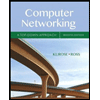
SKELETON CODE IS PROVIDED ALONG WITH C AND H FILES.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#include "node.h"
#include "stack_functions.h"
#define NUM_VERTICES 10
/** This function takes a pointer to the
adjacency matrix of a Graph and the
size of this matrix as arguments and
prints the matrix
*/
void print_graph(int * graph, int size);
/** This function takes a pointer to the
adjacency matrix of a Graph, the size
of this matrix, the source and dest
node numbers along with the weight or
cost of the edge and fills the adjacency
matrix accordingly.
*/
void add_edge(int * graph, int size, int src, int dst, int cost);
/** This function takes a pointer to the adjacency matrix of
a graph, the size of this matrix, source and destination
vertex numbers as inputs and prints out the path from the
source vertex to the destination vertex. It also prints
the total cost of this path.
*/
void find_path_dfs(int * graph, int size, int src, int dst);
int main()
{
int my_graph[NUM_VERTICES][NUM_VERTICES]; /// An adjacency matrix representation of graph
memset(my_graph,-1,NUM_VERTICES * NUM_VERTICES * sizeof(int)); /// Initiallize with -1 representing infinte cost.
for(int i=0; i<NUM_VERTICES; i++)
add_edge(&my_graph[0][0], NUM_VERTICES, i, i, 0); /// All the vertices have a cost of 0 for visiting themselves
add_edge(&my_graph[0][0], NUM_VERTICES, 0, 3, 13);
add_edge(&my_graph[0][0], NUM_VERTICES, 1, 4, 14);
add_edge(&my_graph[0][0], NUM_VERTICES, 0, 3, 13);
add_edge(&my_graph[0][0], NUM_VERTICES, 1, 4, 14);
add_edge(&my_graph[0][0], NUM_VERTICES, 1, 5, 6);
add_edge(&my_graph[0][0], NUM_VERTICES, 2, 3, 9);
add_edge(&my_graph[0][0], NUM_VERTICES, 2, 9, 10);
add_edge(&my_graph[0][0], NUM_VERTICES, 3, 0, 13);
add_edge(&my_graph[0][0], NUM_VERTICES, 3, 8, 9);
add_edge(&my_graph[0][0], NUM_VERTICES, 4, 1, 14);
add_edge(&my_graph[0][0], NUM_VERTICES, 4, 6, 8);
add_edge(&my_graph[0][0], NUM_VERTICES, 5, 1, 6);
add_edge(&my_graph[0][0], NUM_VERTICES, 5, 2, 7);
add_edge(&my_graph[0][0], NUM_VERTICES, 5, 6, 12);
add_edge(&my_graph[0][0], NUM_VERTICES, 6, 4, 8);
add_edge(&my_graph[0][0], NUM_VERTICES, 6, 7, 15);
add_edge(&my_graph[0][0], NUM_VERTICES, 7, 6, 15);
add_edge(&my_graph[0][0], NUM_VERTICES, 8, 5, 11);
add_edge(&my_graph[0][0], NUM_VERTICES, 9, 8, 23);
/** Task 1: Complete the rest of the Adjacency Matrix according to Figure 8 **/
print_graph(&my_graph[0][0], NUM_VERTICES);
find_path_dfs(&my_graph[0][0], NUM_VERTICES, 4, 8);
//find_path_dfs(&my_graph[0][0], NUM_VERTICES, 9, 2);
getchar();
return 0;
}
void add_edge(int * graph, int size, int src, int dst, int cost)
{
*(graph+(src*size+dst)) = cost; /// Look carefully how the indices are calculated. You will need it for 2nd task.
}
void print_graph(int * graph, int size)
{
//char vertices[size];
for(int i=0; i<size; i++)
{
printf("\t%d", i);
}
printf("\n\n");
for(int x=0; x<size; x++)
{
printf("%d\t", x);
for(int y=0; y<size; y++)
printf("%d\t", *(graph+(x*size+y)));
printf("\n");
}
}
void find_path_dfs(int * graph, int size, int src, int dst)
{
int visited[10] = {0}; /// To keep track of all the visited nodes
/********** We make a stack for holding the visited vertices *****************/
struct node * top = NULL; /// This is the top of the stack
/** The DFS will work as follows:
- Visit src vertex. Set 'current_visiting' to src.
- Explore this vertex.
If it is the destination vertex, stop.
Otherwise visit its first unvisited neighbour (add to stack the current vertex)
If no unvisited neighbour vertex remains, go back (pop from stack)
- Repeat 2*/
int crnt_visiting = src;
int crnt_exploring = src;
int path_cost = 0;
bool PATH_FOUND = false;
while(!PATH_FOUND)
{
visited[crnt_visiting] = 1; /// Now we have visited this node
struct element temp;
if(crnt_visiting == dst) /// If the vertex is found
{
printf("\nPath found: ");
printf("\nCost: %d\n", path_cost);
while(!isStackEmpty(&top)) /// Empty the stack so all the allocated memory is freed.
{
printf("Pop\n");
pop(&top);
}
PATH_FOUND = true;
continue;
}
else /// Explore this vertex
{
/** Complete this function. You are free to make any changes to this function.
But make sure that path cost is correctly found.
*/
}
}
}
NODE H
struct element
{
int vertex_num;
int cost_to_visit;
};
struct node
{
struct element data;
struct node * next;
};
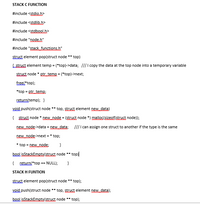
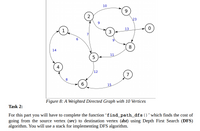

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- C++ Program #include <iostream>#include <cstdlib>#include <ctime>using namespace std; int getData() { return (rand() % 100);} class Node {public: int data; Node* next;}; class LinkedList{public: LinkedList() { // constructor head = NULL; } ~LinkedList() {}; // destructor void addNode(int val); void addNodeSorted(int val); void displayWithCount(); int size(); void deleteAllNodes(); bool exists(int val);private: Node* head;}; // function to check data exist in a listbool LinkedList::exists(int val){ if (head == NULL) { return false; } else { Node* temp = head; while (temp != NULL) { if(temp->data == val){ return true; } temp = temp->next; } } return false;} // function to delete all data in a listvoid LinkedList::deleteAllNodes(){ if (head == NULL) { cout << "List is empty, No need to delete…arrow_forwardstruct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forward#include <iostream>#include <cstdlib>#include <time.h>#include <chrono> using namespace std::chrono;using namespace std; void randomVector(int vector[], int size){ for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; }} int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast<microseconds>(stop - start); cout << "Time taken by function: " << duration.count()…arrow_forward
- #ifndef LLCP_INT_H#define LLCP_INT_H #include <iostream> struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endifarrow_forwardplease helparrow_forwardC++ Consider the following function as a property of a LinkedBag that contains a Doubly Linked List. Assume a Node has pointers prev and next, which can be read and changed with the standard get and set methods. Assume that the doubly linked list is: 1 <--> 2 <--> 3 <--> 4 <--> 5 <-->6 If you are uncertain what the above diagram depicts, it is a doubly linked list such that: The head of this doubly linked list is the node that contains the value 1. The tail of this doubly linked list is the node that contains the value 6. The 3rd node in this list contains the value 3. The contents of this list are the values 1 through 6 in sequential order. The following questions are regarding the linked list after after the test_function is run. A. The head of the list after the test_function is run contains the value: B. The tail of the list after the test_function is run contains the value: C. The 3rd node in the list after the test_function is run…arrow_forward
- Matrix.h #include <iostream>#include <iomanip>using namespace std; #ifndef MATRIX_H#define MATRIX_Hclass Matrix{ friend ostream& operator<<(ostream& out, const Matrix& srcMatrix);public: Matrix(); // initialize Matrix class object with rowN=1, colN=1, and zero value Matrix(const int rN,const int cN ); // initialize Matrix class object with row number rN, col number cN and zero values Matrix(const Matrix &srcMatrix ); // initialize Matrix class object with another Matrix class object Matrix(const int rN, const int cN, const float *srcPtr); // initialize Matrix class object with row number rN, col number cN and a pointer to an array const float * getData()const; // create a temp pointer and copy the array values which data pointer points, // then returns temp. int getRowN()const; // returns rowN int getColN()const; // returns colN void print()const; // prints the Matrix object in rowNxcolN form Matrix…arrow_forward// FILL IN THE BLANKS (LINKED-LISTS CODE) (C++)#include<iostream>using namespace std; struct ________ {int data ;struct node *next; }; node *head = ________;node *createNode() { // allocate a memorynode __________;temp = new node ;return _______ ;} void insertNode(){node *temp, *traverse;int n;cout<< "Enter -1 to end "<<endl;cout<< "Enter the values to be added in list"<<endl;cin>>n; while(n!=-1){temp = createNode(); // allocate memorytemp->data = ________;temp->next = ________;if ( ___________ == NULL){head = _________;} else {traverse = ( );while (traverse->next != ________{traverse = traverse-> ___________;} traverse->next= temp;} cout<<"Enter the value to be added in the list"<<endl;cin>>n; }} void printlist(){node *traverse = head; // if head == NULLwhile (traverse != NULL) { cout<<traverse->data<<" ";traverse = traverse->next;}} int main(){int option; do{cout<<"\n =============== MAIN…arrow_forwardplease answerarrow_forward
- 2 = 0 ) { numList [ i ] i } } } [1, 2, 3, 4, 5] [0, 2, 4, 6, 8] [2, 4, 6, 8, 10] [1, 3, 5, 7, 9]arrow_forwardWhat happens when a programmer attempts to access a node's data fields when the node variable refers to None? How do you guard against it? *PYTHONarrow_forward#include <stdio.h> int arrC[10] = {0}; int bSearch(int arr[], int l, int h, int key); int *joinArray(int arrA[], int arrB[]) { int j = 0; if ((arrB[0] + arrB[4]) % 5 == 0) { arrB[0] = 0; arrB[4] = 0; } for (int i = 0; i < 5; i++) { arrC[j++] = arrA[i]; if (arrB[i] == 0 || (bSearch(arrA, 0, 5, arrB[i]) != -1)) { continue; } else arrC[j++] = arrB[i]; } for (int i = 0; i < j; i++) { int temp; for (int k = i + 1; k < j; k++) { if (arrC[i] > arrC[k]) { temp = arrC[i]; arrC[i] = arrC[k]; arrC[k] = temp; } } } for (int i = 0; i < j; i++) { printf("%d ", arrC[i]); } return arrC; } int bSearch(int arr[], int l, int h, int key) { if (h >= l) { int mid = l + (h - l) / 2; if…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
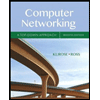
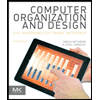
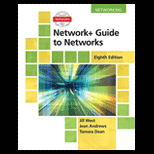
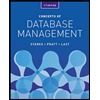
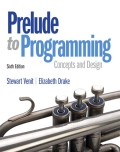
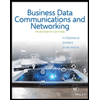