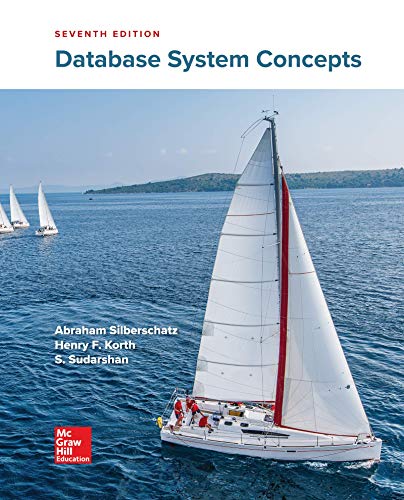
Algorithm
We already have an algorithm for Patient Temperature. It will not change.
The Algorithm for Patient Temperature:
- get the temperature
- implementation: with a prompt box before, now it will come from a widget
- assess it with conditionals and variables
- implementation: pretty much the same as before
- output a user-friendly message
- implementation: with an alert box or text before, now some text and images
Your Mission
For each of the two if-statements:
- replace false with the correct conditional expressions for this program to make sense.
- change each of the three images by finding new urls.
<!DOCTYPE html><html><body>
90°<input type="range" min="90" max="110" id="temperature" step=.1 oninput="changeTemp()">110°
<p id="assessmentText">Move the slider</p>
<img id="assessmentImage" width=50 src='https://www.maxpixel.net/static/photo/1x/Questions-Puzzle-Cartoon-Consider-Smiley-3082809.png'>
<script>
const temperatureFever = 99.5 ; // maximum
const temperatureHypothermia = 95 ; // minimum
const imageFever='https://cdn.pixabay.com/photo/2018/12/24/17/07/catch-a-cold-3893262_960_720.png';
const imageHypothermia='https://cdn.pixabay.com/photo/2018/12/19/20/23/samuel-3884706_960_720.png';
const imageNormal='https://cdn.pixabay.com/photo/2019/02/19/19/45/thumbs-up-4007573_960_720.png';
function changeTemp() { // Automatically happens when the slider
// Algorithm step 1: get the temperature
// Implementation: get it from the range finder:
var userResponse=document.getElementById("temperature").value;
var assessmentText='?';
var assessmentImage='';
// Algorithm step 2: assess
// Implementation: not much different than before
if ( false ) {
assessmentText=" Hypothermia";
assessmentImage=imageHypothermia;
}
else if (false) {
assessmentText=" Fever";
assessmentImage=imageFever;
}
else {
assessmentText=" Normal";
assessmentImage=imageNormal;
}
// Algorithm step 3: display user-friendly output
// Implementation: using variables makes this code work every time
document.getElementById('assessmentText').innerHTML=userResponse+'° '+assessmentText;
document.getElementById('assessmentImage').src=assessmentImage;
}
</script></body></html>

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- Any help with this first problem would be really helpful! Thank you so much!! 1. Write the following exercises using Java code. Add comments in each program to explain what your code is doing. A. Write a program that generates two random integers, both in the range 50 to 100, inclusive. Use the Math class. Print both integers and then display the positive difference between the two integers, but use a selection. Do not use the absolute value method of the Math class. B. Duke Shirts sells Java t-shirts for $24.95 each, but discounts are possible for quantities as follows: 1 or 2 shirts, no discount and total shipping is $10.003-5 shirts, discount is 10% and total shipping is $8.006-10 shirts, discount is 20% and total shipping is $5.0011 or more shirts, discount is 30% and shipping is free Write a Java program that prompts the user for the number of shirts required. The program should then print the extended price of the shirts, the shipping charges, and the total cost of the…arrow_forwardMust be in JAVA. Please show in simplest form and with comments.arrow_forwardJava Conditional statements.arrow_forward
- A B A Circuit 1 Circuit 2 Based on the image, select all true statements. Tip: draw truth tables! Circuit 1 is equivalent to the boolean expression: "Q = (A and B) and ((B or C) and (C and B))" %3D Circuit 1 is equivalent to the boolean expression: "Q = (A and B) or ((B or C) and (C and B))" Circuit 2 is equivalent to the boolean expression: "Q = ((B and C) and (B or C)) or (B and A)" Circuit 2 is equivalent to the boolean expression: "Q = (C and B) or ((B or C) and (A and B))" Circuit 1 is not equivalent to Circuit 2. Circuit 1 is equivalent to Circuit 2.arrow_forwardJava - Text Message (this is not graded)arrow_forwardDesign a program that takes a string as input from the user and prints its length.7. Create a script that takes a string as input from the user and prints it reversed.8. Create a Python script that asks the user to enter two strings and then prints their concatenation.9. Create a Python program that asks the user for their age and prints out a message saying whether theyare a teenager (between 13 and 19 years old) or not.10. Design a program that prompts the user to enter a positive integer and then prints whether it is a primenumber or not.11. Design a program that calculates the factorial of a given number using a loop. Ensure the input numberis a positive integer.12. Develop a program that prompts the user for the lengths of three sides of a triangle and prints whetherthe triangle is equilateral, isosceles, or scalene.arrow_forward
- Complete the following program to implement the user interface of the preceding exercise. For simplicity, only the units cm, m, and in are supported. Hint: The value of factor1 or factor2 should be the conversion factor from the selected unit to cm. Ex: If the selected unit is in, factor1 is 2.54 because 1 in = 2.54 cm."in java"arrow_forwardSelect all alternatives that are correct related to variable names. a. It can have symbols in the middle or at the end. O b. It can not have digits at the end. C. It cannot start with a symbol, except__. O d. It can start with a digit. e. It can start with a capital letter.arrow_forwardAlert dont submit AI generated answer.arrow_forward
- Don't use ai to answer Solve itarrow_forwardCreate a program and inside your program, type the code as given below. Then rewrite the nested ifs with logical operators. 1. int num = 5; // nested if if ( num > 0 ) { if ( num 5) { if (y > 3) { System.out.println ("x is greater and y is greater"); else if(x = 78) { System.out.println("It will snow!"); else { System.out.println ("What am I? The weatherman?"); Output will look like this (the first line being the given code; the repeated line being the rewritten code): num is between 0 and 10 num is between 0 and 10 x is less and y is less x is less and y is less It will snow! It will snow!arrow_forward1.Write a Java program of Rock-Paper-Scissors. Two players will input the first equivalent letter. For example: S for Scissor, R for Rock and P for Paper. Then Display the winner with a message such as: "Player 1 Wins" or "Rock breaks Scissors" or "It's a Tie", ETC. Use if-else statements. 2. Given the flowchart below, what does this Flowchart do? Conver the Flowchart into a Java Program using if-else statements. 3. Write a java program that accepts an ordinary number and outputs its equivalent roman numerals. Below is some samples of ordinary number and roman numerals. Ordinary numbers Roman numerals1 I5 V10 X50 L 100 C500 D1000 M If you input ordinary number 2968…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
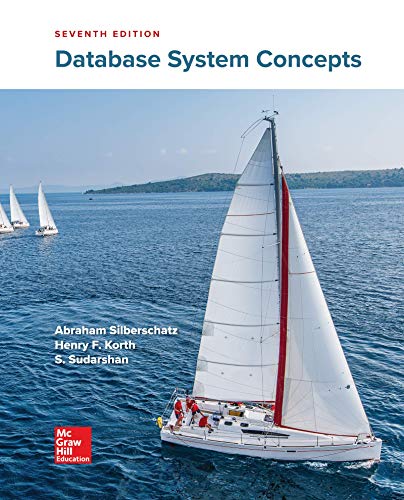
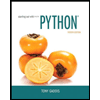
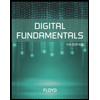
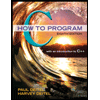
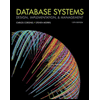
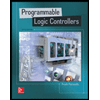