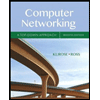
-
-
- add member function
- Signature: Complex add(const Complex &addComplex) const
- Returns a new Complex object that is this object added by the specified object.
- Formula: (a + bi) + (c + di) = (a + c) + (b + d)i
- New Real: (a + c)
- New Imaginary: (b + d)
- subtract member function
- Signature: Complex subtract(const Complex &subtractComplex) const
- Returns a new Complex object that is this object subtracted by the specified object.
- Formula: (a + bi) - (c + di) = (a - c) + (b - d)i
- New Real: (a - c)
- New Imaginary: (b - d)
- multiply member function
- Signature: Complex multiply(const Complex &multiplyComplex) const
- Returns a new Complex object that is this object multiplied by the specified object.
- Formula: (a + bi) * (c + di) = (a*c - b*d) + (b*c + a*d)i
- New Real: (a*c - b*d)
- New Imaginary: (b*c + a*d)
- divide member function
- Signature: Complex divide(const Complex ÷Complex) const
- Returns a new Complex object that is this object divided by the specified object.
- Formula: (a + bi) / (c + di) = ((a*c + b*d)/(c*c + d*d)) + ((b*c - a*d)/(c*c + d*d))i
- New Real: ((a*c + b*d) / (c*c + d*d))
- New Imaginary: ((b*c - a*d) / (c*c + d*d))
- Be sure to have a program description at the top and in-line comments.
- Be clear with your comments and output to the user, so I can understand what the program is doing.
- add member function
-
Code to edit
C++ CODE
#include<iostream>
using namespace std;
class Complex
{
double real,imaginary;
public:
Complex()
{
real=0;
imaginary=0;
}
void set(double r,double i)
{
real=r;
imaginary=i;
}
void print()
{
cout<<"("<<real<<"+("<<imaginary<<"i))";
}
double getReal()
{
return real;
}
double getImaginary()
{
return imaginary;
}
~Complex()
{
cout<<"Destrcutor\n";
}
};
int main()
{
Complex c1;
Complex c2;
Complex c3;
c2.set(3.3,-4.4);
c3.set(5.5,6.6);
cout<<"Test the constructor."<<endl;
cout<<"Complex number complex1 is: ";
c1.print();
cout<<"\n";
cout<<"Test the one set() function."<<endl;
cout<<"Complex number complex2 is: ";
c2.print();
cout<<"\n";
cout<<"Complex number complex3 is: ";
c3.print();
cout<<endl;
cout<<"Test the two get() functions."<<endl;
cout<<"Complex number complex3's real part is: "<<c3.getReal()<<endl;
cout<<"Complex number complex3's imaginary part is: "<<c3.getImaginary()<<endl;
return 0;
}
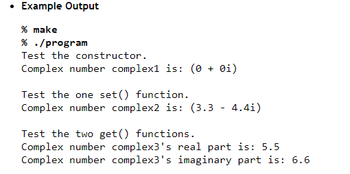

Step by stepSolved in 2 steps

- A class is a(n) OA) method OB) data type OC) function OD) attribute that is defined by the programmer.arrow_forwardis a special built-in pointer that is automatically supplied to all nonstatic member functions as a hidden parameter.arrow_forwardIs there a meaningful distinction that can be drawn between a Class and a Structure in terms of their functions?arrow_forward
- In order to use the same virtual function in a variety of forms and structures, a software application may need to execute a virtual function defined in a base class. A base-class reference or pointer is required.arrow_forwardCalculator Assignment Modify a calculator program that currently displays the Abstract Syntax Tree (AST) structure after parsing an input expression. Instead of printing the AST, enhance the program to evaluate the expression and display the result. Add support for the binary "%" remainder (modulo) operator and introduce variables with the assignment operator "=". Key steps to implement the assignment operator and variables: Introduce a new static attribute in the AstNode class to represent variables: Add a method isIdentifier() to the TokenStream class to detect variables: Modify the primaryRule() in AstNode to handle variables and variable assignment: Handle new node types in the AstNode evaluate() method for variables and assignment: These changes allow the calculator to evaluate expressions, support variables, and handle variable assignments. Users can create and use variables in subsequent expressions.EmployeeJava Type: package week3;import static week3.MainApp.*; public enum…arrow_forwardLanguage: C++ -------- (a) Name an operator that should be overloaded as a member function. Why? (b) Name an operator that should be overloaded as a non-member function. Why?arrow_forward
- Please answer in Programming C++ Define a structure called Class with name (string), units (int) and grade (char) as its member data. Define a structure called Student with ID (int), name (string), num (int), gpa (double) and an array of maximum of 20 Class structures called classes, where ID is a 5 digit number, name is the student's full name, num is the number of classes taken so far and gpa is the current grade point average and classes is all the classes taken so far. Write a function called get_info which takes an array of Student structures called students and array size, reads all the information for as many students as the user wants to enter except for the gpa which is later calculated, storing them in students array. It then returns the number of students entered by the user before quitting. The user indicates the end of data entry by entering -99 for the ID of a student. The user must enter as many classes for each student as indicated by num - number of classes. Write a…arrow_forwardDefine the term Equality Operator.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
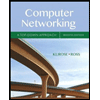
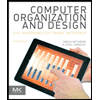
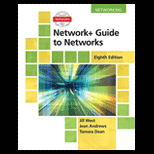
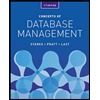
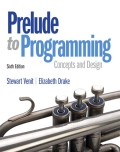
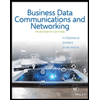