Can anyone please solve this question asap ? Thank yoyu In this question, you are required to implement singly linked list and its concepts to solve the problems of a departmental store described in the scenario given below: A departmental store has a variety of products to sell. Each product type has a unique ID and price associated with it (for example soaps can have an ID of 1 and price of 50 etc.). A customer comes to the store and starts putting items in his/her shopping cart. Once all the items have been placed in the shopping cart, the customer then proceeds to checkout but before that, he/she will sort (in ascending order of IDs) the shopping cart’s items and proceed to remove all the duplicate items from the shopping cart, that is if two soaps have been added to the cart, only 1 will be kept. At the checkout counter, he/she will then remove items from the cart by removing from the end of the shopping cart (linked list). A bill for that customer is then generated and printed. You are required to implement this scenario using singly linked list for a customer named John who has entered the store with the following shopping list: - Chips - Cheese - Chips - Chips - Facewash - Icecream - Chips - Icecream - Chips - Icecream Take the ID and price for these items as specified below. You should hardcode these values inside your initial linked list, user input for these details is not required: - ID for chips: 10 - Price for each packet of chips: 50.00 - ID for cheese: 11 - Price for each pack of cheese: 125.30 - ID for ice cream: 12 - Price for each pack of ice cream: 180.00 - ID for face wash: 13 - Price for each face wash: 320.25 The total cost John would be paying will be: 675.55 (50.00 + 125.30 + 180.00 + 320.25) Implementation guideline: The shopping cart will be a singly linked list with two items being stored at each node (along with a pointer to connect the nodes together, obviously): - ID - Price You are required to make functions for 1. duplicate item removal 2. Printing 3. Addition and Removal of items from the list 4. Sorting in ascending order. Note: If you wish to make any helper functions, you are allowed to do so. Important: Print the state of linked list (shopping cart) after 1. Inserting all items in it. 2. Sorting all items by ID. 3. Removing all duplicate items. 4. Placing each item on the counter. Finally, print the total cost of items that were checked out at the end
Can anyone please solve this question asap ? Thank yoyu
In this question, you are required to implement singly linked list and its concepts to solve the problems of a departmental store described in the scenario given below:
A departmental store has a variety of products to sell. Each product type has a unique ID and price associated with it (for example soaps can have an ID of 1 and price of 50 etc.). A customer comes to the store and starts putting items in his/her shopping cart. Once all the
items have been placed in the shopping cart, the customer then proceeds to checkout but before that, he/she will sort (in ascending order of IDs) the shopping cart’s items and proceed to remove all the duplicate items from the shopping cart, that is if two soaps have been added to the cart, only 1 will be kept. At the checkout counter, he/she will then remove items from the cart by removing from the end of the shopping cart (linked list). A bill for that customer is then generated and printed.
You are required to implement this scenario using singly linked list for a customer named John who has entered the store with the following shopping list:
- Chips
- Cheese
- Chips
- Chips
- Facewash
- Icecream
- Chips
- Icecream
- Chips
- Icecream
Take the ID and price for these items as specified below. You should hardcode these
values inside your initial linked list, user input for these details is not required:
- ID for chips: 10
- Price for each packet of chips: 50.00
- ID for cheese: 11
- Price for each pack of cheese: 125.30
- ID for ice cream: 12
- Price for each pack of ice cream: 180.00
- ID for face wash: 13
- Price for each face wash: 320.25
The total cost John would be paying will be: 675.55 (50.00 + 125.30 + 180.00 + 320.25)
Implementation guideline:
The shopping cart will be a singly linked list with two items being stored at each node (along
with a pointer to connect the nodes together, obviously):
- ID
- Price
You are required to make functions for
1. duplicate item removal
2. Printing
3. Addition and Removal of items from the list
4. Sorting in ascending order.
Note: If you wish to make any helper functions, you are allowed to do so.
Important: Print the state of linked list (shopping cart) after
1. Inserting all items in it.
2. Sorting all items by ID.
3. Removing all duplicate items.
4. Placing each item on the counter.
Finally, print the total cost of items that were checked out at the end

Step by step
Solved in 2 steps with 1 images

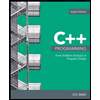
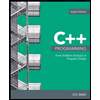