class, so thne test program Make no changes to the test program, but only fix the CarSensor class. changes), to prod
Please check the images for the whole question. Thank you.
/* TestCarSensor.java - program to test the CarSensor class.
*/
public class TestCarSensor
{
public static void main (String[] args)
{
// 1. declare CarSensor objects
CarSensor generic = new CarSensor(); // default sensor, all values "zero"
CarSensor tempCel = new CarSensor("temperature sensor", -50, +300, "C"); // temperature sensor (Celsius)
CarSensor speed = new CarSensor("speed sensor", 0, 200, "km/h"); // speed sensor (kms/hour)
CarSensor speed2 = new CarSensor("speed sensor 2", 0, 200, "m/h"); // speed sensor 2 (miles/hour)
// 2. test changing desc and limits
System.out.println ();
System.out.println ( generic ); // display generic sensor (zero)
generic.setDesc ("special sensor"); // change description
generic.setLimits (-5,5,"units"); // change limits
System.out.println ( generic ); // display generic sensor again
// 3. test displaying object (calling .toString() )
System.out.println ();
System.out.println ( tempCel );
System.out.println ( speed );
System.out.println ( speed2 );
// 4. test setlimits() rule
System.out.println ();
System.out.println ( generic ); // limits at -5 to 5 units
generic.setLimits (10, -10, "blah"); // try to set to 10 to -10 blah
System.out.println ( generic ); // not changed
generic.setLimits (-10, 10, "blah"); // try to set to -10 to 10 blah
System.out.println ( generic ); // changed
generic.setLimits (-5,5,"units"); // set limits back to previous values
// 5. test .equalsLimits()
System.out.println ();
if ( speed.equalsLimits(speed2) ) // test limits if speed sensor = speed 2 sensor -> should be equal
{
System.out.println ( speed + "\n = \n" + speed2 );
}
else
{
System.out.println ( speed + "\n != \n" + speed2 );
}
if ( tempCel.equalsLimits(generic) ) // test limits if tempCel sensor = generic sensor -> should not be equal
{
System.out.println ( tempCel + "\n = \n" + generic );
}
else
{
System.out.println ( tempCel + "\n != \n" + generic );
}
System.out.println ();
// 6. test changing current value
System.out.println ();
speed.setCurrentValue(22); // set current value
System.out.println ( speed ); // is the current value 22?
speed.setCurrentValue(2000); // set current value
System.out.println ( speed ); // is the current value still 22? (unchanged)
System.out.println ();
// 7. test .equals()
System.out.println ();
System.out.println (" speed = speed " + speed.equals(speed) ); // should be true
System.out.println (" speed = speed2 " + speed.equals(speed2) ); // should be false
System.out.println ();
// 8. test changing current value, current value and error, and displaying error range
System.out.println ( tempCel ); // display tempCel
tempCel.setCurrentValue ( 25, 5 ); // change current value and error
System.out.println ( tempCel ); // did the current value and error change?
System.out.println ( tempCel.getErrorDesc() ); // is range: 25 +/- 5 ?
System.out.println ();
}// end of main()
}// end of class
** below is the CarSensor class, or what could be rescued from the damaged .java file
/* CarSensor.java - a class describing any sensor in the "smart" car project.
public class CarSensor
{
// generic car sensor attributes (data)
private String desc="none"; // general description of sensor; simple text
private int limitLow=0; // range of values for sensor; lower limit
private int limitUp=0; // range of values for sensor; upper limit
// rule: limitLow <= limitUp (ensured by setLimits() below)
private String scale = "noscale"; // the "scale" of the sensor (C, F, km, km/h, kg/m, etc.)
private int currVal=0; // current value of sensor
private error=0; // sensor error around current value: +/- error
// rule: limitLow <= currVal <= limitUp
//----------------------
// default constructor - set default values; nothing to do
public CarSensor ()
{
}
// constructor - set attributes to parameter values
private CarSensor (String desc, int low, int upper, String scale)
{
setDesc(desc);
setLimits (low, upper, scale); // set limits and scale, using rule
}
// setDesc() - set the description
public void setDesc(String desc)
{
desc = desc;
}
public void setLimits (int low, int upper, String scale)
{
if (low <= upper) // if rule is true, set lower & upper limits, and scale
{
limitLow = low;
limitUp = upper;
this.scale = scale;
}
}
public void setCurrentValue (int current)
{
setCurrentValue (current, error); // set current, using existing error value
}
public void setCurrentValue (double current, double err)
{
if (limitLow <= current && current <= limitUp) // if rule is true, set current value
{
currVal = current;
error = err;
}
}
public double getCurrentValue ()
{
return currVal;
}
public boolean equals (CarSensor other)
{
return ( this.currVal == other.currVal );
}
public boolean equalsLimits (CarSensor other)
{
return ( this.limitLow == == other.limitUp );
}
getErrorDesc ()
{
return String.format ("error range: %d to %d", (currVal-error), (currVal+error) );
}
public String toString ()
{
String ret = "";
ret += desc;
ret += String.format (" limits: %d to %d %s,", limitLow, limitUp, scale );
ret += String.format (" current value: %f, ", currVal );
ret = getErrorDesc;
return ;
}
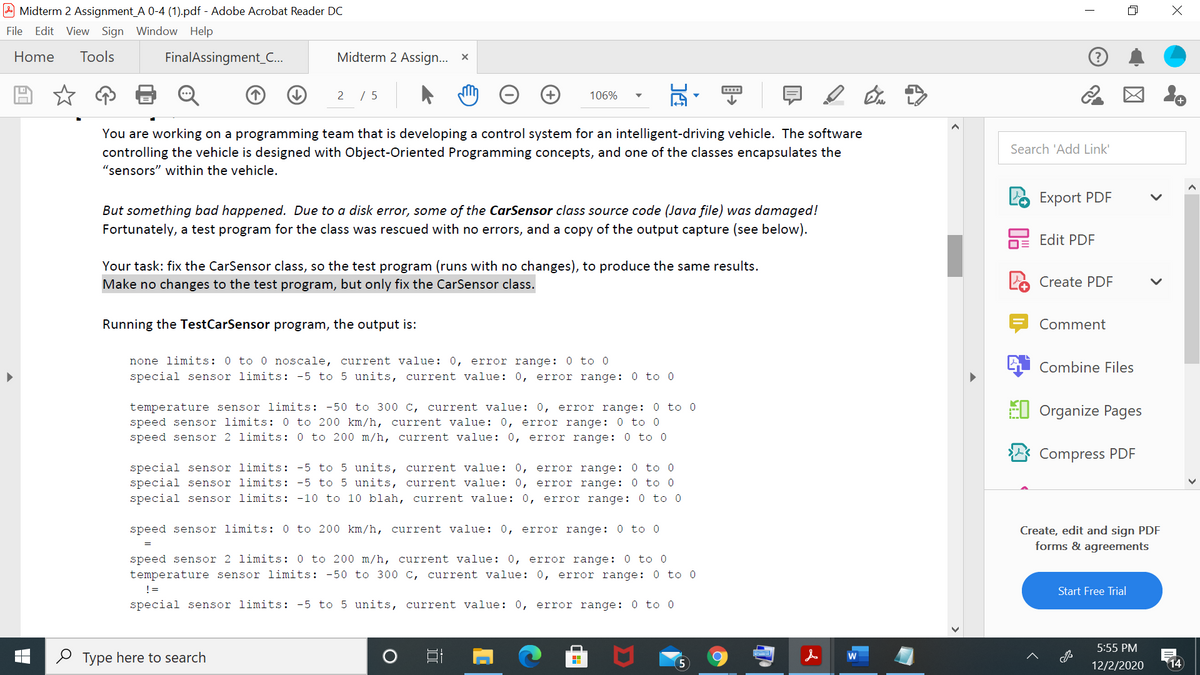
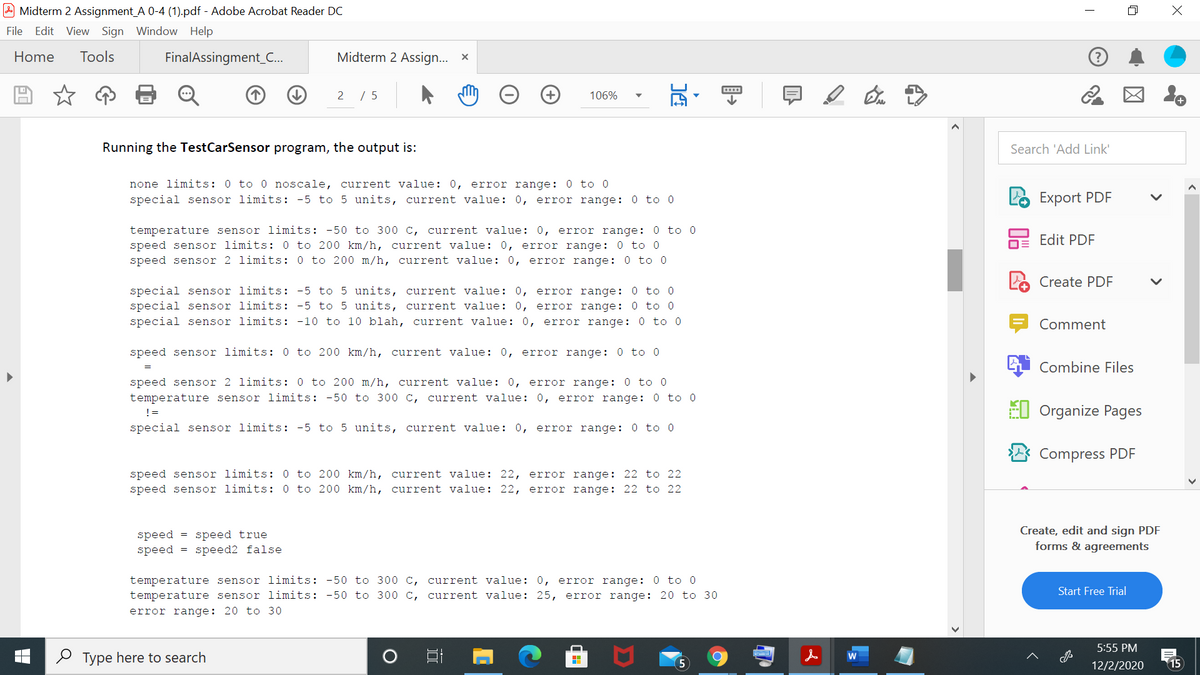

Step by step
Solved in 5 steps with 2 images

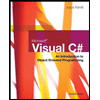
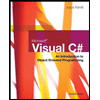