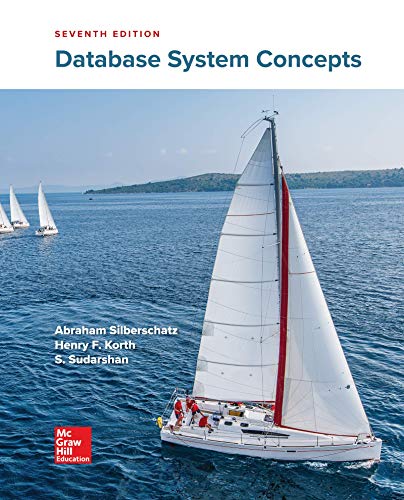
Assignment #2 Instructions:
Through this programming assignment, the students will learn to do the following:
Learn to work with command line options and arguments
Gain more experience with Makefiles
Gain more experience with Unix
Learn to use some of the available math funtions available with C
Usage: mortgagepmt [-s] -r rate [-d downpayment] price
In this assignment, you are asked to perform the mortgage payment calculation. All information needed for this will be passed to the program on the command line. There will be no user input during the execution of the program
You will need a few pieces of information. The price of the home and the amount of the down payment. You will also need to know the interest rate and the term of the mortgage. To figure your mortgage payment, start by converting your annual interest rate to a monthly interest rate by dividing by 12. Next, add 1 to the monthly rate. Third, multiply the number of years in the term of the mortgage by 12 to calculate the number of monthly payments you’ll make. Fourth, raise the result of 1 plus the monthly rate to the negative power of the number of monthly payments you’ll make. Fifth, subtract that result from 1. Sixth, divide the monthly rate by the result. Last, multiple the result by the amount you want to borrow.
In addition, you will add on PMI of 1% of the amount borrowed/12 if the down payment is less than 20% of the price of the home. This will stay the same for the life of the loan.
Options:
-s is optional and means that the mortgage will be a 15 year mortgage instead of the standard 30 year mortgage you would use as a default.
-r is required and the argument is the yearly interest rate for the loan. The interest rat3e should be between 3% and 10%.
-d is optional and the argument is the downpayment that will be made on the loan. It cannot be larger than the price of the home.
You will print out the resulting payment amount as follows. Use rounding to keep money to two decimals and percentages to three decimals.
"The payment on a loan of $###,###.## with an interest rate of #.###% for a term of ## years will be $##,###.##"
There should be no other output at all to the screen.
Please submit your work through Canvas as one zip file called FirstnameLastnameA2.zip. Follow the instructions below carefully (to avoid unnecessary loss of grade). Include your source code and your Makefile in the zip file. I should be able to create the executable by typing ‘make’. The Makefile should also contain a 'clean' target for cleaning up the directory (removing all object files). Make sure you don't include intermediate files: *.o, executables, *~, etc., in your submission. (There'll be a penalty for including unnecessary intermediate files or any folders).
Please make sure you submit homework before the deadline. There will be a late penalty as per the syllabus.
If the program does not compile and do something useful when it runs it will not earn any credit.

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps

- In C++ please, using basic programming one coding The main menu should show the available options: Show the roster: Displays all the player names and jersey numbers in a numbered list. Ex: 1. Susan Martinez (33), 2. Jake Smith (21), etc. Add a player: Prompts the user to type the player's full name and jersey number. Then adds the player to the roster. Remove a player: Displays the roster and prompts the user to enter the number of the player to remove. Then removes the player from the roster. Quit: Quits the program. The program should display the main menu after the user shows the roster, adds a player, or removes a player. The program only terminates when the user chooses the quit option. Data validation should be used where appropriate. Ex: The user should not be able to choose an option that doesn't exist or delete a player that doesn't exist. The roster should be kept in alphabetic order by the players' full names. Ex: If Alice Chang (9) is added to a roster with Jake Smith and…arrow_forwardDirections: Write a program that will perform a casino game using C. Provide comments in the codeexplaining everything. See above deliverable instructions. Requirements: A menu will first appear asking the user to choose which game they would like to play. The userwill be able to choose between blackjack and roulette.The blackjack game will only play one hand at a time against the dealer. If the dealer and the user have the same,then it’s a draw. If you do not know how to play blackjack, then you might need to research the game.The roulette game will ask the user what numbers they would like to bet on. If the random number is chosen, thenthe user wins.Each game should ask the user how much money they would like to bet before the game starts. The user shouldhave a starting amount of money, once the user loses their money, the user will then need to stop playing. Expert Solutionarrow_forwardC++ continued you already gave me the help from the first part. now they have added another set of instructions. original code used. Explanation Here I have created the main method. Inside the main method, I have taken input from the user for height and width and stored them into different variables. Next, I have created a loop that runs till it reaches the height of the rectangle. Inside the loop, I have created another loop that runs till the width of the rectangle. In the inner loop, I have printed the asterisk symbol. After the inner loop, I have used endl to give break and move the pointer to the new line. arrow_forward Answer C++ code: #include<iostream>using namespace std; int main(){ int height, width; // user input cin >> height >> width; // outer loop for(int i = 0; i < height; i++) { // inner loop for(int j = 0; j < width; j++) { //printing the * symbol cout << "*"; }…arrow_forward
- Using a Sentinel Value to Control a while Loop in Java Summary In this lab, you write a while loop that uses a sentinel value to control a loop in a Java program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the file named MovieGuide.java is open. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop to calculate the average star rating. Execute the program by clicking Run. Input the following: 0, 3, 4, 4, 1, 1, 2, -1 Check that the…arrow_forwardcreate a passphrase generator on c++ that follows this. Please make sure the code works on c++ visual studios Diceware™ is a method for picking passphrases that uses ordinary dice to select words at random from a special list called the Diceware Word List. Each word in the list is preceded by a five digit number. All the digits are between one and six, allowing you to use the outcomes of five dice rolls to select a word from the list. Here is a short excerpt from the English Diceware word list: 16655 clause 16656 claw 16661 clay 16662 clean 16663 clear 16664 cleat 16665 cleft 16666 clerk 21111 cliche 21112 click 21113 cliff 21114 climb 21115 clime 21116 cling 21121 clink 21122 clint 21123 clio 21124 clip 21125 clive 21126 cloak 21131 clock The complete list contains 7776 short words, abbreviations and easy-to-remember character strings. The average length of each word is about 4.2 characters. The biggest words are six characters long. The English list is based on a longer word list…arrow_forwardc++ code Screenshot and code is mustarrow_forward
- Asking help for Java Programming Create a program that simulates a meeting reservation system. Part 1: Basic requirements The program shall allow the user to select from the following options: Create a new meeting Show meetings on the calendar Clear all meetings Each meeting has a subject, start day/time and end day/time Subject is a short text description of the meeting Day is a date that contains month, day, and year Meeting times need only deal with hour and minute When the user wants to create a new meeting, the program asks for the subject, start and end day/times for it and adds it to the calendar For the basic requirements, meetings are not allowed to overlap. If a meeting the user wants to schedule overlaps with an existing meeting, the program presents an error message showing which meeting the one the user wants to schedule overlaps with When the user wants to show all meetings for the week, the report displays all meetings each day as follows Show all meetings…arrow_forwardMONTY HALL LAB write this code in python and use # to answers questions - You are only allowed to use the following functions/methods: print(), range(), len(), sum(), max(), min(), and .append(). It is not mandatory to useall of these functions/methods. In this lab, you will write a code that simulates the Monty Hall Game Show. Thegame host gives the participant the choice of selecting one of three doors. Twodoors has a goat behind them and one door has a prize. The set of choices arerandomized each round. The participant needs to select the door with the prizebehind it. When the participant selects a door, the game host reveals a door with agoat behind it. The game host opens a door (different from the one selected by theparticipant) that has a goat behind it. The participant is then given the option tochange their choice. When you run your code, the code would display a message prompting the user toinput their door choice, labelled as 1, 2, and 3. Then the code will display a…arrow_forwardSubject: programming language : c++ Question : Jane has opened a new fitness center with charges of 2500 per month so the cost to become a member of a fitness center is as follow :1.For senior citizens discount is 30 %2. For young one the discount is 15 %3. For adult the discount is 20% Write a menu driven program (using structure c++) thata. Add a new memberb. Display the general information on about the fitness center and its chargesc. Determine the cost of a new membershipd. At any time show the total money made.Use appropriate parameters to pass information on and out of a function.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
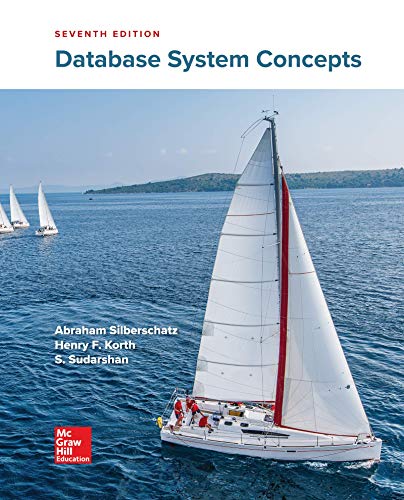
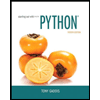
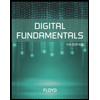
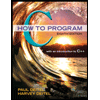
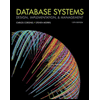
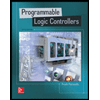