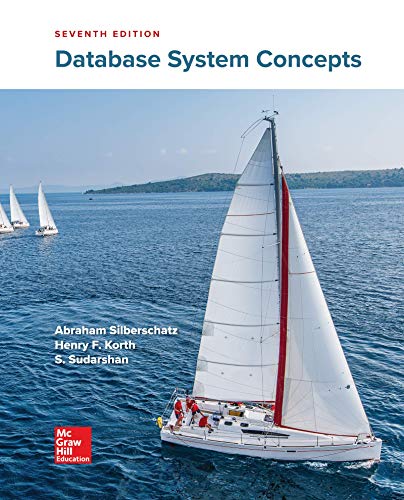
Concept explainers
I am trying to compile a poker program in c++ and it still doesn't work:
main.cpp:
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
#include <sstream>
#include "card.h"
#include "deck.h"
#include "hand.h"
using namespace std;
/************************************************************
* FunctionName *
* Function description *
* *
* *
************************************************************/
int main()
{
string repeat = "Y";
Deck myDeck;
Hand myHand;
string exchangeCards;
while (repeat == "Y" || repeat == "y")
{
cout << endl;
myHand.newHand(myDeck);
myHand.print();
cout << endl;
cout << "Would you like to exchange any cards? [Y / N]: ";
getline(cin, exchangeCards);
while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, exchangeCards);
}
if(exchangeCards == "Y" || exchangeCards == "y")
{
myHand.exchangeCards(myDeck);
}
cout << endl;
myHand.print();
cout << endl;
myDeck.reset(); // Resets the deck for a new game
cout << "Play again? [Y / N]: ";
getline(cin, repeat);
while (repeat != "Y" && repeat != "y" && repeat != "N" && repeat != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, repeat);
}
}
return 0;
}
card.h:
#ifndef CARD_H
#define CARD_H
#include <iostream>
#include <string>
using namespace std;
// Sources: https://en.wikipedia.org/wiki/Standard_52-card_deck
// https://en.wikipedia.org/wiki/Pip_(counting)
const string pips[] = {"Ace", "Two", "Three", "Four", "Five",
"Six", "Seven", "Eight", "Nine", "Ten",
"Jack", "Queen", "King"};
const string suits[] = {"Hearts", "Spades", "Clubs", "Diamonds"};
class Card
{
public:
// Get card value
int get();
// Set card value
void set(int value);
string getPip();
string getSuit();
// Print card value
void print();
private:
int m_cardValue;
};
#endif
Deck.h:
#ifndef DECK_H
#define DECK_H
#include <vector>
#include <cstdlib> // srand(), rand()
#include <ctime> // time()
#include "card.h" // Include card header file here
using namespace std;
class Deck
{
public:
// class Constructor
Deck();
// Reset deck to new state (completely undealt)
void resetDeck();
// Print all cards in the undealt deck
void printUndealtDeck();
// Print all cards in the dealt deck
void printDealtDeck();
// Get size of the undealt deck
const int getSizeUndealtDeck();
// Get size of the dealt deck
const int getSizeDealtDeck();
// Deal a single card
Card dealCard(); // Is the dealCard() here an accessor or mutator function???
private:
vector<Card> m_undealtDeck; // Undealt cards
vector<Card> m_dealtDeck; // Dealt cards
};
#endif
hand.h
#ifndef DECK_H
#define DECK_H
#include <vector>
#include <cstdlib> // srand(), rand()
#include <ctime> // time()
#include "card.h" // Include card header file here
using namespace std;
class Deck
{
public:
// class Constructor
Deck();
// Reset deck to new state (completely undealt)
void resetDeck();
// Print all cards in the undealt deck
void printUndealtDeck();
// Print all cards in the dealt deck
void printDealtDeck();
// Get size of the undealt deck
const int getSizeUndealtDeck();
// Get size of the dealt deck
const int getSizeDealtDeck();
// Deal a single card
Card dealCard(); // Is the dealCard() here an accessor or mutator function???
private:
vector<Card> m_undealtDeck; // Undealt cards
vector<Card> m_dealtDeck; // Dealt cards
};
#endif
This is the error I am getting.
![H *main.cpp - Code:Blocks 20.03
File Edit View Search Project Build Debug Fortran wxSmith Tools Tools+ Plugins DoxyBlocks Settings Help
> E G: 4: A G: II E
E C:
团
| <global>
v main0 : int
/** *<
+ + 2 Aa *
Q Q S C
Management
Start here x *main.cpp x card.h X deck.h x hand.h Xx
•
Projects Files
FSymbols
|| exchangeCards == "y")
52
if(exchangeCards
== "y"
Workspace
53
54
myHand.exchangeCards (myDeck) ;
55
56
cout << endl;
57
58
myHand.print ();
59
60
cout << endl;
61
62 D
myDeck.reset (); // Resets the deck for a new game
63
cout << "Play again? [Y / N]: ";
getline (cin, repeat);
while (repeat != "Y" && repeat != "y" && repeat != "N" && repeat != "n")
64
65
66
67
{
68
cout << "please enter Y or N only: ";
69
getline (cin, repeat);
70
Logs & others
A Code:Blocks X
Search results x Cccc >x
Build log X
Build messages X CppCheck/Vera++ X CppCheck/Vera++ messages X Cscope X
Debuggl
File
Line
Message
=== Build file: "no target" in "no project" (compiler: unknown)
===
C:\Users\Mica...
In function 'int main ()':
C:\Users\Mica...
62
error: 'resetDeck' was not declared in this scope
=== Build failed: 1 error (s), 0 warning (s) (0 minute (s), 2 second (s))
%3%D3D3D
C:\Users\Micai\Downloads\Assignment2\main.cpp
C/C++
Windows (CR+LF)
WINDOWS-1252 Line 55, Col 10, Pos 1444
Insert
Modified
Read/Write
default](https://content.bartleby.com/qna-images/question/e6b9d87e-6e1d-4edc-b4b6-dbb55c2a248d/f44ce78d-c68d-46ec-bd01-a238cb05ad2f/5kt026_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- PuTTY/Ocelot Assignment #2 Instructions: (using C language in the UNIX environment, Systems Programming) Through this programming assignment, the students will learn to do the following: Usage: mortgagepmt [-s] -r rate [-d downpayment] price In this assignment, you are asked to perform a mortgage payment calculation. All information needed for this will be passed to the program on the command line. There will be no user input during the execution of the program You will need a few pieces of information. The price of the home and the amount of the down payment. You will also need to know the interest rate and the term of the mortgage. To figure your mortgage payment, start by converting your annual interest rate to a monthly interest rate by dividing by 12. Next, add 1 to the monthly rate. Third, multiply the number of years in the term of the mortgage by 12 to calculate the number of monthly payments you'll make. Fourth, raise the result of 1 plus the monthly rate to the…arrow_forwardC++ please solve the equationnnnnnarrow_forwardI need help with this project that I've uploaded.arrow_forward
- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardc++ programming one of the image is what the output of the code is supposed to be. please use these if possible: #include <iostream> using namespace std; other stuff such as #include <conio.h> doesn't work on my compiler. Please help me with this program. Thanks!arrow_forwardample code for the reader-writer: #include <pthread.h>#include <semaphore.h>#include <stdio.h> /*This program provides a possible solution for first readers writers problem using mutex and semaphore.I have used 10 readers and 5 producers to demonstrate the solution. You can always play with these values.*/ sem_t wrt;pthread_mutex_t mutex;int cnt = 1;int numreader = 0; void *writer(void *wno){ sem_wait(&wrt);cnt = cnt*2;printf("Writer %d modified cnt to %d\n",(*((int *)wno)),cnt);sem_post(&wrt); }void *reader(void *rno){ // Reader acquire the lock before modifying numreaderpthread_mutex_lock(&mutex);numreader++;if(numreader == 1) {sem_wait(&wrt); // If this id the first reader, then it will block the writer}pthread_mutex_unlock(&mutex);// Reading Sectionprintf("Reader %d: read cnt as %d\n",*((int *)rno),cnt); // Reader acquire the lock before modifying numreaderpthread_mutex_lock(&mutex);numreader--;if(numreader == 0) {sem_post(&wrt); //…arrow_forward
- Subject: programming language : c++ Question : Jane has opened a new fitness center with charges of 2500 per month so the cost to become a member of a fitness center is as follow :1.For senior citizens discount is 30 %2. For young one the discount is 15 %3. For adult the discount is 20% Write a menu driven program (using structure c++) thata. Add a new memberb. Display the general information on about the fitness center and its chargesc. Determine the cost of a new membershipd. At any time show the total money made.Use appropriate parameters to pass information on and out of a function.arrow_forwardDon't give me AI generated answer or plagiarised answer.arrow_forwardC++ Question Hello, Please create the correct code for the attached picture. Please create the code based on the given requirements. Please make sure it is working. Thank you!arrow_forward
- Please I need help to get this homework done, I am not sure how to build the program correctly.arrow_forwardAssignment #2 Instructions: Through this programming assignment, the students will learn to do the following: Learn to work with command line options and arguments Gain more experience with Makefiles Gain more experience with Unix Learn to use some of the available math functions available with C Usage: mortgagepmt [-s] -r rate [-d downpayment] price In this assignment, you are asked to perform a mortgage payment calculation. All information needed for this will be passed to the program on the command line. There will be no user input during the execution of the program You will need a few pieces of information. The price of the home and the amount of the down payment. You will also need to know the interest rate and the term of the mortgage. To figure your mortgage payment, start by converting your annual interest rate to a monthly interest rate by dividing by 12. Next, add 1 to the monthly rate. Third, multiply the number of years in the term of the mortgage by 12 to calculate the…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
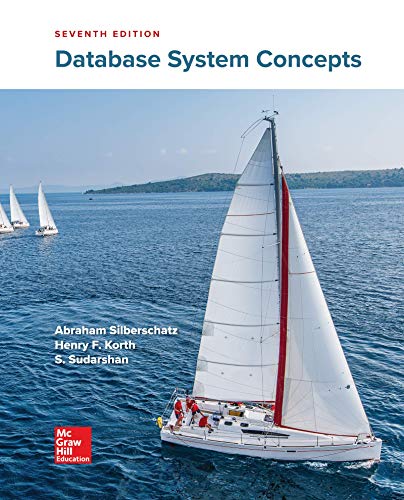
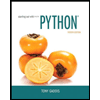
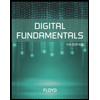
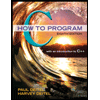
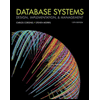
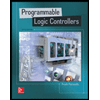