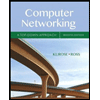
Use this Bird class - i.e. a blueprint for creating Bird objects and using them - to answer questions #1 and #2.
class Bird:
animal_class = 'Aves'
animal_phyla = "Chordata"
lays_eggs = True
def __init__(self, name, species, color):
self.species = species
self.name = name
self.color = color
def get_species(self):
return self.species
def getting_older(self, yrs):
self.age = self_age + yrs
def fly(self):
print("Now I'm flying")
def camouflage(self):
print("Blending in with a " + str(self.color) + " environment")
def sing(self):
print("Tweet Tweet")
Which function call below will define a object of the Bird class with name "Audrey", species "Red Robin", and color "red", assigned to the 'my_bird' variable name?
a) my_bird = Red_Robin("Audrey")
b) my_bird = Red_Robin(Bird("Audrey"))
c) my_bird = Bird("Audrey", "Red Robin", "red")

Step by stepSolved in 2 steps

- Write a member method for the Team class named : calculatePercentage that it will calculate and return the winning percentage of a team. The winning percentage is calculated as follows: wins / ( wins + losses)arrow_forwardWrite a code for a banking program.a) In this question, first, you need to create a Customer class, this class should have:• 2 private attributes: name (String) and balance (double)• Parametrized constructor to initialize the attributes• Methods:i. public String toString() that gives back the name and balanceii. public void addPercentage; this method will take a percentage value andadd it to the balanceb) Second, you will create a driver class and ask the user to enter 6 customers’ informationand then you will create an array of Customer objects.c) Then you use this array used for various operations as shown in the output.• Using the array of customer objects, you need to search for all customers whohave less than $150• Using the array of customer objects, you need to get the average balance of thebalances in this array• Using the array of customer objects, you need to get the customer with thehighest balance and lowest balance• Using the array of customer objects, you need to show all…arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forward
- public class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardclass SavingsAccount(object): RATE = 0.02 def __init__(self, name, pin, balance = 0.0): self._name = name self._pin = pin self._balance = balance def __str__(self): result = 'Name: ' + self._name + '\n' result += 'PIN: ' + self._pin + '\n' result += 'Balance: ' + str(self._balance) return result def __eq__(self,a): if self._name == a._name and self._pin == a._pin and self._balance == a._balance: return True else: return False def __gt__(self,a): if self._balance > a._balance: return True else: return False def getBalance(self): return self._balance def getName(self): return self._name def getPin(self): return self._pin def deposit(self, amount): self._balance += amount return self._balance…arrow_forwardThis is Phython Programmingarrow_forward
- JAVA PROGRAM For this program, you are tasked to implement the Beverage class which has the following private properties: name - a string value volume - this is an integer number which represents its current remaining volume in mL isChilled - this is a boolean field which is set to true if the drink is chilled It should have the following methods: isEmpty() - returns true if the volume is already 0 {toString() - returns the details of the object in the following format: {name} ({volume}mL) {"is still chilled" | "is not chilled anymore"}.Example returned strings: Beer (249mL) is still chilled Water (500mL) is not chilled anymore A constructor method with the following signature: public Beverage(name, volume, isChilled) Getter methods for all the 3 properties. Then, create two final subclasses that inherit from this Beverage class. The first one is the Water class which has the additional private property, type, which is a String and can only be either "Purified", "Regular",…arrow_forwardA field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forwardclass Duration: def __init__(self, hours, minutes): self.hours = hours self.minutes = minutes def __add__(self, other): total_hours = self.hours + other.hours total_minutes = self.minutes + other.minutes if total_minutes >= 60: total_hours += 1 total_minutes -= 60 return Duration(total_hours, total_minutes) first_trip = Duration(3, 36)second_trip = Duration(0, 47) first_time = first_trip + second_tripsecond_time = second_trip + second_trip print(first_time.hours, first_time.minutes) what is the outputarrow_forward
- class Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forwardQuestion 6 Consider: class Bike { } private String color; public Bike() { } public Bike(String newColor) { color = newColor; } public Bike (Bike bike) { color = } bike.color; public String getColor() { } } return color; public void setColor(String newColor) { color = newColor; Bike bike1= new Bike(); = Bike bike2 new Bike("blue"); Bike bike3 = new Bike(bike2); bike2.setColor("green"); Bike bike4 = new Bike(bike2); Which of the following are correct? bike4.getColor() returns null bike4.getColor() return "blue" bike3.getColor() return "green" bike2.getColor() returns "blue" bike4.getColor() return "green" bike1.getColor() returns bike3.getColor() return null bike3.getColor() returns "blue" bike2.getColor() returns "green" bike1.getColor() returns nullarrow_forwardWhen you code a class that implements an interface, you don’t need to implement its ___________ methods.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
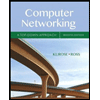
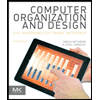
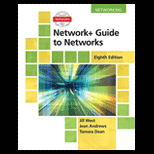
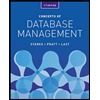
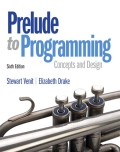
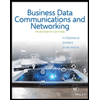