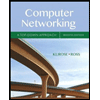
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
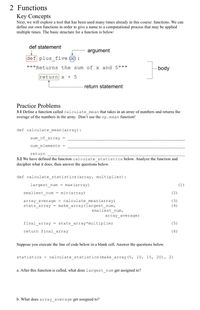
Transcribed Image Text:2 Functions
Key Concepts
Next, we will explore a tool that has been used many times already in this course: functions. We can
define our own functions in order to give a name to a computational process that may be applied
multiple times. The basic structure for a function is below:
def statement
argument
def plus_five (') :
"""Returns the sum of x and 5"""
-body
return x + 5
return statement
Practice Problems
3.1 Define a function called calculate_mean that takes in an array of numbers and returns the
average of the numbers in the array. Don't use the np.mean function!
def calculate_mean(array):
sum_of_array =
num elements =
return
3.2 We have defined the function calculate statistics below. Analyze the function and
decipher what it does, then answer the questions below.
def calculate_statistics (array, multiplier):
largest_num = max(array)
(1)
smallest_num = min(array)
(2)
array_average = calculate_mean(array)
stats_array = make_array (largest_num,
smallest_num,
(3)
(4)
array_average)
final_array = stats_array*multiplier
(5)
return final_array
(6)
Suppose you execute the line of code below in a blank cell. Answer the questions below.
statistics = calculate_statistics (make_array (5, 10, 15, 20), 2)
a. After this function is called, what does largest_num get assigned to?
b. What does array_average get assigned to?
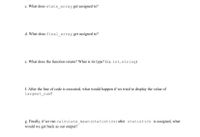
Transcribed Image Text:c. What does stats_array get assigned to?
d. What does final_array get assigned to?
e. What does the function return? What is its type? (i.e. int, string)
f. After the line of code is executed, what would happen if we tried to display the value of
largest_num?
g. Finally, if we ran calculate_mean (statistics) after statistics is assigned, what
would we get back as our output?
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 1 images

Knowledge Booster
Similar questions
- Implement a function that finds the number of values that are less than 0 and the number of values greater than 0 stored in an array of integers. Assume the array is the partially filled array from the previous question, and uses 0 to indicate the end of values stored in it. (18 pts) Note: the results should be passed back to the caller, not directly displayed in the function. Also note, as the following code template requires, the function’s return type is void. Please finish the function header, and function implementation. Hint.. In the beginning, set the lessThan and greaterThan values to be 0. Then as you scan through the array elements one by one, compare the next array element with 0. If the element is greater, update your greaterThan count; otherwise, if the element is less, update the lessThan count, otherwise do nothing. Continue until you see the value 0. GIVEN CODE: #include <iostream>using namespace std; // Find the number of values stored that is less than 0…arrow_forwardAHPA #10:The Secure Array(use C programming)• A programmer that you work with, Peter, is a jerk.• He is responsible for an array [theArray] that is a key part of an importantprogram and he maintains a sum of the array values at location [0] in the array.• He won't give you access to this array; however, your boss has told you that youneed to get input from the user and then place it into the array.• Each evening Peter will scan the code and remove any illegal references to hisarray.• Using pointers, access Peter's array without him knowing it and place threevalues that you got from the user (101, 63, 21) at locations 3, 6, and 9.Recalculate the sum value and update it. ( the output should be same as the picture)arrow_forwardC++, Please add a function where it can display the median, the maximum number, the minimum number, in an array entered by the user. Code: #include<iostream> using namespace std; class Search_Dynamic_Array { public: Search_Dynamic_Array(); int getArrSize(); void getArray(); void setArrsize(int); void setArray(int*); int getSum(int*); private: int arr_size; int arr[]; }; Search_Dynamic_Array::Search_Dynamic_Array() { //ctor } int Search_Dynamic_Array::getArrSize() { return arr_size; } void Search_Dynamic_Array::getArray() { cout << "The elements of the array are: "; for(int i=0; i<arr_size; i++){ cout << arr[i] << " "; } } void Search_Dynamic_Array::setArrsize(int s) { arr_size=s; } void Search_Dynamic_Array::setArray(int a[]) { for(int i=0; i<arr_size; i++){ arr[i]=a[i]; } } int Search_Dynamic_Array::getSum(int a[]) { int sum; for(int i=0; i<arr_size; i++){ sum +=arr[i]; } return sum; } int main() { int s=1, a[s]; cout << "Enter the size of the…arrow_forward
- 1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forwardC++ Double Pointer: Can you draw picture of what this means : Food **table? struct Food { int expiration_date; string brand; } Food **table; What is this double pointer saying? I know its a 2D matrix but I don't understand what I'm dealing with here. Does the statement mean that we have an array of pointers of type Food and what is the other * mean? I'm confused.arrow_forwardC++ Write a function named “checkInvalidHours” that accepts an array of PaySubobjects and its size. It will go through the array and check for invalid hours(negative values). If it is negative, it will reset the hourly payrate to 0. It willreturn how many PayStub objects that it has reset the payrate to 0.Please show you would call and test this function.arrow_forward
- Question 11 mahuse c++ Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forwardWrite a value-returning function that receives an array of structs of the type defined below and the length of the array as parameters. It should return as the value of the function the sum of the offspring amounts in the array. Note: you do not need a main function or to create an array. Assume the array already exists from main. #include <iostream>using namespace std; // struct declaration for Animal struct Animal { // a string for animal typestring animal_type;// a string for color string color;// an integer for number of offspring. int number_of_offspring;}; // Main function to declare a structure variable of type Animal. int main(){struct Animal A1;return 0;}arrow_forwardIn C++ please ! Write a Program that: Initializes an interger array size of 10 with random intergers in range [10,30]. Use a function named 'initialized_array' to acheive that. Determine if all array elements are distinct from each other or not.Use boolean function named 'distinct_elements' to achieve that function 'distinct elements, if the elements in the array are duplicate print out, elements are duplicate if not print out arrayarrow_forward
- (_9.). Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problem. Expect.arrow_forwardC++ Question Hello Please answer the attached C++ programming question correctly, just as the prompt states. Also, make sure that the code is working properly. Thank you.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
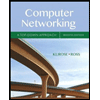
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
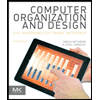
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
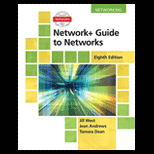
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
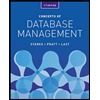
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
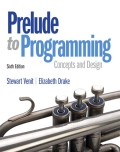
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
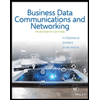
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY