forwardPARTS file with Part# as the hash key (K) includes records with the following Part# values:2360, 3760, 5232, 4692, 4871, 2659, 1821, 3074, 7115, 1620, 2428, 1943, 4750, 2975, 4981, 1111, 3123, 3211, 5654, and 2208. The hash file has 30 cells , numbered 0 to 29. Eachcell holds one record. Calculate the average search length for searching all entries.arrow_forward
- The stack that based on linked list has a. Two data attributes b. One data attribute c. No data attributes d. Three data attributesarrow_forwardAttach File Browse My Computer QUESTION 2 - use Eclipse to create a project. create a new class containing a main function. - Create a function describing the bubble sort to sort a LinkedList of Integers in the decreasing order (from the largest number to the smallest number). The function should accept a LinkedList and returm a LinkedLlist. Add also statements to trace the progress of bubble sort to display the LinkedList contents after each exchange. The main function should contain at least two test examples of the bubble sort function that you have create on various ordered and random LinkedList of Integers. NB: Send only the Java file containing your work. If the program dose not compile, marks will be reduced. if the program dose not properly as described above, marks will be reduced. Attach File Browse My Computer Click Save and Submit to save and submit. Click Save All Answers to save all answers. XI 門arrow_forwardreturn_dict() that takes the name of a CSV file as the input parameter and returns a dictionary, where each key is the Reporting_PHU_ID and the value is a list containing the following data. name of CSV file is "data23.csv"arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
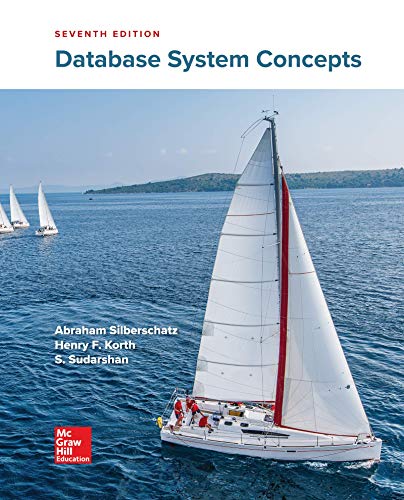
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
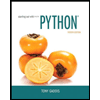
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
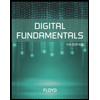
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
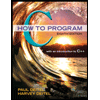
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
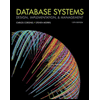
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
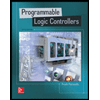
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education