C++ Program #include #include #include using namespace std; int getData() { return (rand() % 100); } class Node { public: int data; Node* next; }; class LinkedList{ public: LinkedList() { // constructor head = NULL; } ~LinkedList() {}; // destructor void addNode(int val); void addNodeSorted(int val); void displayWithCount(); int size(); void deleteAllNodes(); bool exists(int val); private: Node* head; }; // function to check data exist in a list bool LinkedList::exists(int val){ if (head == NULL) { return false; } else { Node* temp = head; while (temp != NULL) { if(temp->data == val){ return true; } temp = temp->next; } } return false; } // function to delete all data in a list void LinkedList::deleteAllNodes(){ if (head == NULL) { cout << "List is empty, No need to delete Nodes" << endl; return; } else { Node* current = head; Node* next; while (current != NULL) { next = current->next; delete current; current = next; } } head = NULL; cout << "All the nodes are deleted" << endl; } // function to add node to a list void LinkedList::addNode(int val) { Node* newnode = new Node(); newnode->data = val; newnode->next = NULL; if (head == NULL) { head = newnode; } else { Node* temp = head; // head is not NULL while (temp->next != NULL) { temp = temp->next; // go to end of list } temp->next = newnode; // linking to newnode } } // function to add node to a list sorted void LinkedList::addNodeSorted(int val) { Node* newnode = new Node(); newnode->data = val; newnode->next = NULL; /* Special case for the head end */ if (head == NULL || head->data >= newnode->data) { newnode->next = head; head = newnode; } else { /* Locate the node before the point of insertion */ Node* current = head; // head is not NULL while (current->next != NULL && current->next->data < newnode->data) { current = current->next; } newnode->next = current->next; current->next = newnode; } } // function to display list void LinkedList::displayWithCount() { if (head == NULL) { cout << "List is empty!" << endl; } else { Node* temp = head; int count = 1; while (temp != NULL) { cout << count <<". " << temp->data <next; count++; } cout << endl; } } //function to list size int LinkedList::size() { int count = 0; if (head == NULL) { return count; } else { Node* temp = head; while (temp != NULL) { temp = temp->next; count++; } } return count; } int main() { /* initialize random seed: */ srand (time(NULL)); int maxSize = 20; //initialize list LinkedList* list = new LinkedList(); //add new nodes for (int i=0 ; i< maxSize; i++){ list->addNode( getData() ); } cout << "Linked List data" << endl; list->displayWithCount(); cout << "Delete Linked List data" << endl; list->deleteAllNodes(); cout << "Add Linked List sorted data" << endl; while( list->size() < maxSize){ int data = getData(); if(list->exists(data) == false){ list->addNodeSorted( data ); } } cout << "Linked List sorted data" << endl; list->displayWithCount(); list->deleteAllNodes(); delete list; return 0; } Copy the completed Assignment 1 to Assignment 2. Re-label comments as needed. Add this feature to the end of the existing code - this is after the data is loaded and displayed in Part 2 of assignment 1: Inspect each element in the Link list If the integer in the list is considered bad data (via method provided below), delete the entry. Display the value being deleted as part of this process. After Step A above is completed - Display all the current values in the Linked List. Delete all entries in the Linked List. // bad data ends in 3 or 5 or 9 bool badData(int myData) { int result = myData % 10; return (result == 3 || result == 5 || result == 9);
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
C++ Program
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
int getData() {
return (rand() % 100);
}
class Node {
public:
int data;
Node* next;
};
class LinkedList{
public:
LinkedList() { // constructor
head = NULL;
}
~LinkedList() {}; // destructor
void addNode(int val);
void addNodeSorted(int val);
void displayWithCount();
int size();
void deleteAllNodes();
bool exists(int val);
private:
Node* head;
};
// function to check data exist in a list
bool LinkedList::exists(int val){
if (head == NULL) {
return false;
}
else {
Node* temp = head;
while (temp != NULL) {
if(temp->data == val){
return true;
}
temp = temp->next;
}
}
return false;
}
// function to delete all data in a list
void LinkedList::deleteAllNodes(){
if (head == NULL) {
cout << "List is empty, No need to delete Nodes" << endl;
return;
}
else {
Node* current = head;
Node* next;
while (current != NULL) {
next = current->next;
delete current;
current = next;
}
}
head = NULL;
cout << "All the nodes are deleted" << endl;
}
// function to add node to a list
void LinkedList::addNode(int val) {
Node* newnode = new Node();
newnode->data = val;
newnode->next = NULL;
if (head == NULL) {
head = newnode;
}
else {
Node* temp = head; // head is not NULL
while (temp->next != NULL) {
temp = temp->next; // go to end of list
}
temp->next = newnode; // linking to newnode
}
}
// function to add node to a list sorted
void LinkedList::addNodeSorted(int val) {
Node* newnode = new Node();
newnode->data = val;
newnode->next = NULL;
/* Special case for the head end */
if (head == NULL || head->data >= newnode->data) {
newnode->next = head;
head = newnode;
}
else {
/* Locate the node before the point of insertion */
Node* current = head; // head is not NULL
while (current->next != NULL && current->next->data < newnode->data) {
current = current->next;
}
newnode->next = current->next;
current->next = newnode;
}
}
// function to display list
void LinkedList::displayWithCount() {
if (head == NULL) {
cout << "List is empty!" << endl;
}
else {
Node* temp = head;
int count = 1;
while (temp != NULL) {
cout << count <<". " << temp->data <<endl;
temp = temp->next;
count++;
}
cout << endl;
}
}
//function to list size
int LinkedList::size() {
int count = 0;
if (head == NULL) {
return count;
}
else {
Node* temp = head;
while (temp != NULL) {
temp = temp->next;
count++;
}
}
return count;
}
int main() {
/* initialize random seed: */
srand (time(NULL));
int maxSize = 20;
//initialize list
LinkedList* list = new LinkedList();
//add new nodes
for (int i=0 ; i< maxSize; i++){
list->addNode( getData() );
}
cout << "Linked List data" << endl;
list->displayWithCount();
cout << "Delete Linked List data" << endl;
list->deleteAllNodes();
cout << "Add Linked List sorted data" << endl;
while( list->size() < maxSize){
int data = getData();
if(list->exists(data) == false){
list->addNodeSorted( data );
}
}
cout << "Linked List sorted data" << endl;
list->displayWithCount();
list->deleteAllNodes();
delete list;
return 0;
}
- Copy the completed Assignment 1 to Assignment 2. Re-label comments as needed.
- Add this feature to the end of the existing code - this is after the data is loaded and displayed in Part 2 of assignment 1:
- Inspect each element in the Link list
- If the integer in the list is considered bad data (via method provided below), delete the entry. Display the value being deleted as part of this process.
- After Step A above is completed - Display all the current values in the Linked List.
- Delete all entries in the Linked List.
- Inspect each element in the Link list
// bad data ends in 3 or 5 or 9
bool badData(int myData) {
int result = myData % 10;
return (result == 3 || result == 5 || result == 9);
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

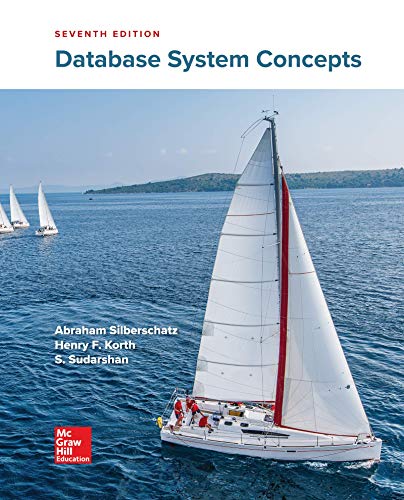
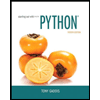
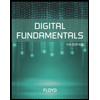
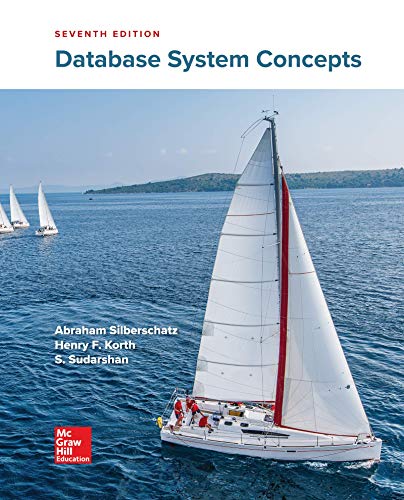
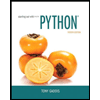
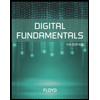
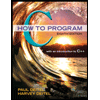
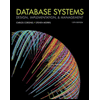
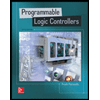