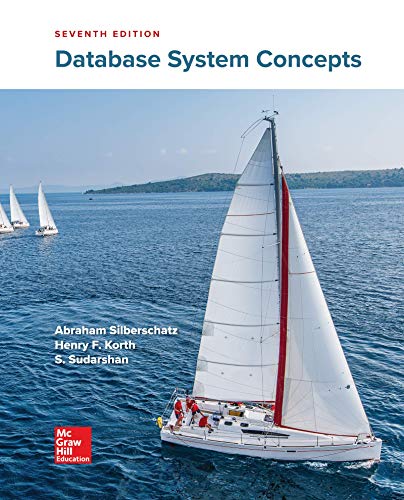
Arrays
Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10}, print:
7 9 11 10 10 11 9 7
Hint: Use two for loops. Second loop starts with i = NUM_VALS - 1. (Notes)
Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element array (int courseGrades[4]). See "How to Use zyBooks".
Also note: If the submitted code tries to access an invalid array element, such as courseGrades[9] for a 4-element array, the test may generate strange results. Or the test may crash and report "
#include <iostream>
using namespace std;
int main() {
const int NUM_VALS = 4;
int courseGrades[NUM_VALS];
int i;
for (i = 0; i < NUM_VALS; ++i) {
cin >> courseGrades[i];
}
/* Your solution goes here */
return 0;
}
Please help me with this problem using c++.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In visual basic Write the code that will sequentially search through the array strFirstNames and will print out the word "Found" if the name "Brian" is in the array.arrow_forwardTicTacToeCreate the board display the board Set token to ‘x’Loop //at most 9 times, but end loop when someone wins{ Ask player where to place token display the board Checks if won Change token (if it was ‘x’ change to ‘o’ and vice versa)}Either congratulate someone on winning or say it was a draw. Create a 17x17 array of characters and put ‘ ‘ in each element.2. Write a loop to make the first horizontal line, and another to do the second horizontal line3. Write a loop to make the first vertical line, and another to create the second vertical line4. Put in the numbers (this is so the player can choose where to put the token). You will need 9 separate assignment statements.arrow_forwardDescribe how to make an array of struct elements.arrow_forward
- # TODO y_values = display(y_values) todo_check([ (y_values.shape == (120,),'y_values does not have the correct shape of (120,)'), (np.all(np.isclose(y_values,…arrow_forwardQuestion Write the code needed to create, instantiate and populate an array. You may select the datatype.arrow_forwardAssume the int array items contains the values 0, 2, 4, 6 and 8. Which of the following uses the enhanced for loop to display each value in the array? Note: The code in the answer choices is written on one line. It should be written on two lines. O for (int i : items) System.out.println(items[i]); O for (int i = 0 : items.length) System.out.printIn(items[i]); O for (int i = 0; i < items.length; i++) System.out.println(items[i]); O for (int i : items) System.out.println(i);arrow_forward
- A(n) ___ is used in an array to across each element? a. Index b. Subscript C. Both a and b d. None of the abovearrow_forwardWrite a loop that subtracts 1 from each element in lowerScores. If the element was already 0 or negative, assign 0 to the element. Ex: lowerScores = {5, 0, 2, -3} becomes {4, 0, 1, 0}. #include <iostream>using namespace std; int main() { const int SCORES_SIZE = 4; int lowerScores[SCORES_SIZE]; int i; for (i = 0; i < SCORES_SIZE; ++i) { cin >> lowerScores[i]; } /* Your solution goes here */ for (i = 0; i < SCORES_SIZE; ++i) { cout << lowerScores[i] << " "; } cout << endl; return 0;}arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
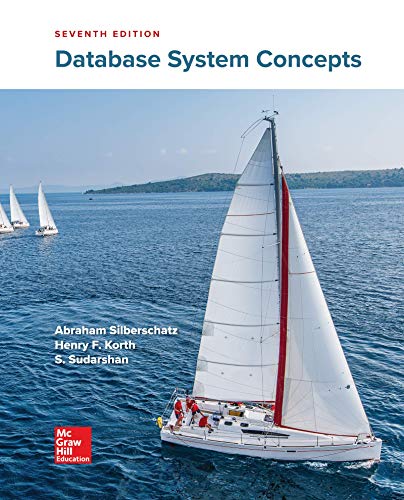
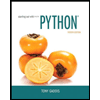
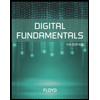
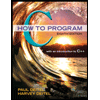
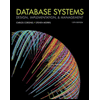
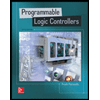