Complete the TicTacToe game we started in class. Specifically, add the following 2 missing aspects (both marked with //TODO in the code): is_draw(board) Determine if the board is in a draw state is_win(board) Determine if the board is in a diagonal win state We already did the row and column checking. Here is code that needs to be fixed: def print_board(board): for row in board: print(row) def get_selection(): user_input = int(input("Enter square number (1-9):\n")) return user_input def convert_selection(selection): selection = selection - 1 row_index = selection // 3 col_index = selection % 3 return (row_index, col_index) def place_mark(board, is_x_turn, position): row, col = position if is_x_turn: marker = "X" else: marker = "O" board[row][col] = marker def is_win(board): winner = None for i in range(3): if board[i][0] == board[i][1] == board[i][2] and board[i][0] != "_": winner = board[i][0] break if board[0][i] == board[1][i] == board[2][i] and board[0][i] != "_": winner = board[0][i] break if board[0][0] == board[1][1] == board[2][2] and board[1][1] != "_": winner = board[1][1] if winner is not None: print_board(board) print(f"{winner} is the winner!!!") return True return False def is_draw(board): for row in board: if "_" in row: return False print_board(board) print("Its a draw!") return True def main(): board = [ ["_", "_", "_"], ["_", "_", "_"], ["_", "_", "_"] ] is_x_turn = True is_game_over = False while not is_game_over: print_board(board) selection = get_selection() position = convert_selection(selection) place_mark(board, is_x_turn, position) is_game_over = is_win(board) or is_draw(board) is_x_turn = not is_x_turn if __name__ == "__main__": main()
Complete the TicTacToe game we started in class. Specifically, add the following 2 missing aspects (both marked with //TODO in the code):
-
is_draw(board)
- Determine if the board is in a draw state
-
is_win(board)
- Determine if the board is in a diagonal win state
- We already did the row and column checking.
Here is code that needs to be fixed:
def print_board(board):
for row in board:
print(row)
def get_selection():
user_input = int(input("Enter square number (1-9):\n"))
return user_input
def convert_selection(selection):
selection = selection - 1
row_index = selection // 3
col_index = selection % 3
return (row_index, col_index)
def place_mark(board, is_x_turn, position):
row, col = position
if is_x_turn:
marker = "X"
else:
marker = "O"
board[row][col] = marker
def is_win(board):
winner = None
for i in range(3):
if board[i][0] == board[i][1] == board[i][2] and board[i][0] != "_":
winner = board[i][0]
break
if board[0][i] == board[1][i] == board[2][i] and board[0][i] != "_":
winner = board[0][i]
break
if board[0][0] == board[1][1] == board[2][2] and board[1][1] != "_":
winner = board[1][1]
if winner is not None:
print_board(board)
print(f"{winner} is the winner!!!")
return True
return False
def is_draw(board):
for row in board:
if "_" in row:
return False
print_board(board)
print("Its a draw!")
return True
def main():
board = [ ["_", "_", "_"],
["_", "_", "_"],
["_", "_", "_"] ]
is_x_turn = True
is_game_over = False
while not is_game_over:
print_board(board)
selection = get_selection()
position = convert_selection(selection)
place_mark(board, is_x_turn, position)
is_game_over = is_win(board) or is_draw(board)
is_x_turn = not is_x_turn
if __name__ == "__main__":
main()
Code is in Python

Step by step
Solved in 2 steps with 1 images

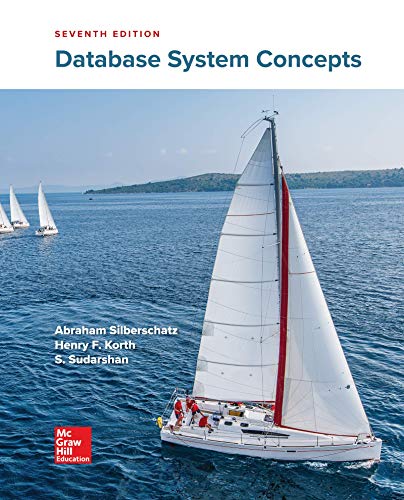
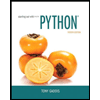
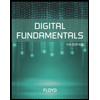
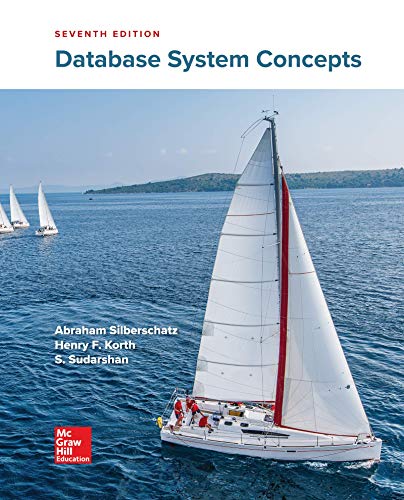
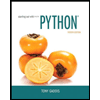
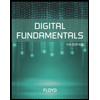
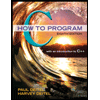
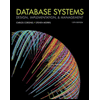
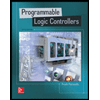