/* Class representing a directed graph using adjacency lists +/ static class Graph { int V; //Number of Vertices LinkedList [] adj; // adjacency lists //Constructor Graph(int V) this.V = V; adj = new LinkedList (V]; for (int i = 0; i < adj.length; i++) adj[i] = new LinkedList(); //To add an edge to graph void addEdge (int v, int w) { adj [v].add (w); // Add w to the list of v.
/* Class representing a directed graph using adjacency lists +/ static class Graph { int V; //Number of Vertices LinkedList [] adj; // adjacency lists //Constructor Graph(int V) this.V = V; adj = new LinkedList (V]; for (int i = 0; i < adj.length; i++) adj[i] = new LinkedList(); //To add an edge to graph void addEdge (int v, int w) { adj [v].add (w); // Add w to the list of v.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Java
Pleasse add Comments and Test-Cases to code.
explore a specific way to perform a Breadth First Search (BFS) of a given Graph [ Figure 1].
![2
4
5
Figure 1: Graph for Traversal
/* Class representing a directed graph using adjacency lists */
static class Graph
int V; //Number of Vertices
LinkedList<Integer> [] adj; // adjacency lists
//Constructor
Graph (int V)
{
this.V = V;
adj - new LinkedList [V];
for (int i = 0; i < adj.length; i++)
adj [i] = new LinkedList<Integer>();
//To add an edge to graph
void addEdge (int v, int w)
{
adj [v].add (w); // Add w to the list of v.
2](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc353cc0f-0c4d-4d5b-a3e6-e746dbb90f94%2Ff4df5066-8b1a-4b48-bdec-02ac6ef5140d%2F5l5grnq_processed.png&w=3840&q=75)
Transcribed Image Text:2
4
5
Figure 1: Graph for Traversal
/* Class representing a directed graph using adjacency lists */
static class Graph
int V; //Number of Vertices
LinkedList<Integer> [] adj; // adjacency lists
//Constructor
Graph (int V)
{
this.V = V;
adj - new LinkedList [V];
for (int i = 0; i < adj.length; i++)
adj [i] = new LinkedList<Integer>();
//To add an edge to graph
void addEdge (int v, int w)
{
adj [v].add (w); // Add w to the list of v.
2
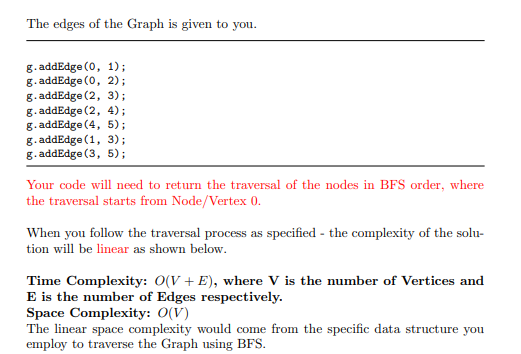
Transcribed Image Text:The edges of the Graph is given to you.
g. addEdge (0, 1);
g. addEdge (0, 2);
g. addEdge (2, 3);
g. addEdge (2, 4);
g. addEdge (4, 5);
g. addEdge (1, 3);
g. addEdge (3, 5);
Your code will need to return the traversal of the nodes in BFS order, where
the traversal starts from Node/Vertex 0.
When you follow the traversal process as specified - the complexity of the solu-
tion will be linear as shown below.
Time Complexity: 0(V + E), where V is the number of Vertices and
E is the number of Edges respectively.
Space Complexity: 0(V)
The linear space complexity would come from the specific data structure you
employ to traverse the Graph using BFS.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
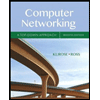
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
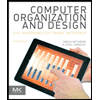
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
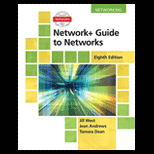
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
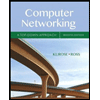
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
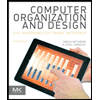
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
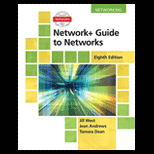
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
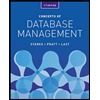
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
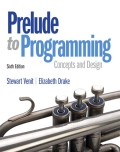
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
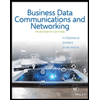
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY