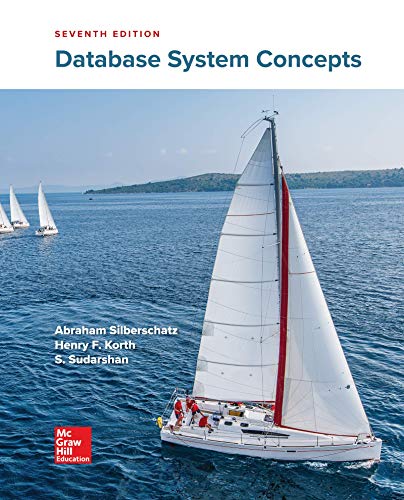
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Java - Variables, input, and casting
![(1) Prompt the user to input an integer, a double, a character, and a string, storing each into separate variables. Then, output those four
values on a single line separated by a space. (2 pts)
Note: This zyLab outputs a newline after each user-input prompt. For convenience in the examples below, the user's input value is shown on
the next line, but such values don't actually appear as output when the program runs. Use next() to read String, not nextLine().
Enter integer:
99
Enter double:
3.77
Enter character:
z
Enter string:
Howdy
99 3.77 z Howdy
(2) Extend to also output in reverse. (1 pt)
Enter integer:
99
Enter double:
3.77
Enter character:
z
Enter string:
Howdy
99 3.77 z Howdy
Howdy z 3.77 99
(3) Extend to cast the double to an integer, and output that integer. (2 pts)
Enter integer:
99
Enter double:
3.77
Enter character:
z
Enter string:
Howdy
99 3.77 z Howdy
Howdy z 3.77 99
3.77 cast to an integer is 3
1 import java.util.Scanner;
2
3 public class BasicInput
4
5
7
8
9
10
11
12
13
14
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
15
16
17
18 }
19}
int userInt;
double userDouble;
// FIXME Define char and string variables similarly
System.out.println("Enter integer: ");
userInt= scnr.nextInt ();
// FIXME (1): Finish reading other items into variables, then output the four values on a single Line separated
// FIXME (2): Output the four values in reverse
// FIXME (3): Cast the double to an integer, and output that integer](https://content.bartleby.com/qna-images/question/d863c081-8226-42e9-a15c-9cd471d16ef3/5b874390-d78a-431a-889c-b70eb665b2d9/rzm0yjr_thumbnail.png)
Transcribed Image Text:(1) Prompt the user to input an integer, a double, a character, and a string, storing each into separate variables. Then, output those four
values on a single line separated by a space. (2 pts)
Note: This zyLab outputs a newline after each user-input prompt. For convenience in the examples below, the user's input value is shown on
the next line, but such values don't actually appear as output when the program runs. Use next() to read String, not nextLine().
Enter integer:
99
Enter double:
3.77
Enter character:
z
Enter string:
Howdy
99 3.77 z Howdy
(2) Extend to also output in reverse. (1 pt)
Enter integer:
99
Enter double:
3.77
Enter character:
z
Enter string:
Howdy
99 3.77 z Howdy
Howdy z 3.77 99
(3) Extend to cast the double to an integer, and output that integer. (2 pts)
Enter integer:
99
Enter double:
3.77
Enter character:
z
Enter string:
Howdy
99 3.77 z Howdy
Howdy z 3.77 99
3.77 cast to an integer is 3
1 import java.util.Scanner;
2
3 public class BasicInput
4
5
7
8
9
10
11
12
13
14
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
15
16
17
18 }
19}
int userInt;
double userDouble;
// FIXME Define char and string variables similarly
System.out.println("Enter integer: ");
userInt= scnr.nextInt ();
// FIXME (1): Finish reading other items into variables, then output the four values on a single Line separated
// FIXME (2): Output the four values in reverse
// FIXME (3): Cast the double to an integer, and output that integer
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- COMPUTER FUNDAMENTALS AND PROGRAMMING 2 USING JAVA CODES Input the temperature, and determine whether or not it is within the typical range for that temperature.arrow_forwardName:- MIT Note:- Please type this java code and also need an output for this given program.arrow_forwardjava If you open a command window and want to see what version of the Java compiler you have installed on your computer, what would you write? If you open a command window and want to see what version of Java you have installed, what would you need to write?arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
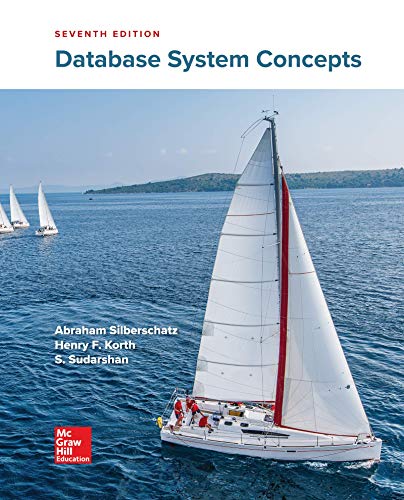
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
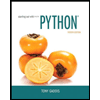
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
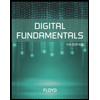
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
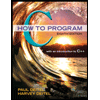
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
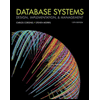
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
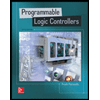
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education