Can anybody fix the error in this program? In my constructor in my City.h file. //City.h file #ifndef Point_H_ #define Point_H_ #include #include #include "Point.h" const size_t MAX_CITYNAME = 80; class City { private: Point location; char cityName[MAX_CITYNAME]; public: City(void); City(Point l, char city[MAX_CITYNAME]) : location{l} { setName(city); } double distance(const City & other) const { return location.calculateDistance(other.location); } void setLocation(Point l) { location = l; } Point getLocation(void) const { return location; } void setName(char city[]) { strcpy(cityName,city); } char* getName(void) { return cityName; } void printInformation(void) { std::cout <<"\tName: " << cityName <<"\nLocation: "; location.outputPoint(); } void updateInformation(Point l, char city[]) { location = l; strcpy(cityName,city); } }; #endif //Point.h file #ifndef POINT_H_ #define POINT_H_ #include #include class Point { private: double x; double y; public: Point(void) : x{0.0}, y{0.0} {} Point(double Xcoordinate, double Ycoordinate) : x{Xcoordinate}, y{Ycoordinate} {} //mutator function void setX(double Xcoordinate) { x = Xcoordinate; } void setY(double Ycoordinate) { y = Ycoordinate; } //accesor function double getX(void) const { return x; } double getY(void) const { return y; } void inputPoint() { char t; std::cin >> t >> x >> t >> y >> t; } void outputPoint(void) const { std::cout << '(' << x << "," << y << ')'; return; } double calculateDistance(const Point & p) const { return sqrt(pow(x - p.x, 2.0) + pow(p.y - y, 2.0)); } Point calculateMidpoint(const Point & p) const { return Point((p.x + x) / 2, (p.y + y) / 2); } }; #endif //calculateCity file #include #include #include "Point.h" #include "City.h" using namespace std; int main() { char choice; //choice the user makes City myCity[5]; //array of city char name[20]; //for storing the length size_t i = 0; Point point; cout <<"\n\t\tWelcome to Calculate Distance between City Program!!!\n"; cout <<"\n 1) Enter city Information." <<"\n2) Calculate Distance between two cities" <<"\n3) Print All cities" <<"\n4) Quit" << endl <<"\nChoice: "; cin >> choice; cin.ignore(numeric_limits::max(), '\n'); switch(choice) { case '1': case 'e' : case 'E' : if (i < 5) { cout <<"Enter the city name: "; cin.getline(name,20); cout <<"Enter the coordinates of the city: "; point.inputPoint(); myCity[i] = City(point,name); i++; } else //if the city name is overload { } break; case '2': case 'c' : case 'C' : if (i >= 2) { if (i == 2) { cout <<"Distance between two cities: "; cout << myCity[0].distance(myCity[1]); cout << endl; } else { int n1, n2; for (size_t j = 0; j < i; j++) { cout << j + 1 << "."; myCity[j].printInformation(); } cout <<"\nEnter the numbers of the cities for the distance: "; cin >> n1 >> n2; cout <<"\nThe distance betweem " << myCity[n1-1].getName() <<" and " << myCity[n2-1].getName() << " is "; cout <
Can anybody fix the error in this program? In my constructor in my City.h file.
//City.h file
#ifndef Point_H_
#define Point_H_
#include <iostream>
#include <cstring>
#include "Point.h"
const size_t MAX_CITYNAME = 80;
class City
{
private:
Point location;
char cityName[MAX_CITYNAME];
public:
City(void);
City(Point l, char city[MAX_CITYNAME])
: location{l} {
setName(city);
}
double distance(const City & other) const
{
return location.calculateDistance(other.location);
}
void setLocation(Point l)
{
location = l;
}
Point getLocation(void) const
{
return location;
}
void setName(char city[])
{
strcpy(cityName,city);
}
char* getName(void)
{
return cityName;
}
void printInformation(void)
{
std::cout <<"\tName: " << cityName <<"\nLocation: ";
location.outputPoint();
}
void updateInformation(Point l, char city[])
{
location = l;
strcpy(cityName,city);
}
};
#endif
//Point.h file
#ifndef POINT_H_
#define POINT_H_
#include <iostream>
#include <cmath>
class Point
{
private:
double x;
double y;
public:
Point(void) : x{0.0}, y{0.0} {}
Point(double Xcoordinate, double Ycoordinate) :
x{Xcoordinate}, y{Ycoordinate} {}
//mutator function
void setX(double Xcoordinate)
{
x = Xcoordinate;
}
void setY(double Ycoordinate)
{
y = Ycoordinate;
}
//accesor function
double getX(void) const
{
return x;
}
double getY(void) const
{
return y;
}
void inputPoint()
{
char t;
std::cin >> t >> x >> t >> y >> t;
}
void outputPoint(void) const
{
std::cout << '(' << x << "," << y << ')';
return;
}
double calculateDistance(const Point & p) const
{
return sqrt(pow(x - p.x, 2.0) + pow(p.y - y, 2.0));
}
Point calculateMidpoint(const Point & p) const
{
return Point((p.x + x) / 2, (p.y + y) / 2);
}
};
#endif
//calculateCity file
#include <iostream>
#include <limits>
#include "Point.h"
#include "City.h"
using namespace std;
int main()
{
char choice; //choice the user makes
City myCity[5]; //array of city
char name[20]; //for storing the length
size_t i = 0;
Point point;
cout <<"\n\t\tWelcome to Calculate Distance between City Program!!!\n";
cout <<"\n 1) Enter city Information."
<<"\n2) Calculate Distance between two cities"
<<"\n3) Print All cities"
<<"\n4) Quit" << endl
<<"\nChoice: ";
cin >> choice;
cin.ignore(numeric_limits<streamsize>::max(), '\n');
switch(choice)
{
case '1': case 'e' : case 'E' :
if (i < 5)
{
cout <<"Enter the city name: ";
cin.getline(name,20);
cout <<"Enter the coordinates of the city: ";
point.inputPoint();
myCity[i] = City(point,name);
i++;
}
else //if the city name is overload
{
}
break;
case '2': case 'c' : case 'C' :
if (i >= 2)
{
if (i == 2)
{
cout <<"Distance between two cities: ";
cout << myCity[0].distance(myCity[1]);
cout << endl;
}
else
{
int n1, n2;
for (size_t j = 0; j < i; j++)
{
cout << j + 1 << ".";
myCity[j].printInformation();
}
cout <<"\nEnter the numbers of the cities for the distance: ";
cin >> n1 >> n2;
cout <<"\nThe distance betweem " << myCity[n1-1].getName()
<<" and " << myCity[n2-1].getName() << " is ";
cout <<myCity[n1-1].distance(myCity[n2-1]) << endl;
}
}
else
{
cout <<"\nNot enought city in the map. ";
}
break;
default :
cout <<"\nYou have entered an invalid choice!!!\n";
break;
}
return 0;
}


Step by step
Solved in 5 steps with 1 images

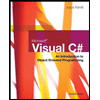
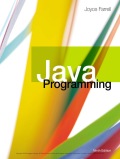
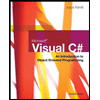
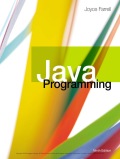