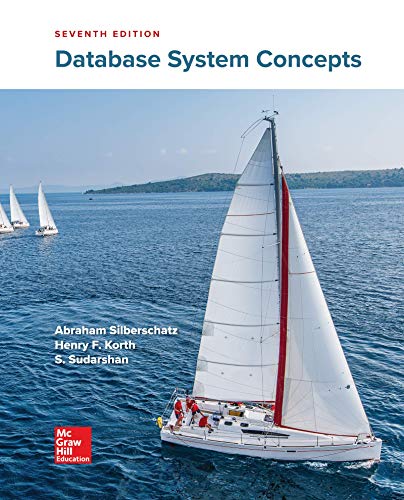
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
(1) What's the Time
Build a Python script that tells you the current time.- Create a new script called current_time.py in Jupyter or a text editor.
- Add a docstring to the script to explain what it does.
- Import the datetime module.
- Get the current time using datetime.now().
- Print the result, but only if the script is to be executed. *** hint, __name__==__main__
- Execute the script in the terminal to check if it prints the time.
- Import the time into a Python console and check if the console output does not print the time.
The output of the terminal should be in this format: HH:MM:SS.SSSSSS
In [ ]:
(2) Formatting Customer Names
Build a function that displays a customer's name and location if applicableSuppose that you are building a Customer Relationship Management (CRM) system, and you want to display a user record in the following format: John Smith (California). However, if you don't have a location in your system, you just want to see "John Smith." Create a format_customer() function that takes two required positional arguments, first_ name and last_name, and one optional keyword argument, location. It should return a string in the required format.
- Create the customer.py file.
- Define the format_customer() function.
- Open a Python shell (Or Jupyter Notebook) and import your format_customer() function.
- Try running a few examples. The calls should look like this:
from customer import format_customer
format_customer('John', 'Smith', location='California')and the output should look like this:
John Smith (California)
In [ ]:
(3) The Fibonacci Function with an Iteration
Calculate a Fibonacci sequenceYou work in an IT firm, and your colleague has realized that being able to quickly compute elements of the Fibonacci sequence will reduce the time taken to execute the testing suite on one of your internal applications. You will use an iterative approach to create a fibonacci_iterative function that returns the nth value in the Fibonacci sequence.
- Create a fibonacci.py file.
- Define a fibonacci_iterative function that takes a single positional argument representing which number term in the sequence you want to return.
- Run the following code:
from fibonacci import fibonacci_iterative
fibonacci_iterative(3)
You should get the following output:
2
Another example to test your code can be as mentioned in the following code snippet:
fibonacci_iterative(10)
You should get the following output:
55
In [ ]:
(4) The Fibonnaci Function with Recursion
Remake your previous fibonacci program as a recursive functionIn [ ]:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In Python: So this is part of a large project I am working on but I'm having a hard time finding out how to read from one text file and then correct that information to another or same txt file as well as to do it alphabetically. Any help is appreciated. The code I've added was from a previous project that was similar as to what this one wants. I can't use custom classes and I believe I'm supposed to be opening the bad information file, writing it and corrections to a temp file and then creating a new file with the right information. I'm in a beginner class and we haven't covered anything past lists. Problem #1: How much should I study outside of class? Issue: Your fellow students liked the 2nd version of study hour’s application and want to expand it again by adding the features listed below. Minimum Study Hours per Week per Class Grade 15 A 12…arrow_forwardPasswords that are created by users are usually very simple and easy to guess. Create a Python program that takes a simpler pswrd + makes it stronger by taking characters using the rules below, and by appending "!" to the ending of the input string. i becomes 1 a becomes @ m becomes M B becomes 8 s becomes $ Example: If the input is: mypassword the output is: Myp@$$word! Hint: Python strings are immutable, but support string concatenation. Store and build the stronger password in the given password variable This is what I have so far.... word = input()password = ''arrow_forwardAnswer the given question with a proper explanation and step-by-step solution. In Python, please.arrow_forward
- The class I'm taking is assembly programming. I have attached the instructions. i have also attached my work. Can you please tell me how to fix it so I can successfully run and compile program in visual studio . i keep getting syntax errors on line 11 and 12. Thank you so much for your help!arrow_forwardAssignment First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects). Then create a new Java application called "MultiTable" (without the quotation marks) that uses nested for loops to print a multiplication table as shown below. The value of each cell should be computed using the values in the row and column headings (e.g., 1 * 1; 1 * 2; 1 * 3; etc.). Make sure to match the below results as shown. * | 1 2 3 4 5 6 7 8 9 ------------------------------- 1 | 1 2 3 4 5 6 7 8 9 2 | 2 4 6 8 10 12 14 16 18 3 | 3 6 9 12 15 18 21 24 27 4 | 4 8 12 16 20 24 28 32 36 5 | 5 10 15 20 25 30 35 40 45 6 | 6 12 18 24 30 36 42 48 54 7 | 7 14 21 28 35 42 49 56 63 8 | 8 16 24 32 40 48 56 64 72 9 | 9 18 27 36 45 54 63 72 81Copy and paste at least one sample run in your source code as a block comment at the bottom below the closing curly brace.arrow_forwardFirst, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects). Then create a new Java application called "MultiTable" (without the quotation marks) that uses nested for loops to print a multiplication table as shown below. The value of each cell should be computed using the values in the row and column headings (e.g., 1 * 1; 1 * 2; 1 * 3; etc.). Make sure to match the below results as shown. * | 1 2 3 4 5 6 7 8 9 ------------------------------- 1 | 1 2 3 4 5 6 7 8 9 2 | 2 4 6 8 10 12 14 16 18 3 | 3 6 9 12 15 18 21 24 27 4 | 4 8 12 16 20 24 28 32 36 5 | 5 10 15 20 25 30 35 40 45 6 | 6 12 18 24 30 36 42 48 54 7 | 7 14 21 28 35 42 49 56 63 8 | 8 16 24 32 40 48 56 64 72 9 | 9 18 27 36 45 54 63 72 81Copy and paste at least one sample run in your source code as a block comment at the bottom below the closing curly brace.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
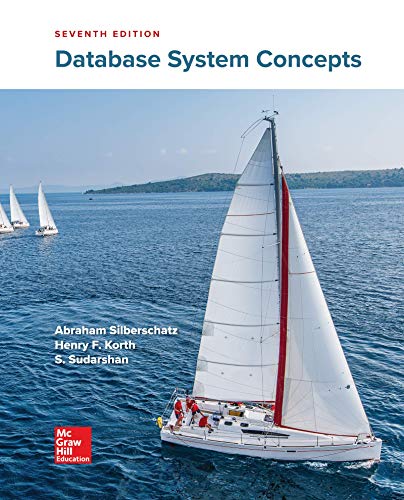
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
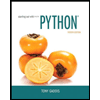
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
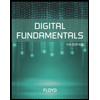
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
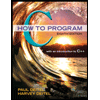
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
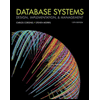
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
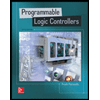
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education