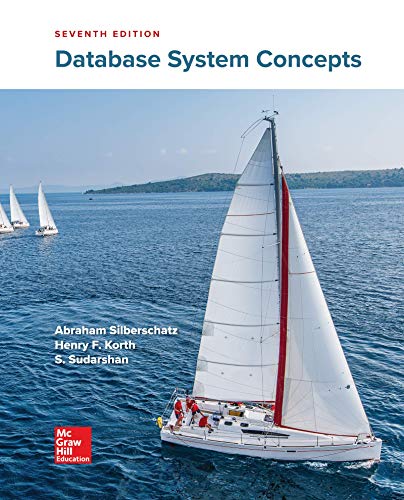
===========Answer question below:===========
- Write program in Java.
(Convert infix to postfix)
Note:
- Postfix notation is a way of writing expression without using parentheses. For example, the expression ( 11 + 12 ) * 13 would be written as 11 12 + 13 *
- Assume that ALWAYS there is a space between operands and operators in the input expression.
- Use two stacks, one to store the operands and one to store the operators.
- Your program only accpets following operators :
- ( )
- +
- -
- /
- *
Write a method to converts an infix expression into a postfix expression using the following method:
String infixToPostfix(String expression)
For example, the method should convert the infix expression
( 13 + 25 ) * 34 to 13 25 + 34 *
and
20 * ( 10 + 30 ) to 20 10 30 + *.
- solution.java:
import java.util.*;
import java.lang.*;
import java.io.*;
class InfixToPostfix {
public String infixToPostfix(String expression) {
}
}
class DriverMain {
public static void main(String args[]) {
Scanner input = new Scanner(System.in);
InfixToPostfix postfix = new InfixToPostfix();
try {
System.out.println(postfix.infixToPostfix(input.nextLine()));
}
catch (Exception ex) {
System.out.println("Wrong expression");
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- provide answer with full explanation ( only type ) java pleasearrow_forward[Python Language] A common back-of-the-envelope technique for estimating a runner’s marathon time is to take their best half-marathon time, multiply by two and add ten minutes. This works pretty well unless the marathon course is hilly.If it is hilly, we add an extra 20 minutes to the estimate. Write a program that takes two lines of input: a float giving the half- marathon time in minutes and an integer indicating whether the marathon course is hilly(1) or not (0). It prints a float giving the estimated number of minutes the runner will take to run the full marathon. Write your pro- gram in a file named marathon.py.arrow_forwardPaoBLEM# 39 I Simplify the following expressions using Boolean algebra. Cite the laws and therorems used. • AB + A(CD + CD') • (BC' + A'D) (AB' + CD')arrow_forward
- 1. Simplify the following expressions. Play with them b) Y = C.(AC.B)arrow_forwardWarm-up: Integer Operations (with C++) Write a program that asks the user to enter two integers and prints the Difference, Product, Area of an Ellipse, and Average of these numbers. Here is an example of the execution of the program: Enter the first number: 23Enter the second number: 17**********The results are:Difference: 6Product: 391Area of an Ellipse: 1228Average: 20arrow_forward2. Express the following Boolean expressions in simpler form; that is, use fewer operators. x is an inE (a) !(x == 2)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
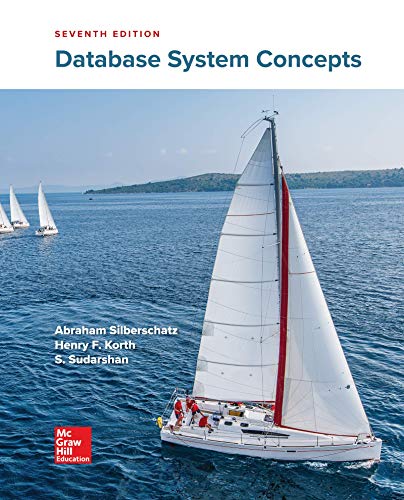
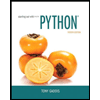
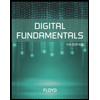
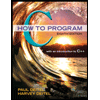
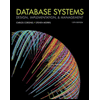
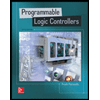