09 randArray Part 4 DESCRIPTION Get a value from the user and using a loop, search for the value in the array. Report all the index locations where it is found Report if it is not found anywhere #include <iostream>using namespace std;#include <cstdlib> int main(){ srand(17); const int ARRAYSIZE = 20; int RandArray[ARRAYSIZE]; int i; for (i = 0; i < ARRAYSIZE; i++) RandArray[i] = rand() % 100; for (i = 0; i < ARRAYSIZE; i++) cout <<"randArray["<< i <<"]=" << RandArray[i] << endl; int smallestFoundSoFar=90;int indexOfSmallest = -1;int largestFoundSoFar=-1;int indexOfLargest = -1; for (i = 0; i < ARRAYSIZE; i++) { if (RandArray[i] > largestFoundSoFar) { largestFoundSoFar=RandArray[i]; indexOfLargest = i; } } cout << "\nlargestFoundSoFar=" << largestFoundSoFar << " at index " << indexOfLargest; for (i = 0; i < ARRAYSIZE; i++) { if (RandArray[i] < smallestFoundSoFar) { smallestFoundSoFar=RandArray[i]; indexOfSmallest = i; } } cout << "\nsmallestFoundSoFar=" << smallestFoundSoFar<< " at index " << indexOfSmallest; return 0;}
09 randArray Part 4
DESCRIPTION
- Get a value from the user and using a loop, search for the value in the array.
- Report all the index locations where it is found
- Report if it is not found anywhere
#include <iostream>
using namespace std;
#include <cstdlib>
int main()
{
srand(17);
const int ARRAYSIZE = 20;
int RandArray[ARRAYSIZE];
int i;
for (i = 0; i < ARRAYSIZE; i++)
RandArray[i] = rand() % 100;
for (i = 0; i < ARRAYSIZE; i++)
cout <<"randArray["<< i <<"]=" << RandArray[i] << endl;
int smallestFoundSoFar=90;
int indexOfSmallest = -1;
int largestFoundSoFar=-1;
int indexOfLargest = -1;
for (i = 0; i < ARRAYSIZE; i++)
{
if (RandArray[i] > largestFoundSoFar)
{
largestFoundSoFar=RandArray[i];
indexOfLargest = i;
}
}
cout << "\nlargestFoundSoFar=" << largestFoundSoFar << " at index " << indexOfLargest;
for (i = 0; i < ARRAYSIZE; i++)
{
if (RandArray[i] < smallestFoundSoFar)
{
smallestFoundSoFar=RandArray[i];
indexOfSmallest = i;
}
}
cout << "\nsmallestFoundSoFar=" << smallestFoundSoFar<< " at index " << indexOfSmallest;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

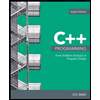
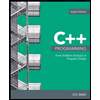