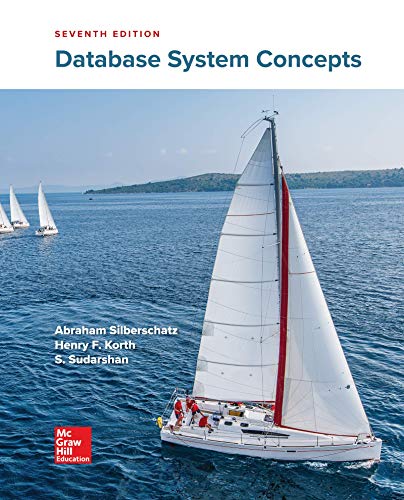
assume the input data is structured as follows: first there is a non negative inetger specifying the number of empolyee timesheets to be read in. This is followed by data for each of the employees.
The first number for each employee is an integer that specifies their pay per hour in cents. Following this are 5 integers, the numbers of hours they worked on each of the days of the workweek.
Given this data, write a loop and any necessary code that reads the data and stores the total payroll of all employees into the variable total. note that you will have to add up the numbers worked by each employee and multiply that by that particular emplyee's pay rate to get the employee's pay for the week and sum those valuses into total. using python and nested loops

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- You are tasked with writing a program to process students’ end of semester marks. For each student you must enter into your program the student’s id number (an integer), followed by their mark (an integer out of 100) in 4 courses. A student number of 0 indicates the end of the data. A student moves on to the next semester if the average of their 4 courses is at least 60 (greater than or equal to 60). Write a program to read the data and print, for each student: The student number, the marks obtained in each course, final mark of the student (average of all the marks), their final grade (A,B,C,D or F) and whether or not they move on to the next semester. In addition, after all data entry is completed, print The number of students who move on to the next semester and the number who do not. The student with the highest final mark (ignore the possibility of a tie). Grades are calculated as follows: A = 70 and over, B = 60 (inclusive) -70, C = 50(inclusive)-60 D =…arrow_forwardC++ Language 2arrow_forwardCreating a function table, python code from the following pictures:arrow_forward
- code language in c++ using for loops and nested for loopsarrow_forwardDo not use global variables for this assignment Choose descriptive variable names in all programs. Currency format. There should be no space between the $ sign and the first digit. In python, Write a program that uses a custom function named as you wish and the main function. In main, prompt the user for a first name and an integer less than 10 and then execute the custom function with these two inputs as arguments. The custom function should output one line displaying the name as many times as specified by the integer, separating each repetition of the name with a space.arrow_forwardpython Now write another program that reads the text file created in the program above and calculates the user's weekly pay. The program should begin by prompting the user for the regular hourly pay rate. The program should then loop through the file and report the days and hours worked. The program should finish by calling a custom void function named calc_pay that calculates and prints the weekly pay, including overtime, if applicable. By law, weekly hours in excess of 40 should be paid at 1.5 times the regular hourly rate. The calc_pay function takes two arguments, total hours and hourly pay rate, and it must be imported from a separate module file. Note that three outputs are required for the pay.Sample Output 1Enter your hourly pay rate 25.00Here are your hours this weekMonday hours : 10.0Tuesday hours : 8.0Wednesday hours : 12.0Friday hours : 12.0Saturday hours : 8.0You worked 50.0 hours this weekYou worked 10.0 hours overtimeYour hourly pay rate is $25.00 Regular pay :…arrow_forward
- in python Write a Python program that uses a for loop with the range function to inspect all integers from 300 down to 1. See page 170-172 to learn about the range function. Code in the loop should find exact multiples of 23 and print them in fields 5 characters wide on one line. The loop should also determine two totals; the total of the even multiples and the total of the odd multiples of 23. When the loop ends, the program should display these two totals.arrow_forwardCode should be in Python. Prompt the user to enter a string of their choosing. Output the string.Ex: Enter a sentence or phrase: The only thing we have to fear is fear itself. You entered: The only thing we have to fear is fear itself. Complete the get_num_of_characters() function, which returns the number of characters in the user's string. We encourage you to use a for loop in this function. Extend the program by calling the get_num_of_characters() function and then output the returned result. Extend the program further by implementing the output_without_whitespace() function. output_without_whitespace() outputs the string's characters except for whitespace (spaces, tabs). Note: A tab is '\t'. Call the output_without_whitespace() function in main().Ex: Enter a sentence or phrase: The only thing we have to fear is fear itself. You entered: The only thing we have to fear is fear itself. Number of characters: 46 String with no whitespace: Theonlythingwehavetofearisfearitself.arrow_forwardInstructions C++ 8-17 A company hired 10 temporary workers who are paid hourly and you are given a data file that contains the last name of the employees, the number of hours each employee worked in a week, and the hourly pay rate of each employee. You are asked to write a program that computes each employee’s weekly pay and the average salary of all employees. The program then outputs the weekly pay of each employee, the average weekly pay, and the names of all the employees whose pay is greater than or equal to the average pay. If the number of hours worked in a week is more than 40, then the pay rate for the hours over 40 is 1.5 times the regular hourly rate. Use two parallel arrays: a one-dimensional array to store the names of all the employees (Name) a two-dimensional array of 10 rows and 3 columns to store the number of hours an employee worked in a week (Hrs Worked), the hourly pay rate (Pay Rate), and the weekly pay (Salary). Your program must contain at least the following…arrow_forward
- Hello! I am having trouble writing this code! It is in Python! Any help is appreciated! program7_1.pyFollow instructions carefully to avoid point deductions. The text file is not required. It will be created when your program is graded. Write a program that begins by calling a custom function named as you wish. This function must create and return a list of 8 random integers, all in the range from 10-100. use a for loop to display the 8 random integers all on one line separated by a single space. print the highest number in the list. print the lowest number in the list. print the total of all list elements. sort the list in descending order. use another loop to display the sorted numbers in descending order, all on the same line, separated by a single space. This loop should also contain code to count the number of even integers and the number of odd integers. report both counts when the loop above has ended. use slicing syntax to make another list holding the middle 2 elements of…arrow_forwardThis program in C++ obtains a series of letter grades as input from a user. The program should calculate and output the total grade points and the grade point average (note: you may have to track the total number of inputs and the grade points to calculate the grade point average). The algorithm should execute 3 times for testing purposes.Use the appropriate loop for the main structure of the program that will stop accepting letter grades when the user inputs X. Also, choose the appropriate selection statement to convert the letter grade to the correct grade points.Additional Requirements:• Use appropriate data types and variable names throughout the code.• Use constants appropriately. Output needs to look like the image below.arrow_forwardEnter an integer: -29 -29 is negative and odd! // OUTPUT Sample Run #2 We write code to manipulate data, which are provided by the user(s), to produce the required outcome in the most efficient way! CIS 25 Fall 2023 - Homework #1 - Page 5 of 7 CIS 25 C++ Programming Laney College Your Name Information Assignment: Implemented by: Required Submission Date: Actual Submission Date: CIS 25 C++ Programming Laney College Your Name Enter an integer: -294258 -294257 is negative and even! // OUTPUT Sample Run #3 We write code to manipulate data, which are provided by the user(s), to produce the required outcome in the most efficient way! Information -- Assignment: Implemented by: Required Submission Date: Actual Submission Date: HW #1 Exercise #1 Your Name 2023/09/18 JJ- CIS 25 - C++ Programming Laney College Your Name HW #1 Exercise #1 Your Name 2023/09/18 Enter an integer: 41294258 41294258 is positive and even! // OUTPUT Sample Run #4 We write code to manipulate data, which are provided by…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
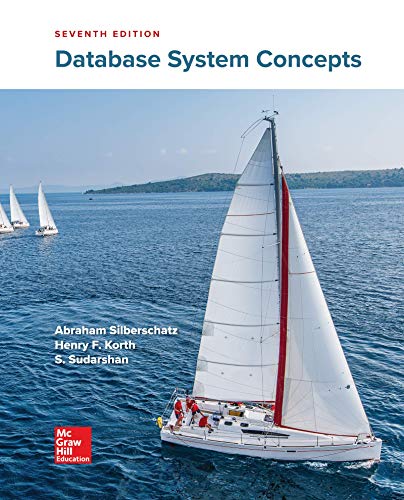
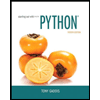
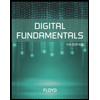
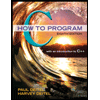
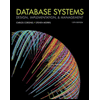
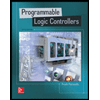