Consider the following program. #include using namespace std; void summer(int&, int); void fall(int, int&); int x; int main() { int intNum1 = 2; int intNum2 = 5; x = 6; summer(intNum1, intNum2); cout << intNum1 << " " << intNum2 << " " << x << endl; fall(intNum1, intNum2); cout << intNum1 << " " << intNum2 << " " << x << endl; return 0; } void summer(int& a, int b) { int intNum1; intNum1 = b + 12; a = 2 * b + 5; b = intNum1 + 4; } void fall(int u, int& v) { int intNum2; intNum2 = x; v = intNum2 * 4; x = u - v; } Answer the following questions: a. What is the output? Consider variable scope. b. Considering the function summer, parameter 1 is called by reference. What is passed into the function for parameter 1, i.e., what value does parameter 1 receive? c. Again, considering the function summer, parameter 2 is called by value. What occurs in memory for parameter 2 and local variable int intNum1? Hint: consider memory, parameters and local variables
2. Consider the following program.
#include <iostream>
using namespace std;
void summer(int&, int);
void fall(int, int&);
int x;
int main() {
int intNum1 = 2;
int intNum2 = 5;
x = 6;
summer(intNum1, intNum2);
cout << intNum1 << " " << intNum2 << " " << x << endl;
fall(intNum1, intNum2);
cout << intNum1 << " " << intNum2 << " " << x << endl;
return 0;
}
void summer(int& a, int b)
{
int intNum1;
intNum1 = b + 12;
a = 2 * b + 5;
b = intNum1 + 4;
}
void fall(int u, int& v)
{
int intNum2;
intNum2 = x;
v = intNum2 * 4;
x = u - v;
}
Answer the following questions:
a. What is the output? Consider variable scope.
b. Considering the function summer, parameter 1 is called by reference. What is passed into the function for parameter 1, i.e., what value does parameter 1 receive?
c. Again, considering the function summer, parameter 2 is called by value. What occurs in memory for parameter 2 and local variable int intNum1? Hint: consider memory, parameters and local variables

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

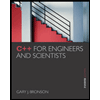
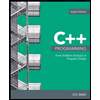
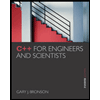
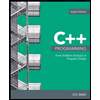