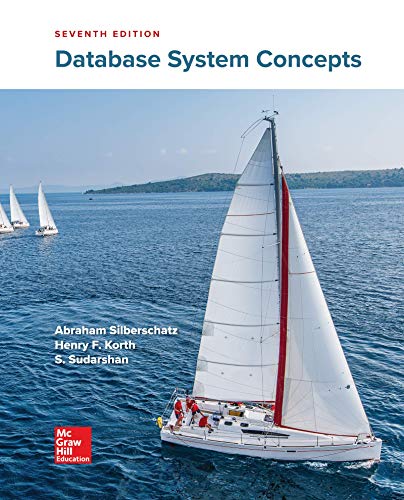
Concept explainers
1. Square Roots
Create a class with a method that, given an integer, returns an array of double-precision floating point numbers (known in Java as double), each of which is a square root of a number between 2 and the parameter to the method. For example, if the parameter is 5, the method should return an array of 4 doubles, with approximate values 1.4142135623, 1.7320508075, 2.0, and 2.2360679774. Your method should check the validity of the parameter, and take appropriate action if the parameter is invalid.
2. Reading Files
Create a class with a method that, given a string representing a file name, returns an integer with the number of characters in the file. For example, if the file has 10 characters, your method must return the number 10.
3. Main Class
Create a class HW1.java with a main method that does both of the following: calls the method from part 1 with a random integer between 0 and 10, and prints each of the numbers in the result. The numbers should all be printed on the same line, separated by commas, and with a newline at the end. calls the method from part 2 with each file name specified in the command-line arguments to the main method (see here if you don't remember how to use command-line arguments in Eclipse). For each result, the file name and the number of characters should be printed, one on each line, as in this example:
hello.txt 77
world.txt 199

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Java code Screenshot and output is mustarrow_forwardIn java Write a Payroll class that uses the following arrays as fields:• employeeId. An array of seven integers to hold employee identification numbers. Thearray should be initialized with the following numbers:5658845 4520125 7895122 87775418451277 1302850 7580489• hours. An array of seven integers to hold the number of hours worked by eachemployee• payRate. An array of seven doubles to hold each employee’s hourly pay rate• wages. An array of seven doubles to hold each employee’s gross wagesThe class should relate the data in each array through the subscripts. For example, thenumber in element 0 of the hours array should be the number of hours worked by theemployee whose identification number is stored in element 0 of the employeeId array. Thatsame employee’s pay rate should be stored in element 0 of the payRate array.In addition to the appropriate accessor and mutator methods, the class should have amethod that accepts an employee’s identification number as an argument and returns…arrow_forwardJAVA PROGRAM Chapter 7. PC #1. Rainfall Class Write a RainFall class that stores the total rainfall for each of 12 months into an array of doubles. The program should have methods that return the following: • the total rainfall for the year • the average monthly rainfall • the month with the most rain • the month with the least rain Demonstrate the class in a complete program. Main class name: RainFall (no package name) est Case 1 Enter the rainfall amount for month 1:\n1.2ENTEREnter the rainfall amount for month 2:\n2.3ENTEREnter the rainfall amount for month 3:\n3.4ENTEREnter the rainfall amount for month 4:\n5.1ENTEREnter the rainfall amount for month 5:\n1.7ENTEREnter the rainfall amount for month 6:\n6.5ENTEREnter the rainfall amount for month 7:\n2.5ENTEREnter the rainfall amount for month 8:\n3.3ENTEREnter the rainfall amount for month 9:\n1.1ENTEREnter the rainfall amount for month 10:\n5.5ENTEREnter the rainfall amount for month 11:\n6.6ENTEREnter…arrow_forward
- JAVA Program Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forwardJAVA PROGRAM Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forwardHow is this program written in java?arrow_forward
- in java Integer numVals is read from input and integer array userCounts is declared with size numVals. Then, numVals integers are read from input and stored into userCounts. If the first element is less than the last element, then assign Boolean firstSmaller with true. Otherwise, assign firstSmaller with false. Ex: If the input is: 5 40 22 41 84 77 then the output is: First element is less than last element 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 import java.util.Scanner; public class UserTracker { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intnumVals; int[] userCounts; inti; booleanfirstSmaller; numVals=scnr.nextInt(); userCounts=newint[numVals]; for (i=0; i<userCounts.length; ++i) { userCounts[i] =scnr.nextInt(); } /* Your code goes here */ if (firstSmaller) { System.out.println("First element is less than last element"); } else { System.out.println("First element is not less…arrow_forwardJAVA PROGRAM Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forwardIn C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forward
- In Java Assignment 5B : Maze Game! 2D Arrays can be used to store and represent informationabout video game levels or boards. In this exercise, you will use this knowledge tocreate an interactive game where players attempt to move through a maze. You willstart by creating a pre-defined 2D array with the following values:{"_","X","_","X","X"}{"_","X","_","X","W"}{"_","_","_","X","_"}{"X","X","_","_","_"}{"_","_","_","X","X"}You will then set the player (represented by “O”) at index 0, 0 of the array, the top-leftcorner of the maze. You will use a loop to repeatedly prompt the user to enter adirection (“Left”, “Right”, “Up”, or “Down”). Based on these directions, you will try tomove the player.• If the location is valid (represented by “_”), you will move the player there• If the location is out of bounds (e.g. index 0, -1) or the command is invalid, youwill inform the player and prompt them to enter another direction• If the location is a wall (represented by “X”), you will tell the…arrow_forwardCharge Account ValidationCreate a class with a method that accepts a charge account number as its argument. The method should determine whether the number is valid by comparing it to the following list of valid charge account numbers:5658845 4520125 7895122 8777541 8451277 13028508080152 4562555 5552012 5050552 7825877 12502551005231 6545231 3852085 7576651 7881200 4581002These numbers should be stored in an array. Use a sequential search to locate the number passed as an argument. If the number is in the array, the method should return true, indicating the number is valid. If the number is not in the array, the method should return false, indicating the number is invalid.Write a program that tests the class by asking the user to enter a charge account number. The program should display a message indicating whether the number is valid or invalid.arrow_forwardJAVA PROGRAM Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
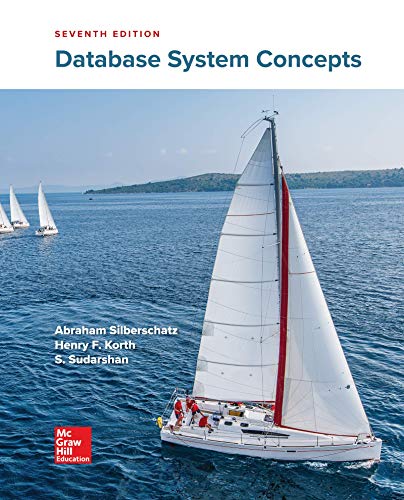
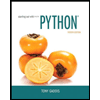
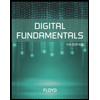
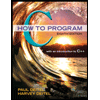
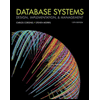
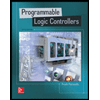