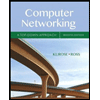
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
please complete following c++ code question
![Computation
a. Write a function countBits(int) that counts the number of bits that are 1. For example
countBits(9) should return 2 because 9 = 1001 while countBits(16) should return 1
(10000 has one 1-bit).
b. Write a function polynomial that takes an array of double, a length, and x as shown:
double c[] = {3.0, 1.5, 1.0, -2.5}:
double y = polynomial(c, 4, 2.0):
should compute the polynomial 3.0x + 1.5x² + 1.0x - 2.5
Note that the efficient way to do this is Horner's form:
((3.0 * x + 1.5) * x + 1.0) * x - 2.5
You must use a loop, not hardcode it. Your code should work for:
double c[] = {5.0, 1.0, 2.0, 3.5, 5.2};
cout << polynomial(c, 5, 2.2);
c. Given the following 2-dimensional matrix, write loops to print out the sum of every row
then column:
constexpr int rows = 3, cols = 4;
double x[rows][cols] = {
{1, 2, 3.5},
{2, -1, 1.5}
}:
Your loop should compute 1+2+3.5 = 6.5 and 2-1+1.5 = 2.5 for the two rows, then for the
columns: 1+2 = 3, 2-1 = 1, and 3.5+1.5 = 5.
The output of the program should be:
6.5 2.5
315
d. Write a template function that sums and prints out the arrays in the following main:
int main() {
string a[] = {"yo", "ho"};
int b[] = {1, 2, 3};
cout << sum(a, 2) << \n';
cout << sum(b, 3) << \n':](https://content.bartleby.com/qna-images/question/bcfb8dea-921b-49cc-9763-7233db60feda/fba90f36-ba7f-448f-b515-b67978800de9/q6kco6t_thumbnail.png)
Transcribed Image Text:Computation
a. Write a function countBits(int) that counts the number of bits that are 1. For example
countBits(9) should return 2 because 9 = 1001 while countBits(16) should return 1
(10000 has one 1-bit).
b. Write a function polynomial that takes an array of double, a length, and x as shown:
double c[] = {3.0, 1.5, 1.0, -2.5}:
double y = polynomial(c, 4, 2.0):
should compute the polynomial 3.0x + 1.5x² + 1.0x - 2.5
Note that the efficient way to do this is Horner's form:
((3.0 * x + 1.5) * x + 1.0) * x - 2.5
You must use a loop, not hardcode it. Your code should work for:
double c[] = {5.0, 1.0, 2.0, 3.5, 5.2};
cout << polynomial(c, 5, 2.2);
c. Given the following 2-dimensional matrix, write loops to print out the sum of every row
then column:
constexpr int rows = 3, cols = 4;
double x[rows][cols] = {
{1, 2, 3.5},
{2, -1, 1.5}
}:
Your loop should compute 1+2+3.5 = 6.5 and 2-1+1.5 = 2.5 for the two rows, then for the
columns: 1+2 = 3, 2-1 = 1, and 3.5+1.5 = 5.
The output of the program should be:
6.5 2.5
315
d. Write a template function that sums and prints out the arrays in the following main:
int main() {
string a[] = {"yo", "ho"};
int b[] = {1, 2, 3};
cout << sum(a, 2) << \n';
cout << sum(b, 3) << \n':
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Similar questions
- Complete the C++ function given below: bool check_anagram(string s1, string s2){ I/returns true if the strings s1, s2 are anagrams, else returns false }arrow_forwardWhich of the following is a reason that one might use an enumeration instead of an integer in C++17? Pick ONE option Enumerations can only take a discrete set of values, as opposed to the fully numerical range of an integer Enumerations provide a layer of type-safety not available with integers The names of all enumerators are automatically scoped to their enumeration, so you can define different "global constants" with the same name Enumerators are more efficient to hash for use as the key in associative containers Enumerators can be used as non-type template parameters, whereas integers cannot bearrow_forwardC++ code please Suppose that the enum flowerType is defined Write a C++ function that can be used to input value in a variable of type flowerType.arrow_forward
- C++ Can a variable name be passed to a value parameter in C++ functions?arrow_forwardSummary In this lab, you complete a C++ program with the provided data files. The program calculates the amount of tax withheld from an employee’s weekly salary, the tax deduction to which the employee is entitled for each dependent, and the employee’s take- home pay. The program output includes state tax withheld, federal tax withheld, dependent tax deductions, salary, and take-home pay. Instructions Ensure the source code file named Payroll.cpp is open in the code editor. Variables have been declared and initialized for you as needed, and the input and output statements have been written. Read the code carefully before you proceed to the next step. Write the C++ code needed to perform the following: Calculate state withholding tax (stateTax) at 6.5 percent Calculate federal withholding tax (federalTax) at 28.0 percent. Calculate dependent deductions (dependentDeduction) at 2.5 percent of the employee’s salary for each dependent. Calculate total withholding (totalWithholding)…arrow_forwardc++ This program prompts the user to enter three distinct int type values and display the maximum, median, and minimum. Your program does not need to check the uniqueness of the inputs. No additional library/package is allowed.arrow_forward
- Code in C++ please, with comments to guide the code.arrow_forwardWrite a C code. You should use scanf() and use printf to show the values that you have assigned. The structure type must have following information:Name of structure: city_pComponents of structure:Name (name of the city)Population (number of people in the city)In_California (is the city inside California or not)Speed_limit (usual speed limit inside the city)arrow_forwardCan I please get help with this program?arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
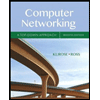
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
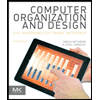
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
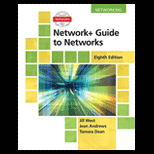
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
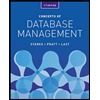
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
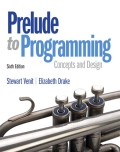
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
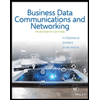
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY