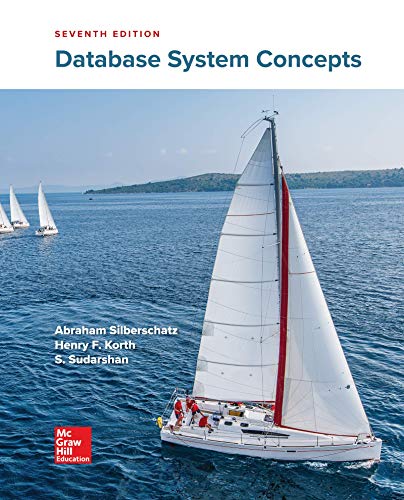
Concept explainers
create a file in c++.
Download the attached CreateRandomNumbersFile.cpp file, open it in Dev C++, and then compile and run it. The program should generate a file called "numbers_lastname.txt" in the same folder as the program (replace lastname with your last name).
Write a program that asks for the name of an input file. Then, read all the numbers in the file, and display the following information to the screen:
- name of the input file
- count of numbers in the file
- sum of all numbers in the file
- average of all numbers in the file (to 2 decimal places)
- count of numbers in each range (100-199, 200-299, 300-399, etc.)
The program should:
- display a hello message
- ask the user for an input file
- display the name of the input file
- display statistical information as shown above
- display a goodbye message
create random numbers:
/* This program will ask the user for their last name, which will be used for
* naming an output file. The output file will consist of 500-999 random
* integers, all between 100-999.
*
* COSC-1436 Fundamentals of
* Author: Richard Herschede
* Date: 7/23/2019
*/
//LIBRARIES
#include <iostream> //for input/output
#include <string> //for string functions
#include <fstream> //for files
#include <cstdlib> //for rand() and srand()
#include <ctime> //for system time
using namespace std;
//GLOBAL CONSTANTS
const int MAX_COUNT = 999; //maximum count of numbers to generate
const int MIN_COUNT = 500; //minimum count of numbers to generate
const int MAX_NUM = 999; //maximum value of random number
const int MIN_NUM = 100; //minimum value of random number
//MAIN FUNCTION
int main()
{
//hello
cout << "This program will generate 500-999 random numbers, and write" << endl;
cout << "them to a file. The user's last name will determine the name" << endl;
cout << "of the output file. Each random number will be between 100-999." << endl << endl;
//define local variables
ofstream outfile; //output file stream
string username, //user's last name
filename = "numbers_"; //name of output file
int num, //variable to hold random number
count; //variable to hold count of numbers
//get system time and seed random number generator
unsigned seed = time(0);
srand(seed);
//set file name for output file
cout << "Please enter your last name: ";
getline(cin,username);
filename += username + ".txt";
//open the output file (will create new or overwrite existing)
outfile.open(filename);
//get count of random numbers to generate
count = (rand() % (MAX_COUNT - MIN_COUNT + 1)) + MIN_COUNT;
cout << "Generating " << count << " random numbers to file " << filename << endl;
//generate random numbers and write them to output file
for (int i = 0; i < count; i++)
{
num = (rand() % (MAX_NUM - MIN_NUM + 1)) + MIN_NUM;
outfile << num << " ";
} //end for
//close the output file
outfile.close();
//goodbye
cout << "\nProgram complete. The output file, " << filename << ", is located" << endl;
cout << "in the same directory as this program." << endl;
return 0;
} //end main()

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

- Create a new file (in Dev C++) In Lab 2, you created a menu for a simple calculator program. In Lab 6, you added some functionality based on the user selection. In this lab, you will add some more functionality to the program. Use loops to keep running the program until the user chooses the exit condition (9). When dividing, use a loop to validate user input, making sure the denominator (second number) is not zero. If the user enters a 0 for the second number, display an error message and keep prompting until a non-zero number is entered. 1) Add two numbers 2) Subtract two numbers 3) Multiply two numbers 4) Divide two numbers 9) Exit program The program should: contain header comments as shown in class display a hello message before presenting the menu display the menu prompt user for selection echo the selection back to the user if the selection was invalid, display error message to user and then loop back to menu if the selection was valid, prompt the user for two numbers…arrow_forwardin C++arrow_forwardWrite a C++ program that reads first name and last name from two txt files, input1.txt and input2.txt respectively and stores them in two separate arrays. Then merges these two arrays into another txt file output.txt. Example: Intput1.txt: Markus Input2.txt: Stocker Output.txt: Markus Stockerarrow_forward
- in C++ Write a short program to open a file called 'books.txt', read its contents into memory, and write them back out to the end of 'books.txt'. Words beginning with 'd' should be left out (not written out).arrow_forwardPython PLEASEEEEEEEE.arrow_forwardMake the following modifications for the solution above: name the output/input file "myRandomNumbers.txt" use a loop to output 17 random numbers to the file after creating the file, close it open the file for input and read the contents and then total the numbers in the file determine the average of the numbers in the file determine the highest and lowest numbers in the file DO NOT USE PYTHON BUILTIN FUNCTIONS TO CALCULATE THE AVERAGE OR THE HIGHEST AND LOWEST NUMBERS an expected number of modules/functions would be in the 5-8 range Display your results: the numbers generated on a single horizontal line the calculated total and average the highest and lowest number in the file Be sure to use clear prompts/labeling for input and output.arrow_forward
- python please Do not need create the file"summer.txt", just need steps.arrow_forwardPython programming language Write a program that asks the user for a number. Write the number to a file called total.txt. Then ask the user for a second number. Write the new number on line 2 with the total for both numbers next to it separated by a comma. Then ask the user for a third number and do the same. Keep asking for numbers until the person puts in a zero to terminate the program. Close the file. Be sure to use at least 2 functions [main() and total_numbers(number, total)]. Put all inputs and outputs into the main function, with all calculations into the total_numbers function. Give me a number: 13 Give me another number: 14 14 + 13 = 27 Give me another number: 3 3 + 27 = 30 Give me another number: 4 4 + 30 = 34 Give me another number (0 for quit): 0 Your total is 34. Write a program that displays the lines from the total.txt file in the following output. Use a try catch phrase to check for errors. Use only one function for this portion [main()]. 13 14, 27 3,…arrow_forwardCan you help me write a C++ Program that does the following: Create a program that reads a file containing a list of songs andprints the songs to the screen one at a time. After each song is printed,except for the last song, the program asks the user to press enter for more.After the last song, the program should say that this was the last song andquit. If there were no songs in the file to begin with, the program shouldsay that there are no songs to show and quit.The program should begin by asking the user for the name of the input file.Each song consists of a title, artist and year.In the file, each song is given on three consecutive lines.Create this program using the latest version of the class Song you created. The latest version of the class song I created is below: #include <iostream>#include <string>using namespace std;class Song {private: string Title; string Artist; int Year;public: Song() : Title("invalid"), Artist("invalid"), Year(-1) {} Song(const string…arrow_forward
- The objective of this problem is to show that you can write a program that reads a text file and uses string methods to manipulate the text. File cart.txt contains shopping cart type data from golfsmith.com representing the gifts you have purchased for your dad for this past Fathers Day. Good for you. You have been very generous! The first item in each row of the file is the part number, the second the quantity, the third the price and the fourth the description. Your program will read the file line by line and compute the total cost of all of the items in the list. You will then display a message that looks like this, assuming the cost of each item adds up to 168.42: Here's what your output should look like: The total price for the awesome Fathers Day gifts you bought is $168.42. The shopping cart can be found here: cart.txt. Right click on the link and save the file to the folder where you have your Python files.arrow_forwardThis is a C++ program, to be done in a .cpp file Problem Statement: Write rules for the user on how to play the game in a text file. Then, create a program that will read in the text file and display it out to the user. LCR Game Rules: The Dice● There are three dice rolled each turn. Each die has the letters L, C, and R on it along with dots on the remaining three sides. These dice determine where the player’s chips will go.○ For each L, the player must pass one chip to the player sitting to the left.○ For each R, the player must pass one chip to the player sitting to the right.○ For each C, the player must place one chip into the center pot and those chips are now out of play.○ Dots are neutral and require no action to be taken for that die.The Chips● Each player will start with three chips.● If a player only has one chip, he/she rolls only one die. If a player has two chips left, he/she rolls two dice. Once a player is out of chips, he/she is still in the game (as he/she may get…arrow_forwardThis is for Python version 3.8.5 and please include pseudocode. A file named gang.txt is shown below. Write Python code that opens this file, reads only the first two lines, and prints “Penny is 19” gang.txt (contents of file) - please create on with the data provided in order to complete this question. Thank you! Penny 19 Kenny 20 Benny 21 Jenny 20arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
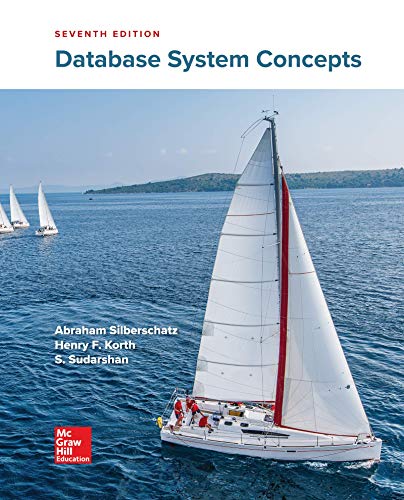
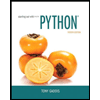
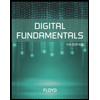
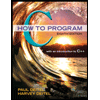
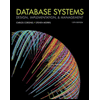
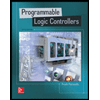