/Animal.cpp is the main function file of the Animal class //CSIS 112/B16 #include using namespace std; //defining Animal class class Animal { public: //Name and age of class string name; int age; //default contstructor Animal() { name = ""; age = 0; cout << "Invoking Animal 2 argument constructor?" << endl; } //virtual function virtual void move() {} //getter and setter functions string getName() { return name; } void setName(string n) { name = n; } int getAge() { return age; } void setAge(int a) { age = a; } }; //Dog derives Animal publically class Dog : public Animal { public: //dogs constructor Dog(string n, int a) { name = n; age = a; cout << "Invoking Dog constructor" << endl; } //implementing virtual function virtual void move() { cout << "I run" << endl; } }; class Rabbit : public Animal { public: Rabbit(string n, int a) { name = n; age = a; cout << "Invoking Rabbit constructor," << endl; } ~Rabbit() { cout << "Invoking Rabbit destructor" << endl; } virtual void move() { cout << "I hop" << endl; } }; class Fish : public Animal { public: Fish(string n, int a) { name = n; age = a; cout << "Invoking Fish constructor" << endl; } ~Fish(){ cout << "Invoking Fish destructor" << endl; } virtual void move() { cout << "I swim" << endl; } }; class Snake : public Animal { public: Snake(string n, int a) { name = n; age = a; cout << "Invoking Snake constructor" << endl; } virtual void move() { cout << "I slither" << endl; } }; int main() { cout << ("Steven_Brightwell-Assignment 8\n"); int i = 0; Animal animals[4]; while (i < 3) { cout << endl << "Press (1) for dog\t(2)for rabbit\t(3) for fish\t(4) for snake" << endl; cout << "Choice:"; int choice; cin >> choice; switch (choice) { case 1: { int age = rand() % (20 - 1) + 1;; string name; cout << "Name:"; cin >> name; animals[i] = Dog(name, age); break; } case 2: { int age = rand() % (20 - 1) + 1; string name; cout << "Name:"; cin >> name; animals[i] = Rabbit(name, age); break; } case 3: { int age = rand() % (20 - 1) + 1; string name; cout << "Name:"; cin >> name; animals[i] = Fish(name, age); break; } case 4: { int age = rand() % (20 - 1) + 1; string name; cout << "Name:"; cin >> name; animals[i] = Snake(name, age); break; } } i++; } for (int i = 0; i < 5; i++) { cout << "hello" << endl;
How would I fix this to get it looking like output #2?
//Animal.cpp is the main function file of the Animal class
//CSIS 112/B16
#include <iostream>
using namespace std;
//defining Animal class
class Animal {
public:
//Name and age of class
string name;
int age;
//default contstructor
Animal() {
name = "";
age = 0;
cout << "Invoking Animal 2 argument constructor?" << endl;
}
//virtual function
virtual void move() {}
//getter and setter functions
string getName() {
return name;
}
void setName(string n) {
name = n;
}
int getAge() {
return age;
}
void setAge(int a) {
age = a;
}
};
//Dog derives Animal publically
class Dog : public Animal {
public:
//dogs constructor
Dog(string n, int a) {
name = n;
age = a;
cout << "Invoking Dog constructor" << endl;
}
//implementing virtual function
virtual void move() {
cout << "I run" << endl;
}
};
class Rabbit : public Animal {
public:
Rabbit(string n, int a) {
name = n;
age = a;
cout << "Invoking Rabbit constructor," << endl;
}
~Rabbit() {
cout << "Invoking Rabbit destructor" << endl;
}
virtual void move() {
cout << "I hop" << endl;
}
};
class Fish : public Animal {
public:
Fish(string n, int a) {
name = n;
age = a;
cout << "Invoking Fish constructor" << endl;
}
~Fish(){
cout << "Invoking Fish destructor" << endl;
}
virtual void move() {
cout << "I swim" << endl;
}
};
class Snake : public Animal {
public:
Snake(string n, int a) {
name = n;
age = a;
cout << "Invoking Snake constructor" << endl;
}
virtual void move() {
cout << "I slither" << endl;
}
};
int main() {
cout << ("Steven_Brightwell-Assignment 8\n");
int i = 0;
Animal animals[4];
while (i < 3) {
cout << endl << "Press (1) for dog\t(2)for rabbit\t(3) for fish\t(4) for snake" << endl;
cout << "Choice:";
int choice;
cin >> choice;
switch (choice) {
case 1: {
int age = rand() % (20 - 1) + 1;;
string name;
cout << "Name:";
cin >> name;
animals[i] = Dog(name, age);
break;
}
case 2: {
int age = rand() % (20 - 1) + 1;
string name;
cout << "Name:";
cin >> name;
animals[i] = Rabbit(name, age);
break;
}
case 3: {
int age = rand() % (20 - 1) + 1;
string name;
cout << "Name:";
cin >> name;
animals[i] = Fish(name, age);
break;
}
case 4: {
int age = rand() % (20 - 1) + 1;
string name;
cout << "Name:";
cin >> name;
animals[i] = Snake(name, age);
break;
}
}
i++;
}
for (int i = 0; i < 5; i++) {
cout << "hello" << endl;
animals[i].move();
Looks like this:
Steven_Brightwell-Assignment 8
Invoking Animal 2 argument constructor?
Invoking Animal 2 argument constructor?
Invoking Animal 2 argument constructor?
Invoking Animal 2 argument constructor?
Press (1) for dog (2)for rabbit (3) for fish (4) for snake
Choice:1
Name:dog
Invoking Animal 2 argument constructor?
Invoking Dog constructor
Press (1) for dog (2)for rabbit (3) for fish (4) for snake
Choice://Animal.cpp is the main function file of the Animal class
Press (1) for dog (2)for rabbit (3) for fish (4) for snake
Choice:hello
hello
hello
hello
hello
C:\Users\hpsha\OneDrive Liberty\OneDrive - Liberty University\CSIS 112\CSIS Assinment 8\ANIMAL\Debug\ANIMAL.exe (process 16532) exited with code 0.
To automatically close the console when debugging stops, enable Tools->Options->Debugging->Automatically close the console when debugging stops.
Press any key to close this window . . .
Needs to look like this:
PHOTO ATTACHED OF WHAT IT SHOULD LOOK LIKE. IT DOES NOT THOUGH
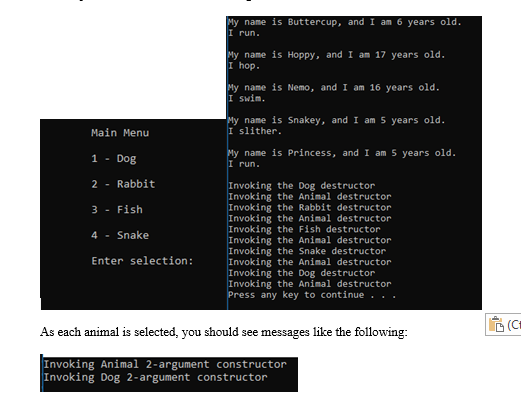

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

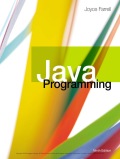
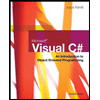
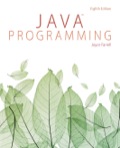
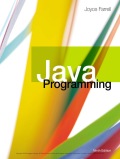
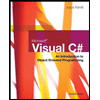
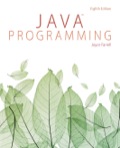