Complete this code using Python m1 = [] #Matrix 1 m2 = [] #Matrix 2 #Write a function that will return the addition of Matrix A and B. #Create a new matrix C that will hold the addtion result of Matrix A and B (A+B). #Return the resultant matrix C def addMatrix(A,B): #Write your code here #Write a function that will return the subtraction of Matrix B from A. #Create a new matrix C that will hold the substraction result of Matrix B from A (A-B). #Return the resultant matrix C def subsMatrix(A,B): #Write your code here #Write a function that will return the multiplication of Matrix A and B. #Create a new matrix C that will hold the multiplication result of Matrix A and B (A*B). #Keep in mind,in order to perform matrix multiplication, the number of columns in Matrix A must be equal to the number of columns in Matrix B. #Return the resultant matrix C def multipyMatrix(A,B): #Write your code here #Write a function that will transform matrix A to the transpose of matrix A. #The transpose of a matrix means the rows of the matrix will become the columns of the matrix, the columns will become rows of the matrix def transpose(A): #Write your code here def takeRowColInput(): row = int(input("Please enter the number of rows in the matrix:")) col = int(input("Please enter the number of columns in the matrix:")) matrixInitialization(m1,row,col) matrixInitialization(m2,row,col) printMatrix(m1) printMatrix(m2) def matrixInitialization(m,row,col): for r in range(row): l=[] for c in range(col): l.append(0) m.append(l) def printMatrix(m): for row in range(len(m)): print("| ",end="") for col in range(len(m[row])): print(m[row][col], end =" ") print("|") print() def takeMatrixInput(m): for row in range(len(m)): for col in range(len(m[row])): m[row][col] = int(input(f"Enter value for [{row},{col}] : ")) printMatrix(m) takeRowColInput() takeMatrixInput(m1) takeMatrixInput(m2) addRes = addMatrix(m1,m2) #m1+m2 printMatrix(addRes) subRes = subsMatrix(m1,m2) #m1-m2 printMatrix(subRes) mulRes = multipyMatrix(m1,m2) #m1*m2 print(mulRes) transpose(m1) printMatrix(m1) transpose(m2) printMatrix(m2)
Complete this code using Python
m1 = [] #Matrix 1
m2 = [] #Matrix 2
#Write a function that will return the addition of Matrix A and B.
#Create a new matrix C that will hold the addtion result of Matrix A and B (A+B).
#Return the resultant matrix C
def addMatrix(A,B):
#Write your code here
#Write a function that will return the subtraction of Matrix B from A.
#Create a new matrix C that will hold the substraction result of Matrix B from A (A-B).
#Return the resultant matrix C
def subsMatrix(A,B):
#Write your code here
#Write a function that will return the multiplication of Matrix A and B.
#Create a new matrix C that will hold the multiplication result of Matrix A and B (A*B).
#Keep in mind,in order to perform matrix multiplication, the number of columns in Matrix A must be equal to the number of columns in Matrix B.
#Return the resultant matrix C
def multipyMatrix(A,B):
#Write your code here
#Write a function that will transform matrix A to the transpose of matrix A.
#The transpose of a matrix means the rows of the matrix will become the columns of the matrix, the columns will become rows of the matrix
def transpose(A):
#Write your code here
def takeRowColInput():
row = int(input("Please enter the number of rows in the matrix:"))
col = int(input("Please enter the number of columns in the matrix:"))
matrixInitialization(m1,row,col)
matrixInitialization(m2,row,col)
printMatrix(m1)
printMatrix(m2)
def matrixInitialization(m,row,col):
for r in range(row):
l=[]
for c in range(col):
l.append(0)
m.append(l)
def printMatrix(m):
for row in range(len(m)):
print("| ",end="")
for col in range(len(m[row])):
print(m[row][col], end =" ")
print("|")
print()
def takeMatrixInput(m):
for row in range(len(m)):
for col in range(len(m[row])):
m[row][col] = int(input(f"Enter value for [{row},{col}] : "))
printMatrix(m)
takeRowColInput()
takeMatrixInput(m1)
takeMatrixInput(m2)
addRes = addMatrix(m1,m2) #m1+m2
printMatrix(addRes)
subRes = subsMatrix(m1,m2) #m1-m2
printMatrix(subRes)
mulRes = multipyMatrix(m1,m2) #m1*m2
print(mulRes)
transpose(m1)
printMatrix(m1)
transpose(m2)
printMatrix(m2)

Step by step
Solved in 3 steps with 2 images

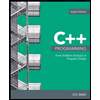
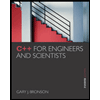
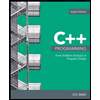
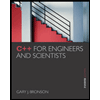