(1) The given program outputs a fixed-height triangle using a * character. Modify the given program to output a right triangle that instead uses the user-specified triangleChar character. (2) Modify the program to use a nested loop to output a right triangle of height triangleHeight. The first line will have one user-specified character, such as % or *. Each subsequent line will have one additional user-specified character until the number in the triangle's base reaches triangleHeight. Output a space after each user-specified character, including after the line's last user-specified character. Example output for triangleChar = % and triangleHeight = 5:
This program will output a right triangle based on user specified height triangleHeight and symbol triangleChar. It is strongly suggested to use the for loop for this solution. https://www.tutorialspoint.com/cprogramming/cnestedloops.htm
(1) The given program outputs a fixed-height triangle using a * character. Modify the given program to output a right triangle that instead uses the user-specified triangleChar character.
(2) Modify the program to use a nested loop to output a right triangle of height triangleHeight. The first line will have one user-specified character, such as % or *. Each subsequent line will have one additional user-specified character until the number in the triangle's base reaches triangleHeight. Output a space after each user-specified character, including after the line's last user-specified character.
Example output for triangleChar = % and triangleHeight = 5:
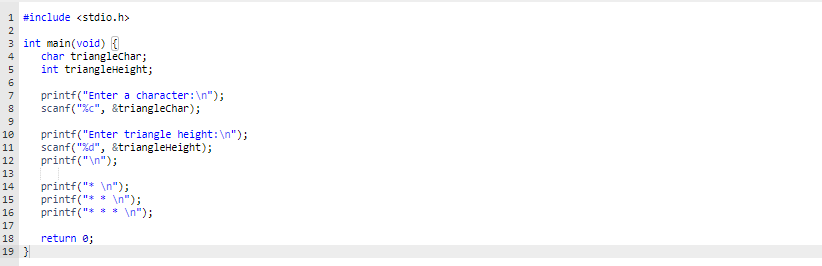
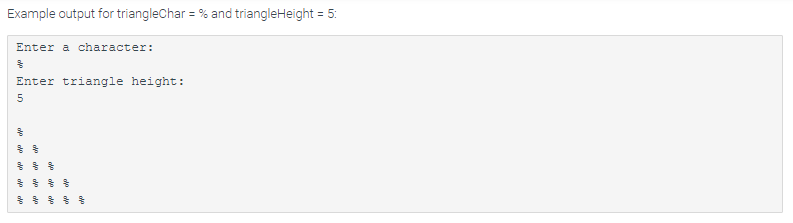

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

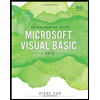
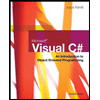
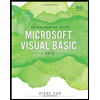
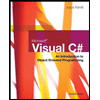