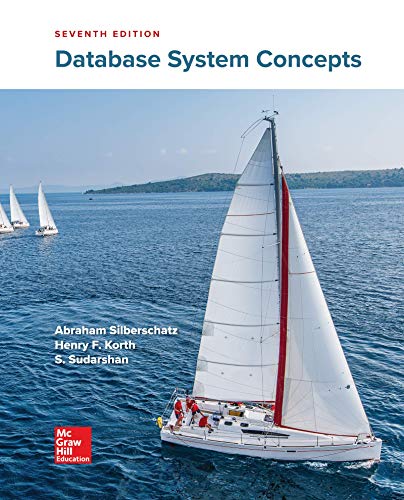
C
Problem to solve
1) Write a program that will ask the user to enter 5 exam scores then print the score highest to lowest.
Specifications
1) Array with user input
2) Function that calculates the highest score

Program:
#include <stdio.h>
int highest_score (int arr[]);
void highest_to_lowest (int arr[]);
int main()
{
int arr[100], c, d, s;
printf("Enter 5 Exam scores:\n");
for (c = 0; c < 5; c++)
scanf("%d", &arr[c]);
for (c = 0 ; c < 5 - 1; c++)
{
for (d = 0 ; d < 5 - c - 1; d++)
{
if (arr[d] < arr[d+1])
{
s = arr[d];
arr[d] = arr[d+1];
arr[d+1] = s;
}
}
}
highest_to_lowest(arr);
printf ("The highest score is: %d", highest_score(arr));
printf ("\n");
}
void highest_to_lowest (int arr[])
{
printf("The score highest to lowest:\n");
for (int c = 0; c < 5; c++)
printf("%d\n", arr[c]);
}
int highest_score (int arr[])
{
for (int i = 1; i < 5; ++i) {
if (arr[0] < arr[i])
arr[0] = arr[i];
}
return arr[0];
}
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Create a string variable containing your full name (with spaces) 2) print the string as shown below 3) Create a blank 5x10 character array 4) Create a function printArray() that will print every row and column of the array 5) Create a function getNextCharacter() that gets passed the name string and returns the next non-blank character. If you have reached the end of the string then continue at the beginning. You may need to use a global variable 6) Create a function fillArray() that will fill the 2 dimensional array with the letters of your name skipping blanks. Use the above getNextCharacter() function to fill the array with only the characters in your last name. 7) Print the entire array. 8) Request a row number to print and use a sentinal loop to make sure the row number is valid. If not then request a row number again 9) Create a function printIter() that prints only the passed row using iteration 10) Create a function printRecur() that prints only the passed row using recursion…arrow_forwardWrite a flowchart and C code for a program that does the following: Declare an array that will store 9 values Use a for() statement to store user entered scores for all 9- holes Create a function and pass the array into the definition of the function to compute the total Use an accumulating total statement to compute the total score Display the overall total to the output screen so that the golfer can see if he won.arrow_forwardC# Question Assume that you have an array of integers called numbers that has been declared and filled with values. Write the heading of a for loop that can be used to iterate through the entire array. Use i as the loop variable.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
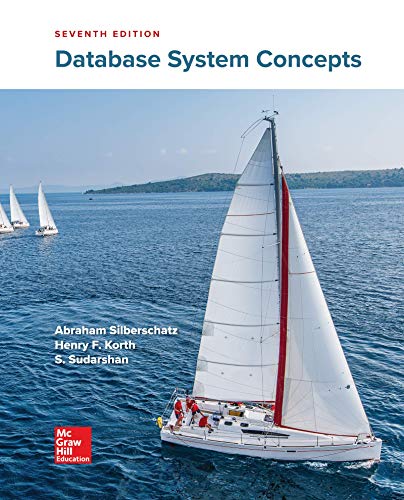
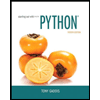
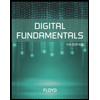
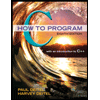
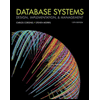
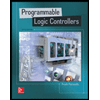