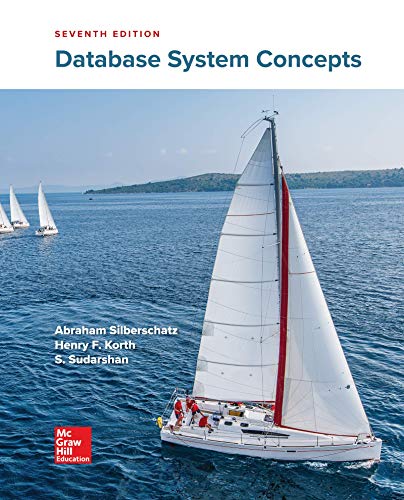
COSC 1336 –
Program 12 – Classes and Object-Oriented Programming
The owners of the Annan Supermarket would like to have a program that computes the weekly gross pay of their employees. The user will enter an employee’s first name, last name, the hourly rate of pay, and the number of hours worked for the week. In addition, Annan Supermarkets would like the program to compute the employee’s net pay and overtime pay. Overtime hours, any hours over 40, are paid at 1.5 the regular hourly rate. Net pay is gross pay minus taxes (Refer to the tax table on the attached image).
Define a class called Employee. The class must have private attributes to store the employee's name, hourly rate, and regular (≤ 40) and overtime hours worked. The class must also have methods to perform the following tasks:
• A constructor to initialize the hourly rate to the minimum wage of $7.25 per hour
and the hours worked (regular and overtime) to 0.0.
• A method to get (a setter/mutator method)
⬧ the employee's name
⬧ the hourly rate
⬧ the hours work for the month (by the week – assume 4 weeks in a month).
Do not write separate setters for regular and overtime hours.
• A method to return (a getter/accessor method)
⬧ the employee's name
⬧ the hourly rate
⬧ the total regular hours work for the month
⬧ the total overtime hours for the month
• A method to return (a getter/accessor method)
⬧ the monthly regular pay
• A method to return (a getter/accessor method)
⬧ the monthly overtime pay
• A __str__ method to display the output which must include the following
information:
⬧ Employee's name
⬧ Total regular hours worked
⬧ Total overtime hours worked
⬧ Total hours worked
⬧ Pay rate
⬧ Monthly Regular Pay
⬧ Monthly overtime pay
⬧ Monthly gross pay
⬧ Monthly taxes
⬧ Monthly net pay
Write a main function that declares an object for the class defined and test the functions
and methods written for the class. Allow the user to run the program as many times as possible. No input, processing, or output should happen in the main function. All work should be delegated to other functions. Include the recommended minimum documentation for each function.
Run your program three times with the data provided in the image.
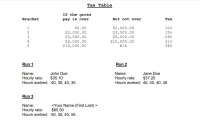

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

- def area(side1, side2): return side1 * side2s1 = 12s2 = 6Identify the statements that correctly call the area function. Select ALL that apply. Question options: area(s1,s2) answer = area(s1,s2) print(f'The area is {area(s1,s2)}') result = area(side1,side2)arrow_forwardObject-Oriented Programming ❤❤❤ Matchmaker with Java ❤❤❤ Summary: Create a Java application that will determine your true love. Prerequisites: Java, VS Code, and Terminal In this assignment you will develop and test a command-line application written in the Java language that asks questions to determine if a person is your true love. More specifically your application should ask five questions in the form of statements and allow the user to respond to each statement with the numbers 1 through 5 with 1 indicating strongly disagree and 5 indicating strongly agree. You will then compare the person’s answers with your desired “true love” answers. The closer the answers are to your desired “true love” answers the better match the two of you are for each other. For example, suppose you choose the statement “Broccoli is delicious.” and your desired answer was 1 (strongly disagree) because you really don’t like broccoli. If the application user entered 4 (agree), then the two of you would not…arrow_forwardDon't use AI.arrow_forward
- In Java, the assignment operator is ______ and the relational operator to determine if two primitive data types are equal is _____arrow_forwardText-based adventure game: Pretend you are creating a text-based adventure game. At different points in the game, you want the user to select to fight, run, or hide from certain enemies. Modify the application below (week3.py) so that the selection variable is sent as an argument into the choice() function. The user should enter 1 to fight, 2 to run, or 3 to hide in the main(). The choice() function should print one of the three options. You will need to add an if statement in the choice() function to make the correct selection.arrow_forward(Financial application: payroll) Write a program that reads the following infor- mation and prints a payroll statement: Employee's name (e.g., Smith) Number of hours worked in a week (e.g., 10) Hourly pay rate (e.g., 9.75) Federal tax withholding rate (e.g., 20%) State tax withholding rate (e.g., 9%) A sample run is shown below: Enter employee's name: Smith Enter number of hours worked in a week: 10 Enter -Enter Enter hourly pay rate: 9.75 Enter federal tax withholding rate: 0.20 -Enter -Enter Enter state tax withholding rate: 0.09 Enter Employee Name: Smitharrow_forward
- 1. Retail Price CalculatorWrite an application that accepts from the user the wholesale cost of an item and its markup percentage. (For example, if an item’s wholesale cost is $5 and its retail price is $10, then the markup is 100%.)The program should contain a function named CalculateRetail that receives the wholesale cost and markup percentage as arguments, and returns the retail price of the item. The application’s form should look something like the one shown in Figure 6-22.arrow_forwardPlease written by computer source Unit 4 - Lab – Individual Retirement Account Calculation Assignment An Individual Retirement Account (IRA) is a special bank / brokerage account that a person can use to save money for retirement. An individual can put up to $5,500 per year tax free and the money grows tax free until the person is ready to retire. The tax rules related to IRA’s are complex, but for this assignment, we’ll assume that the person puts in the same amount of money every year (up to $5,500) and the money grows at a constant interest rate. Write a Flowgorithm program that asks the user to input an annual IRA contribution (in dollars), and interest rate (percent), and a time period (years). Calculate the value of the IRA every year for the duration of the time period provided by the user. Be sure and provide user friendly prompts. Include a comment at the beginning of the program with your name, date, and a short description of the program.arrow_forwardstructions: Write the following programs in C# using concepts learnt in this chapter and abmit the .cs file with the screenshot of your output for each question in the Lab Assignment 2 Submission page. 1. FRENCH TRANSLATOR Look at the following list of French words and their meanings: In French: gauche milieu droite middle In English: right left Create an application that translates the French words to English. The form should have three buttons, one for each French word. When the user clicks a button, the application should display the English translation in a Label control.arrow_forward
- tranlate source program in the picture into quadruples tranlate source program in the picture into quadruples tranlate source program in the picture into quadruplesarrow_forwardMadLib Game bigGame.py Write a program that plays a MadLib game with the user. The program should ask the user to enter the following words: A plural noun (PNOUN1) A person’s first name (FNAME) A noun (NOUN1) A person’s last name (LNAME) A second plural noun (PNOUN2) A place (PLACE1) A third plural noun (PNOUN3) Another place (PLACE2) A fourth plural noun (PNOUN4) A second noun (NOUN2) An adjective (ADJECTIVE1) A second adjective (ADJECTIVE2) A verb (VERB) A third adjective (ADJECTIVE3) After the user has entered these items, the program should display the following story, inserting the user’s input into the appropriate locations: Hello there, sports PNOUN1!This is FNAME, talking to you from the press NOUN1in LNAME Stadium, where 57,000 cheering PNOUN2Have gathered to watch (the) PLACE1 PNOUN3take on (the) PLACE2 PNOUN4.Even though the NOUN2 is shining, it’s a/an ADJECTIVE1cold day with the temperature in the ADJECTIVE2 20s.We’ll be back for the opening VERB -off after a few…arrow_forwardThe program must include two classesclGradesGraph and GradesGrapghDemo. GradesGraphDemo (one method)- Main method only GradesGraph- Include at least 4 methods (No credits without at least 4 methods!!!) Create a class that represents the grade distribution for a given course. In this class you should write methods to perform the following tasks: Read the number of each of the letter grades A, B, C D and F Set the number of letter grades A, B, C, D and F Return the total number of grades Return the percentage of each letter grade as a whole number between 0 and 100 inclusive Draw a bar graph of the grade distributionThe graph should have five bars, one per grade. Each bar can be a horizontal row of asterisks, such that the number of asterisks in a row is proportionate to the percentage of grades in each category. For example, let on asterisk represent 2%, so 50 asterisks correspond to 100%. Mark the horizontal axis at 10% increments from 0 to 100% and label each line with a letter…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
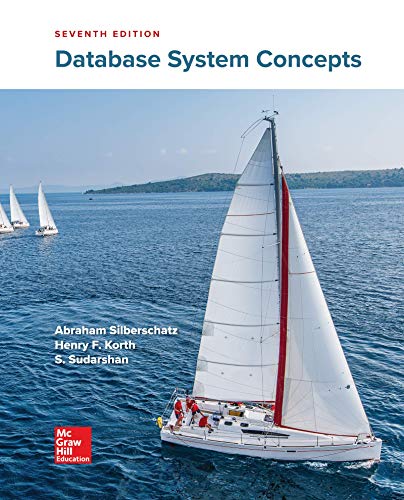
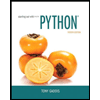
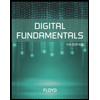
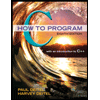
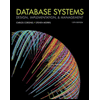
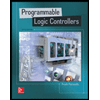